最終効果
点击链接也可以进入对应的sheet中
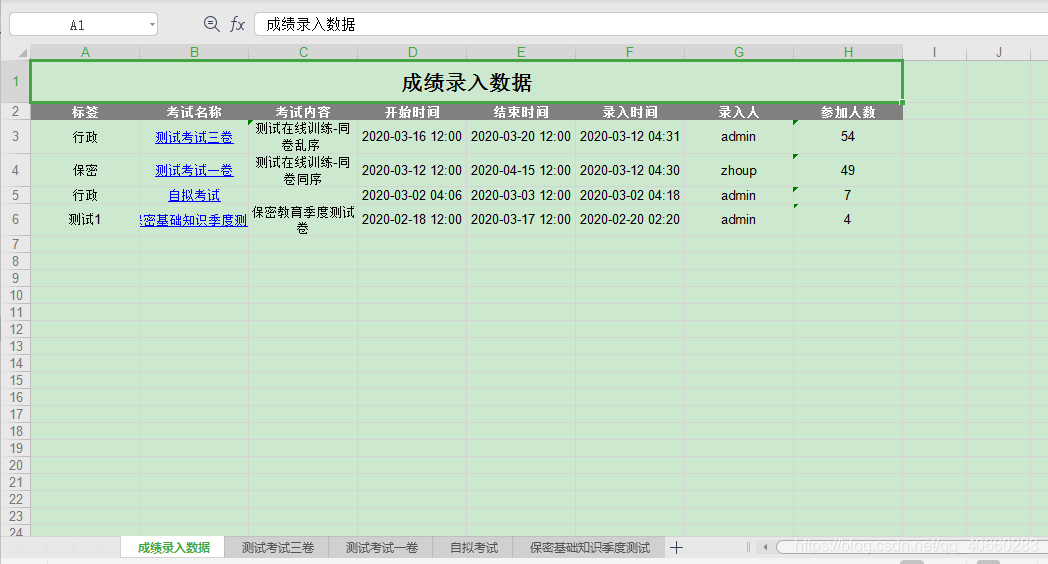
1. POIエクスポートツールクラス
package org.springblade.common.utils.excel.util;
import java.io.Serializable;
import java.util.List;
public class ExcelExp implements Serializable {
private String fileName;
private String[] handers;
private String titleName;
private List<String[]> dataset;
public ExcelExp(String titleName,String fileName, String[] handers, List<String[]> dataset) {
this.fileName = fileName;
this.titleName = titleName;
this.handers = handers;
this.dataset = dataset;
}
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String[] getHanders() {
return handers;
}
public void setHanders(String[] handers) {
this.handers = handers;
}
public List<String[]> getDataset() {
return dataset;
}
public void setDataset(List<String[]> dataset) {
this.dataset = dataset;
}
public String getTitleName() {
return titleName;
}
public void setTitleName(String titleName) {
this.titleName = titleName;
}
}
package org.springblade.common.utils.excel.util;
import org.apache.poi.common.usermodel.HyperlinkType;
import org.apache.poi.hssf.usermodel.*;
import org.apache.poi.hssf.util.HSSFColor;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.CellRangeAddress;
import org.springblade.core.tool.utils.DateUtil;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.util.List;
public class ExcelExportUtil {
public static void exportManySheetExcel(List<ExcelExp> mysheets,HttpServletResponse response){
HSSFWorkbook wb = new HSSFWorkbook();
CreationHelper createHelper = wb.getCreationHelper();
List<ExcelExp> sheets = mysheets;
HSSFCell cell3 = null;
HSSFCellStyle style = wb.createCellStyle();
style.setAlignment(HorizontalAlignment.CENTER);
style.setVerticalAlignment(VerticalAlignment.CENTER);
HSSFFont font1 = wb.createFont();
font1.setFontName("Arial");
font1.setFontHeightInPoints((short)16);
font1.setBold(true);
style.setFont(font1);
HSSFCellStyle styleSecond = wb.createCellStyle();
styleSecond.setAlignment(HorizontalAlignment.CENTER);
style.setVerticalAlignment(VerticalAlignment.CENTER);
styleSecond.setFillPattern(FillPatternType.SOLID_FOREGROUND);
styleSecond.setFillBackgroundColor(IndexedColors.GREY_50_PERCENT.getIndex());
styleSecond.setFillForegroundColor(IndexedColors.GREY_50_PERCENT.getIndex());
String str = "#FFFFFF";
int[] color=new int[3];
color[0]=Integer.parseInt(str.substring(1, 3), 16);
color[1]=Integer.parseInt(str.substring(3, 5), 16);
color[2]=Integer.parseInt(str.substring(5, 7), 16);
HSSFPalette palette = wb.getCustomPalette();
palette.setColorAtIndex(HSSFColor.BLACK.index,(byte)color[0], (byte)color[1], (byte)color[2]);
HSSFFont font2 = wb.createFont();
font2.setColor(HSSFColor.BLACK.index);
HSSFCellStyle cellStyle=wb.createCellStyle();
cellStyle.setFont(font2);
font2.setFontHeightInPoints((short)10);
font2.setFontName("Arial");
font2.setBold(true);
styleSecond.setFont(font2);
HSSFFont font3 = wb.createFont();
font3.setFontName("Arial");
font3.setFontHeightInPoints((short) 10);
HSSFCellStyle styleThree = wb.createCellStyle();
styleThree.setAlignment(HorizontalAlignment.CENTER);
styleThree.setVerticalAlignment(VerticalAlignment.CENTER);
styleThree.setWrapText(true);
styleThree.setFont(font3);
HSSFCellStyle linkStyle = wb.createCellStyle();
HSSFFont cellFont= wb.createFont();
cellFont.setColor(HSSFColor.BLUE.index);
linkStyle.setFont(cellFont);
linkStyle.setVerticalAlignment(VerticalAlignment.CENTER);
for(ExcelExp excel: sheets){
HSSFSheet sheet = wb.createSheet(excel.getFileName());
String[] handers = excel.getHanders();
sheet.addMergedRegion(new CellRangeAddress(0, 0, 0, handers.length-1));
HSSFRow rowFirst = sheet.createRow(0);
rowFirst.setHeightInPoints(16);
rowFirst.setHeight((short) 0x280);
HSSFCell cell = rowFirst.createCell(0);
cell.setCellValue(excel.getTitleName());
cell.setCellStyle(style);
rowFirst = sheet.createRow(1);
for(int i=0;i< handers.length;i++){
HSSFCell cell1 = rowFirst.createCell(i);
cell1.setCellValue(handers[i]);
cell1.setCellStyle(styleSecond);
sheet.setColumnWidth(i, 4000);
}
List<String[]> dataset = excel.getDataset();
for(int i=0;i<dataset.size();i++){
String[] data = dataset.get(i);
rowFirst = sheet.createRow(i+2);
for(int j=0;j<data.length;j++){
cell3 = rowFirst.createCell(j);
cell3.setCellValue(data[j]);
cell3.setCellStyle(styleThree);
if("成绩录入数据".equals(excel.getFileName())&&data[1].equals(data[j])){
Hyperlink hyperLink = createHelper.createHyperlink(HyperlinkType.DOCUMENT);
hyperLink.setAddress("#"+data[1]+"!A1");
cell3.setHyperlink(hyperLink);
cell3.setCellValue(data[1]);
cell3.setCellStyle(linkStyle);
}
}
}
}
try {
String filePath ="D:\\test";
String fileName ="\\成绩录入数据"+ DateUtil.format(DateUtil.now(), "yyyyMMddHHmmss") +".xls";
File file =new File(filePath);
if (!file .exists() && !file .isDirectory())
{
file.mkdirs();
}
FileOutputStream out = new FileOutputStream(filePath+fileName);
out.flush();
wb.write(out);
out.close();
getExcel(filePath,fileName,response);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void getExcel(String url, String fileName, HttpServletResponse response){
try {
response.setContentType("multipart/form-data");
response.setHeader("Content-Disposition", "attachment;filename="
+ new String(fileName.getBytes("gb2312"), "ISO-8859-1") + ".xls");
FileInputStream in = new FileInputStream(url+fileName);
OutputStream out = new BufferedOutputStream(response.getOutputStream());
int b = 0;
byte[] buffer = new byte[2048];
while ((b=in.read(buffer)) != -1){
out.write(buffer,0,b);
}
in.close();
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
コントローラーで使用
@GetMapping("/exportScoreEnter")
@ApiOperationSupport(order = 2)
@ApiOperation(value = "成绩录入数据", notes = "传入scoreEnterId")
public void expExcel(@RequestParam String ids, HttpServletResponse response) throws IOException {
SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd hh:mm");
List<ScoreEnterView> list = null;
List<ScoreEnterRecord> list2 = null;
List<ExcelExp> mysheet = new ArrayList<ExcelExp>();
Long[] idArr = Func.toLongArray(ids);
String url ="D:\\test";
list = scoreEnterService.getExportList(idArr);
String[] handers1 = {
"标签", "考试名称", "考试内容", "开始时间", "结束时间", "录入时间", "录入人", "参加人数"};
List<String[]> dataset = new ArrayList<String[]>();
ExcelExp e1 = new ExcelExp("成绩录入数据", "成绩录入数据", handers1, dataset);
ExcelExp e2 = null;
for (int i = 0; i < list.size(); i++) {
String[] arr = new String[8];
arr[0] = list.get(i).getTagName() == null ? "" : list.get(i).getTagName();
arr[1] = list.get(i).getExamTitle() == null ? "" : list.get(i).getExamTitle();
arr[2] = list.get(i).getTitle() == null ? "" : list.get(i).getTitle();
arr[3] = list.get(i).getStartDate() == null ? "" : sdf2.format(list.get(i).getStartDate());
arr[4] = list.get(i).getEndDate() == null ? "" : sdf2.format(list.get(i).getEndDate());
arr[5] = list.get(i).getEnterTime() == null ? "" : sdf2.format(list.get(i).getEnterTime());
arr[6] = list.get(i).getEnterPeople() == null ? "" : list.get(i).getEnterPeople();
arr[7] = list.get(i).getParticipateInNumber() == null ? "" : list.get(i).getParticipateInNumber().toString();
dataset.add(arr);
}
mysheet.add(e1);
for (int i = 0; i < list.size(); i++) {
list2 = scoreEnterRecordService.getByScoreEnterId(Func.toLong(list.get(i).getId()));
List<String[]> dataset2 = new ArrayList<String[]>();
String[] handers2 = {
"用户姓名", "单位名称", "部门名称", "分数"};
for (int j = 0; j < list2.size(); j++) {
String[] arr2 = new String[4];
arr2[0] = list2.get(j).getUserName() == null ? "" : list2.get(j).getUserName();
arr2[1] = list2.get(j).getFullName() == null ? "" : list2.get(j).getFullName();
arr2[2] = list2.get(j).getDeptName() == null ? "" : list2.get(j).getDeptName();
arr2[3] = list2.get(j).getExamScore() == null ? "" : list2.get(j).getExamScore().toString();
dataset2.add(arr2);
}
e2 = new ExcelExp("成绩详情数据", list.get(i).getExamTitle(), handers2, dataset2);
mysheet.add(e2);
}
ExcelExportUtil.exportManySheetExcel(mysheet,response);
}