Article Directory
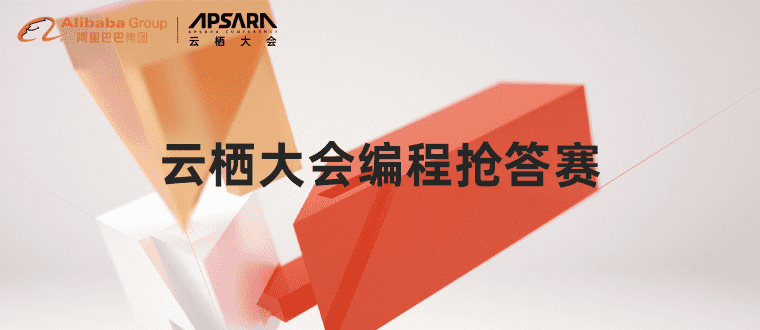
1. Yunqi Conference Limited-Time Rush Answer Match-Morning Field
The title of this session has the original title on LeetCode, and the link to the solution is as follows:
LeetCode 862. The shortest subarray whose sum is at least K (prefix and +deque monotonic stack)
2. Yunqi Conference Limited Time Rush Answer Match-Noon Field
Topic: Sliding Puzzle
description:
In a 3x3 grid, there are 8 boards numbered 1 to 8, and a space numbered 0.
One move can exchange space 0 with one of the four adjacent boards.
Given the initial and target board layout, return to the target layout with the least number of moves .
If you cannot move from the initial arrangement to the target arrangement, return -1.
样例1 :
输入:
[
[2,8,3],
[1,0,4],
[7,6,5]
]
[
[1,2,3],
[8,0,4],
[7,6,5]
]
输出:
4
解释:
[ [
[2,8,3], [2,0,3],
[1,0,4], --> [1,8,4],
[7,6,5] [7,6,5]
] ]
[ [
[2,0,3], [0,2,3],
[1,8,4], --> [1,8,4],
[7,6,5] [7,6,5]
] ]
[ [
[0,2,3], [1,2,3],
[1,8,4], --> [0,8,4],
[7,6,5] [7,6,5]
] ]
[ [
[1,2,3], [1,2,3],
[0,8,4], --> [8,0,4],
[7,6,5] [7,6,5]
] ]
样例 2:
输入:
[[2,3,8],[7,0,5],[1,6,4]]
[[1,2,3],[8,0,4],[7,6,5]]
输出:
-1
With LeetCode 773. Slider Puzzle (BFS state transition map the shortest distance) is almost identical
Just change the code above slightly
- Breadth-first search, compress the 3x3 map into a string, record the status, and do not repeat visits
class Solution {
vector<vector<int>> dir = {
{
1,0},{
0,1},{
0,-1},{
-1,0}};
int m, n, i, j, k, step = 0, size, x, y;
public:
/**
* @param init_state: the initial state of chessboard
* @param final_state: the final state of chessboard
* @return: return an integer, denote the number of minimum moving
*/
int minMoveStep(vector<vector<int>> &board, vector<vector<int>> &final_state) {
m = board.size(), n = board[0].size();
string ans, state;
for(i = 0; i < 3; ++i)
for(j = 0; j < 3; ++j)
ans += '0'+final_state[i][j];
int x0, y0, xi, yi;
pair<int,int> xy0;//0的坐标
queue<string> q;
unordered_set<string> visited;//访问标记集合
state = boardToString(board);//初始状态
if(state == ans)
return step;
q.push(state);
visited.insert(state);
while(!q.empty())
{
step++;
size = q.size();
while(size--)
{
xy0 = stringToBoard(q.front(), board);//还原地图,并得到0的坐标
q.pop();
x0 = xy0.first;
y0 = xy0.second;
for(k = 0; k < 4; ++k)
{
//0可以4个方向交换
xi = x0+dir[k][0];
yi = y0+dir[k][1];
if(xi>=0 && xi<m && yi>=0 && yi<n)
{
swap(board[xi][yi], board[x0][y0]);//交换
state = boardToString(board);//新的状态
if(state == ans)
return step;
if(!visited.count(state))//没有出现过该地图
{
visited.insert(state);
q.push(state);
}
swap(board[xi][yi], board[x0][y0]);//还原现场
}
}
}
}
return -1;
}
string boardToString(vector<vector<int>>& board)
{
//地图转成字符串
string s;
for (i = 0; i < m; i++)
for(j = 0; j < n; j++)
s.push_back(board[i][j]+'0');
return s;
}
pair<int,int> stringToBoard(string &s, vector<vector<int>>& board)
{
//字符串还原成地图,并return 0的坐标,方便下次挪动
for (i = m-1; i >= 0; i--)
for(j = n-1; j >= 0; j--)
{
board[i][j] = s.back()-'0';
s.pop_back();
if(board[i][j] == 0)
x = i, y = j;
}
return make_pair(x, y);
}
};
1106ms C++
3. Yunqi Conference Limited-time Rush Answer-Evening Field
Topic: Map Jump
description:
For a given n×n
map, each cell has a height, and each time you can only move to the adjacent cell, and require these two cells 高度差不超过target
.
You can't get out of the map.
Find the smallest target that satisfies from the upper left corner (0,0)
to the lower right corner(n-1,n-1)
n < = 1000 < = a r r [ i ] [ j ] < = 100000 n <= 1000 <= arr[i][j] <= 100000 n<=1000<=arr[i][j]<=100000
例 1:
输入:[[1,5],[6,2]],
输出:4,
解释:
有2条路线:
1. 1 -> 5 -> 2 这条路线上target为4。
2. 1 -> 6 -> 2 这条路线上target为5。
所以结果为4
例 2:
输入:[[1,5,9],[3,4,7],[6,8,1]],
输出:6
Problem solving:
- Binary search
- DFS judges whether there is a path on which the absolute value of the difference between each adjacent point is <= target
class Solution {
vector<vector<int>> dir = {
{
1,0},{
0,1},{
-1,0},{
0,-1}};
int n;
public:
/**
* @param arr: the map
* @return: the smallest target that satisfies from the upper left corner (0, 0) to the lower right corner (n-1, n-1)
*/
int mapJump(vector<vector<int>> &arr) {
// Write your code here.
if(arr.empty()) return 0;
n = arr.size();
int l = 0, r = 100000, mid, ans;
bool way = false;
while(l <= r)
{
mid = (l + r)/2;
way = false;
vector<vector<bool>> vis(n, vector<bool>(n, false));
vis[0][0] = true;
ok(arr, 0, 0, vis, mid, way);//DFS
if(way)//有满足要求的路径
{
ans = mid;
r = mid-1;
}
else
l = mid+1;
}
return ans;
}
void ok(vector<vector<int>> &arr, int x, int y, vector<vector<bool>>& vis, int target, bool& way)
{
if(way) return;
if(x==n-1 && y==n-1)
{
way = true;
return;
}
int i, j, k;
for(k = 0; k < 4; ++k)
{
i = x + dir[k][0];
j = y + dir[k][1];
if(i >= 0 && i < n && j >= 0 && j < n && !vis[i][j] && abs(arr[i][j]-arr[x][y])<=target)
{
vis[i][j] = true;
ok(arr,i,j,vis,target,way);
}
}
}
};
101ms C++
Similar topics: LeetCode 1102. The path with the highest score (Priority Queue BFS/Minimax Binary Search)
My CSDN blog address https://michael.blog.csdn.net/
Long press or scan the QR code to follow my official account (Michael Amin), come on together, learn and make progress together!