The back-end server can be opened on CSDN, the price is favorable
[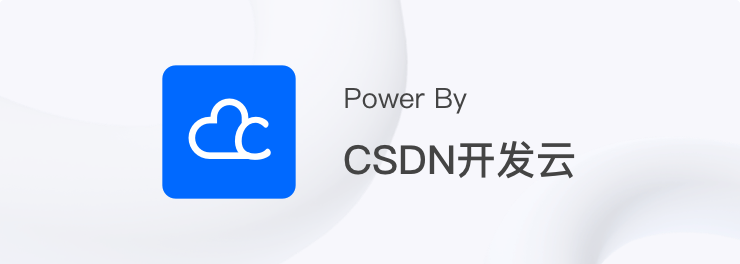](https://dev.csdn.net/activity?utm_source=sale_source&sale_source=q4AnCOkys7)
Register the Mini Program on the WeChat public platform and obtain the AppID.
Create a login page in the applet, including user name, password input box and login button.
Send username and password to backend server via HTTPS POST request.
The backend server verifies whether the username and password are correct, and if correct, generates and returns a session_key.
After receiving the session_key, the front end saves it in the local storage.
Send session_key to backend server for authentication.
After the identity verification is successful, the user information is returned to the front end, and the user information includes user ID, nickname, avatar, etc.
// 前端代码
wx.request({
url: 'https://example.com/api/login',
method: 'POST',
data: {
username: 'example',
password: 'password'
},
success: function (res) {
wx.setStorageSync('session_key', res.data.session_key);
},
fail: function (res) {
console.log('登录失败')
}
})
// 后端代码
app.post('/api/login', function (req, res) {
// 获取用户名和密码
const { username, password } = req.body;
// 在数据库中验证用户名和密码是否正确
if (checkPassword(username, password)) {
// 生成 session_key
const session_key = uuid.v4();
// 将 session_key 存储在数据库中
saveSessionKey(username, session_key);
// 返回 session_key
res.json({ session_key: session_key });
} else {
res.status(401).send('用户名或密码错误');
}
})
// 鉴权代码
app.get('/api/user', function (req, res) {
// 从请求头中获取 session_key
const session_key = req.headers['session-key'];
// 验证 session_key 是否正确
if (checkSessionKey(session_key)) {
// 如果验证通过,返回用户信息
const userInfo = getUserInfo(session_key);
res.json(userInfo);
} else {
res.status(401).send('未授权访问');
}
})
The checkPassword , saveSessionKey , checkSessionKey , and getUserInfo functions in the above code need to be implemented according to the actual situation. After successful login, the session_key can be saved in the local storage through wx.setStorageSync , and the session_key can be obtained through wx.getStorageSync when used in other pages . In the interface that needs to be authenticated, you can get the session_key from the request header, and then perform authentication and user information acquisition.