Start:
browser input:
http://127.0.0.1:15672 The
default account and password are both guest,
Add a user such as the account heima, and the password is also heima.
Create a virtual machine (general/beginning) and set it for heima to use:
the setting is successful:
1. RabbitMQ-Quick Start with Simple Mode
The following demonstrates the simple mode:
create two maven projects without skeletons:
1. Producer:
1.pom.xml :
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.itcast</groupId>
<artifactId>RabbitMQ_provider1</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.rabbitmq</groupId>
<artifactId>amqp-client</artifactId>
<version>5.6.0</version>
</dependency>
</dependencies>
</project>
2.Producer_HelloWorld:
package com.itheima.producer;
/**
* @author QLBF
* @version 1.0
* @date 2021/2/26 10:55
*/
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
/**
* 发送消息
*/
public class Producer_HelloWorld {
public static void main(String[] args) throws IOException, TimeoutException {
//1.创建连接工厂
ConnectionFactory factory=new ConnectionFactory();
//2. 设置参数
factory.setHost("127.0.0.1"); //ip,写RabbitMQ启动的ip
factory.setPort(5672);//端口 默认值 5672
factory.setVirtualHost("/itcast"); //虚拟机 默认值/
factory.setUsername("heima"); //用户名 默认 guest
factory.setPassword("heima"); //密码 默认值 guest
//3. 创建连接 Connection
Connection connection=factory.newConnection();
//4. 创建Channel
Channel channel=connection.createChannel();
//5. 创建队列Queue
/*
queueDeclare(String queue, boolean durable, boolean exclusive, boolean autoDelete, Map<String, Object> arguments)
参数:
1. queue:队列名称
2. durable:是否持久化,当mq重启之后,还在
3. exclusive:
* 是否独占。只能有一个消费者监听这队列
* 当Connection关闭时,是否删除队列
*
4. autoDelete:是否自动删除。当没有Consumer时,自动删除掉
5. arguments:参数。
*/
//如果没有一个名字叫hello_world的队列,则会创建该队列,如果有则不会创建
channel.queueDeclare("hello_world",true,false,false,null);
/*
basicPublish(String exchange, String routingKey, BasicProperties props, byte[] body)
参数:
1. exchange:交换机名称。简单模式下交换机会使用默认的 ""(简单模式相当于没交换机)
2. routingKey:路由名称,与上面的队列对应
3. props:配置信息
4. body:发送消息数据
*/
String boby="hello rabbitMQ1...";
//6. 发送消息
channel.basicPublish("","hello_world",null,boby.getBytes());
//7.释放资源
channel.close();
connection.close();
}
}
Then run, tomcat, and then run the above class, you can see it in the browser:
2. Consumers: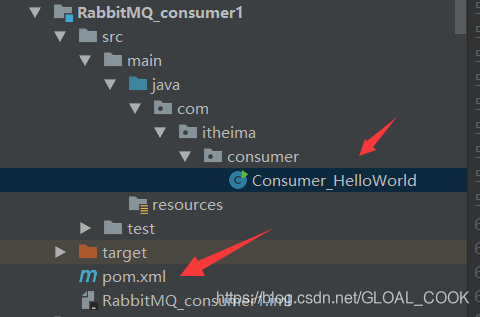
(1) pom.xml:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.itcast</groupId>
<artifactId>RabbitMQ_consumer1</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.rabbitmq</groupId>
<artifactId>amqp-client</artifactId>
<version>5.6.0</version>
</dependency>
</dependencies>
</project>
(2)com.itheima.consumer.Consumer_HelloWorld:
package com.itheima.consumer;
import com.rabbitmq.client.*;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
/**
* @author QLBF
* @version 1.0
* @date 2021/2/26 11:39
*/
public class Consumer_HelloWorld {
public static void main(String[] args) throws IOException, TimeoutException {
//1.创建连接工厂
ConnectionFactory factory=new ConnectionFactory();
//2. 设置参数
factory.setHost("127.0.0.1"); //ip,写RabbitMQ启动的ip
factory.setPort(5672);//端口 默认值 5672
factory.setVirtualHost("/itcast"); //虚拟机 默认值/,这个是我在启动Rabbit网站设置的
factory.setUsername("heima"); //用户名 默认 guest
factory.setPassword("heima"); //密码 默认值 guest
//3. 创建连接 Connection
Connection connection=factory.newConnection();
//4. 创建Channel
Channel channel=connection.createChannel();
//5. 创建队列Queue
/*
queueDeclare(String queue, boolean durable, boolean exclusive, boolean autoDelete, Map<String, Object> arguments)
参数:
1. queue:队列名称
2. durable:是否持久化,当mq重启之后,还在
3. exclusive:
* 是否独占。只能有一个消费者监听这队列
* 当Connection关闭时,是否删除队列
*
4. autoDelete:是否自动删除。当没有Consumer时,自动删除掉
5. arguments:参数。
*/
//如果没有一个名字叫hello_world的队列,则会创建该队列,如果有则不会创建
channel.queueDeclare("hello_world",true,false,false,null);
/*
basicConsume(String queue, boolean autoAck, Consumer callback)
参数:
1. queue:队列名称,从生产者的hello_world拿出来
2. autoAck:是否自动确认
3. callback:回调对象
*/
// 6.接收消息
Consumer consumer=new DefaultConsumer(channel){
/*
回调方法,当收到消息后,会自动执行该方法
1. consumerTag:标识
2. envelope:获取一些信息,交换机,路由key...
3. properties:配置信息
4. body:数据
*/
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("consumerTag:"+consumerTag);
System.out.println("Exchange:"+envelope.getExchange());
System.out.println("RoutingKey:"+envelope.getRoutingKey());
System.out.println("properties:"+properties);
System.out.println("body:"+new String(body)); //获取生产者发给MQ的消息,转为字符串
}
};
channel.basicConsume("hello_world",true,consumer);
//7关闭资源?不要
}
}
Then run it directly, and it can get the message sent by the producer from the queue:
remember to run the producer's tomcat first, then run its sending information class, and then run the consumer's receiving information class.