腾讯精选50题—Day2题目7,8,9
今天是打卡的第二天,元气满满的冲鸭~~~
目录
1. 题目7 整数反转
(1)题目描述
Given a 32-bit signed integer, reverse digits of an integer.
Note: Assume we are dealing with an environment that could only store integers within the 32-bit signed integer range: [−231, 231 − 1]. For this problem, assume that your function returns 0 when the reversed integer overflows.
示例:
约束:
(2)题目思路
简单的反转数字题,注意不能越界。
(3)题解
class Solution {
public:
int reverse(int x) {
int sum = 0;
if(x>= -pow(2,31) && x <=(pow(2,31)-1))
{
//-2147483648 2147483647
while(x != 0)
{
int tmp = x % 10;//取末尾
if(sum > 214748364 || (sum == 214748364 && tmp > 7))
{
return 0;
}
if(sum < -214748364 || (sum == -214748364 && tmp <-8))
{
return 0;
}
sum = sum * 10 + tmp;
x /= 10;
}
}
else{
return 0;
}
return sum;
}
};
结果:
时间复杂度:
空间复杂度:
2. 题目8 实现C/C++ atoi函数
(1)题目描述
Implement the myAtoi(string s) function, which converts a string to a 32-bit signed integer (similar to C/C++'s atoi function).
The algorithm for myAtoi(string s) is as follows:
Read in and ignore any leading whitespace. Check if the next character (if not already at the end of the string) is '-' or '+'. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present. Read in next the characters until the next non-digit charcter or the end of the input is reached. The rest of the string is ignored. Convert these digits into an integer (i.e. "123" -> 123, "0032" -> 32). If no digits were read, then the integer is 0. Change the sign as necessary (from step 2). If the integer is out of the 32-bit signed integer range [-231, 231 - 1], then clamp the integer so that it remains in the range. Specifically, integers less than -231 should be clamped to -231, and integers greater than 231 - 1 should be clamped to 231 - 1. Return the integer as the final result.
Note: Only the space character ' ' is considered a whitespace character. Do not ignore any characters other than the leading whitespace or the rest of the string after the digits.
示例:
约束:
(2)题目思路
这道题目需要实现C/C++的atoi函数,以一种安全的方式将字符串转换为数字,如果单纯用判断条件来做也可以,但是容易出错,一种比较好的做法是使用构建确定的有限自动机的DFA,如图所示:
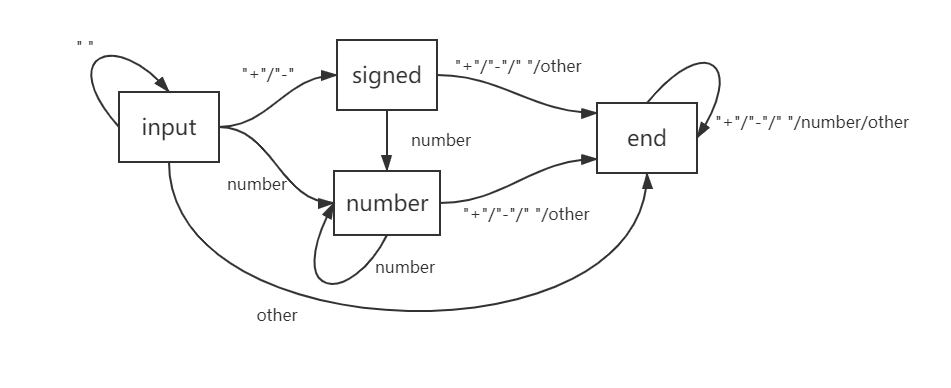
分析题目可知,我们可以对输入的字符串每一个字符进行分析,并不断变换状态state:
a. 首先到达input状态(初试状态),判断首字符是否是空格,如果是,则吸收n个空格字符,仍然停留在input状态,直到遇到下一个字符不是空格;如果首字符不是空格,则转移到end状态;
b. 如果遇到的下一个字符是"+"或者"-",那么转换到signed状态;再判断接下来遇到的字符,如果是数字,则转换到number状态;否则如果遇到的是非数字字符,例如"+"或者"-"或者" "或者其他字符,则转换到终止态end;
c. 如果遇到的下一个字符是数字,那么转换到number状态;不断吸收n个number字符;如果再遇到非数字的字符,例如"+"或者"-"或者" "或者其他字符,则转换到终止态end;
d. end状态还可以不断吸收n个任意字符。
(3)题解
题目关键点:
a. 构建DFA,转换为map存储和访问;
b. 越界判断。
class DFA
{
public:
long long ans = 0;
int sign = 1;
string state = "input";
unordered_map<string, vector<string>> table = {
{"input", {"input", "signed", "number", "end"}},
{"signed", {"end", "end", "number", "end"}},
{"number", {"end", "end", "number", "end"}},
{"end", {"end", "end", "end", "end"}}
};
int get_char(char ch)
{
if (ch == ' ')
return 0;
else if (ch == '+' || ch == '-')
return 1;
else if (isdigit(ch))
return 2;
else
return 3;
}
bool get(char ch)
{
state = table[state][get_char(ch)];
if (state == "number")
{
ans = ans * 10 + ch - '0';
if (sign == 1)
{
ans = min(ans, (long long)INT_MAX);
}
else {
ans = min(ans, -(long long)INT_MIN);
}
return true;
}
else if (state == "signed")
{
if (ch == '-')
sign = -1;
return true;
}
else if (state == "input")
{
return true;
}
else {
return false;
}
}
};
class Solution {
public:
int myAtoi(string str) {
DFA dfa;
for (int i=0; i<str.size(); i++)
{
char c = str[i];
bool flag = dfa.get(c);
if (!flag)
break;
}
return dfa.sign * dfa.ans;
}
};
运行结果:
额......需要避免DFA不必要的查询,等优化!!
时间复杂度:
空间复杂度:
3. 题目9 判断回文数字
(1)题目描述
Determine whether an integer is a palindrome. An integer is a palindrome when it reads the same backward as forward.
示例:
约束:
(2)题目思路
简单思路就是将数字转换为字符串,然后判断是否是回文,不将数字转换为字符串待优化~。
(3)题解
class Solution {
public:
bool isPalindrome(int x) {
string str = to_string(x);
int len = str.length();
for(int i=0; i<=len/2; i++)
{
if(str[i] != str[len-1-i])
return false;
}
return true;
}
};
结果:
时间复杂度:
空间复杂度: