Title description
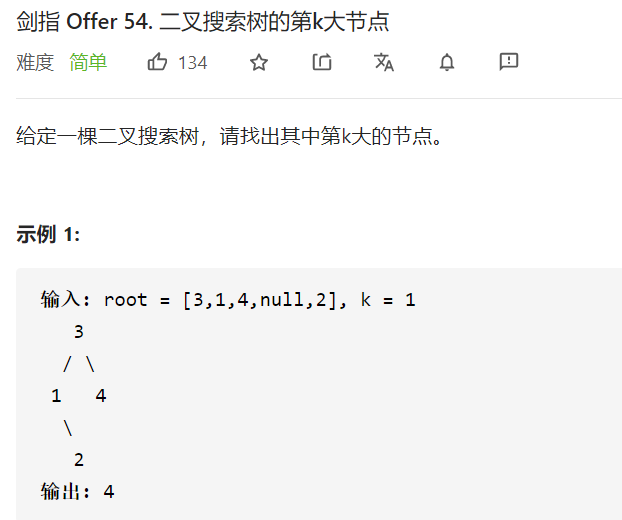
Problem-solving ideas
- First build a collection to store all the elements traversed.
- Use DFS to traverse the binary tree and store the result in the collection.
- Convert collection to array
- Sort in descending order by the sort method
- The element with subscript [k-1] of the sorted array is the K-th largest element of the binary search tree
Implementation code
var kthLargest = function(root, k) {
const set = new Set();
const dfs = function(node) {
if (node === null) {
return;
}
set.add(node.val);
dfs(node.left);
dfs(node.right);
}
dfs(root);
const arr = [...set];
arr.sort((a,b) => {
return b-a;
});
return arr[k-1];
};