[LeetCode] 390. Elimination Game (Medium) (JAVA)
Title address: https://leetcode.com/problems/elimination-game/
Title description:
There is a list of sorted integers from 1 to n. Starting from left to right, remove the first number and every other number afterward until you reach the end of the list.
Repeat the previous step again, but this time from right to left, remove the right most number and every other number from the remaining numbers.
We keep repeating the steps again, alternating left to right and right to left, until a single number remains.
Find the last number that remains starting with a list of length n.
Example:
Input:
n = 9,
1 2 3 4 5 6 7 8 9
2 4 6 8
2 6
6
Output:
6
General idea
Given a list of integers sorted from 1 to n.
First, from left to right, starting from the first number, delete every other number until the end of the list.
The second step is to delete every other number from right to left from right to left, and delete every other number until the beginning of the list.
We keep repeating these two steps, alternating from left to right and from right to left, until only one number remains.
Return the last remaining number in the list of length n.
Problem-solving method
1 2 3 4 5 6 7 8 9
2 4 6 8
2 6
6
1 2 3 4 5 6 7 8 9
1 2 3 4 -> k * 2
1 2 -> (k * 2 - 1) * 2
2 -> (2 * 2 - 1) * 2
- Recursive method can be used
- From left to right: In fact, the first time is to delete all the odd numbers, only the even numbers are left. If you divide all the numbers by 2, you will find that the element k is still found in [1, n / 2], and the result is k * 2 is enough
- From right to left: At this time, if the number is an even number, all the even-numbered bits are deleted and the odd number is left, such as: a total of ten numbers n = 10, and the rest is [1, 3, 5, 7, 9 ] -> [1 * 2-1, 2 * 2-1, …, 5 * 2-1], can be converted to 1, n/2 to find the element k, and then the result is k * 2-1
- From right to left: If the number is an odd number, all odd bits are deleted, leaving the even number: Divide all the numbers by 2, you will find that the element k is still found in [1, n / 2], and then the result k * 2 is enough, similar to the odd number algorithm
- In the end, the recursion continues until only 1 element remains
class Solution {
public int lastRemaining(int n) {
return lH(n, true);
}
public int lH(int n, boolean right) {
if (n <= 1) return n;
if (!right && (n % 2) == 0) {
return lH(n / 2, !right) * 2 - 1;
}
return lH(n / 2, !right) * 2;
}
}
Execution time: 3 ms, defeating 98.59% of Java users
Memory consumption: 37.3 MB, defeating 60.73% of Java users
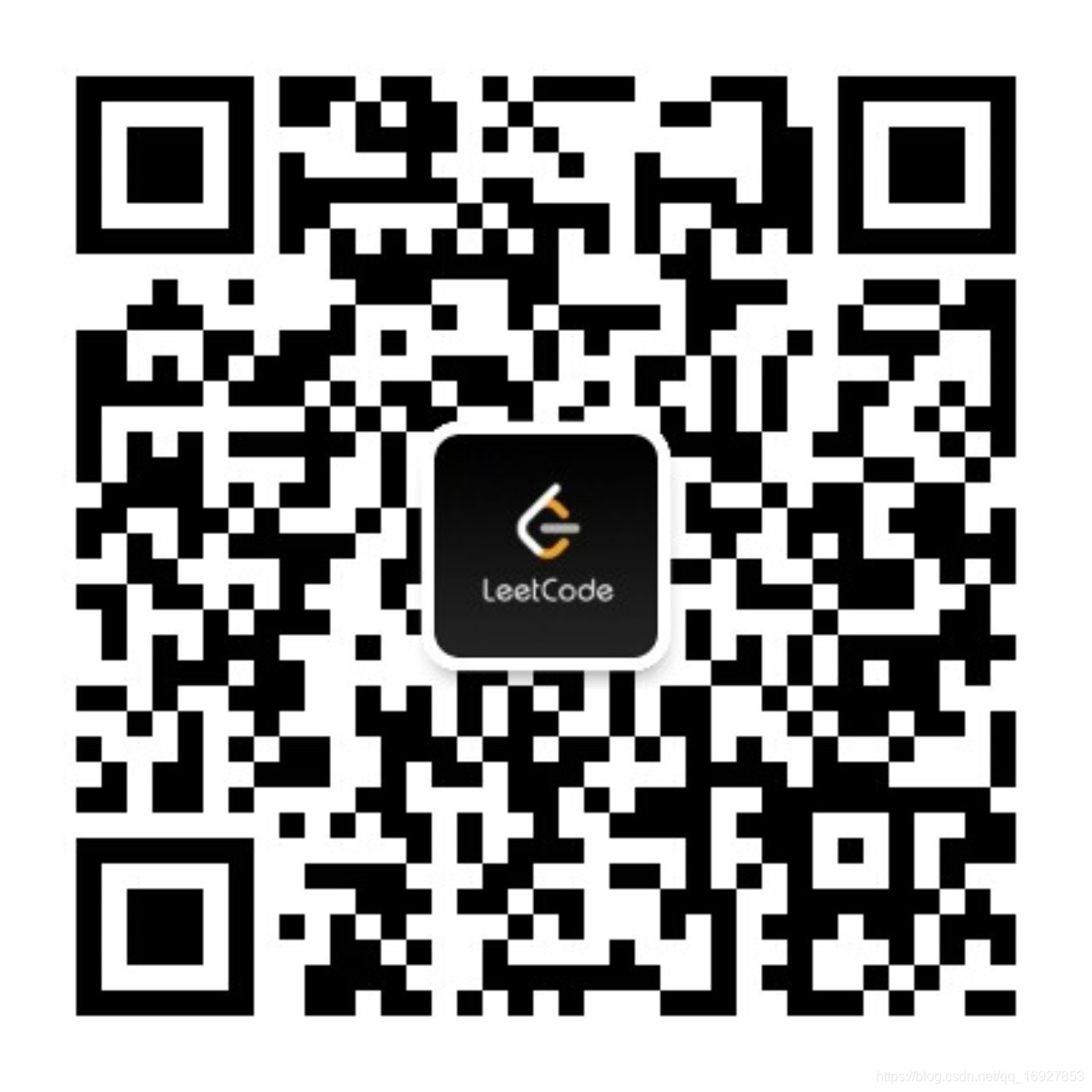