【LeetCode】389. Find the Difference (Easy) (JAVA)
Title address: https://leetcode-cn.com/problems/find-the-difference/
Title description:
You are given two strings s and t.
String t is generated by random shuffling string s and then add one more letter at a random position.
Return the letter that was added to t.
Example 1:
Input: s = "abcd", t = "abcde"
Output: "e"
Explanation: 'e' is the letter that was added.
Example 2:
Input: s = "", t = "y"
Output: "y"
Example 3:
Input: s = "a", t = "aa"
Output: "a"
Example 4:
Input: s = "ae", t = "aea"
Output: "a"
Constraints:
- 0 <= s.length <= 1000
- t.length == s.length + 1
- s and t consist of lower-case English letters.
General idea
Given two strings s and t, they only contain lowercase letters.
The string t is randomly rearranged by the string s, and then a letter is added at a random position.
Please find the letter added in t.
Problem-solving method
- This question is similar to the question where the other elements in the array appear twice, and the only element appears once.
- The characteristic of XOR can be borrowed: a b a = b, which is equivalent to the repeated elements being XORed twice and finally eliminated
- So as long as all the elements of s and t are XORed once, the result is
class Solution {
public char findTheDifference(String s, String t) {
char res = 0;
for (int i = 0; i < s.length(); i++) {
res ^= s.charAt(i);
res ^= t.charAt(i);
}
res ^= t.charAt(t.length() - 1);
return res;
}
}
Execution time: 2 ms, beating 76.78% of Java users
Memory consumption: 37 MB, beating 37.49% of Java users
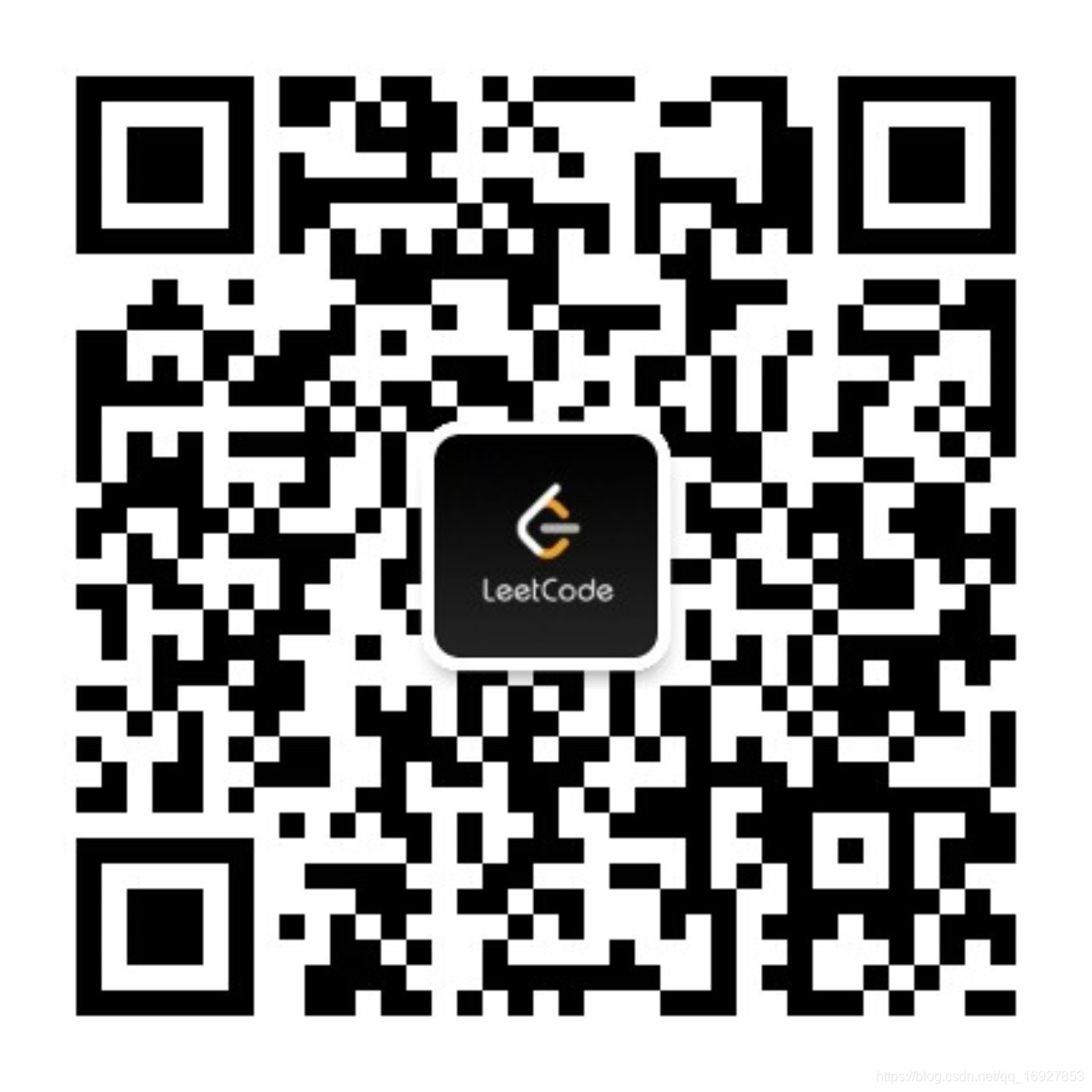