The first two articles have basically set up the environment for geodjango development. Of course, you must have python and django environments on your computer (I won't introduce this, and search a lot on the Internet), I use python3.5 myself And django2.0 (after all, 2.0 is out, and I also follow the times to learn the new version).
Reminder: If you are not familiar with django, you must first look at the official documentation of django, and you can go through his voting tutorial (it takes about a day for a pure novice)
Here's the thing:
Create a virtual environment (separate the project's library from other projects and install your own packages in it)
python -m venv myshape_env
activation
source myshape_env/bin/activate
(After the activation is successful, the command line front-end displays your virtual environment)
Install django:
pip install django
Create a new project:
django-admin startproject geodjango
Create a new application:
cd geodjango
python manage.py startapp world
Configure setting.py under geodjango
Applications that need to be introduced (add what you lack)
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'django.contrib.gis',
'world',
]
Database configuration: (comment out the original sqlite3 configuration, add the configuration that matches your own database, the following is mine)
# Database
# https://docs.djangoproject.com/en/2.0/ref/settings/#databases
DATABASES = {
'default': {
#'ENGINE': 'django.db.backends.sqlite3',
#'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
'ENGINE':'django.contrib.gis.db.backends.postgis',
'NAME': 'geodjango',
'USER': 'postgres',
'PASSWORD':'123',
'HOST':'localhost',
'PORT':'5432',
}
}
Spatial data: http://thematicmapping.org/downloads/TM_WORLD_BORDERS-0.3.zip
mkdir world/data
cd world/data
wget http://thematicmapping.org/downloads/TM_WORLD_BORDERS-0.3.zip
unzip TM_WORLD_BORDERS-0.3.zip
cd ../..
The following is the data (interested students can query the role of data in different formats by themselves)
Detecting data with ogrinfo
1.ogrinfo world/data/TM_WORLD_BORDERS-0.3.shp
2.ogrinfo -so world/data/TM_WORLD_BORDERS-0.3.shp TM_WORLD_BORDERS-0.3
Define the spatial data model: (the model field corresponds to the feature field above)
from django.contrib.gis.db import models
# Create your models here.
class WorldBorder(models.Model):
# Regular Django fields corresponding to the attributes in the
# world borders shapefile.
name = models.CharField(max_length=50)
area = models.IntegerField()
pop2005 = models.IntegerField('Population 2005')
fips = models.CharField('FIPS Code', max_length=2)
iso2 = models.CharField('2 Digit ISO', max_length=2)
iso3 = models.CharField('3 Digit ISO', max_length=3)
un = models.IntegerField('United Nations Code')
region = models.IntegerField('Region Code')
subregion = models.IntegerField('Sub-Region Code')
lon = models.FloatField()
lat = models.FloatField()
# GeoDjango-specific: a geometry field (MultiPolygonField)
mpoly = models.MultiPolygonField()
# Returns the string representation of the model.
def __str__(self): # __unicode__ on Python 2
return self.name
data migration:
python manage.py makemigrations
python manage.py migrate
You can see that the world_worldborder table has been generated in pgadmin3
Import data: create a new load.py under the world application
import os
from django.contrib.gis.utils import LayerMapping
from .models import WorldBorder
# Create your views here.
world_mapping = {
'fips' : 'FIPS',
'iso2' : 'ISO2',
'iso3' : 'ISO3',
'un' : 'UN',
'name' : 'NAME',
'area' : 'AREA',
'pop2005' : 'POP2005',
'region' : 'REGION',
'subregion' : 'SUBREGION',
'lon' : 'LON',
'lat' : 'LAT',
'mpoly' : 'MULTIPOLYGON',
}
world_shp = os.path.abspath(
os.path.join(os.path.dirname(__file__), 'data', 'TM_WORLD_BORDERS-0.3.shp'),
)
def run(verbose=True):
lm = LayerMapping(
WorldBorder, world_shp, world_mapping,
transform=False, encoding='iso-8859-1',
)
lm.save(strict=True, verbose=verbose)
Each key in the world_mapping dictionary corresponds to a field in the WorldBorder model. The value is the name of the shapefile field into which the data is loaded.
implement:
python manage.py shell
>>> from world import load >>> load . run ()
background management spatial data:
register the model in the project admin.py
from django.contrib.gis import admin from .models import WorldBorder admin . site . register ( WorldBorder , admin . GeoModelAdmin )
to create a superuser:
python manage.py createsuperuser
(requires username, password)
python manage.py runserver
Take a look at the effect:
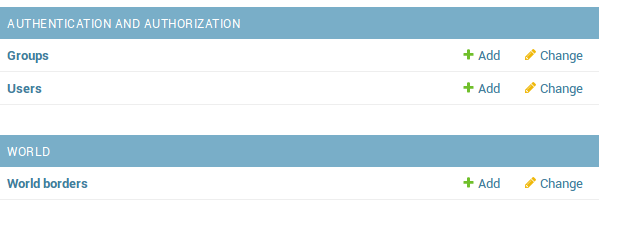
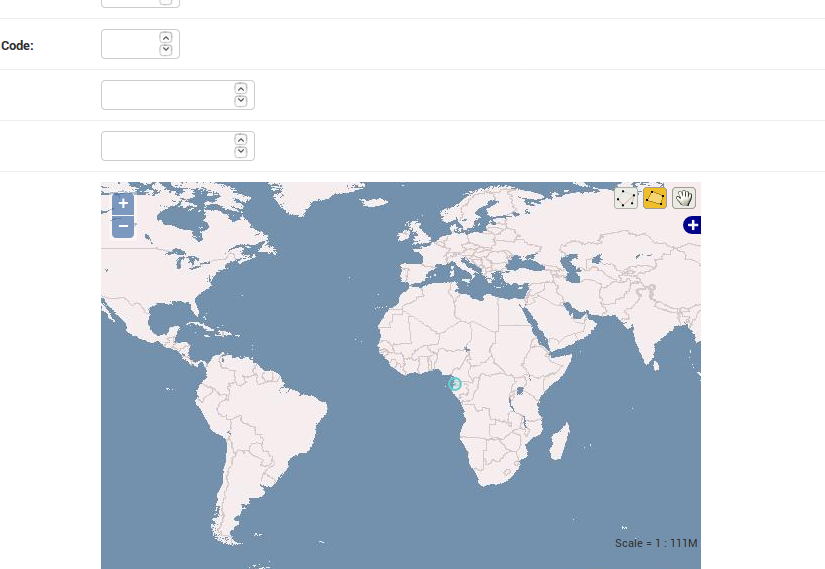
The above is just a simple test exercise, I will update it from time to time (if you have any questions, you can leave a message), everyone can learn together