【LeetCode】832. Flipping an Image (Easy) (JAVA)
Title address: https://leetcode.com/problems/perfect-squares/
Title description:
Given a binary matrix A, we want to flip the image horizontally, then invert it, and return the resulting image.
To flip an image horizontally means that each row of the image is reversed. For example, flipping [1, 1, 0] horizontally results in [0, 1, 1].
To invert an image means that each 0 is replaced by 1, and each 1 is replaced by 0. For example, inverting [0, 1, 1] results in [1, 0, 0].
Example 1:
Input: [[1,1,0],[1,0,1],[0,0,0]]
Output: [[1,0,0],[0,1,0],[1,1,1]]
Explanation: First reverse each row: [[0,1,1],[1,0,1],[0,0,0]].
Then, invert the image: [[1,0,0],[0,1,0],[1,1,1]]
Example 2:
Input: [[1,1,0,0],[1,0,0,1],[0,1,1,1],[1,0,1,0]]
Output: [[1,1,0,0],[0,1,1,0],[0,0,0,1],[1,0,1,0]]
Explanation: First reverse each row: [[0,0,1,1],[1,0,0,1],[1,1,1,0],[0,1,0,1]].
Then invert the image: [[1,1,0,0],[0,1,1,0],[0,0,0,1],[1,0,1,0]]
Notes:
- 1 <= A.length = A[0].length <= 20
- 0 <= A[i][j] <= 1
General idea
Given a binary matrix A, we want to flip the image horizontally first, then invert the image and return the result.
To flip a picture horizontally is to flip each line of the picture, that is, reverse the order. For example, the result of horizontal flipping [1, 1, 0] is [0, 1, 1].
Inverting the picture means that all 0s in the picture are replaced by 1s, and all 1s are replaced by 0s. For example, the result of reversing [0, 1, 1] is [1, 0, 0].
Problem-solving method
- To flip each line, just change the position of the head and tail
- While exchanging, the numbers are also reversed
class Solution {
public int[][] flipAndInvertImage(int[][] A) {
for (int i = 0; i < A.length; i++) {
int start = 0;
int end = A[i].length - 1;
while (start < end) {
int temp = A[i][start];
A[i][start] = A[i][end] == 1 ? 0 : 1;
A[i][end] = temp == 1 ? 0 : 1;
start++;
end--;
}
if (start == end) A[i][start] = A[i][start] == 1 ? 0 : 1;
}
return A;
}
}
Execution time: 0 ms, beating 100.00% of Java users
Memory consumption: 38.6 MB, beating 67.02% of Java users
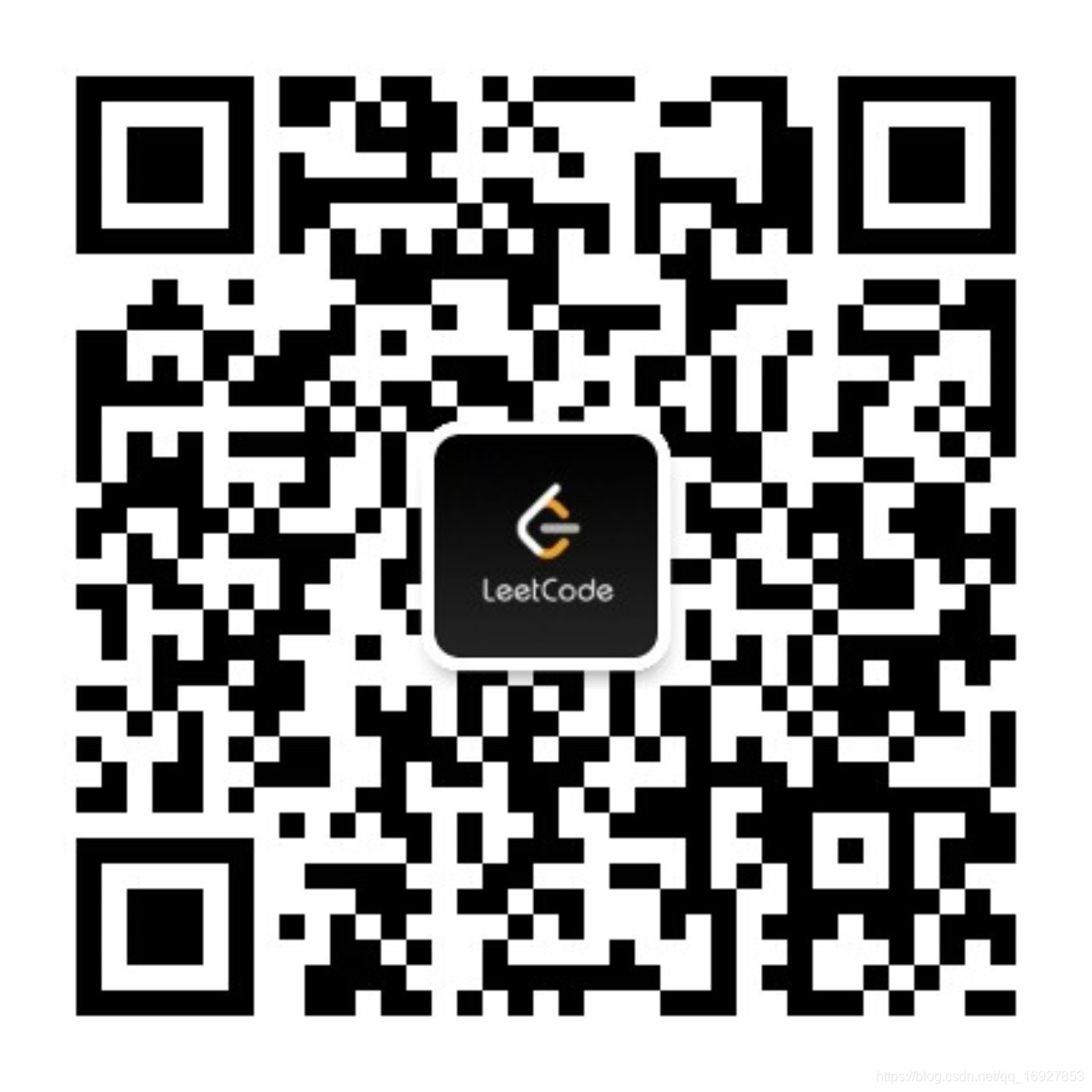