Classification:
-
Insertion sort (direct insertion sort, Hill sort)
-
Exchange sort (bubble sort, quick sort)
-
Selection sort (direct selection sort, heap sort)
-
merge sort
-
Allocation sort (radix sort)
-
Needs the most auxiliary space: merge sort
-
Minimal auxiliary space required: heapsort
-
Fastest on average: Quicksort
-
Unstable: Quick Sort, Hill Sort, Heap Sort.
5. Bubble sort
Basic idea: In a set of numbers to be sorted, compare and adjust two adjacent numbers from top to bottom for all the numbers in the range that are not yet sorted, and let the larger number go down Sink, and the smaller ones go up. That is: whenever two adjacent numbers are compared and found that their sorting is opposite to the sorting requirement, they are swapped.
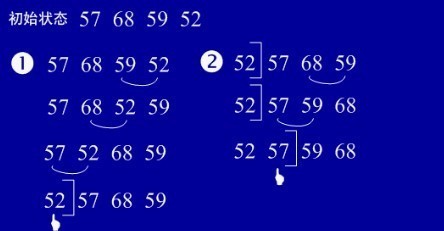
1 public static void bubbleSort(int[] array) {
2 int temp = 0;
3 for (int i = 0; i < array.length - 1; i++) {
4 for (int j = 0; j < array.length - 1 - i; j++) {
5 if (array[j] > array[j + 1]) {
6 temp = array[j];
7 array[j] = array[j + 1];
8 array[j + 1] = temp;
9 }
10 }
11 }
12 System.out.println(Arrays.toString(array) + " bubbleSort");
13 }
6. Quick Sort
Basic idea: select a reference element, usually the first element or the last element, and divide the column to be sorted into two parts through a scan, one part is smaller than the reference element, and the other part is greater than or equal to the reference element. At this time, the reference element is in its The correct position after sorting, and then use the same method to recursively sort the two parts of the division.
1 public static void quickSort(int[] array) {
2 _quickSort(array, 0, array.length - 1);
3 System.out.println(Arrays.toString(array) + " quickSort");
4 }
5
6
7 private static int getMiddle(int[] list, int low, int high) {
8 int tmp = list[low]; //数组的第一个作为中轴
9 while (low < high) {
10 while (low < high && list[high] >= tmp) {
11 high--;
12 }
13
14
15 list[low] = list[high]; //比中轴小的记录移到低端
16 while (low < high && list[low] <= tmp) {
17 low++;
18 }
19
20
21 list[high] = list[low]; //比中轴大的记录移到高端
22 }
23 list[low] = tmp; //中轴记录到尾
24 return low; //返回中轴的位置
25 }
26
27
28 private static void _quickSort(int[] list, int low, int high) {
29 if (low < high) {
30 int middle = getMiddle(list, low, high); //将list数组进行一分为二
31 _quickSort(list, low, middle - 1); //对低字表进行递归排序
32 _quickSort(list, middle + 1, high); //对高字表进行递归排序
33 }
34 }
7. Merge sort
Basic sorting: The Merge sorting method is to merge two (or more than two) ordered lists into a new ordered list, that is, to divide the sequence to be sorted into several subsequences, and each subsequence is ordered. Then the ordered subsequences are merged into an overall ordered sequence.
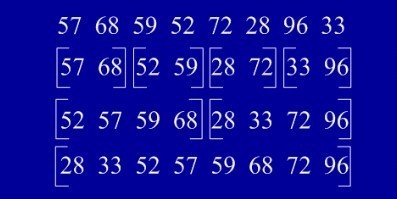
1 public static void mergingSort(int[] array) {
2 sort(array, 0, array.length - 1);
3 System.out.println(Arrays.toString(array) + " mergingSort");
4 }
5
6 private static void sort(int[] data, int left, int right) {
7 if (left < right) {
8 //找出中间索引
9 int center = (left + right) / 2;
10 //对左边数组进行递归
11 sort(data, left, center);
12 //对右边数组进行递归
13 sort(data, center + 1, right);
14 //合并
15 merge(data, left, center, right);
16 }
17 }
18
19 private static void merge(int[] data, int left, int center, int right) {
20 int[] tmpArr = new int[data.length];
21 int mid = center + 1;
22 //third记录中间数组的索引
23 int third = left;
24 int tmp = left;
25 while (left <= center && mid <= right) {
26 //从两个数组中取出最小的放入中间数组
27 if (data[left] <= data[mid]) {
28 tmpArr[third++] = data[left++];
29 } else {
30 tmpArr[third++] = data[mid++];
31 }
32 }
33
34 //剩余部分依次放入中间数组
35 while (mid <= right) {
36 tmpArr[third++] = data[mid++];
37 }
38
39 while (left <= center) {
40 tmpArr[third++] = data[left++];
41 }
42
43 //将中间数组中的内容复制回原数组
44 while (tmp <= right) {
45 data[tmp] = tmpArr[tmp++];
46 }
47 }
8. Radix sorting
Basic idea: Unify all the values to be compared (positive integers) into the same digit length, and pad zeros in front of numbers with shorter digits. Then, starting from the lowest bit, sort them one by one. In this way, after the sorting from the lowest bit to the highest bit is completed, the sequence becomes an ordered sequence.
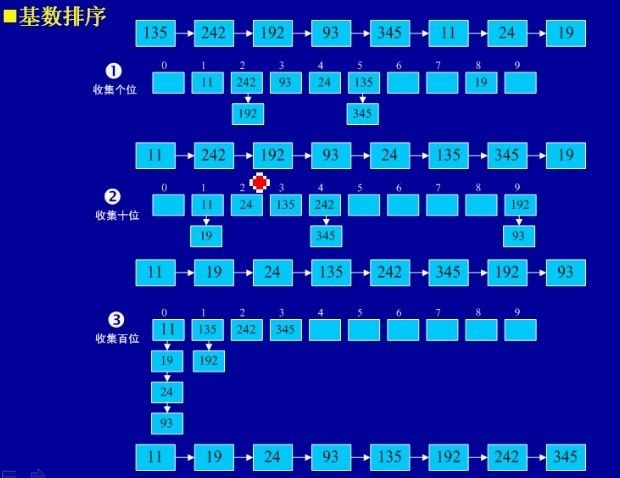
1 public static void radixSort(int[] array) {
2 //首先确定排序的趟数;
3 int max = array[0];
4 for (int i = 1; i < array.length; i++) {
5 if (array[i] > max) {
6 max = array[i];
7 }
8 }
9 int time = 0;
10 //判断位数;
11 while (max > 0) {
12 max /= 10;
13 time++;
14 }
15
16
17 //建立10个队列;
18 ArrayList<ArrayList<Integer>> queue = new ArrayList<>();
19 for (int i = 0; i < 10; i++) {
20 ArrayList<Integer> queue1 = new ArrayList<>();
21 queue.add(queue1);
22 }
23
24
25 //进行time次分配和收集;
26 for (int i = 0; i < time; i++) {
27 //分配数组元素;
28 for (int anArray : array) {
29 //得到数字的第time+1位数;
30 int x = anArray % (int)Math.pow(10, i + 1) / (int)Math.pow(10, i);
31 ArrayList<Integer> queue2 = queue.get(x);
32 queue2.add(anArray);
33 queue.set(x, queue2);
34 }
35 int count = 0;//元素计数器;
36 //收集队列元素;
37 for (int k = 0; k < 10; k++) {
38 while (queue.get(k).size() > 0) {
39 ArrayList<Integer> queue3 = queue.get(k);
40 array[count] = queue3.get(0);
41 queue3.remove(0);
42 count++;
43 }
44 }
45 }
46 System.out.println(Arrays.toString(array) + " radixSort");
47 }