Table of contents
1.3 Implementation of stackEdit
2.3 Queue simulation implementation
1. 栈(Stack)
1.1 Concept
Stack: a speciallinear table whoseInsertion and deletion of elements are only allowed at the fixed end. One end where data insertion and deletion operations are performed is called the top of the stack, and the other end is called the bottom of the stack. The data elements in the stack comply withLIFOLIFO(Last In First Out) principle.
Push:The insertion operation of the stack is called push/push/Push, < /span>. The input data is on the top of the stack
Pop:The deletion operation of the stack is called popping. Put the data on the top of the stack
1.2 use
Sample code:
public static void main(String[] args) {
Stack<Integer> s = new Stack();
s.push(1);
s.push(2);
s.push(3);
s.push(4);
System.out.println(s.size()); // 获取栈中有效元素个数---> 4
System.out.println(s.peek()); // 获取栈顶元素---> 4
s.pop(); // 4出栈,栈中剩余1 2 3,栈顶元素为3
System.out.println(s.pop()); // 3出栈,栈中剩余1 2 栈顶元素为3
if(s.empty()){
System.out.println("栈空");
}else{
System.out.println(s.size());
}
}
1.3 realistic appearance
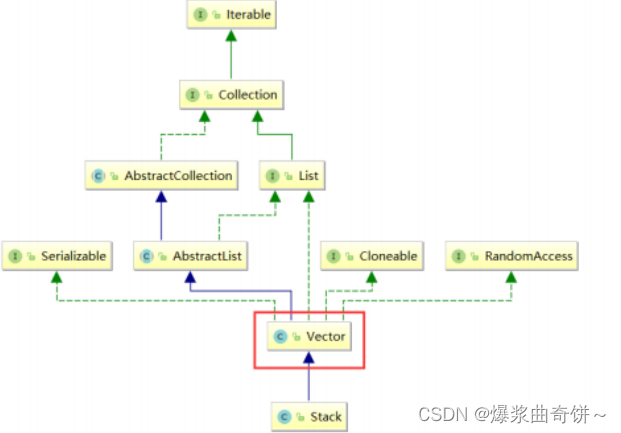
2. Queue(Queue)
2.1 Concept
Queue: A special linear table that only allows insertion of data at one end and deletion of data at the other end. The queue has a first-in-first-outFIFO (First In First Out).
Enqueue:The end where the insertion operation is performed is calledthe tail of the queue (Tail/Rear).
Dequeue: The end that performs the deletion operation is called the queue head (< a i=4>Head/Front).
2.2 Use of queue
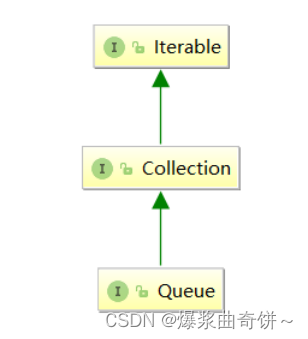
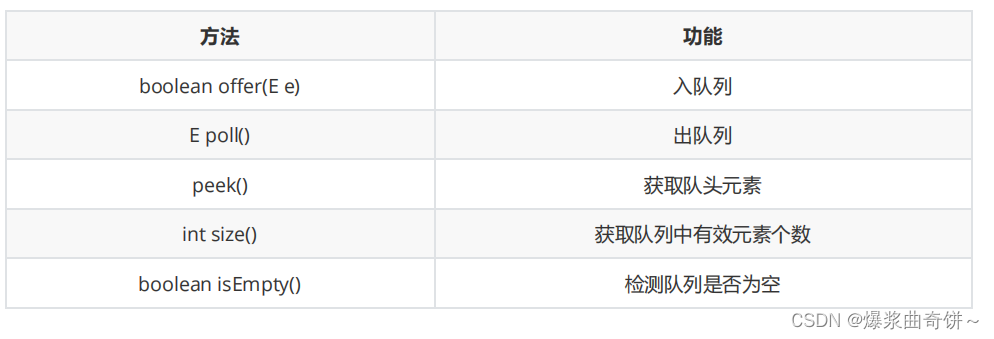
Note:Queue is an interface and must be instantiated when instantiatingLinkedList object, becauseLinkedList implements the Queue interface .
Sample code:
public static void main(String[] args) {
Queue<Integer> q = new LinkedList<>();
q.offer(1);
q.offer(2);
q.offer(3);
q.offer(4);
q.offer(5); // 从队尾入队列
System.out.println(q.size());
System.out.println(q.peek()); // 获取队头元素
q.poll();
System.out.println(q.poll()); // 从队头出队列,并将删除的元素返回
if(q.isEmpty()){
System.out.println("队列空");
}else{
System.out.println(q.size());
}
}
2.3 Queue simulation implementation
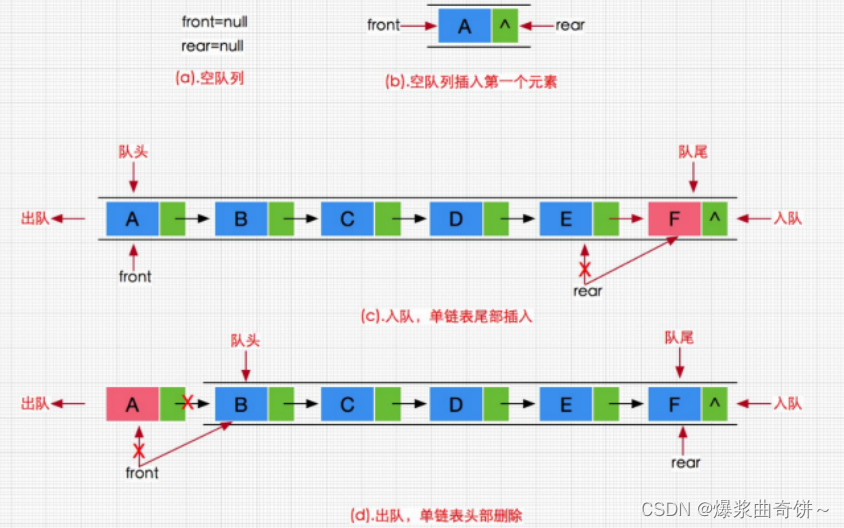
2.4 Cycling train
In practice, we sometimes use a queue called a circular queue. For example, when the operating system course explains the producer-consumer model, circular queues can be used.Ring queues are usually implemented using arrays.
3. double-end column (Deque)
The two -terminal queue (Dequ ) refers to the queue that allows both ends to enter the team and the team, deque is the abbreviation of "double ended queue" . That means that elements can be dequeued and entered from the head of the team, and can also be dequeued and entered from the end of the team.
Deque is an interface. When using it, you must create an object ofLinkedList
In actual projects, the Deque interface is commonly used, and both stacks and queues can use this interface.
Deque<Integer> stack = new ArrayDeque<>();//双端队列的线性实现
Deque<Integer> queue = new LinkedList<>();//双端队列的链式实现