1.载入文档
1 #!/usr/bin/python 2 # -*- coding: utf-8 -*- 3 4 import pandas as pd 5 import re 6 import jieba 7 from sklearn.feature_extraction.text import CountVectorizer, TfidfTransformer 8 9 10 #加载文本 11 dataPath1='D:/machinelearning data/crawlerData/mi6x_JD500.csv' 12 dataPath2='D:/machinelearning data/crawlerData/huaWei_P20_JD100.csv' 13 dataPath3='D:/machinelearning data/crawlerData/test1.txt' 14 stopWord_Path='D:/论文文件/学习文件/情感分析/dict词典/哈工大stopword .txt'#停用词路径 15 f1=pd.read_csv(dataPath1,sep=',',encoding='GBK')#.iloc[:,1]#加载文本1 16 f2=pd.read_csv(dataPath2,sep=',',encoding='GBK')#.iloc[:,1]#加载文本2 17 f3=pd.read_csv(dataPath3,sep=',',encoding='GBK',header=0)#.iloc[:,1]#加载文本3
2.加载停用词
把停用词典的停用词存到列表里,下面去停用词要用到
1 #加载停用词,停用词要是列表形式才能使用 2 stopWord=[] 3 with open(stopWord_Path,'r',encoding='utf-8') as fr:#加载停用词 4 for word in fr.readlines(): 5 stopWord.append(word.strip()) 6 #print(stopWord)
3.文本分词
这里有两个切分词的函数,第一个是手动去停用词,第二个是下面在CountVectorizer()添加stop_words参数去停用词。两种方法都可用。
1 #文本切分函数,用来对中文文本分词,包括除去数字字母以及停用词,得到一个分词用空格隔开的字符串,便于下面向量化(因为这个CountVouterizer()是针对英文分词的,英文之间直接用空格隔开的) 2 def cut_word(sent): 3 line=re.sub(r'[a-zA-Z0-9]*','',sent) 4 wordList=jieba.lcut(line,cut_all=False) 5 return ' '.join([word for word in wordList if word not in stopWord and len(word)>1])#文本分词,并且用空格连接起来,便于下面向量化 6 7 #也是文本切分函数,只不过这个没有去停用词,CountVouterizer()中可以直接添加停用词表参数,不统计文档中的停用词的数量 8 def cutword(sent): 9 line=re.sub(r'[a-zA-Z0-9]*','',sent) 10 wordList=jieba.lcut(line,cut_all=False) 11 return ' '.join([word for word in wordList if len(word)>1])
4.对文本进行分词,向量化
(1)对文本进行分词,并且将分词结果加到'word_list’列中。
f3['word_list']=f3.comment.apply(cutword)#将文本分词,并且分词用空格隔开变成文本存才DataFrame中 print(f3)
结果如下
comment word_list 0 你好吗,你的我的他的都不行,非常好,中国加油! 你好 不行 非常 中国 加油 1 真的非常好,你知道吗,手机性能不行 真的 非常 知道 手机 性能 不行 2 昨天晚上下单,今早拿到机器。这物流很给力!电池不耐用,不优秀。严重发烫,希望小米后续优化跟进。 昨天晚上 下单 今早 拿到 机器 物流 电池 耐用 优秀 严重 发烫 希望 小米 后续 优化 跟进
(2)对文本向量化,sklearn中的CounterVectorizer()向量化为系数矩阵,文本必须是空格隔开的字符串,因为CounterVectorizer()是针对英文分词的,英文之间直接用空格隔开的。
get_feature_names()获得上面稀疏矩阵的列索引,即特征的名字(就是分词)。这样就能知道稀疏矩阵中的每一列表示的是哪个词了
1 wordList=list(f3.word_list)#必须变成列表个是才能输入下面的向量化函数 2 count_vect = CountVectorizer(min_df=1,stop_words=stopWord,analyzer ='word') # 并且设置了停用词表为列表stopWord,即在向量化时去掉停用词不统计,词至少在1个文档中出现过 3 words_vec = count_vect.fit_transform(wordList) 4 print(words_vec.toarray())#得到分词的系数矩阵 5 #print(words_vec.todense()) 6 vec1=pd.DataFrame(words_vec.toarray()) 7 print(count_vect.get_feature_names())#获得上面稀疏矩阵的列索引,即特征的名字(就是分词)
向量化的结果
[[0 1 1 0 0 0 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0] [0 1 0 0 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 1 0 0] [1 0 0 1 1 1 0 0 1 1 1 1 0 0 1 1 1 1 1 0 1 1]]
(3)count_vect.get_feature_names()得到的分词索引列表
扫描二维码关注公众号,回复:
2623603 查看本文章
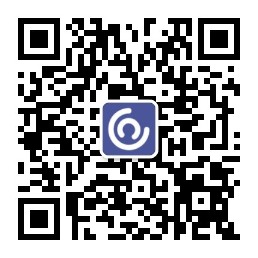
['下单', '不行', '中国', '今早', '优化', '优秀', '你好', '加油', '发烫', '后续', '小米', '希望', '性能', '手机', '拿到', '昨天晚上', '机器', '物流', '电池', '真的', '耐用', '跟进']
对应上面的稀疏矩阵就是,第一列为‘下单’,在文档1,2,3中分别出现0,0,1次。其他的同理