Table of contents
1. The significance and purpose of the experiment
3. Introduction of Matlab related functions
5. Reference code and extended code flow chart
(1) Try different threshold selection methods to achieve grayscale image binarization
(2) Transform parameters to achieve morphological filtering and check the filtering effect
(3) Change the number of reconstruction boundary points and check the effect
1. The significance and purpose of the experiment
2. Experimental content
3. Introduction of Matlab related functions
ifft2(F) : Perform two-dimensional IDFT operation on F.
4. Algorithm principle
Image segmentation refers to an image processing technology that divides an image into different areas with specific properties, and extracting these areas for further feature extraction is a key step from image processing to image analysis. Because of its importance, image segmentation has always been the focus of research in the field of image processing. The threshold segmentation method is a method for image segmentation by determining a certain threshold according to the distribution characteristics of image gray values. Let the original grayscale image be f ( x , y ) , select a grayscale value T as the threshold by a certain criterion, and compare the relationship between each pixel value and T : the pixels whose pixel value is greater than or equal to T are classified into one class, and the pixel value is changed is 1; the pixel whose pixel value is less than T is another type, and its pixel value is changed to 0 . The formula is as follows:
Boundary tracking refers to finding the pixels on the contour of the target object according to some strict "detection criteria", that is, to determine the starting search point of the boundary. Find other pixels on the target object according to a certain "tracking criterion" until the tracking termination condition is met.
Boundary description refers to the use of related methods and data to represent the boundaries of regions. Boundary description contains both geometric information and rich shape information, which is a very common image object description method. The method of Fourier delineation mainly uses DFT to delineate the boundary curve of sub-reconstruction area. Since the high-frequency components of Fourier correspond to some details, and the low-frequency components correspond to the basic shape, only the first M coefficients of the complex sequence can be used for reconstruction, and the rest are set to zero .
Regarding image segmentation, we have to mention the classic and traditional watershed algorithm. The traditional watershed segmentation algorithm is a segmentation method of mathematical morphology based on topology theory, and the traditional watershed segmentation method is a segmentation method of mathematical morphology based on topology theory. The traditional watershed segmentation method is a mathematical morphology segmentation method based on topology theory. Its basic idea is to regard the image as a topological landform in geodesy, and the gray value of each pixel in the image represents the altitude of the point. height, each local minimum and its area of influence are called catchment basins, and the boundaries of catchment basins form watersheds. The concept and formation of watersheds can be illustrated by simulating the immersion process. Puncture a small hole on the surface of each local minimum value, and then slowly immerse the whole model in water. As the immersion deepens, the influence domain of each local minimum value on the surface of the minimum value slowly outwards Expansion, building a dam at the confluence of two water catchment basins. The influence domain of each local minimum value slowly expands outward. Building a dam at the confluence of two water catchment basins is shown in the figure below, which forms a watershed. Watershed transformation separates regions through different water system drainage, and achieves the image as shown in the image, that is, forms a watershed. Watershed transformation separates regions through different water system drainage to achieve the purpose of image segmentation.
5. Reference code and extended code flow chart
(1) Reference code flow chart
(2) Extended code flow chart
6. Reference code
Image1=im2double(imread('plane.jpg'));
gray=rgb2gray(Image1);
T=graythresh(gray);%使用 Otsu 方法获取阈值,T 被归一化到[0,1]区间。
BW=im2bw(gray,T);%以 T 为阈值把灰度图像 I 转变为二值图像。
figure,imshow(BW),title('二值化图像');
SE=strel('square',3);%创建一个由square指定的结构元素,参数3控制 SE 的大小。
Morph=imopen(BW,SE); %对图像BW进行开运算,返回图像为Morph,SE是结构元素
Morph=imclose(Morph,SE);
figure,imshow(Morph),title('形态学滤波');
[B L]=bwboundaries(1-Morph);%搜索二值图像BW的外边界和内边界
figure,imshow(L),title('划分的区域');
hold on;
for i=1:length(B)
boundary=B{i};
plot(boundary(:,2),boundary(:,1),'r','LineWidth',2);
end
M=zeros(length(B));
for k=1:length(B)
N=length(B{k});
if N/2~=round(N/2)
B{k}(end+1,:)=B{k}(end,:);
N=N+1;
end
M(k)=[N*3/4];
end
S=zeros(size(Morph));
figure,imshow(S);
hold on;
for k=1:length(B)
z=B{k}(:,2)+1i*B{k}(:,1);
Z=fft(z);
[Y I]=sort(abs(Z));
for count=1:M(k)
Z(I(count))=0;
end
zz=ifft(Z);%对Z进行IDFT 运算。
plot(real(zz),imag(zz),'w');
end
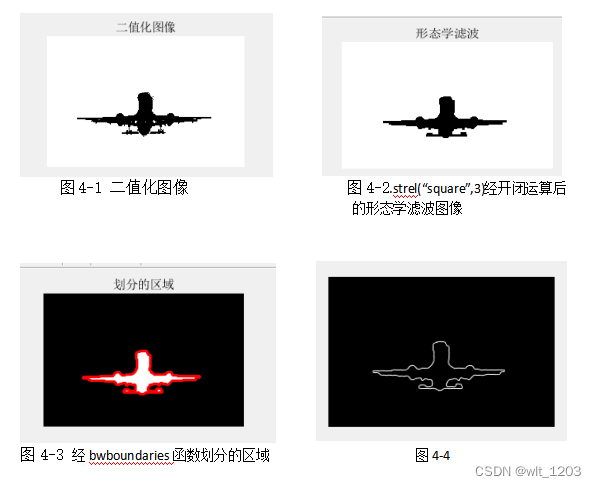
7. Experimental requirements
( 1 ) Try different threshold selection methods to achieve grayscale image binarization
code:
I=imread('plane.jpg');
%人工选定阈值进行分割,选择阈值为150
[width,height]=size(I);
T1=150;
for i=1:width
for j=1:height
if(I(i,j)<T1)
BW1(i,j)=0;
else
BW1(i,j)=1;
end
end
end
BW2 = im2bw(BW1);
figure;imshow(BW2),title('人工阈值进行分割');
operation result:
(2) Transform parameters to achieve morphological filtering and check the filtering effect
code:
Image1=im2double(imread('plane.jpg'));
gray=rgb2gray(Image1);
T=graythresh(gray);
BW=im2bw(gray,T);
figure,imshow(BW),title('二值化图像');
SE=strel('disk',3);
Morph=imopen(BW,SE);
Morph=imclose(Morph,SE);
figure,imshow(Morph),title('形态学滤波');
operation result:
(3) Change the number of reconstruction boundary points and check the effect
code:
Image1=im2double(imread('plane.jpg'));
gray=rgb2gray(Image1);
T=graythresh(gray);
BW=im2bw(gray,T);
[B L]=bwboundaries(1-BW);
figure,imshow(L),title('划分的区域');
hold on;
for i=1:length(B)
boundary=B{i};
plot(boundary(:,2),boundary(:,1),'r','LineWidth',1);
end
M=zeros(length(B),4);
for k=1:length(B)
N=length(B{k});
if N/2~=round(N/2)
B{k}(end+1,:)=B{k}(end,:);
N=N+1;
end
M(k,:)=[N/2 N*7/8 N*15/16 N*63/64];
end
S=zeros(size(Morph));
for m = 1:4
figure,imshow(S);
hold on;
for k=1:length(B)
z=B{k}(:,2)+1i*B{k}(:,1);Z=fft(z);
[Y I]=sort(abs(Z));
for count=1:M(k,m)
Z(I(count))=0;
end
zz=ifft(Z);
plot(real(zz),imag(zz),'w');
end
end
operation result:
(4) Self-designed method to achieve image segmentation, and calculate the relevant parameters of the segmented area
code:
img=imread('plane.jpg');
subplot(2,3,1);
imshow(img);
C = makecform('srgb2lab'); %设置转换格式
img_lab = applycform(img, C);
ab = double(img_lab(:,:,2:3)); %取出lab空间的a分量和b分量
nrows = size(ab,1);
ncols = size(ab,2);
ab = reshape(ab,nrows*ncols,2);
nColors = 3; %分割的区域个数为3
[cluster_idx cluster_center] = kmeans(ab,nColors,'distance','sqEuclidean','Replicates',3); %重复聚类3次
pixel_labels = reshape(cluster_idx,nrows,ncols);
subplot(2,3,2);
imshow(pixel_labels,[]), title('聚类结果');
%显示分割后的各个区域
segmented_images = cell(1,3);
rgb_label = repmat(pixel_labels,[1 1 3]);
for k = 1:nColors
color = img;
color(rgb_label ~= k) = 0;
segmented_images{k} = color;
end
subplot(2,3,3);
imshow(segmented_images{1}), title('分割结果——区域1');
subplot(2,3,4);
imshow(segmented_images{2}), title('分割结果——区域2');
subplot(2,3,5);
imshow(segmented_images{3}), title('分割结果——区域3');
operation result: