Problem Description
The number of vertices in a weighted undirected graph is v, and the number of edges is e. When v is large, the number of elements in the adjacency matrix v*v is large, and manual creation is too troublesome.
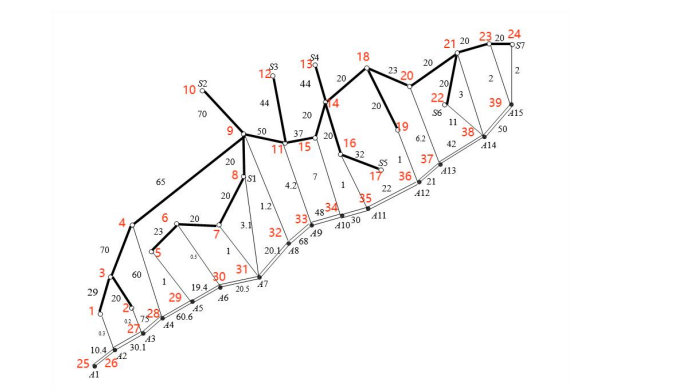
Solution
step.1
According to all the edges in the graph (each edge contains two vertices i, j, and the weight w of the edge),
Create a text file tu.txt with a total of e lines and 3 columns,
The first column is i, the second column is j, the third column is w,
Each row contains information about an edge in the graph.
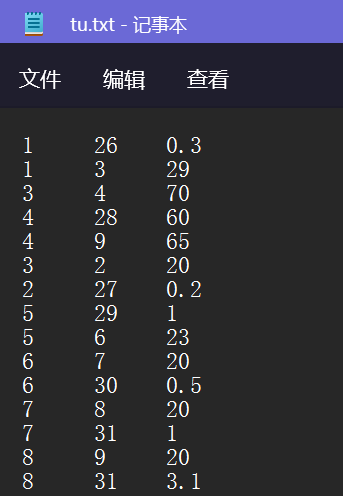
step.2
Write MATLAB functions,
Input: number of vertices, number of edges, matrix B with e rows and 3 columns (B is generated according to tu.txt)
Output: adjacency matrix G of the graph
function [G] = adjacentmatrix(v,e,B)
% 根据带权无向图的所有边组成的矩阵B,生成图的邻接矩阵G
G=inf(v);
for i=1:v
for j=1:v
if i==j
G(i,j)=0;
end
for m=1:e
if B(m,1)==i && B(m,2)==j
G(i,j)=B(m,3);
elseif B(m,1)==j && B(m,2)==i
G(i,j)=B(m,3);
end
end
end
end
end
step.3
Execute the main function main
clear
clc
v=39;%顶点个数
e=54;%边数
%% 生成图的邻接矩阵G
fileID=fopen('tu.txt');%tu.txt文件每一行为(顶点1,顶点2,边的权值)
A=textscan(fileID,'%f %f %f');
fclose(fileID);
B=cell2mat(A);
G=adjacentmatrix(v,e,B);
Note: program structure
The following three files need to be placed in the same folder
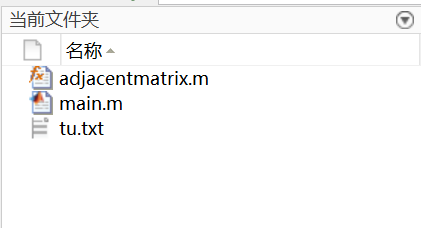