homework requirement one
Submission list for PTA assignments
first job
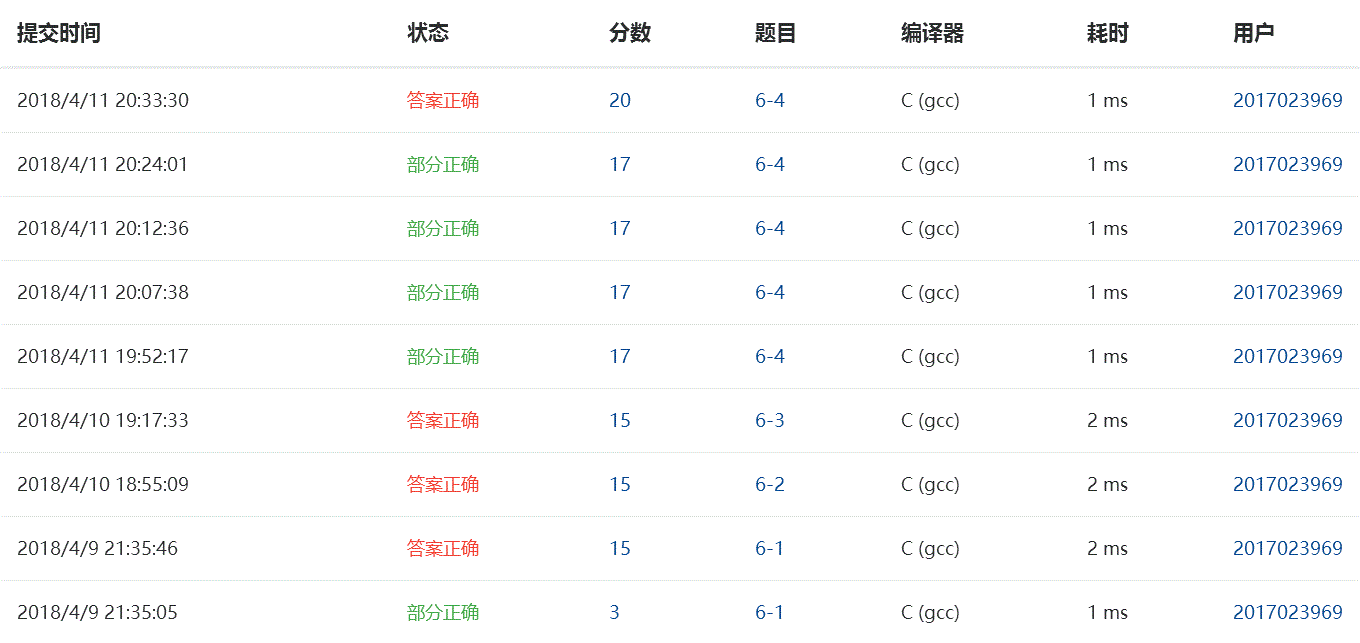
second job
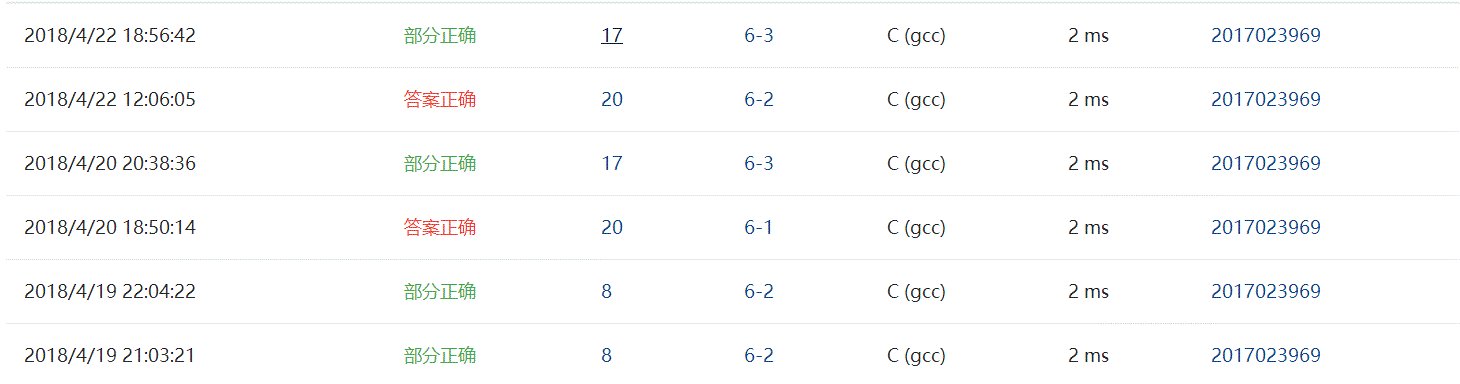
A programming question:
There is an array of axb, which sequentially stores numbers from 1 to a*b. Where a is the first three digits of your university number, and b is the last four digits of your university number. For example, if your student number is 2017023936, then the array size is 201 x 3936, and the array is sequentially stored from 1 to 791136 (201 and The integer of the product of 3936). It is required to use the screening method to find out the prime numbers in the array and print them out. The print format is 5 prime numbers on one line, and the numbers are separated by spaces.
The specific method of the screening method is to first arrange the N natural numbers in order. 1 is not a prime number, nor is it a composite number, it should be crossed out. The second number 2 is a prime number left over, and all numbers that are divisible by 2 after 2 are crossed out. The first number that is not crossed out after the 2 is 3, leave the 3, and then cross out all the numbers after the 3 that are divisible by 3. The first uncrossed number after the 3 is 5, leave the 5, and then cross out all the numbers after the 5 that are divisible by 5. Doing this all the time will filter out all composite numbers up to N, leaving all prime numbers up to N.
experimental code
#include <stdio.h>
int main(void)
{
int a, b;
scanf("%d %d", &a, &b);
int as[a*b], i, j;
for(i = 2; i <= a * b; i++)
as[i] = i;
for(i = 2; i <= (a * b) / i; i++)
for(j = i + i; j <= a * b; j = i + j)
as[j] = NULL;
for(i = 2, j = 0; i <= a * b; i++)
if(as[i] != NULL)
{
printf("%7d", as[i]);
j++;
if(j % 5 == 0)
printf("\n");
}
return 0;
}
homework requirement two
Question 1. Output the English name of the month (function question)
1. Design ideas
- (1) Algorithm
Step 1: Define a two-dimensional character array as a global variable.
Step 2: Call the defined function.
Step 3: Determine whether n is a month value, if so, return the corresponding string address; if not, make the address empty and return.
- (2) Flowchart: omitted.
2. Experimental code
char s[12][10]={"January","February","March","April","May","June","July","August","September","October","November","December"};
char *getmonth( int n )
{
char *month;
if(n > 0 && n <= 12)
{
month = s[n-1];
return month;
}
month = NULL;
return month;
}
full code
#include <stdio.h>
char *getmonth( int n );
int main()
{
int n;
char *s;
scanf("%d", &n);
s = getmonth(n);
if ( s==NULL ) printf("wrong input!\n");
else printf("%s\n", s);
return 0;
}
char s[12][10]={"January","February","March","April","May","June","July","August","September","October","November","December"};
char *getmonth( int n )
{
char *month;
if(n > 0 && n <= 12)
{
month = s[n-1];
return month;
}
month = NULL;
return month;
}
3. Problems encountered in the debugging process of this question and solutions
There is a bigger problem here.
When I did this problem, I used the switch statement to do it first, which is simple and easy to make mistakes. But after I finished it, I felt that the switch statement had little to do with the knowledge points we practiced recently, so I rewritten it in a different way, and there was a problem. It's actually easy to correct mistakes, but I've been slow to understand why. After several days of discussion and communication and the teacher's explanation, I finally understood that the error was caused by memory allocation.
Question 2. Find the week (function question)
1. Design ideas
- (1) Algorithm
Step 1: Call the defined function.
Step 2: Define a two-dimensional array representing the week.
Step 3: Use the loop statement to determine whether there is an array of characters that is the same as the input string. If there is, jump out of the loop, end the call; otherwise, return -1.
- (2) Flowchart
2. Experimental code
int getindex( char *s )
{
int i;
char day[7][10]={"Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"};
for(i = 0; i < 7; i++)
if(strcmp(s,day[i]) == 0)
break;
if(i == 7)
i = -1;
return i;
}
full code
#include <stdio.h>
#include <string.h>
#define MAXS 80
int getindex( char *s );
int main()
{
int n;
char s[MAXS];
scanf("%s", s);
n = getindex(s);
if ( n==-1 ) printf("wrong input!\n");
else printf("%d\n", n);
return 0;
}
int getindex( char *s )
{
int i;
char day[7][10]={"Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"};
for(i = 0; i < 7; i++)
if(strcmp(s,day[i]) == 0)
break;
if(i == 7)
i = -1;
return i;
}
3. Problems encountered in the debugging process of this question and solutions
without.
Question 3. Calculate the longest string length (function question)
1. Design ideas
- (1) Algorithm
Step 1: Call the defined function.
Step 2: Use the selection sort method to determine the length of the string.
Step 3: Return the longest string address.
- (2) Flowchart: omitted.
2. Experimental code
int max_len( char *s[], int n )
{
int i,t,k,j;
for(i=0;i<(n-1);i++)
{
k=i;
for(j=i+1;j<n;j++)
{
if(strlen(*(s+j))>strlen(*(s+k)))
{
k=j;
}
}
if(i!=k)
{
t=*(s+i); *(s+i)=*(s+k); *(s+k)=t;
}
}
return strlen(*s);
}
full code
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAXN 10
#define MAXS 20
int max_len( char *s[], int n );
int main()
{
int i, n;
char *string[MAXN] = {NULL};
scanf("%d", &n);
for(i = 0; i < n; i++) {
string[i] = (char *)malloc(sizeof(char)*MAXS);
scanf("%s", string[i]);
}
printf("%d\n", max_len(string, n));
return 0;
}
int max_len( char *s[], int n )
{
int i,t,k,j;
for(i=0;i<(n-1);i++)
{
k=i;
for(j=i+1;j<n;j++)
{
if(strlen(*(s+j))>strlen(*(s+k)))
{
k=j;
}
}
if(i!=k)
{
t=*(s+i); *(s+i)=*(s+k); *(s+k)=t;
}
}
return strlen(*s);
}
3. Problems encountered in the debugging process of this question and solutions
without.
Question 4. Specify the position to output a string (function question)
1. Design ideas
- (1) Algorithm
The first step: call the defined function, define the pointer, make it point to null.
Step 2: Use the double loop structure to judge whether there is a character matching ch1 in the input string in the outer loop. If so, the pointer points to s[i]; use the loop structure again, and judge whether there is a character in the input string in the inner loop. If the character matching ch2 exists, the pointer p is returned. If it does not exist, the pointer p is returned after the inner loop ends.
Step 3: If a character matching ch1 cannot be found in the input string in the outer loop, make the pointer point to '\0', and return the pointer p.
- (2) Flowchart: omitted.
2. Experimental code
char *match( char *s, char ch1, char ch2 )
{
int i, j;
char *p = NULL;
for(i = 0; s[i] != '\0'; i++)
{
if(s[i] == ch1)
{
p = &s[i];
for(j = i; s[j] != '\0'; j++)
{
if(s[j] != ch2)
printf("%c",s[j]);
else
{
printf("%c\n",s[j]);
return p;
}
}
printf("\n");
return p;
}
}
if(s[i] == '\0')
p = &s[i];
printf("\n");
return p;
}
full code
#include <stdio.h>
#define MAXS 10
char *match( char *s, char ch1, char ch2 );
int main()
{
char str[MAXS], ch_start, ch_end, *p;
scanf("%s\n", str);
scanf("%c %c", &ch_start, &ch_end);
p = match(str, ch_start, ch_end);
printf("%s\n", p);
return 0;
}
char *match( char *s, char ch1, char ch2 )
{
int i, j;
char *p = NULL;
for(i = 0; s[i] != '\0'; i++)
{
if(s[i] == ch1)
{
p = &s[i];
for(j = i; s[j] != '\0'; j++)
{
if(s[j] != ch2)
printf("%c",s[j]);
else
{
printf("%c\n",s[j]);
return p;
}
}
printf("\n");
return p;
}
}
if(s[i] == '\0')
p = &s[i];
printf("\n");
return p;
}
3. Problems encountered in the debugging process of this question and solutions
There are many wrong answers in test point 1 of this question.
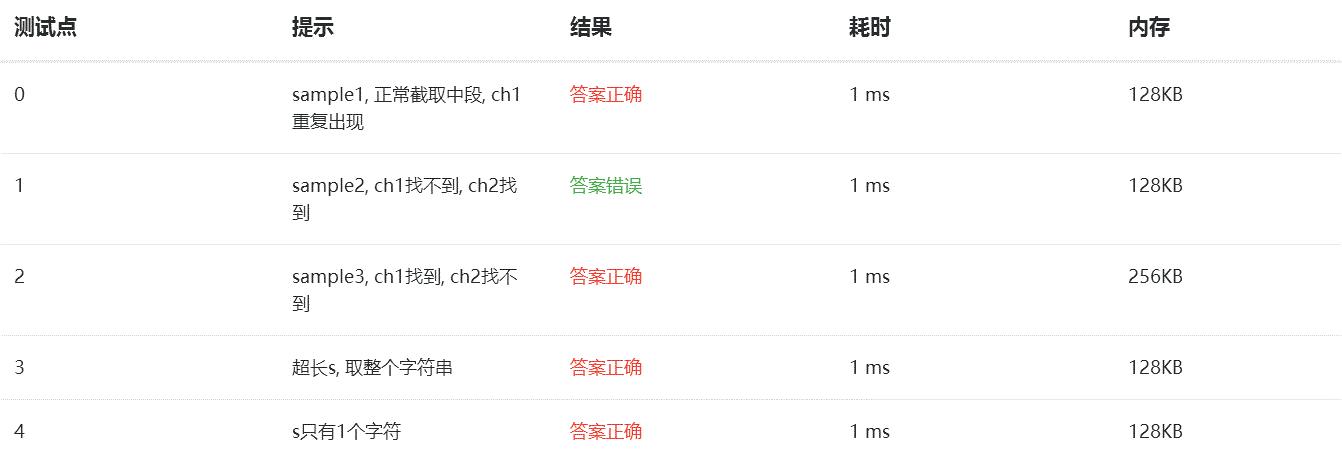
Error reason: When ch1 is not found and ch2 is found, the main function needs to output a blank line, but because the initial code defines the pointer to be empty, it cannot output a blank line.
Solution: Make the pointer point to the end character of the string to output a blank line.
Topic 5. Linked list of odd-valued nodes (function problem)
experimental code
#include <stdio.h>
#include <stdlib.h>
struct ListNode
{
int data;
struct ListNode *next;
};
struct ListNode *readlist();
struct ListNode *getodd( struct ListNode **L );
void printlist( struct ListNode *L )
{
struct ListNode *p = L;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main()
{
struct ListNode *L, *Odd;
L = readlist();
Odd = getodd(&L);
printlist(Odd);
printlist(L);
return 0;
}
struct ListNode *readlist()
{
struct ListNode *head=NULL,*tail=NULL,*p=NULL;
int data;
scanf("%d",&data);
while(data!=-1)
{
p=(struct ListNode *)malloc(sizeof(struct ListNode)); //动态内存分配
p->data = data; //赋值
p->next = NULL;
if(head == NULL) //判断是否为头指针
head=p;
else
tail->next=p;
tail=p; //使尾指针指向p所指向
scanf("%d",&data);
}
return head; //返回头指针
}
struct ListNode *getodd( struct ListNode **L )
{
struct ListNode *head=NULL,*head1=NULL,*p=NULL,*str=NULL,*str1=NULL; //动态内存分配
p=*L; //使p指针指向L的地址
if(p == NULL) //p指向为空时,返回0
return 0;
for(; p!=NULL; p=p->next)
{
if(p->data % 2 == 0) //判断变量p->data是否为奇数,将不是奇数的连接起来,形成链表
{
if(head == NULL)
head = p;
else
str->next = p;
str = p;
}
else //将是奇数的连接起来,形成新的链表
{
if(head1 == NULL)
head1 = p;
else
str1->next = p;
str1 = p;
}
}
if(str1 != NULL) //使链表最后指向空
str1->next = NULL;
if(str != NULL) //使链表最后指向空
str->next = NULL;
*L = head;
return head1;
}
Problems encountered in the debugging process of this problem and solutions
Question: Each linked list question has the same problem, that is, when you see the question, there is a feeling that you can't start.
Solution: Find relevant information and practice more.
Topic 6. Processing of Student Score List (Function Question)
experimental code
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stud_node
{
int num;
char name[20];
int score;
struct stud_node *next;
};
struct stud_node *createlist();
struct stud_node *deletelist( struct stud_node *head, int min_score );
int main()
{
int min_score;
struct stud_node *p, *head = NULL;
head = createlist();
scanf("%d", &min_score);
head = deletelist(head, min_score);
for ( p = head; p != NULL; p = p->next )
printf("%d %s %d\n", p->num, p->name, p->score);
return 0;
}
struct stud_node *createlist()
{
struct stud_node *head=NULL,*tail=NULL,*p=NULL; //动态分配空间
int num=0;
char name[20];
int score;
scanf("%d %s %d",&num,name,&score);
while(num!=0)
{
p=(struct stud_node *)malloc(sizeof(struct stud_node)); //动态分配空间
p->num=num; //赋值
strcpy(p->name,name);
p->score=score;
p->next = NULL;
if(head == NULL) //判断是否为头指针
head=p;
else
tail->next=p;
tail=p;
scanf("%d",&num);
if(num==0) //当输入num输入为0时,跳出,循环结束
break;
else //否则,输入,循环继续
scanf("%s %d",name,&score);
}
return head;
}
struct stud_node *deletelist( struct stud_node *head, int min_score )
{
struct stud_node *ptr = head, *ptr1=head; //动态分配内存
if(head==NULL) //p指向为空时,返回NULL
return NULL;
for(; ptr!=NULL; ptr=ptr->next)
{
if(ptr->score < min_score) //判断学生成绩是否小于设置的最低成绩
{
if(ptr==head) ////判断是否为头指针
head = ptr->next;
else
ptr1->next = ptr->next;
free(ptr); //动态分配释放
ptr=ptr1;
}
ptr1 = ptr;
}
return head;
}
Problems encountered in the debugging process of this problem and solutions
Question: as pictured
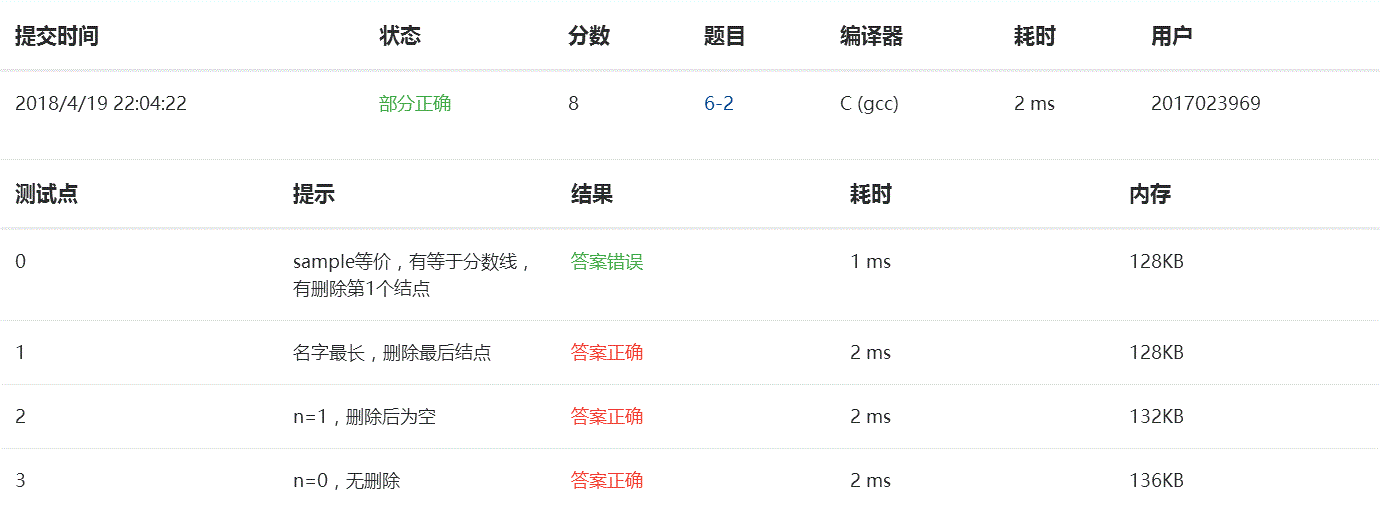
Solution: When the student's grade is less than the set minimum grade, ptr=ptr1 is not set, just add it.
Topic 7. Linked list splicing (function question)
experimental code
Problems encountered in the debugging process of this problem and solutions
This question is not written correctly at the end of the PTA time, and there is a timeout error.
struct ListNode *mergelists(struct ListNode *list1, struct ListNode *list2)
{
struct ListNode *head1=list1, *head2=list2, *p1=head1, *p2=head2, *str1=NULL, *str2=NULL,*q=NULL;
if(p1==NULL)
return head2;
if(p2==NULL)
return head1;
for(;p2!=NULL;p2=str2)
{
str2=p2->next;
q=p2;
for(; p1!=NULL;p1=str1->next)
{
if(p2->data <= p1->data)
{
p2->next = p1;
if(p1==head1)
head1 = p2;
else
str1->next = p2;
str1=q;
break;
}
str1 = p1;
}
if(p1==NULL)
str1->next = head2;
}
return head1;
}
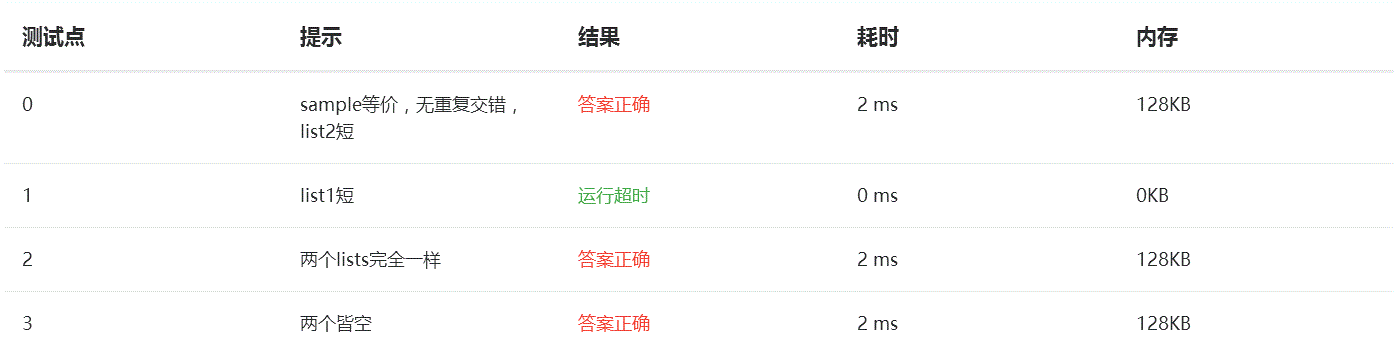
homework requirement three
1. Learning summary
(1) How to understand an array of pointers, and how does it relate to pointers and arrays? Why can you operate on arrays of pointers with secondary pointers?
Answer: An array of pointers is an array whose elements are pointers, and each element in this array is a pointer to an address. Secondary pointers can operate on the address of an array of pointers.
(2) Change any topic of the third PTA assignment (1) of C Advanced to use the second-level pointer to operate on the pointer array.
output month English name
char *getmonth( int n ) {
char *s[12] = {"January","February","March","April","May","June","July","August","September","October","November","December"};
char **p = &s, *q = '\0';
if(n > 0 && n <= 12)
q = *(p+n-1);
return q;
}
(3) What are the advantages of handling multiple strings with an array of pointers? Is it possible to directly input multiple strings to an uninitialized array of pointers? Why?
A: When each string has a different length, it saves space and is less prone to memory allocation problems.
Can not. Because an uninitialized pointer may point to some indeterminate address.
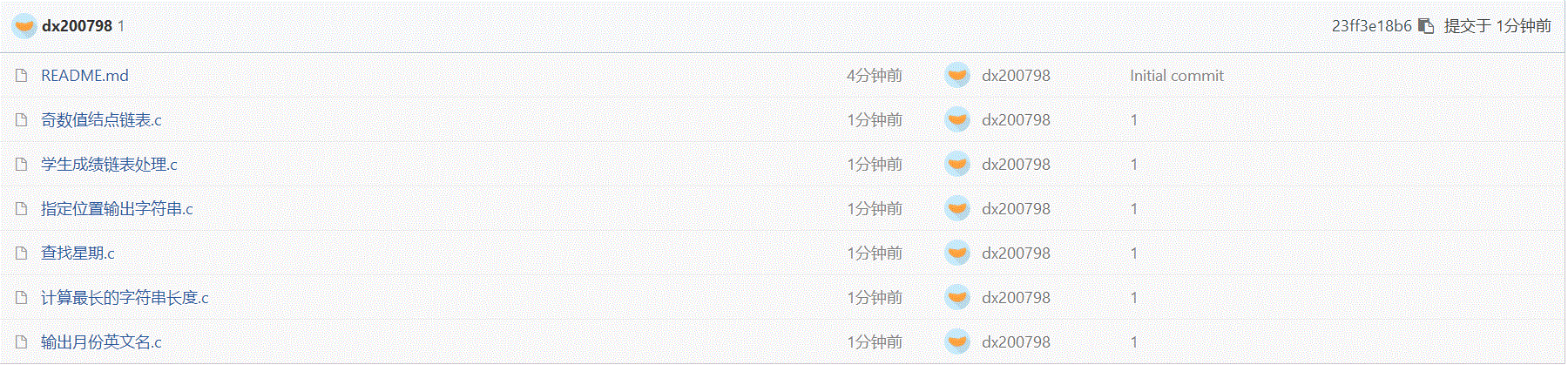
3. Review link
4. Charts
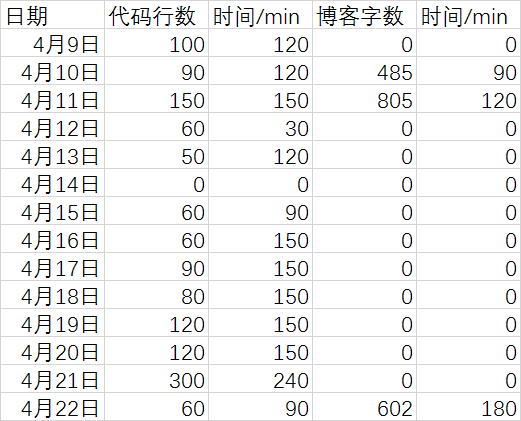
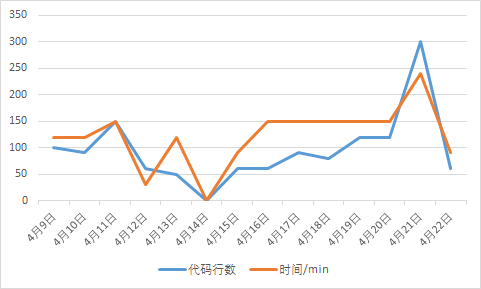
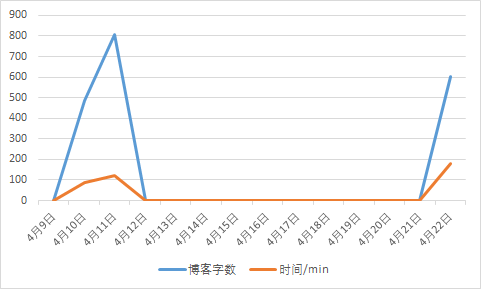