content
2. Method definition and invocation
The main content of this chapter:
3. Definition and invocation of methods with parameters
Format 1: (method definition with static)
Error example 1: The parameter types are different
Error example 2: The number of parameters is different
Format 2: No static in the method
4. Definition and call of parameter method with return value
5. Nested definitions and nested calls
1. Transfer of basic data types
2, the transfer of reference data types
Concept of reference data type:
I. Introduction
Earlier we learned the concept and use of arrays, now let's learn the method in Java, this method and the c language
The functions are very similar.
2. Methods
1. Overview of the method
2. Method definition and invocation
3. Definition and invocation of methods with parameters
4. Definition and invocation of methods with return values
5. Notes on the method
6. Method overloading
7 parameter passing of methods
1. Overview of the method
A method is a piece of code with a certain function, and we use this to solve a problem, which is a collection of pieces of code.
(equivalent to functions in c language)
2. Method definition and invocation
method definition
Format 1:
public static void 方法名{
//方法的内容
}
Where static indicates that this is a static method. Static members and static methods can only access static members and methods.
To access non-static members or methods, you need to create an object to access it, such as class name identifier = new class name ();
Non-static members can access both static members and methods as well as non-static members and methods.
(This is the content of the later stage, and it will be covered in advance)
Example image 1:
Example Figure 2:
Code 1:
package com.haha;
public class test {
public static void main(String[] args) {
hello();//在同一类下,静态成员之间的访问直接通过 方法名();
}
public static void hello(){
System.out.println("好好学习,天天编程");
}
}
Code 2:
package com.haha;
public class test1 {
public static void main(String[] args) {
test1 nb=new test1();//创建对象
nb.hello();//若静态方法想访问非静态方法,则得通过创建对象才能访问
}
public void hello(){//没有static表示非静态方法
System.out.println("好好学习,天天编程");
}
}
Format 2:
public 返回类型 方法名(){
//方法定义的实现
}
public int haha(){
}
In fact, the definition method is very simple, just like the function of C language, but there are some more modifiers public in java
, private, protected, etc. modifiers, which are used for access rights.
Modifier Access Scope:
The main content of this chapter:
1. Definition and invocation of methods with parameters
2. Method definition and call with return value
3. Definition and invocation of methods with parameters
Method definition:
Format 1: (method definition with static)
public static void 方法名(参数){
//方法内容
}
Example:
public static void compare(int a,int b){
System.out.println(a>b?a:b);
}
Note: If a program wants to run, it needs to have a main method, and the program is entered from the main method. So compare
The full writing is:
sample graph: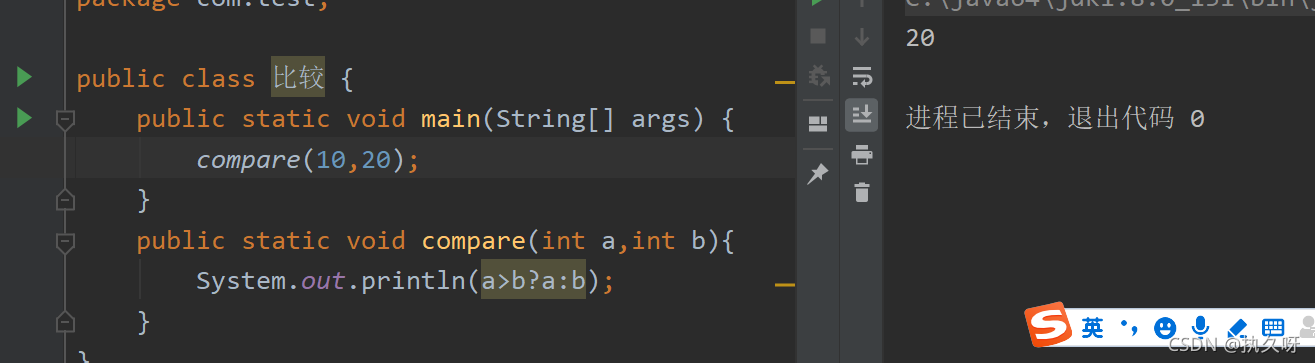
Code:
package com.test;
public class 比较 {
public static void main(String[] args) {
compare(10,20);
}
public static void compare(int a,int b){
System.out.println(a>b?a:b);
}
}
Note : When defining a method, the parameters in the call should correspond one-to-one with the parameters in the method, and the parameter types should also be one-to-one
Yes, otherwise an error will be reported. As the compare(int, int) method in the above figure defines two methods of type int, then in other ways
The method (not necessarily the main method) also needs to write two parameters of type int. In fact, methods can be overloaded, and overloading is the parameter
At least one of the numbers or parameter types is different... (in the next part)
Error Example 1: Different parameter types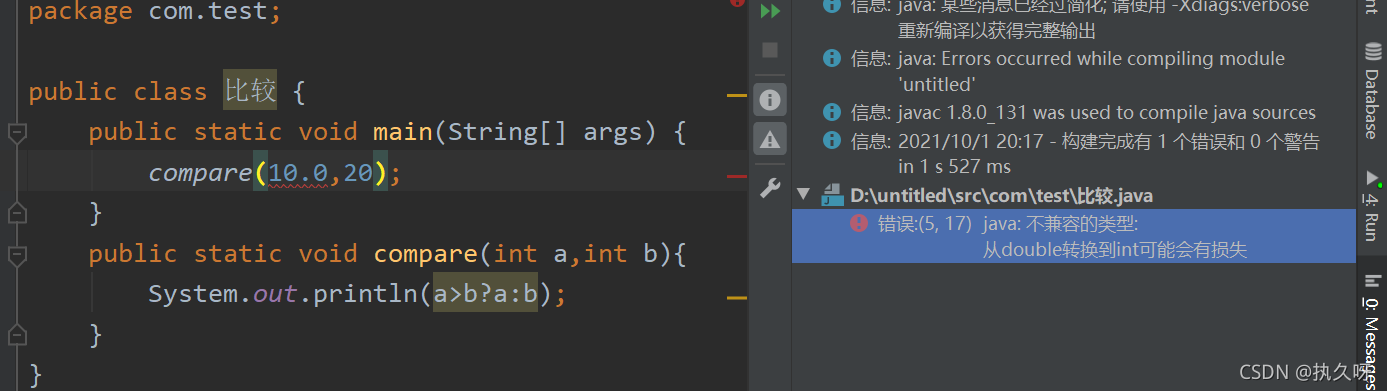
Error example 2: The number of parameters is different
Format 2: No static in the method
This when the main method calls him, you need to create the object in the main method first (said before)
In the same class, other methods without static can directly call other methods (as mentioned in the previous article, not repeated)
Method call:
The format is: method name();
Is it super simple, where the parameters in parentheses should be consistent with the defined parameters.
Such as: compare(10,20); you can also count the parameters through Scanner
4. Definition and call of parameter method with return value
Look carefully to see if there are only three words with the return value added to the above one. In fact, this definition is also more than this one.
Method definition format:
(It is also divided without static, the same as above, so I will not talk about it separately)
public static 返回类型 方法名(参数){
//方法体
return 数据
}
Such as:
public static int compare(int a,int b){
return a>b?a:b;
}
Note: The method with return value must have return to return more than one data, otherwise an error will be reported, and the return type is
For void, there can be a return, but nothing is followed after the return, just return;
Example: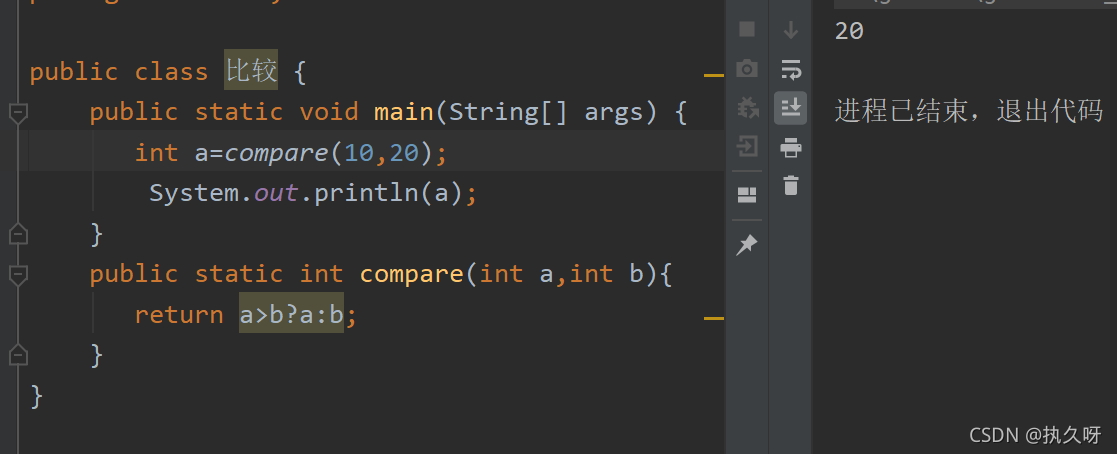
Code:
package com.test;
public class 比较 {
public static void main(String[] args) {
int a=compare(10,20);
System.out.println(a);
}
public static int compare(int a,int b){
return a>b?a:b;
}
}
Note: When calling a method, there must be a variable to accept the return type (if it is not received, it will not report an error), there is no any
It doesn't make sense for a variable to accept the return value
5. Nested definitions and nested calls
Methods cannot be defined nested, but can be called nested,
What is a nested definition , in fact, a method wraps another method
What is nested application, that is, the calls of various methods, a method can call one or more,
For example, method a calls method b, and method b calls methods c, d, and so on.
Such as
Wrong spelling:
public static void main(String[] args){
public static void getmax(int a,int b){
}
}
At this point there is a problem, the main method nests the getmax method.
Correct spelling:
public static void main(String[] args){
}
public static void getmax(int a,int b){
}
6. Method overloading
We know that methods are commonly used in java and are often used in the process of writing code, but we are calling the same
When the method of the function needs to pass different types of data, it is inconvenient if we define many methods, so in java
Out of method overloading.
What is method overloading?
Overloading is also called rewriting, and it satisfies the following conditions:
1. The same method name
2. The type of data is different or the number of data is different or the order is different (three can satisfy one or more)
3. The defined methods are placed under the same class
Such as: overloading of the initial method
public static void add(float f,int i)
The following are overloads of the above method:
Different kinds:
public static void add(float f,float i)
public static void add(int a,int n)
The number of data is different:
public static void add(float f,int i,float v)
The order is different:
public static void add(int i,float f)
Note: The difference in the order must be that the two data types have changed positions, not the parameter name. The value of the parameter name is arbitrary
Wrong spelling:
public static void add(float i,int f)
Note: method overloading has nothing to do with return type
The following two belong to the same method:
public static int add(float f,int i)
public static void add(float f,int i)
7. Method parameter passing
1. Transfer of basic data types
The so-called several data types are 8 basic data types ( byte char short int boolean float long double)
For the transfer of basic data types, the change of the formal parameter will not change the value of the actual parameter
This is equivalent to call by value in C language.
Such as:
Code:
public class Demo {
public static void main(String[] args) {
int a=10;
play(a);
System.out.println("实参的a的值是"+a);
}
public static void play(int a){
a=100;
System.out.println("形参中a的值是"+a);
}
}
Demo run graph:
2, the transfer of reference data types
In addition to the 8 basic data types, the rest are reference data types
Concept of reference data type:
A reference type is a data type that stores not a value but an address in memory only in a variable. That is to say
The address in the memory where the value of this variable is stored is stored in the variable. Every time this variable is called, it refers to this
address and get the real value so it is called reference type
Reference type:
It is an object type, and its value is a reference to the memory space, which is actually the value stored in the memory pointed to by the address
To put it bluntly, pass-by-reference is the pass-by-reference in C language, pass -by-reference, and actual parameters will change with the change of formal parameters.
Demo image:
Code:
public class Demo1 {
public static void main(String[] args) {
int[]arr={1,2,3,4};
System.out.println("调用前arr[0]:"+arr[0]);
arrDemo(arr);
System.out.println("调用后arr[0]:"+arr[0]);
}
public static void arrDemo(int[]arr){
arr[0]=10;
}
}