【LeetCode】386. Lexicographical Numbers (Medium) (JAVA)
Title address: https://leetcode.com/problems/lexicographical-numbers/
Title description:
Given an integer n, return 1 - n in lexicographical order.
For example, given 13, return: [1,10,11,12,13,2,3,4,5,6,7,8,9].
Please optimize your algorithm to use less time and space. The input size may be as large as 5,000,000.
General idea
Given an integer n, return the lexicographic order from 1 to n.
E.g,
Given n=1 3, return [1,10,11,12,13,2,3,4,5,6,7,8,9].
Please optimize the time complexity and space complexity of the algorithm as much as possible. The input data n is less than or equal to 5,000,000.
Problem-solving method
- If you want to sort lexicographically, you must sort all the ones that start with 1 first, and then the ones that start with 2.
- Count all numbers starting with 1 first
1 -> 1 * 10 + 0, 1 * 10 + 1, ..., 1 * 10 + 9
/ \
(1 * 10 + 0) * 10 + 0, (1 * 10 + 0) * 10 + 1, ..., (1 * 10 + 0) * 10 + 9
- Calculate in the form of recursion, put 1 first, then 10 + i, calculate 100 + i before 10 + 1, and continue to recurse until n
class Solution {
public List<Integer> lexicalOrder(int n) {
List<Integer> list = new ArrayList<>();
for (int i = 1; i < 10; i++) {
if (i > n) break;
list.add(i);
lH(list, n, i);
}
return list;
}
public void lH(List<Integer> list, int n, int num) {
num *= 10;
for (int i = 0; i < 10; i++) {
if (num + i > n) break;
list.add(num + i);
lH(list, n, num + i);
}
}
}
Execution time: 2 ms, defeating 96.39% of Java users
Memory consumption: 44.2 MB, defeating 61.26% of Java users
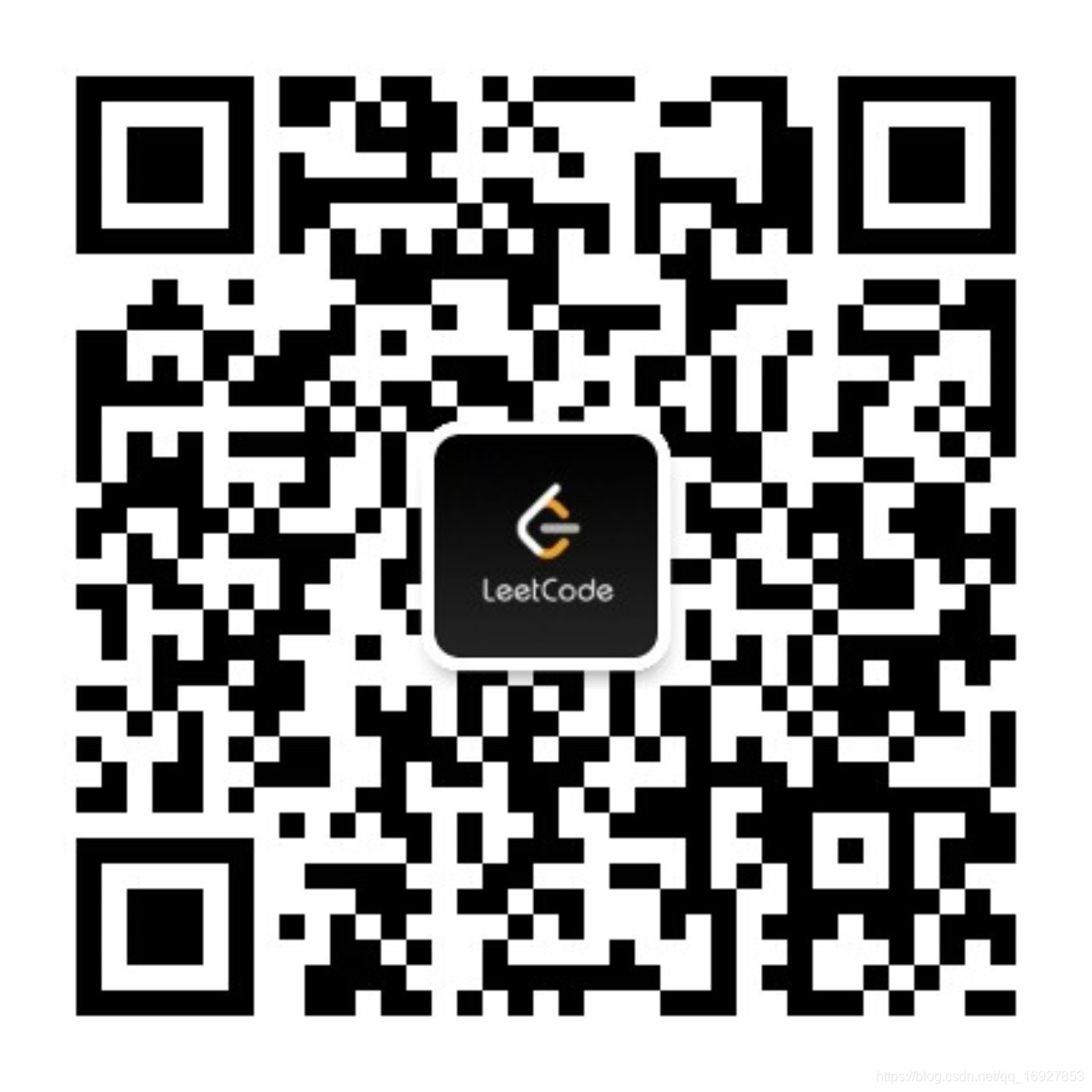