【LeetCode】766. Toeplitz Matrix (Easy) (JAVA)
Subject address: https://leetcode.com/problems/toeplitz-matrix/
Title description:
Given an m x n matrix, return true if the matrix is Toeplitz. Otherwise, return false.
A matrix is Toeplitz if every diagonal from top-left to bottom-right has the same elements.
Example 1:
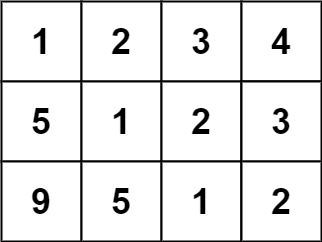
Input: matrix = [[1,2,3,4],[5,1,2,3],[9,5,1,2]]
Output: true
Explanation:
In the above grid, the diagonals are:
"[9]", "[5, 5]", "[1, 1, 1]", "[2, 2, 2]", "[3, 3]", "[4]".
In each diagonal all elements are the same, so the answer is True.
Example 2:
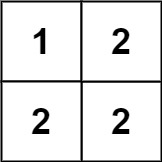
Input: matrix = [[1,2],[2,2]]
Output: false
Explanation:
The diagonal "[1, 2]" has different elements.
Constraints:
- m == matrix.length
- n == matrix[i].length
- 1 <= m, n <= 20
- 0 <= matrix[i][j] <= 99
Follow up:
- What if the matrix is stored on disk, and the memory is limited such that you can only load at most one row of the matrix into the memory at once?
- What if the matrix is so large that you can only load up a partial row into the memory at once?
General idea
Give you a matrix of mxn. If this matrix is a Toplitz matrix, return true; otherwise, return false.
If the elements on each diagonal from the upper left to the lower right of the matrix are the same, then the matrix is a Toplitz matrix.
Advanced:
- What if the matrix is stored on disk and the memory is so limited that only one row of the matrix can be loaded into the memory at a time?
- What if the matrix is so large that only an incomplete row can be loaded into memory at a time?
Problem-solving method
- Follow the Toeplitz matrix to traverse each diagonal
- Advanced 1 (What should I do if the matrix is stored on the disk and the memory is limited, so that only one row of the matrix can be loaded into the memory at a time?): The result of the next row can be calculated from the previous row
- Advanced 1 (What should I do if the matrix is too large to load an incomplete row into the memory at a time?): Divide a row, and record the content of the divided row and the boundary content in front of the previous row at the same time
class Solution {
public boolean isToeplitzMatrix(int[][] matrix) {
if (matrix.length == 0 || matrix[0].length == 0) return true;
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; (i + j) < matrix.length && j < matrix[0].length; j++) {
if (matrix[i + j][j] != matrix[i][0]) return false;
}
}
for (int i = 0; i < matrix[0].length; i++) {
for (int j = 0; (i + j) < matrix[0].length && j < matrix.length; j++) {
if (matrix[j][i + j] != matrix[0][i]) return false;
}
}
return true;
}
}
Execution time: 1 ms, beating 100.00% of Java users
Memory consumption: 38.6 MB, beating 47.69% of Java users
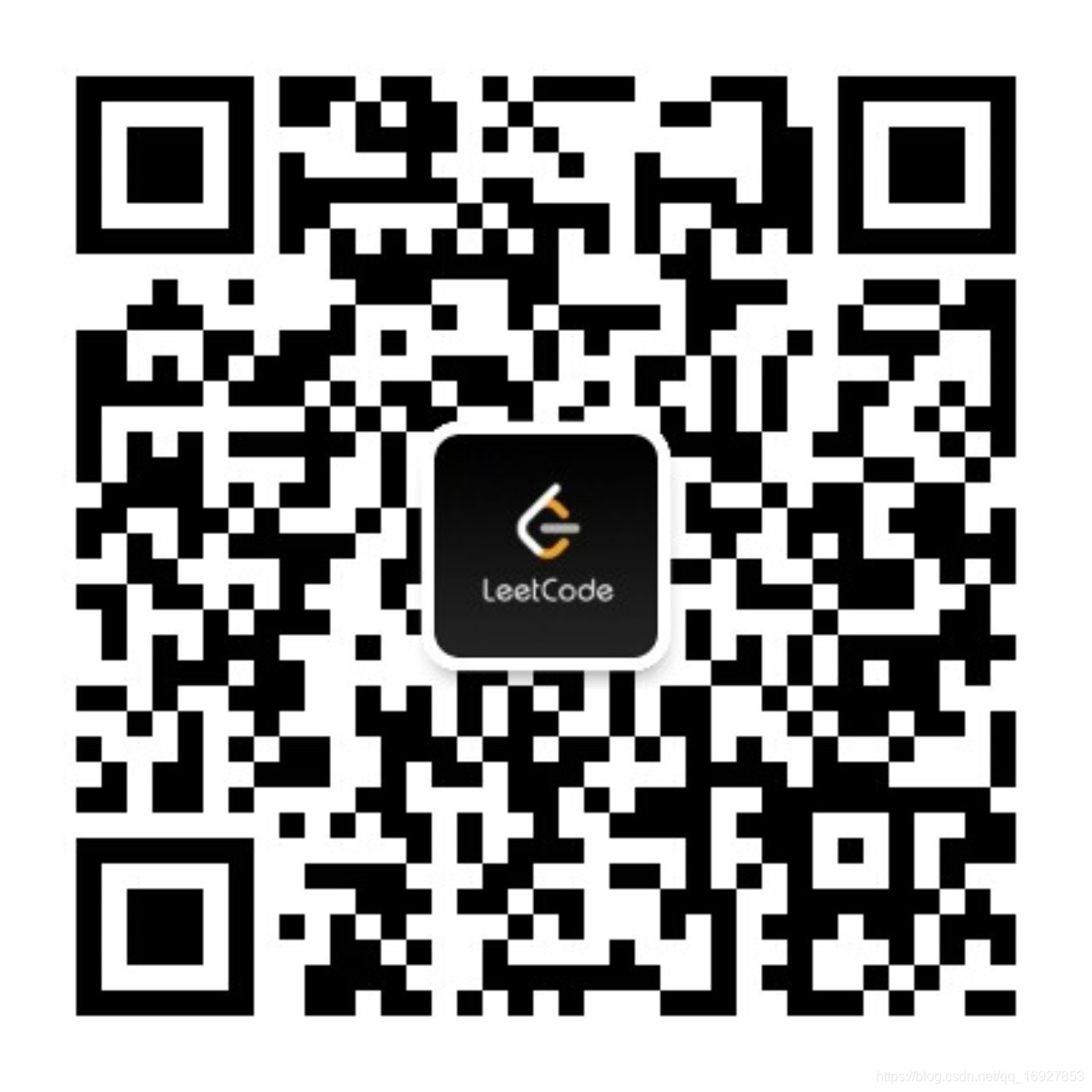