1.
Stack
(Stack)
1.1 Concept
Stack: A special linear list that allows insertion and removal of elements only at one fixed end. One end where data insertion and deletion are performed is called the top of the stack, and the other end is called the bottom of the stack. The data elements in the stack follow the LIFO
(
Last In First Out
) principle.
Push stack: The insertion operation of the stack is called push
/
push
/ push
, and the pushed
data is at the top of the stack
.
Pop: The deletion of the stack is called pop.
The output data is on the top of the stack.
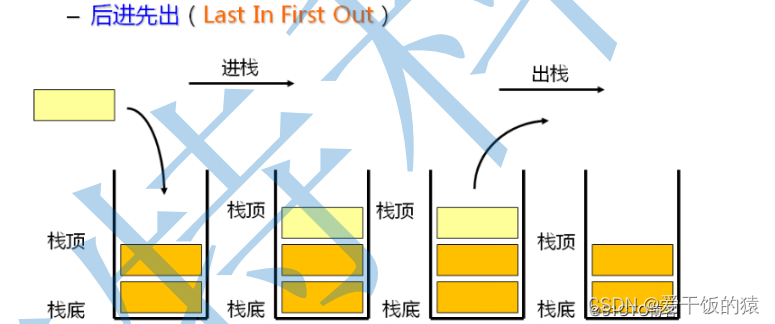
1.2 Implementation
1. Use the sequence table to achieve, that is, use the method of tail insertion
+
tail deletion
2.
Use linked list to achieve, then both head and tail can be
Relatively speaking, the implementation of the sequence table is simpler, so we prefer to use the sequence table to implement the stack.
Array-based sequential stack, the implementation code is as follows:
import java.util.ArrayList;
import java.util.List;
import java.util.NoSuchElementException;
/**
* 基于数组的顺序栈实现
* @param <E>
*/
public class MyStack<E> {
// 当前栈的数据个数
private int size;
// 实际存储数据的动态数组 - ArrayList
private List<E> data=new ArrayList<>();
//入栈
public void push(E val){
//尾插
data.add(val);
size++;
}
//出栈,并返回栈顶元素
public E pop(){
if(isEmpty()){
// 栈为空,没有栈顶元素
throw new NoSuchElementException("stack is empty! cannot pop!");
}
// 删除栈顶元素
E val = data.remove(size - 1);
size --;
return val;
// 等同于 return data.remove(--size);
}
//只返回栈顶元素
public E peek(){
if(isEmpty()){
// 栈为空,没有栈顶元素
throw new NoSuchElementException("stack is empty! cannot peek!");
}
return data.get(size-1);
}
//判断栈是否为空
public boolean isEmpty(){
return size == 0;
}
@Override
public String toString() {
StringBuilder sb=new StringBuilder();
sb.append("[");
for (int i = 0; i < size; i++) {
sb.append(data.get(i));
if(i!=size-1){
// 此时还没到栈顶,还没到数组末尾
sb.append(",");
}
}
sb.append("]");
return sb.toString();
}
}
The reference method is as follows:
public class StackTest {
public static void main(String[] args) {
MyStack<Integer> stack=new MyStack<>();
stack.push(1);
stack.push(2);
stack.push(3);
//打印栈所有元素
System.out.println(stack);
//打印栈顶元素
System.out.println(stack.peek());
//出栈,并打印栈顶元素
stack.pop();
System.out.println(stack);
}
}
Based on the above input results are as follows:
2.
Queue
_
2.1 Concept
Queue: A special linear table that only allows data insertion operations at one end and deletion data operations at the other end. The queue has a first-in-first-out (First In First Out) entry queue: The end of the insertion operation is called the tail of the queue ( Tail/ Rear ) out of the queue: the end of the deletion operation is called the head of the queue
first in first out
2.2 Implementation
The queue can also be implemented in the structure of an array and a linked list. It is better to use the structure of a linked list, because if the structure of an array is used, the efficiency of dequeuing and outputting data at the head of the array will be relatively low.
The basic queue implemented based on linked list, the implementation code is as follows:
Interface class:
public interface IQueue<E> {
// 入队
void offer(E val);
//出队
E poll();
//返回队首元素
E peek();
//判断队列是否为空
boolean isEmpty();
}
Queue class:
import stack_queue.queue.IQueue;
import java.util.NoSuchElementException;
/**
* 基于链表实现的基础队列
* @param <E>
*/
public class MyQueue<E> implements IQueue<E> {
// 链表的每个节点
private class Node{
E val;
Node next;
public Node(E val){
this.val=val;
}
}
// 当前队列中的元素个数
private int size;
// 队首
private Node head;
//队尾
private Node tail;
@Override
public void offer(E val) {
Node node=new Node(val);
if(head==null){
// 此时链表为空
head=tail=node;
}else {
tail.next=node;
tail=node;
}
size++;
}
@Override
public E poll() {
if(isEmpty()){
throw new NoSuchElementException("queue is empty! cannot poll");
}
Node node=head;
head=node.next;
// 将原来头节点脱钩
node.next=null;
size--;
return node.val;
}
@Override
public E peek() {
if(isEmpty()){
throw new NoSuchElementException("queue is empty! cannot peek");
}
return head.val;
}
@Override
public boolean isEmpty() {
return size==0;
}
@Override
public String toString() {
StringBuilder sb=new StringBuilder();
sb.append("[");
// 链表的遍历
for(Node x=head;x!=null;x=x.next){
sb.append(x.val);
if(x.next!=null){
// 还没走到链表尾部
sb.append(",");
}
}
sb.append("]");
return sb.toString();
}
}
The reference method is as follows:
import stack_queue.queue.impl.MyQueue;
public class QueueTest {
public static void main(String[] args) {
IQueue iQueue=new MyQueue();
iQueue.offer(1);
iQueue.offer(2);
iQueue.offer(3);
System.out.println(iQueue);
System.out.println(iQueue.peek());
iQueue.poll();
System.out.println(iQueue);
}
}
Based on the above input results are as follows: