# clock_in
Introduction
Jilin University automatic health check-in v2.0
Software Architecture
- selenium-chromedriver [call chrmoe]
- win32gui [Cool Q is blocked, so use this program instead]
Development ideas
- Use selenium to complete the test, get the "completed project" data in the web page, open the corresponding QQ chat window through win32gui, and create a plan on the server is the best
Installation tutorial
- Store the chrmoe driver in the Application folder under the chrome root directory [C:\Program Files (x86)\Google\Chrome\Application]
- Added system variable [path C:\Program Files (x86)\Google\Chrome\Application]
- Install pip, selenium, win32api, win32gui
Instructions for use
- 1. Modify personal information
- username = "zhuyu18" # Account
- pwd = "#######" # Password
- recv_qq = "zy" # QQ receiving feedback
- 2. The corresponding QQ chat window must be kept open
- 3. Computer, create a plan, turn on and run the script at a fixed time every day [The best deployment on the server]
************** detailed steps **************
1. Install chromedriver
1.1 Open the chrome browser, type chrome://version, query the version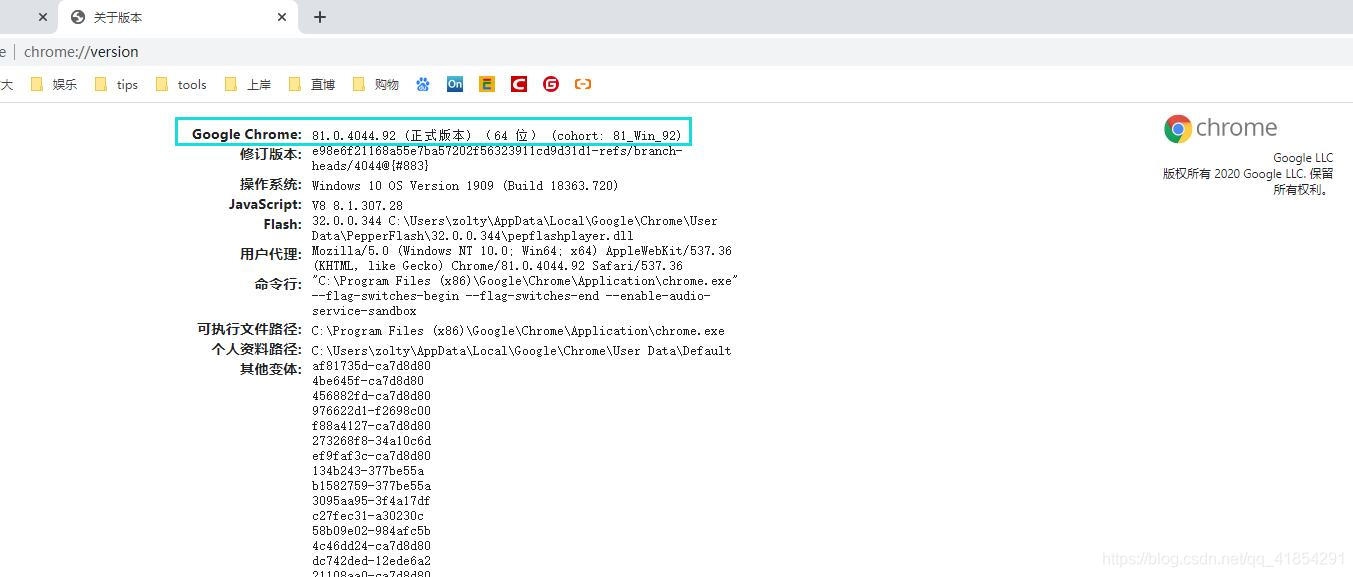
1.2 Download the corresponding version of the driver
Address: https://npm.taobao.org/mirrors/chromedriver/
1.3 Place the driver in the Application directory under the chrome root directory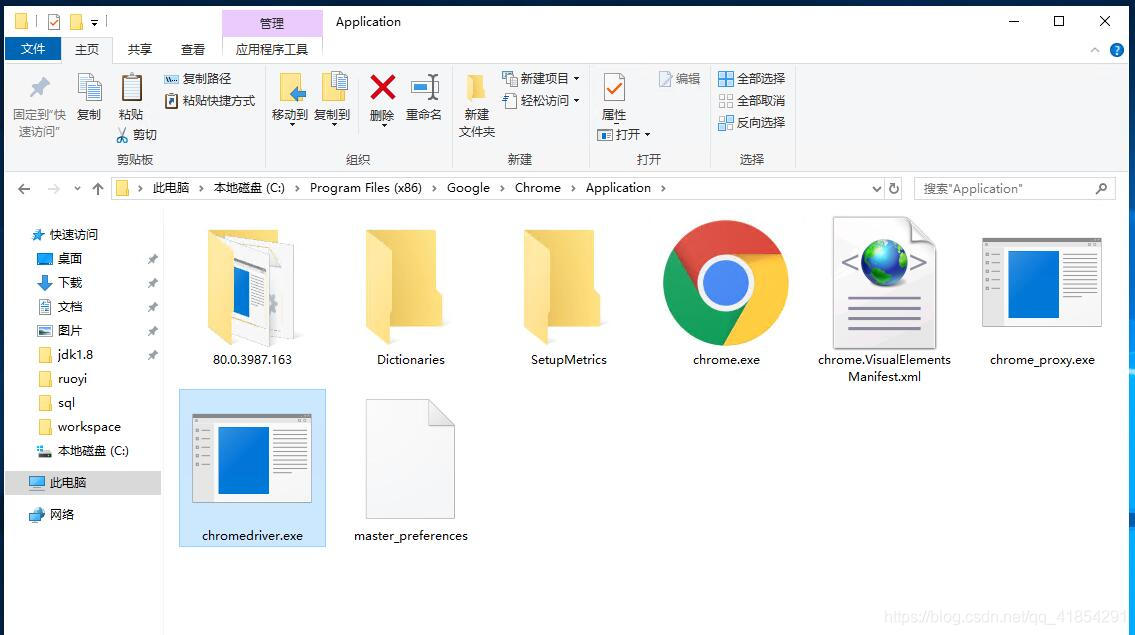
1.4 Add system variables
2. Create a plan
2.1 win+R type compmgmt.msc to open the computer management
2.2 Create clock_in folder
2.3 Create a filepath that plans to fill in clock_in
3. Modify the py file
3.1 Import dependencies, install pip, pip install selenium...
from selenium import webdriver
import win32gui
import win32con
import win32clipboard as w
import time
3.2 Modify personal information, username, pwd, recv_qq
3.3 xpath acquisition, web page F12, use div id name select and other tags to obtain
4. Results display
5.demo
# -*- coding:utf-8 -*-
from selenium import webdriver
import win32gui
import win32con
import win32clipboard as w
import time
# ========================= 信息-需修改 =========================
username = "zhuyu18" # 账号
pwd = "########" # 密码
recv_qq = "zy" # 接收反馈的QQ
loading_time = 10 # 预留页面加载时间10秒 , 视电脑配置修改
# ========================= url-无需修改 =========================
login_url = "https://ehall.jlu.edu.cn/jlu_portal/login" # 登录url
remind_url = "https://ehall.jlu.edu.cn/taskcenter/workflow/done" # 登录url
# ========================= xpath-无需修改 =========================
uid_xpath = './/input[@name="username"]' # 账号xpath
pwd_xpath = './/input[@name="password"]' # 密码xpath
button_xpath = './/input[@name="login_submit"]' # 登录按钮xpath
stu_xpath = './/a[@href="/jlu_portal/guide?id=83E36211-C52A-4533-913F-F4D42C677D2A"]' # 研究生健康打卡按钮xpath
handle_xpath = './/input[@value="我要办理"]' # 我要办理按钮xpath
loc_con_xpath = './/div[@id="V1_CTRL119_Container"]' # 所在市容器按钮xpath
loc_xpath = './/div[@class="infoplus_suggester_item suggest_selected"]' # 所在市下拉框xpath
checkbox_xpath = './/input[@name="fieldCNS"]' # 承诺按钮xpath
confirm_xpath = './/a[@class="command_button_content"]' # 确认按钮xpath
ok_xpath = './/button[@class="dialog_button default fr"]' # 好按钮xpath
# ========================= 驱动-无需修改 =========================
global driver
driver = webdriver.Chrome() # 设置Chrome驱动
def web_init():
driver.maximize_window() # 最大化窗口
driver.implicitly_wait(6) # 隐式等待
driver.get(login_url) # 获取网页
def web_close():
driver.quit() # 关闭所有关联的窗口
def web_load():
time.sleep(loading_time) # 预留页面加载时间5秒
def auto_login(username_, pwd_, uid_xpath_, pwd_xpath_, button_xpath_):
web_load()
driver.find_element_by_xpath(uid_xpath_).clear() # 清空已存在内容
driver.find_element_by_xpath(uid_xpath_).send_keys(username_) # 传入用户名
web_load()
driver.find_element_by_xpath(pwd_xpath_).clear() # 清空已存在内容
driver.find_element_by_xpath(pwd_xpath_).send_keys(pwd_) # 传入密码
web_load()
driver.find_element_by_xpath(button_xpath_).click() # 通过登录按钮并单击登录
def submit(stu_xpath_, handle_xpath_, loc_con_xpath_, loc_xpath_, checkbox_xpath_, confirm_xpath_, ok_xpath_):
driver.find_element_by_xpath(stu_xpath_).click() # 研究生健康状况申报
web_load()
driver.find_element_by_xpath(handle_xpath_).click() # 我要办理
web_load()
driver.switch_to.window(driver.window_handles[1]) # 切换窗口句柄至最新窗口
web_load()
# ========================= 该部分代码针对需要手动选择市区的用户,可以选择注释 =========================
driver.find_element_by_xpath(loc_con_xpath_).click() # 定位到复选框容器V1_CTRL119_Container
web_load()
driver.find_element_by_xpath(loc_xpath_).click()
web_load()
# ========================= 该部分代码针对需要手动选择市区的用户,可以选择注释 =========================
driver.find_element_by_xpath(checkbox_xpath_).click() # 本人承诺以上填写内容均真实可靠
web_load()
driver.find_element_by_xpath(confirm_xpath_).click() # 确认填报
web_load()
driver.find_element_by_xpath(ok_xpath_).click() # 好
web_load()
def remind(remind_url_, recv_qq):
driver.get(remind_url_) # 获取网页
web_load()
row = driver.find_elements_by_tag_name('tr')
result_list = []
for i in row:
j = i.find_elements_by_tag_name('td')
for item in j:
text = item.text
result_list.append(text)
today = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime(time.time()))
result_msg = today+'-'+result_list[0]+'-'+result_list[1]+'-'+result_list[2]+'-'+result_list[3]
print(result_msg)
send(recv_qq, result_msg) # 发送最近的一条打卡记录
def send(name, msg):
# 打开剪贴板
w.OpenClipboard()
# 清空剪贴板
w.EmptyClipboard()
# 设置剪贴板内容
w.SetClipboardData(win32con.CF_UNICODETEXT, msg)
# 获取剪贴板内容
date = w.GetClipboardData()
# 关闭剪贴板
w.CloseClipboard()
# 获取qq窗口句柄
handle = win32gui.FindWindow(None, name)
if handle == 0:
print('未找到窗口!')
# 显示窗口
win32gui.ShowWindow(handle, win32con.SW_SHOW)
# 把剪切板内容粘贴到qq窗口
win32gui.SendMessage(handle, win32con.WM_PASTE, 0, 0)
# 按下后松开回车键,发送消息
win32gui.SendMessage(handle, win32con.WM_KEYDOWN, win32con.VK_RETURN, 0)
win32gui.SendMessage(handle, win32con.WM_KEYUP, win32con.VK_RETURN, 0)
time.sleep(2) # 延缓进程
if __name__ == '__main__':
web_init() # 驱动初始化
auto_login(username, pwd, uid_xpath, pwd_xpath, button_xpath) # 登录操作
submit(stu_xpath, handle_xpath, loc_con_xpath, loc_xpath, checkbox_xpath, confirm_xpath, ok_xpath) # 提交操作
remind(remind_url, recv_qq) # 发送提醒信息
web_close() # 测试结束