From scratch, build a simple shopping platform (9):
https://blog.csdn.net/time_____/article/details/105465499
Project source code (continuous update): https://gitee.com/DieHunter/myCode/ Tree/master/shopping has been
delayed for a long time. Please forgive me for the peak period of the company’s projects in the past few months.
This article mainly describes part of the new order module of the back-end management system (the front-end is slightly modified under the previous plan, introducing the order function, but does not include the payment function), because it is slightly different from user management and commodity management , So take it out and introduce it separately
Front end effect:
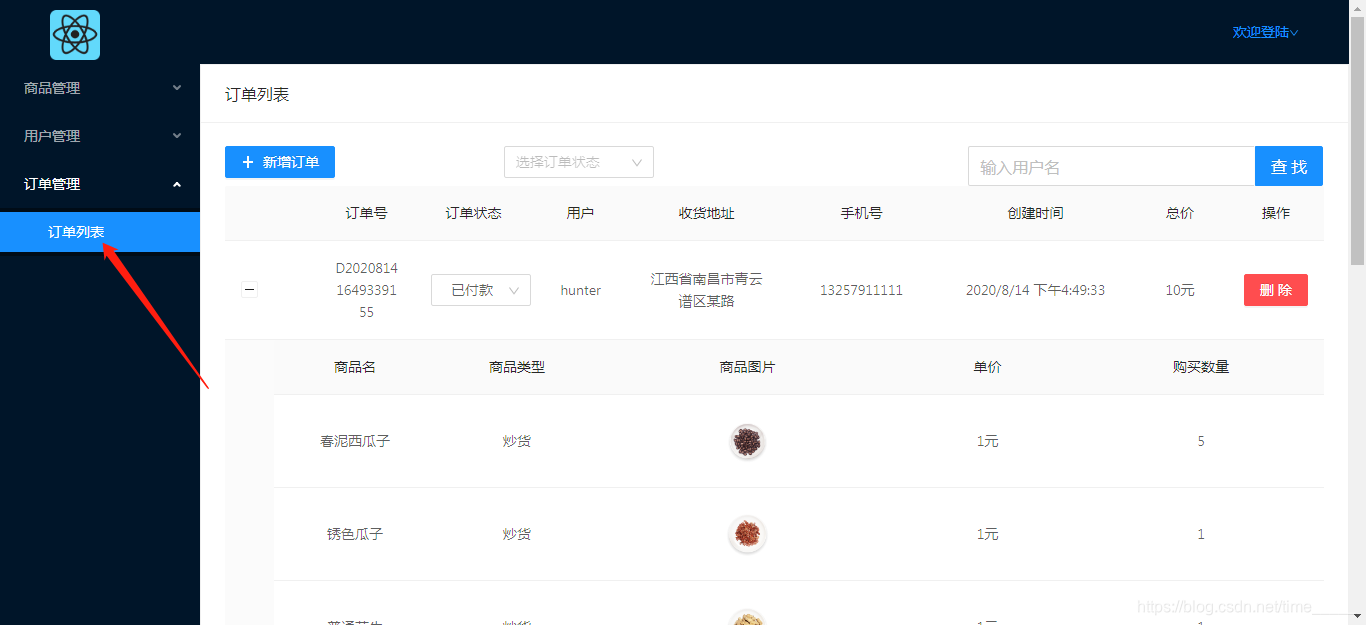
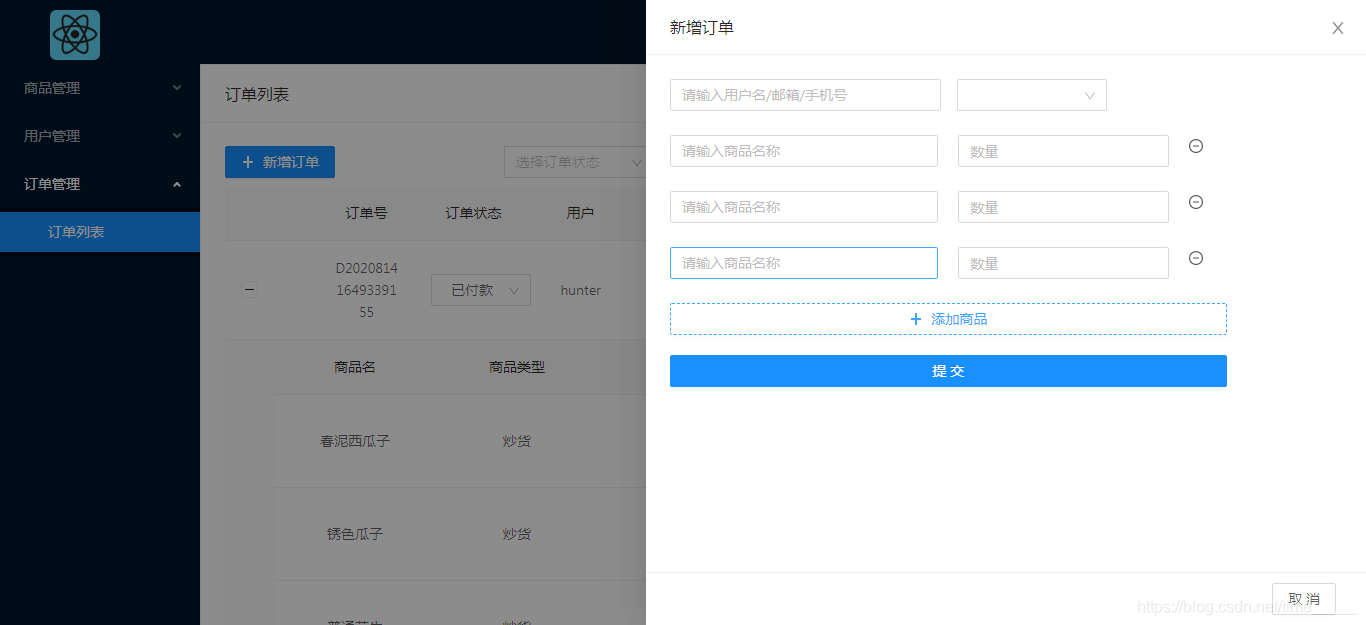
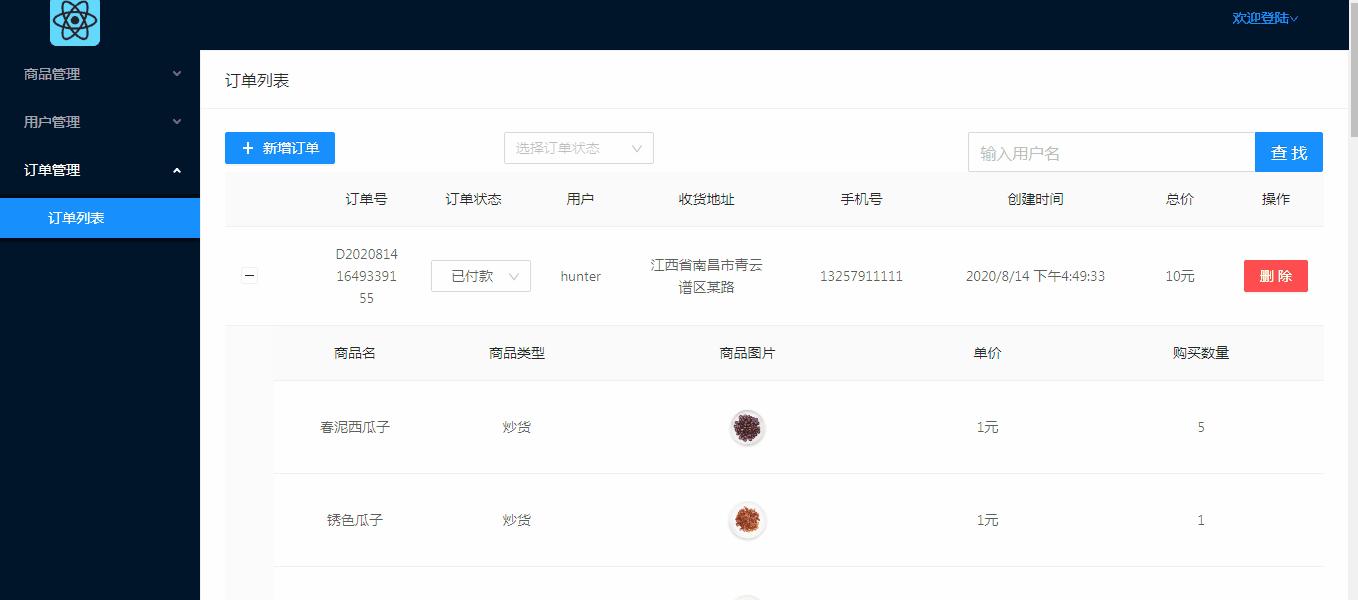
For the order module, the main functions include order list display, order status modification (not involving other data modification other than order status), delete orders, and add new orders (for new orders, only add them through the product name index, considering that The order addition of the management system is not the main function and is almost useless, so there is no detailed function). This article mainly introduces the implementation of back-end order management and interface docking.
Server:
- First, we need to configure the database table structure on the server, which mainly contains the following fields
Order: { modelName: "orders", data: { orderId: { // 订单号 type: String, required: true, }, username: { //用户名 type: String, required: true, }, phoneNum: { //手机号 type: String, required: true, }, address: { //具体地址 type: String, required: true, }, orderState: { //订单状态 type: String, required: true, }, orderPrice: { // 订单总价 type: String, required: true, }, shopList: { //商品列表 type: Array, required: true, }, orderTime: { //订单创建时间 type: String, required: true, }, }, },
- Use npm start to start the server
- Then create an order folder in the controller directory under the src directory for order interface and logic implementation. The mod file is the same as before, and the new order table is created
- Introduce the dependency package in the order. It should be noted that adding a new order not only requires an order table, but also needs to be linked with the user and shop tables. Therefore, we need to call methods in other interface logic. The imported package names are as follows
const router = require("express").Router();//路由 const UserMod = require("../user/mod");//user表联动 const ShopMod = require("../shopList/mod");//shop表联动 const Mod = require("./mod");//order表 const Util = require("../../../utils/utils");//工具类 const Config = require("../../../config/config");//配置文件 const Bussiness = require("../../bussiness/bussiness");//接口逻辑 const { addData, updateData, findData } = require("../../command/command");//数据库操作
-
The first is the new order interface. This interface is also the place that needs attention and the most pits. The following is to detect the existence of users, user addresses, and products
let userFindRes = await Bussiness.hasUser(req, res, UserMod);//检测用户及地址是否存在 if (!userFindRes) { return; } if (!userFindRes[0].alladdress || !userFindRes[0].address) { res.send({ result: -1, msg: "添加失败,请完善收货地址", }); return; } let shopFindRes = await findData(ShopMod, {//检测商品是否存在 shopName: { $in: res._data.shopList.map((item) => { return item.shopName; }), }, }); if ( !shopFindRes || !shopFindRes.length || shopFindRes.length != res._data.shopList.length ) { res.send({ result: -2, msg: "有商品不存在", }); return; }
In the next step, the database information will be retrieved (note: the database information is read-only and can only be modified with database commands, so conventional heap memory access cannot be used and a simple heap variable copy is required)
static deepCopy(org) { //简单的对象复制 return JSON.parse(JSON.stringify(org)); }
Use the copy function to take out the database information and make subsequent modifications
let _shopFindRes = Util.deepCopy(shopFindRes); //解决数据库对象只读属性
Before returning to the front end, calculate the total price and order adding time
let _orderPrice;//初始化商品总价 let _shopFindRes = Util.deepCopy(shopFindRes); //解决数据库对象只读属性 _shopFindRes.forEach((item, index) => {//合计总费用 if (index == 0) { _orderPrice = 0; } _shopFindRes[index].shopCount = res._data.shopList[index].shopCount; _orderPrice += _shopFindRes[index].shopCount * _shopFindRes[index].shopPrice; }); res._data = { ...res._data, username: userFindRes[0].username, phoneNum: userFindRes[0].phoneNum, address: userFindRes[0].alladdress.join("") + userFindRes[0].address, orderId: Util.createOrderNo(), orderTime: Util.joinDate(), shopList: _shopFindRes, orderPrice: _orderPrice, }; let addRes = await addData(Mod, res._data); if (!addRes || !addRes.length) { res.send({ result: 0, msg: "添加失败", }); return; } res.send({ result: 1, msg: "添加成功", orderId: res._data.orderId, });
-
Compared with order query, deletion, and modification, adding an order is a logically different function. There are fewer places where the code can be reused. The other three functions can be implemented by imitating the product and user interface writing.
-
Get order list
router.get(Config.ServerApi.orderList, Util.checkToken, async (req, res) => { Bussiness.findInfo( req, res, Mod, { orderTime: res._data.sort,//时间排序 }, { orderId: new RegExp(res._data.orderId, "i"),//orderId(订单号)模糊过滤 username: new RegExp(res._data.keyWord, "i"),//订单用户名模糊过滤 orderState: new RegExp(res._data.orderState, "i"),//订单状态模糊过滤 } ); });
-
Delete order
router.get(Config.ServerApi.delOrder, Util.checkToken, async (req, res) => { if (!Bussiness.isAdmin(res)) { return; } Bussiness.delInfo(req, res, Mod); });
-
Modify order status
router.post(Config.ServerApi.updateOrder, Util.checkToken, async (req, res) => { let updateRes = await updateData(Mod, res._data._id, res._data); if (updateRes) { res.send({ result: 1, msg: "修改成功", }); return; } res.send({ result: 0, msg: "修改失败", }); });
The above is the order management server interface and logic part
to sum up:
When writing object-oriented code and implementing its functions, minimize the coupling between functions (the connection between functions and functions) to enhance reusability and greatly save development efficiency