Please read the following articles before browsing
In the urls.py file in the project directory, we will see such a url configuration
Start the service, enter the URL in the browser http://127.0.0.1:8000/admin/
, the result is as follows
Django provides a very powerful management background, only a few lines of commands can generate a background management system
Press <Ctrl + C> to close the service, we execute the following command in the terminal to create an administrator account
python manage.py migrate
# 按照提示输入账户和密码,密码强度符合一定的规则要求
python manage.py createsuperuser
Results as shown below
After the creation is complete, restart the server and visit the URL https://127.0.0.1:8000/admin/ in the browser to access the project background login page provided by Django
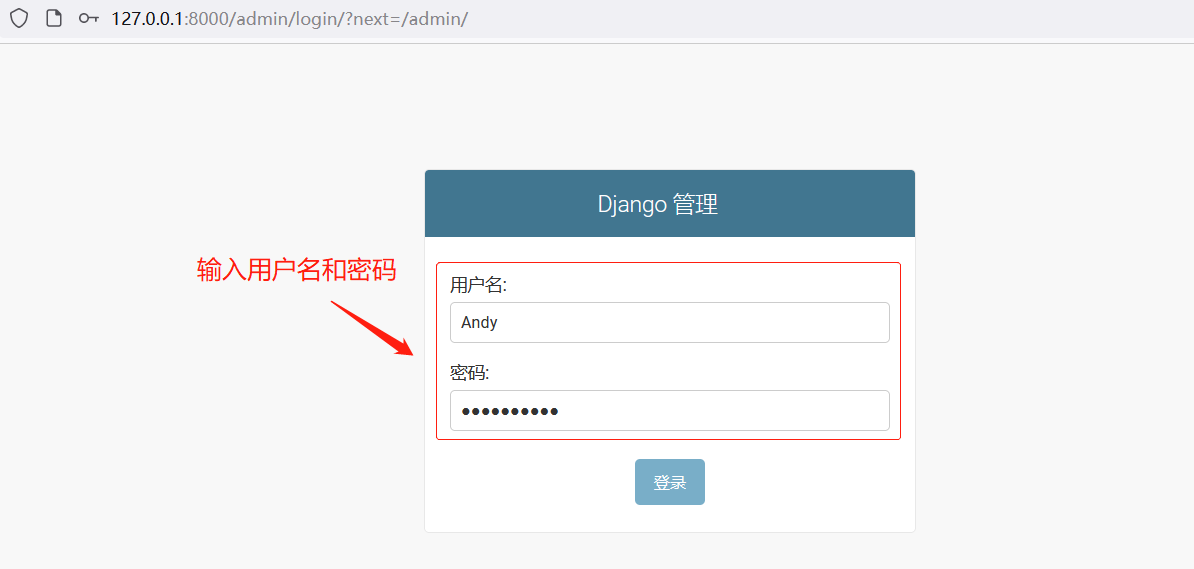
Log in with the username and password you just created, and you can see the background management interface
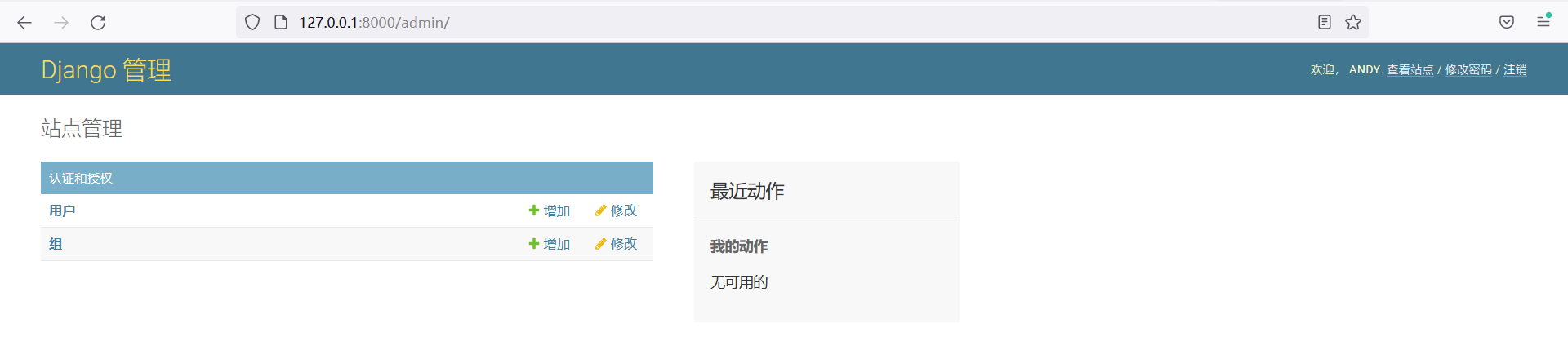
Tips: The management interface is not prepared for website visitors, but for managers
Custom Admin Pages
Define the data model in the article/model.py file
from django.db import models # 引入django.db.models模块
class User(models.Model):
"""
User模型类,数据模型应该继承于models.Model或其子类
"""
id = models.IntegerField(primary_key=True) # 主键
username = models.CharField(max_length=30) # 用户名,字符串类型
email = models.CharField(max_length=30) # 邮箱,字符串类型
class Article(models.Model):
"""
Article模型类,数据模型应该继承于models.Model或其子类
"""
id = models.IntegerField(primary_key=True) # 主键
title = models.CharField(max_length=120) # 标题,字符串类型
content = models.TextField() # 内容,文本类型
publish_date = models.DateTimeField() # 出版时间,日期时间类型
user = models.ForeignKey(User, on_delete=models.CASCADE) # 设置外键
Modify the article/admin.py configuration file, in the admin.py file, create the UserAdmin and ArticleAdmin background control model classes, all inherit the admin.ModelAdmin class, and set the properties, and finally bind the data model to the management background
from django.contrib import admin
from .models import User, Article
class UserAdmin(admin.ModelAdmin):
"""
创建UserAdmin类,继承于admin.ModelAdmin
"""
# 配置展示列表,在User板块下的列表展示
list_display = ('username', 'email')
# 配置过滤查询字段,在User板块下的右侧右侧过滤框
list_filter = ('username', 'email')
# 配置可以搜索的字段,在User板块下的右侧搜索框
search_fields = (['username', 'email'])
class ArticleAdmin(admin.ModelAdmin):
"""
创建ArticleAdmin类,继承于admin.ModelAdmin
"""
# 配置展示列表,在Article板块下的列表展示
list_display = ('title', 'content', 'publish_date')
# 配置过滤查询字段,在Article板块下的右侧右侧过滤框
list_filter = ('title',)
# 配置可以搜索的字段,在Article板块下的右侧搜索框
search_fields = ('title',)
# 绑定User模型到UserAdmin管理后台
admin.site.register(User, UserAdmin)
# 绑定Article模型到ArticleAdmin管理后台
admin.site.register(Article, ArticleAdmin)
Tips
① Register the model that needs to be displayed on the background management page in the admin.py file. If it is not registered, it will not be displayed
② Django provides the admin.ModelAdmin class, which defines the display mode of the model in the Admin interface by defining the subclass of ModelAdmin
③ Set the attribute list_filter, search_fields can be a list or a tuple
④ Call the admin.site.register method to register
After the configuration is complete, start the development server, enter the URL https://127.0.0.1:8000/admin/ again in the browser , and an ARTICLE class management will be added in the background panel. There are two models Articles and Users below.
When we select a model, we can implement corresponding operations such as adding, deleting, modifying and querying the model. For example, click the [Add] button on the right side of the "Articles" model to perform the operation of adding article information
Tips: After clicking the [Add] button on the right side of the "Articles" model, if the above page cannot pop up, and an error similar to the following django.db.utils.OperationalError: no such table: article_user appears , we need to execute it in the terminal The following two commands can solve the situation that the added article page cannot be loaded
python manage.py makemigrations
python manage.py migrate
We add the following article information, and the result is shown in the figure below