introduce:
Due to the need to accurately obtain the data of advertising (the platform for advertising, preventing data loss, etc.). We use the Google Play Install Referrer API and Facebook App Ads Referral to collect data, and then record the data through the background service. Finally, compare the data recorded by the server with the data provided by Facebook to check the authenticity of the data, the packet loss profile, etc. Finally, conclusions can be used for subsequent operation strategies.
Official website:
Require:
Fix network problems. (you know)
A Google Play Console account is required.
A Facebook admin account is required.
Phones require the Google Play app version 8.3.73 or higher. Devices automatically provide this API.
Preparations before integration
Mock Ad Link: Can be generated by Google Play URL Builder .
My simulation is generated as follows:
https://play.google.com/store/apps/details?id=com.UCMobile.intl&referrer=utm_source%3Dtaobao%26utm_medium%3Dbanner%26utm_term%3Drunning%26utm_content%3D%25E6%2588%2591%25E6%2598%25AF%25E5%25B0%258F%25E5%25BC%25BA%26utm_campaign%3D%25E6%2595%25B2%25E4%25BB%25A3%25E7%25A0%2581%25E7%259A%2584%25E5%25B0%258F%25E5%25BC%25BA%26anid%3Dadmob
Google Play Install Referrer API Collection:
Add the following line to the dependencies section of your app's build.gradle file:
dependencies {
implementation "com.android.installreferrer:installreferrer:2.2"
}
Connect to Google Play for install referrers
hint:
Use try catch. installVersion may be null. A null pointer will be reported.
The place where the Log is printed is to pass the result of response.installReferrer (encrypted) to the backend. The backend then decrypts it via Facebook. Get the data for advertising.
response.installReferrer returns the encrypted data, and you need to get the Facebook secret key to decrypt it. (The location of the key will be introduced below)
package com.UCMobile.intl
import android.content.Context
import android.util.Log
import com.android.installreferrer.api.InstallReferrerClient
import com.android.installreferrer.api.InstallReferrerStateListener
import com.android.installreferrer.api.ReferrerDetails
/**
* @author 小强
*
* @time 2023/1/9 15:25
*
* @desc Google Play Install Referrer API库
*
*/
class InstallReferrer {
/**
* 初始化Play Install Referrer 库
*/
fun initReferrerClient(context : Context) {
//启动并与 Play 商店应用的连接
val referrerClient : InstallReferrerClient = InstallReferrerClient.newBuilder(context).build()
referrerClient.startConnection(object : InstallReferrerStateListener {
//onInstall Referrer安装完成
override fun onInstallReferrerSetupFinished(responseCode : Int) {
when (responseCode) {
InstallReferrerClient.InstallReferrerResponse.OK -> {
try {
//连接已建立后获取安装引荐来源
val response : ReferrerDetails = referrerClient.installReferrer
//已安装软件包的引荐来源网址。
val referrerUrl : String = response.installReferrer
//引荐来源网址点击事件发生时的客户端时间戳(以秒为单位)。
val referrerClickTime : Long = response.referrerClickTimestampSeconds
//应用安装开始时的客户端时间戳(以秒为单位)。
val appInstallTime : Long = response.installBeginTimestampSeconds
//引荐来源网址点击事件发生时的服务器端时间戳(以秒为单位)。
val appServerTime : Long = response.referrerClickTimestampServerSeconds
//首次安装应用时的应用版本。
val installVersion : String = response.installVersion
//表明应用的免安装体验是否为过去 7 天内发布的。
val instantExperienceLaunched : Boolean = response.googlePlayInstantParam
//断开服务连接(断开连接将有助于避免出现泄露和性能问题。)
referrerClient.endConnection()
Log.e("hzq", "推荐url-->" + referrerUrl)
Log.e("hzq", "推荐点击事件发生时的时间戳(单位是秒)-->" + referrerClickTime)
Log.e("hzq", "app安装时间时间戳(单位是秒)-->" + appInstallTime)
Log.e("hzq", "服务器端时间戳(单位是秒)-->" + appServerTime)
Log.e("hzq", "首次安装应用时的应用版本-->" + installVersion)
Log.e("hzq", "免安装体验是否过去7天内发布的-->" + instantExperienceLaunched)
} catch (e : Exception) {
}
}
InstallReferrerClient.InstallReferrerResponse.FEATURE_NOT_SUPPORTED -> {
//当前Play Store应用程序上没有API。
}
InstallReferrerClient.InstallReferrerResponse.SERVICE_UNAVAILABLE -> {
//无法建立连接。
}
}
}
//onInstall Referrer服务已断开连接
override fun onInstallReferrerServiceDisconnected() {
Log.e("hzq", "onInstall Referrer服务已断开连接")
//尝试在下次请求时重新启动连接
//通过调用startConnection()方法来Google Play。
}
})
}
}
Print result:
response-->com.android.installreferrer.api.ReferrerDetails@f2f9a14
推荐url-->utm_source=%E6%B7%98%E5%AE%9D&utm_medium=banner&utm_term=%E5%B0%8F%E5%BC%BA&utm_content=%E6%88%91%E6%98%AF%E5%B0%8F%E5%BC%BA&utm_campaign=%E6%95%B2%E4%BB%A3%E7%A0%81%E7%9A%84%E5%B0%8F%E5%BC%BA&anid=admob
推荐点击事件发生时的时间戳(单位是秒)-->1673259864
app安装时间时间戳(单位是秒)-->1673259870
服务器端时间戳(单位是秒)-->1673259863
首次安装应用时的应用版本-->13.4.0.1306
免安装体验是否过去7天内发布的-->false
Key point: simulated installation test ( the mobile application must have the Google Play application store )
If the Google Play application store already has its own application: you can fill in your own application package name when simulating the advertisement link. Click the ad delivery link to enter the app store, and click Install. Then cancel the installation again. Then run it on the phone in the local project. Finally, after the installation is successful, start the application and there will be InstallReferrerClient information.
If the Google Play application store does not have its own application: we need to change our application package name to an existing application package name in the application store. When my current project is simulated, I use the package name of UC ( com.UCMobile.intl ). Then click the ad delivery link to enter the app store, and click Install. Then cancel the installation again. Then run it on the phone in the local project. Finally, after the installation is successful, start the application and there will be InstallReferrerClient information.
Obtaining Facebook App Ads Referral Key:
You need to add an application in the Facebook App management platform by yourself.
It can be found in the app's Settings -> Basic -> Google Play . As shown below:
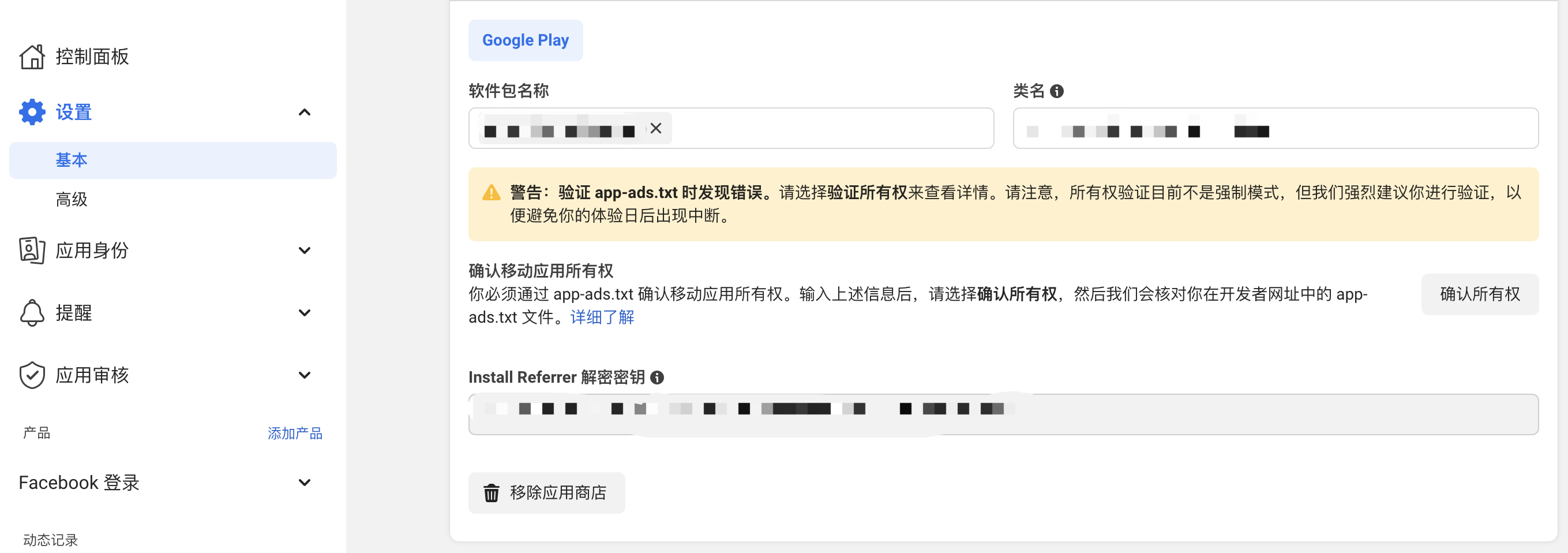