1. 1 つ目は json2image です。あまりナンセンスではありません。コードを見てください。
import base64
import json
import os
import os.path as osp
import uuid
import imgviz
import PIL.Image
from labelme import utils
if __name__ == "__main__":
base_dir = osp.dirname(osp.abspath(__file__))
out_dir_name = "output"
out_dir = osp.join(base_dir, out_dir_name)
if not osp.exists(out_dir):
os.mkdir(out_dir)
label_names = []
label_file = "./label.txt"
label_value_dict = {
}
with open(label_file) as f:
labels = f.readlines()
if len(labels) > 0:
for i, item in enumerate(labels):
item = item.strip()
label_value_dict[item] = i
json_file_name = "json"
json_file_path = osp.join(base_dir, json_file_name)
new_filename = 1095
for file_name in os.listdir(json_file_path):
print(file_name)
if file_name.endswith(".json"):
filePath = osp.join(json_file_path, file_name)
data = json.load(open(filePath))
imageData = data.get("imageData")
if not imageData:
imagePath = os.path.join(os.path.dirname(filePath),
data["imagePath"])
with open(imagePath, "rb") as f:
imageData = f.read()
imageData = base64.b64encode(imageData).decode("utf-8")
img = utils.img_b64_to_arr(imageData)
for shape in sorted(data["shapes"], key=lambda x: x["label"]):
label_name = shape["label"]
if label_name not in label_value_dict:
label_value = len(label_value_dict)
label_value_dict[label_name] = label_value
lbl, _ = utils.shapes_to_label(img.shape, data["shapes"],
label_value_dict)
label_names = [None] * (max(label_value_dict.values()) + 1)
for name, value in label_value_dict.items():
label_names[value] = name
lbl_viz = imgviz.label2rgb(lbl,
imgviz.asgray(img),
label_names=label_names,
loc="rb")
PIL.Image.fromarray(img).save(
osp.join(out_dir,
str(new_filename) + ".jpg"))
utils.lblsave(
osp.join(out_dir,
str(new_filename)+ ".png"), lbl)
new_filename = new_filename + 1
for name, value in label_value_dict.items():
label_names[value] = name
with open("./label.txt", "w") as f:
for lbl_name in label_names:
f.write(lbl_name + "\n")
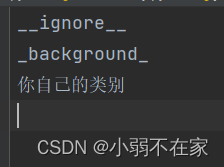
2.マスク2バイナリ
import cv2
import os
def convert_png2seg(input_dir,output_dir):
files = os.listdir(input_dir)
for file in files:
part = file.split('.')
part0 = part[0]
part1 = part[1]
img = cv2.imread(input_dir+file)
img_gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
retval, binary = cv2.threshold(img_gray, 10, 255, cv2.THRESH_BINARY)
cv2.imwrite(output_dir + part0 + '.' + part1, binary)
if __name__ == "__main__":
input_dir = r"./masks/"
output_dir = r"./output masks/"
convert_png2seg(input_dir,output_dir)
3. 画像のトリミング (必要な画像領域を横切る対角座標)
import cv2
import os
def update(input_img_path, output_img_path):
image = cv2.imread(input_img_path)
cropped = image[120:760, 100:940]
cv2.imwrite(output_img_path, cropped)
dataset_dir = 'images/1'
output_dir = 'out/1'
def auto_create_path(output_dir):
if os.path.exists(output_dir):
print( 'dir exists' )
else:
print( 'dir not exists')
os.makedirs(output_dir)
auto_create_path(output_dir)
image_filenames = [(os.path.join(dataset_dir, x), os.path.join(output_dir, x))
for x in os.listdir(dataset_dir)]
for path in image_filenames:
update(path[0], path[1])