1. The principle of webSocket
1. Why use websocket when there is HTTP?
Because the HTTP protocol has a defect: the communication can only be initiated by the client, and it does not have the ability to push the server.
For example, if we want to know and query today's real-time data, the only way is for the client to send a request to the server, and the server returns the query result. The HTTP protocol cannot allow the server to actively push information to the client.
Before the emergence of the WebSocket protocol, creating a web application that enters dual-channel communication with the server needs to rely on the HTTP protocol for continuous polling , which will cause some problems :
The server is forced to maintain a large number of different connections from each client
A large number of polling requests will cause high overhead, such as extra headers, resulting in useless data transmission.
2. The difference between HTTP and WebSocket
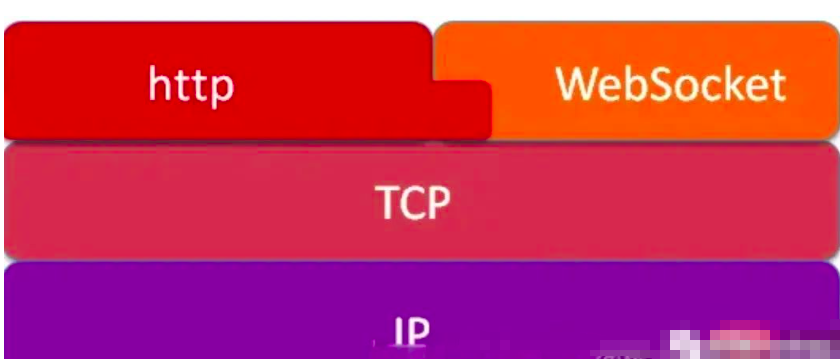
The same point : they are all based on TCP, and they are all reliable transmission protocols. Both are application layer protocols.
Contact: When WebSocket establishes a handshake, data is transmitted via HTTP. But after the establishment, the HTTP protocol is not required for actual transmission.
Differences :
1. WebSocket is a two-way communication protocol, simulating the Socket protocol, which can send or receive information in two directions, while HTTP is one-way;
2. WebSocket requires a handshake between the browser and the server to establish a connection, while http is a connection initiated by the browser to the server.
3. Although HTTP/2 also has the server push function, HTTP/2 can only push static resources, and cannot push specified information.
3. Advantages and disadvantages of WebSocket protocol
advantage:
Once the WebSocket protocol is proposed, the request header consumed by communicating with each other is very small
The server can push messages to the client
shortcoming:
A small number of browsers do not support it, and the degree and method of browser support are different (IE10)
Second, the use of websocket
The websocket object provides a websocket connection for creation and management, and an API that can send and receive data through the connection
webSocket event
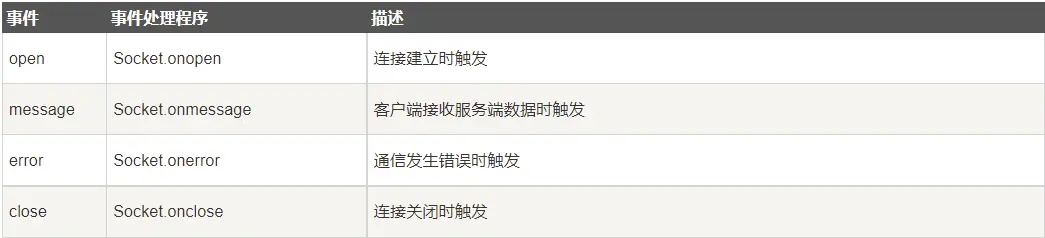
websocket method

create connection
websocket constructor, create a websocket object
Parameter url: Specify the connection URL, interface address.
The parameter protocol is optional and specifies the acceptable subprotocols.
A protocol string or an array containing protocol strings, which are used to specify sub-protocols, so that a single server can implement multiple WebSocket sub-protocols (different types of interactions can be handled by a server according to the specified protocol (protocols) , if no protocol string is specified, the empty string is assumed)
//websocket构造函数,创建websocket对象
var ws = new WebSocket(url,protocols)
Callback function ws.onopen() after successful connection
The callback function after the connection is successful. It is called when the readyState of the websocket connection becomes 1, which means it is ready to send and receive data
var ws = new WebSocket('url')
ws.onopen = function(params){
console.log('连接成功')
//向服务器发送消息
ws.send('hello')
}
or
var ws = new WebSocket('url')
ws.addEventListener('open',function(event){
ws.send('连接成功')
})
The callback function ws.onmessage() when receiving information from the server
var sw = new WebSocket('url')
ws.onmessage = function(event){
console.log('收到服务器响应',event.data)
var data = event.data
}
or
var ws = new WebSocket('url')
ws.addEventListener('message',function(event){
var data = event.data
})
The callback function ws.onclose() after the connection is completed
var ws = new WebSocket('url')
ws.onclose = function(event){
console.log('关闭客户端连接')
var code = event.code;//表示服务端发送的关闭码
var reason = event.reason;//表示服务器关闭连接的原因
var wasClean = event.wasClean//表示连接是否完全关闭
}
or
var ws = new WebSocket('url')
ws.addEventListener('close',function(event){
console.log('关闭客户端连接')
var code = event.code;//表示服务端发送的关闭码
var reason = event.reason;//表示服务器关闭连接的原因
var wasClean = event.wasClean//表示连接是否完全关闭
})
Callback function ws.onerror() after connection failure
var ws = new WebSocket('url')
ws.onerror = function(event){
console.log('连接失败',event)
ws.send('连接失败')
}
or
var ws = new WebSocket('url')
ws.addEventListener('error',function(event){
console.log('连接失败',event)
ws.send('连接失败')
})
3. Heartbeat
Heartbeat is a concept in websocket, which is to use a timer in websocket to send a message content to the server at regular intervals. We agree that if the backend receives the message content, it will return a message content to the front end . If the front end does not receive a message reply, the websocket may be disconnected, and then a reconnection operation is required to ensure that our websocket is in a connected state.
let url = `${process.env['VUE_APP_WEBSOCKET']}/websocket`
let ws = new WebSocket(url)
ws.addEventListener('open', e => {
console.log('长连接连接成功')
// 执行心跳方法
dispatch('wsHeartStart')
})
readyState returns the current state of the instance object
switch (ws.readyState) {
case WebSocket.CONNECTING://可以用0,CONNECTING值为0,表示正在连接
//dosomething
break;
case WebSocket.OPEN://值为1,OPEN值为1,表示连接成功可以通信
//dosomething
break;
case WebSocket.CLOSING://CLOSING值为2,表示连接正在关闭
//dosomething
break;
case WebSocket.CLOSED://CLOSED值为3,表示打开连接失败或连接已经关闭
//dosomething
break;
default:
//this never happens
break;
}