Performance optimization in Android is divided into the following aspects:
layout optimization
Network Optimization
Installation package optimization
memory optimization
Caton optimization
start optimization
……
1. Layout optimization
The essence of layout optimization is to reduce the hierarchy of View. Common layout optimization schemes are as follows:
-
In the case where both LinearLayout and RelativeLayout can complete the layout, LinearLayout is preferred, which can reduce the level of View, but note that the same component may take a long time to draw RelativeLayout
-
Use the <include> tag to extract the common parts of commonly used layout components for reuse.
-
Use the < ViewStub > tag to load unused layouts, lazy loading (loaded in the activity when needed)
-
Use < Merge > tags to reduce layout nesting levels
2. Drawing optimization
Drawing optimization means that View's onDraw method should avoid performing a large number of operations, which is mainly reflected in two aspects:
1. Do not create new local objects in onDraw.
Because the onDraw method may be called frequently, a large number of temporary objects will be generated in an instant, which not only takes up too much memory, but also causes the system to gc more frequently, reducing the execution efficiency of the program.
2. Do not do time-consuming tasks in the onDraw method,
Thousands of loop operations cannot be performed. Although each loop is very lightweight, a large number of loops still occupy CPU time slices, which will cause the View's drawing process to be unsmooth.
According to the standards in the performance optimization model officially given by Google, the drawing frequency of View is guaranteed to be 60fps is the best, which requires that the drawing time of each frame should not exceed 16ms (16ms = 1000/60), although it is difficult for the program to guarantee the time of 16ms , but it is always practical to minimize the complexity in the onDraw method.
3. Network optimization
Common network optimization schemes are as follows:
-
Minimize network requests, and merge as much as possible
-
Avoid DNS resolution. Domain name query may take hundreds of milliseconds, and there may be a risk of DNS hijacking. You can use the method of adding dynamic IP updates according to business needs, or switch to the domain name access method when the IP method access fails.
-
A large amount of data is loaded in a paging manner
-
Network data transmission adopts GZIP compression
-
Join the cache of network data to avoid frequent network requests
-
When uploading pictures, compress pictures when necessary
4. Installation package optimization
-
The core of installation package optimization is to reduce the size of apk. Common solutions are as follows:
-
Reduce unnecessary resource files in the application, such as pictures, and compress the pictures as much as possible without affecting the effect of the APP, which has a certain effect
-
When using the SO library, keep the v7 version of the SO library first, and delete other versions of the SO library. The reason is that in 2018, the v7 version of the SO library can meet most of the requirements on the market. It may not be possible for mobile phones eight or nine years ago, but we don't need to adapt to outdated mobile phones. The effect of reducing the apk volume in actual development is very significant. If you use a lot of SO libraries, for example, a version of the SO library has a total of 10M, then only keep the v7 version, delete the armeabi and v8 versions of the SO library, and the total can be reduced. 20M volume.
-
res resource optimization
(1) Use only one set of pictures, and use high-resolution pictures.
(2) UI design Install the TinyPNG plug-in in ps to compress the pictures losslessly.
(3) svg pictures: the description of some pictures sacrifices the computing power of the CPU to save space. Principles used: simple icons.
(4) The image uses the WebP (https://developers.google.com/speed/webp/) format (Facebook, Tencent, Taobao are using it.) Disadvantage: The loading is much slower than PNG. But the configuration is relatively high. Tools: http://isparta.github.io/
(5) Use tintcolor (android - Change drawable color programmatically) to achieve button inversion effect.
-
Code optimization
(1) Realize the logic simplification of functional modules
(2) The Lint tool checks useless files and lists useless resources in "UnusedResources: Unused resources" and deletes them.
(3) Remove useless dependent libraries.
-
lib resource optimization
(1) Dynamically downloaded resources.
(2) The plug-in of some modules is added dynamically.
(3) Clipping and compression of so files.
-
assets resource optimization
(1) It is better to use lossy compression format for audio files, such as opus, mp3 and other formats, but it is best not to use lossless compression music format
(2) To compress the ttf font file, you can use the FontCreator tool to extract only the text you need. For example, when doing date display, only digital fonts are needed, but using the original font library may require a size of 10MB. If you just extract the fonts you need, the generated font file is only 10KB.
-
Code obfuscation.
-
Use the proGuard code obfuscator tool, which includes minification, optimization, obfuscation, and more.
-
Plug-in
-
Function modules can be placed on the server and loaded when needed.
-
7z extreme compression
5. Android memory optimization
1. Android memory management mechanism
Android applications run on the Android virtual machine, and memory allocation and garbage collection are performed by the Android virtual machine.
2. Common memory leaks
In fact, the essence of memory leaks is that objects with a longer life cycle refer to objects with a shorter life cycle.
2.1 Memory leaks
Cause of memory leak: The object allocated on the heap will no longer be used, but the GC collector cannot recycle it, and this object is referenced by a strong application.
Memory leaks caused by static variables
Solution: Set the inner class as a static inner class or separate it; use context.getApplicationContext().
Memory leak caused by singleton mode
Solution: pass parameter context.getApplicationContext().
Memory leak caused by property animation
Solution: Call Animator.cancel() in Activity.onDestroy() to stop the animation.
Memory leak caused by Handler
Solution: Use static inner class + WeakReference weak reference; clear the message queue when the outer class ends its life cycle.
memory leaks caused by threads
Solution: Set AsyncTask and Runnable as static inner classes or separate them; use weak references to save Context references inside threads.
Memory leaks caused by unclosed resources
Solution: Close or log off in time when the Activity is destroyed. For example:
① BroadcastReceiver: Call unregisterReceiver() to log out;
②Cursor, Stream, File: Call close() to close;
③Bitmap: call recycle() to release the memory (no need to manually after version 2.3).
Memory leak caused by Adapter
Details: Instead of using the cache and only relying on getView() to re-instantiate the Item every time, it will put pressure on the gc.
Solution: Use the cached convertView when constructing the Adapter.
Memory leak caused by WebView.
Details: WebView is special, even if its destroy method is called, it will still cause a memory leak.
Solution: In fact, the best way to avoid memory leaks caused by WebView is to make the Activity where WebView is located in another process. When this Activity ends, kill the current process where WebView is located. I remember that the WebView of Ali Dingding is another process. A process that is opened should also use this method to avoid memory leaks.
collection class leak
Details: For example, there are static applications such as global maps, but they are not deleted in the end.
Solution: Recycle unnecessary collections during onDestry.
2.2 Expand memory
The SDKs of big manufacturers may have fewer memory leaks, but the quality of SDKs of some small manufacturers is less reliable. The best way to deal with this situation that we cannot change is to expand the memory.
There are usually two ways to expand memory:
One is to add the attribute largeHeap="true" under Application in the manifest file, and the other is to open multiple processes for the same application to expand the total memory space of an application.
The second method is actually very common. For example, I have used the SDK of Getui, and the Service of Getui is actually in another separate process.
Memory optimization in Android is generally open source and throttling. Open source is to expand memory, and throttling is to avoid memory leaks.
2.3 Tools for detecting and analyzing memory leaks
MemoryMonitor: Changes in memory usage over time
MAT: input HRPOF file, output analysis results
a. Histogram: View different types of objects and their sizes
b.DominateTree: the memory occupied by the object and its reference relationship
c.MAT tutorial
LeakCanary: A library for real-time monitoring of memory leaks (LeakCanary principle)
6. Caton optimization plan
-
Do not perform network access/IO operations on large files in the main thread
-
When drawing the UI, try to reduce the drawing UI level; reduce unnecessary view nesting, you can use the Hierarchy Viewer tool to detect it, which will be described in detail later;
-
When our layout uses FrameLayout, we can change it to merge, which can avoid overlapping calculations between our own frame layout and the system's ContentFrameLayout frame layout (measure and layout)
-
To improve the display speed, use ViewStub: it will only be occupied when loading. It is hidden when it is not loaded, and only takes up space.
-
In the case of the same view level, try to use LinerLayout instead of RelativeLayout; because RelativeLayout will measure twice when measuring, and LinerLayout will measure once, you can see their source code;
-
Delete useless properties in the control;
-
Layout reuse. For example, listView layout reuse
-
Try to avoid overdrawing (overdraw), for example: the background is often easy to cause overdrawing. Since our layout has set a background, the MaterialDesign theme used at the same time will default to a background. At this time, the background added by the theme should be removed;
-
Optional background in XML
-
Custom View optimization. Use canvas.clipRect() to help the system identify those visible areas, only within this area will be drawn. Also avoid overdrawing.
-
Startup optimization, monitoring the startup speed, discovering the problems that affect the startup speed, optimizing the startup logic, and improving the startup speed of the application. For example, splash screen pages, reasonable optimization of layout, optimization of loading logic, and data preparation.
-
Reasonable refresh mechanism, minimize the number of refreshes, try to avoid high CPU threads running in the background, and reduce the refresh area.
7. Power consumption optimization
There are actually many reasons for power consumption. Here I will talk about several optimization schemes. The opposite of the optimization scheme is the reason. Several optimization schemes are as follows:
-
Reasonable use of wake_lock lock, wake_lock lock is mainly relative to the sleep of the system (here is to save power, it is done), which means that my program adds this lock to the CPU and the system will not sleep. Do this The purpose is to fully cooperate with the operation of our program. In some cases, if you don’t do this, there will be some problems, such as WeChat and other instant messaging heartbeat packets will stop network access shortly after the screen is turned off. Therefore, there are a lot of wake_lock locks used in WeChat.
-
Use jobScheduler2 to centrally process some network requests, and some that do not need to be processed in a timely manner can be processed while charging, such as image processing, APP download and update, etc.;
-
Calculation optimization, avoiding floating-point operations, etc.
-
When data is transmitted on the network, try to compress the data before transmission. It is recommended to use FlatBuffer serialization technology, which is many times more efficient than json. If you don’t know FlatBuffer, it is recommended to find information to learn.
Aiming at the key point of "performance optimization", I would like to share with you a "360° All-round Android Performance Optimization Analysis". This study manual will lead you to explore the performance optimization of Android step by step, so that the performance of the product can be improved in all aspects. , I hope you like it.
This document has a total of 721 pages, 4 major points, and 25 small chapters. It not only has a detailed analysis of the underlying principles, but also has special practical cases!
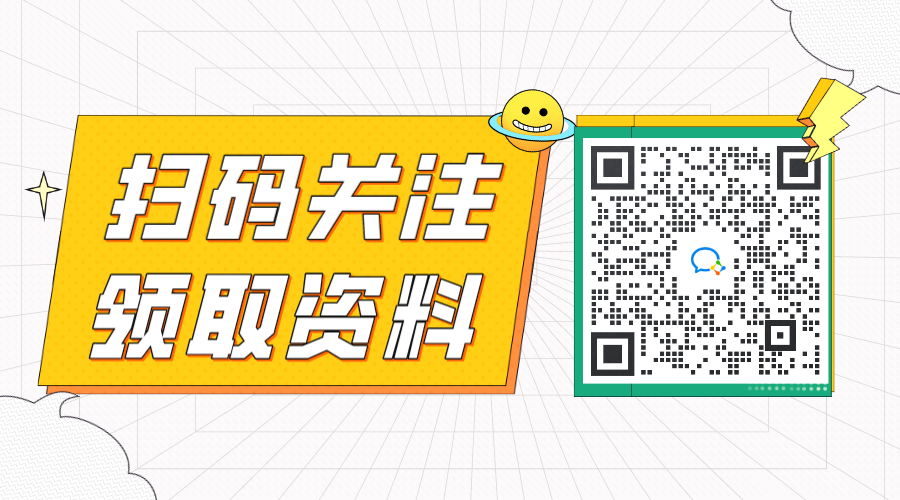
"Analysis of 360° All-round Android Performance Optimization"
Chapter 1 Design Ideas and Code Quality Optimization
1. Six principles
-
Single Responsibility Principle
-
Liskov Substitution Principle
-
Dependency Inversion Principle
-
Interface Segregation Principle
-
……
2. Design Patterns
-
Structural mode: bridge mode, adapter mode, decorator mode, proxy mode, facade (appearance) mode...
-
Creational patterns: builder pattern, singleton pattern, abstract factory pattern, factory method pattern...
-
Data structures: arrays, stacks, queues, linked lists, trees...
-
Algorithms: sorting algorithms, search algorithms...
Chapter 2 Program Performance Optimization
1. Optimization of startup speed and execution efficiency
-
Cold start and hot start analysis
-
App startup black and white screen solution
-
APP freeze problem analysis and solution
-
StrictMode for startup speed and execution efficiency optimization
-
……
2. Layout detection and optimization
-
Layout level optimization
-
over rendering
-
……
3. Memory optimization
-
Memory Thrashing and Memory Leaks
-
big memory
-
Bitmap memory optimization
-
Profile memory monitoring tool
-
Mat large objects and leak detection
-
Power consumption optimization
-
Network transmission and data storage optimization Network transmission and data storage optimization
-
APK size optimization
-
screen adaptation
-
……
4. Power consumption optimization
-
Doze&Standby
-
Battery Historian
-
job scheduler
-
WorkManager
5. Network transmission and data storage optimization
-
google serialization tool protobuf
-
7z extreme compression
-
……
6. APK size optimization
-
APK Slimming
-
WeChat resource confusion principle
-
……
7. Screen adaptation
-
The principle of adaptation
-
Adaptation principle of screen resolution qualifier and smallestWidth qualifier
-
Why choose the smallestWidth qualifier for adaptation
-
How to adapt to other modules
-
FAQ
……
8. Analysis of OOM problem principle
-
adj memory management mechanism
-
JVM memory recycling mechanism and GC algorithm analysis
-
Summary of Life Cycle Related Issues
-
Summary of Bitmap compression scheme
-
……
9. ANR problem analysis
-
AMS system time adjustment principle
-
Analysis of program waiting principle
-
ANR problem resolution
-
……
10. Crash monitoring solution
-
Java Layer Monitoring Solution
-
Nativie layer monitoring solution
-
……
Chapter 3 Development Efficiency Optimization
- Distributed version control system Git
Scenario Introduction of Enterprise Efficient Continuous Integration Platform
GIT distributed version control system
-
GIT branch management
-
……
2. Automatic build system Gradle:
-
Gradle and Android plugin: the relationship between gradle and android gradle plugin, the basic use of Gradle Transform API...
-
Basic usage of Gradle Transform API: what is Transform, Transform usage scenarios, Transform API learning, input type...
-
Custom plug-in development: Gradle plug-in introduction, preparation, practice, custom Gradle plug-in, buildSrc module method...
-
Plug-in combat: multi-channel packaging, automatic version release...
Chapter 4 APP performance optimization practice
1. Startup speed
-
General flow of application startup
-
cold start and warm start
-
Measurement of startup speed
-
Start window optimization
-
thread optimization
-
System Scheduling Optimization
-
GC optimization
-
I/O optimization
-
Resource rearrangement
-
Home page layout optimization
-
Class loading optimization
-
Choose the right startup framework
-
Reduce the jump level of Activity
-
Vendor optimization
-
background keep alive
-
……
-
2. Fluency
-
Some tools and routines for performance problem analysis
-
Data analysis through performance data
-
Performance Cases Caused by Android Platform Performance
-
Performance issues caused by the Android App itself
-
Data and Behavioral Characteristics of Low Memory
-
App treasure
-
Analysis of the whole machine freeze caused by Xunfei input method barrier-free service
-
Bytedance: Today’s Toutiao’s Graphic and Text Details Page Opens in Seconds
-
……
-
3. Douyin’s practice in APK package size resource optimization
-
Image Compression
-
webp non-intrusive compatibility
-
Multi-DPI optimization
-
Duplicate Resource Consolidation
-
shrinkResource strict mode
-
Resource confusion (compatible with aab mode)
-
ARSC Slimming
-
……
-
4. Full Analysis of Youku Responsive Layout Technology
-
Overview of Youku APP Responsive Layout Technology
-
Youku APP Responsive Layout Android Landing
-
Landing in the distribution scene
-
Landing in the consumption scene
-
Youku APP Responsive Layout Test Scheme
-
……
-
5. Network optimization
-
Mobile Taobao link optimization in the network
-
Practice of Baidu APP in deep network optimization
-
……
-
6. Reveal the Secret of Mobile Taobao Double Eleven Performance Optimization Project
-
Realization of the one-second rule
-
20% increase in startup time and page frame rate
-
Save 50% on Android phone memory
-
……
-
7. AutoNavi APP full link source code dependency analysis
-
Gaode APP Platform Architecture
-
Basic realization principle
-
project structure
-
Application Scenarios and Implementation Principles
-
……
-
8. Share the practical experience of completely killing OOM
-
Troubleshoot memory leaks
-
bottom line strategy
-
Memory peak is too high
-
Extra-large image troubleshooting and optimization
-
……
-
9. WeChat Android terminal memory optimization practice
-
Activity leak detection
-
Bitmap allocation and deallocation tracking
-
Native memory leak detection
-
thread monitoring
-
memory monitor
-
……
Summarize
Performance optimization cannot be solved by updating one or two versions. It is a continuous requirement, continuous integration and iterative feedback. In actual projects, at the beginning of the project, due to the constraints of manpower and project completion time, the priority of performance optimization is relatively low. When the project is put into use, the priority needs to be raised. When building a solution, performance optimization points also need to be considered early, which reflects the technical skills of a programmer. I hope this " 360° All-round Android Performance Optimization Analysis " can help you.
The information in this article can be scanned for free by scanning the CSDN official certification card below!
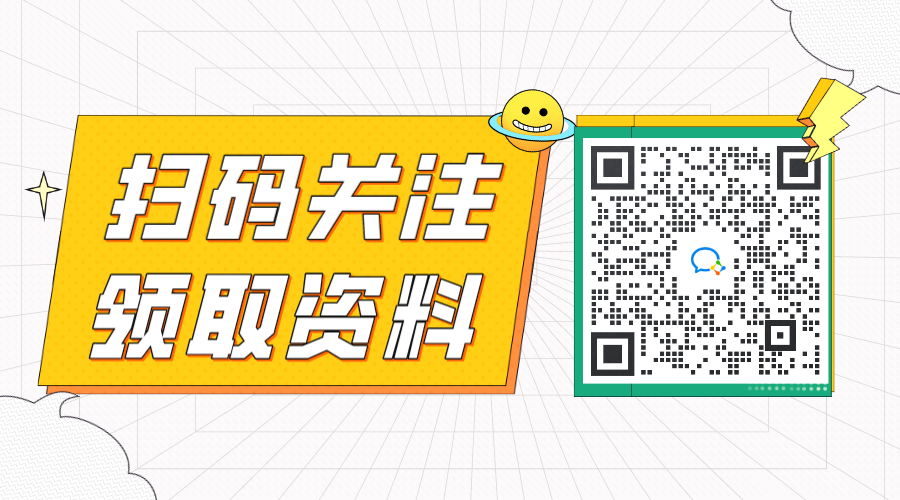