1. クラス ライブラリの主要な部分を作成します: ログイン、デバイス時間の読み取り、デバイス時間の調整
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
public class HIK_NVR
{
public int m_lUserID = -1;
public string ip;
public ushort port = 8000;
public string user;
public string pass;
private uint iLastErr = 0;
public CHCNetSDK.NET_DVR_USER_LOGIN_INFO m_struLogInInfo;
public CHCNetSDK.LOGINRESULTCALLBACK LoginResultCallback;
public CHCNetSDK.NET_DVR_DEVICEINFO_V40 m_struDeviceInfo;
public CHCNetSDK.NET_DVR_TIME m_struTimeCfg;
public HIK_NVR(string _ip, ushort _port, string _user, string _pass)
{
ip = _ip;
port = _port;
user = _user;
pass = _pass;
}
public static bool Init()
{
bool flag = CHCNetSDK.NET_DVR_Init();
flag = CHCNetSDK.NET_DVR_SetLogToFile(3, $"{
AppDomain.CurrentDomain.BaseDirectory}SdkLog\\", true);
return flag;
}
public void LogIn(out string msg)
{
msg = "ok";
if (m_lUserID < 0)
{
m_struLogInInfo = new CHCNetSDK.NET_DVR_USER_LOGIN_INFO();
byte[] byIP = Encoding.Default.GetBytes(ip);
m_struLogInInfo.sDeviceAddress = new byte[129];
byIP.CopyTo(m_struLogInInfo.sDeviceAddress, 0);
m_struLogInInfo.wPort = port;
byte[] byUserName = Encoding.Default.GetBytes(user);
m_struLogInInfo.sUserName = new byte[64];
byUserName.CopyTo(m_struLogInInfo.sUserName, 0);
byte[] byPassword = Encoding.Default.GetBytes(pass);
m_struLogInInfo.sPassword = new byte[64];
byPassword.CopyTo(m_struLogInInfo.sPassword, 0);
m_struLogInInfo.bUseAsynLogin = false;
m_lUserID = CHCNetSDK.NET_DVR_Login_V40(ref m_struLogInInfo, ref m_struDeviceInfo);
if (m_lUserID == -1)
{
iLastErr = CHCNetSDK.NET_DVR_GetLastError();
msg = "登录失败,输出错误号" + iLastErr;
return;
}
else
{
}
}
else
{
if (!LogOut())
{
iLastErr = CHCNetSDK.NET_DVR_GetLastError();
msg = "注销登录失败,输出错误号:" + iLastErr;
}
else
{
m_lUserID = -1;
}
}
}
public bool GetNvrTime(out DateTime nvrDateTime, out string msg)
{
bool flag = false;
msg = "ok";
nvrDateTime = new DateTime(1970, 1, 1, 0, 0, 0);
try
{
uint dwReturn = 0;
int nSize = Marshal.SizeOf(m_struTimeCfg);
IntPtr ptrTimeCfg = Marshal.AllocHGlobal(nSize);
Marshal.StructureToPtr(m_struTimeCfg, ptrTimeCfg, false);
if (m_lUserID < 0)
{
LogIn(out msg);
}
if (!CHCNetSDK.NET_DVR_GetDVRConfig(m_lUserID, CHCNetSDK.NET_DVR_GET_TIMECFG, -1, ptrTimeCfg, (uint)nSize, ref dwReturn))
{
iLastErr = CHCNetSDK.NET_DVR_GetLastError();
msg = "获取设备时间失败,输出错误号: " + iLastErr;
nvrDateTime = new DateTime(1970, 1, 1, 0, 0, 0);
flag = false;
}
else
{
m_struTimeCfg = (CHCNetSDK.NET_DVR_TIME)Marshal.PtrToStructure(ptrTimeCfg, typeof(CHCNetSDK.NET_DVR_TIME));
nvrDateTime = new DateTime(
(int)m_struTimeCfg.dwYear,
(int)m_struTimeCfg.dwMonth,
(int)m_struTimeCfg.dwDay,
(int)m_struTimeCfg.dwHour,
(int)m_struTimeCfg.dwMinute,
(int)m_struTimeCfg.dwSecond
);
flag = true;
}
Marshal.FreeHGlobal(ptrTimeCfg);
}
catch (Exception ex)
{
flag = false;
msg = ex.Message;
}
return flag;
}
public bool SetNvrTime(DateTime nvrDateTime, out string msg)
{
bool flag = false;
msg = "ok";
try
{
m_struTimeCfg.dwYear = (uint)nvrDateTime.Year;
m_struTimeCfg.dwMonth = (uint)nvrDateTime.Month;
m_struTimeCfg.dwDay = (uint)nvrDateTime.Day;
m_struTimeCfg.dwHour = (uint)nvrDateTime.Hour;
m_struTimeCfg.dwMinute = (uint)nvrDateTime.Minute;
m_struTimeCfg.dwSecond = (uint)nvrDateTime.Second;
int nSize = Marshal.SizeOf(m_struTimeCfg);
IntPtr ptrTimeCfg = Marshal.AllocHGlobal(nSize);
Marshal.StructureToPtr(m_struTimeCfg, ptrTimeCfg, false);
if (m_lUserID < 0)
{
LogIn(out msg);
}
if (!CHCNetSDK.NET_DVR_SetDVRConfig(m_lUserID, CHCNetSDK.NET_DVR_SET_TIMECFG, -1, ptrTimeCfg, (uint)nSize))
{
iLastErr = CHCNetSDK.NET_DVR_GetLastError();
msg = "设置时间失败,输出错误号:" + iLastErr;
flag = false;
}
else
{
flag = true;
}
Marshal.FreeHGlobal(ptrTimeCfg);
}
catch (Exception ex)
{
flag = false;
msg = ex.Message;
}
return flag;
}
public bool LogOut()
{
return CHCNetSDK.NET_DVR_Logout(m_lUserID);
}
}
2. 設定ファイルの書き込み [ルール:分岐、カンマ区切り]
192.168.1.88,8000,admin,a1234567,录像机1
192.168.1.89,8000,admin,a1234567,录像机2
3. フォームのレイアウト、フォーム コントロールのドラッグ アンド ドロップ、コードの記述
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace 海康校时
{
public partial class 主窗体 : Form
{
public 主窗体()
{
InitializeComponent();
HIK_NVR.Init();
}
private void 主窗体_Load(object sender, EventArgs e)
{
var nvrList = File.ReadAllLines($@"{
AppDomain.CurrentDomain.BaseDirectory}nvr_list.txt");
HIK_NVR[] nvrs = new HIK_NVR[nvrList.Length];
DataTable dt = new DataTable();
dt.Columns.Add("设备名称");
dt.Columns.Add("设备IP地址");
dt.Columns.Add("设备端口");
dt.Columns.Add("设备时间");
dt.Columns.Add("本地电脑时间");
dt.Columns.Add("时钟差");
for (int i = 0; i < nvrList.Length; i++)
{
var nvrInfo = nvrList[i].Split(',');
nvrs[i] = new HIK_NVR(nvrInfo[0], ushort.Parse(nvrInfo[1]), nvrInfo[2], nvrInfo[3]);
dt.Rows.Add(new string[] {
nvrInfo[4], nvrInfo[0], nvrInfo[1], "", "", "" });
}
dataGridView1.DataSource = dt;
Thread th = new Thread(() =>
{
while (true)
{
for (int i = 0; i < nvrs.Length; i++)
{
if (nvrs[i].GetNvrTime(out DateTime nvrDateTime, out string msg))
{
var now = DateTime.Now;
var ts = (now - nvrDateTime).TotalSeconds;
if (Math.Abs(ts) > 5)
{
nvrs[i].SetNvrTime(DateTime.Now, out string m);
}
dt.Rows[i]["设备时间"] = $@"{
nvrDateTime:yyyy-MM-dd HH:mm:ss}";
dt.Rows[i]["本地电脑时间"] = $@"{
now:yyyy-MM-dd HH:mm:ss}";
dt.Rows[i]["时钟差"] = ts.ToString();
}
else
{
dt.Rows[i]["设备时间"] = msg;
}
}
Thread.Sleep(1000);
}
})
{
IsBackground = true
};
th.Start();
}
}
}
4. 運用観察効果
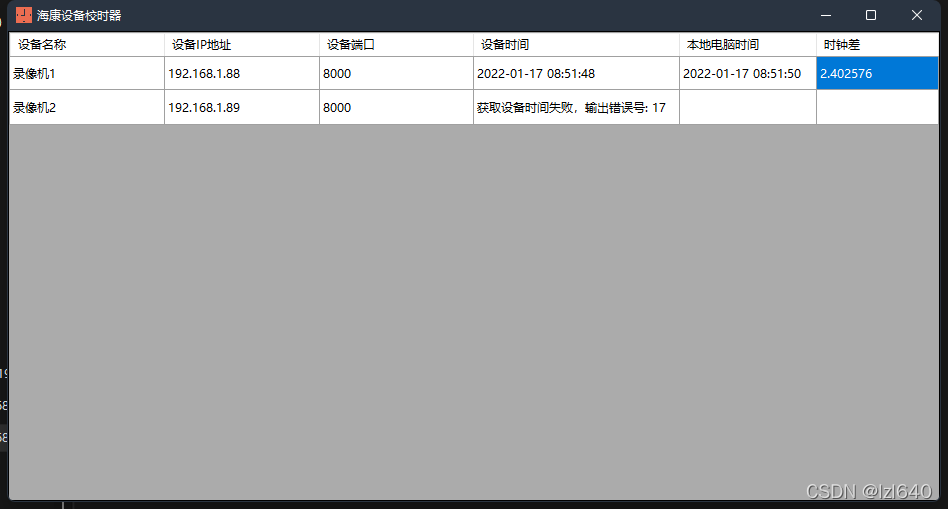