JavaScript
Insert Style:
JS file can not be run directly embedded into the HTML file for an execution;
JavaScript code in the html file in any position, but we are generally on the head or body section of the page.
In the <head>
The most common way is to place <script> element in the head section of the page, the browser parses the code it will execute this head portion before the rest of the page is parsed.
On the <body> portion
JavaScript code to read the page when the statement is executed.
Comment:
Single-line comments before footnotes symbol "//."
Multi-line comments "/ *" beginning to end "* /."
variable:
Define variables using the keyword var, the following syntax:
var variable name
Variable names can be named, but to follow the naming rules:
1. Variables must be a letter, underscore (_) or dollar sign ($) to start.
2. Then you can use any number of letters, numbers, underscores (_) or dollar sign ($) composition.
3. You can not use JavaScript reserved words keywords and JavaScript.
First variable assignment statement again, can be repeated a variable assignment and is case sensitive in JS;
if the judge sentences:
grammar:
if (condition) {code is executed when the condition is satisfied} the else {code is executed when the condition is not satisfied}
function:
The basic syntax is as follows:
function name of the function () { function codes; }
Keyword 1. function defined functions.
2. "function" you take a function name.
3. "the function code" is replaced with the completion of a specific function code.
Call:
<the INPUT of the type = "the Button" value = "Click me" onclick = "function name" /> such as:

Output content:
Warning (alert message dialog box)
grammar:
alert (string or variable);
note:
1. In the dialog box, click the "OK" button before, can not perform any other operations.
2. The message dialog box can usually be used for debugging programs.
3. alert output content, or can be a string variable, with similar document.write.
Confirmation (confirm message dialog box)
grammar:
confirm(str);
Parameter Description:
str: dialog text message to be displayed Return Value: Boolean value
return value:
When the user clicks the "OK" button to return true when the user clicks the "Cancel" button to return false
Note: The return value can determine what the user clicks on the button
example:
<Script of the type = "text / JavaScript"> var = Confirm The MyMessage ( "JavaScript you like it?"); IF (== MyMessage to true) {document.write ( "well, come on!");} the else {the Document. write ( "JS powerful, to learn Oh!");} </ Script>
Question (prompt message dialog box)
prompt
弹出消息对话框,通常用于询问一些需要与用户交互的信息。弹出消息对话框(包含一个确定按钮、取消按钮与一个文本输入框)。
语法:
prompt(str1, str2);
参数说明:
str1: 要显示在消息对话框中的文本,不可修改 str2:文本框中的内容,可以修改
返回值:
1. 点击确定按钮,文本框中的内容将作为函数返回值 2. 点击取消按钮,将返回null
打开新窗口(window.open)
语法:
window.open([URL], [窗口名称], [参数字符串])
参数说明:
URL:可选参数,在窗口中要显示网页的网址或路径。如果省略这个参数,或者它的值是空字符串,那么窗口就不显示任何文档。 窗口名称:可选参数,被打开窗口的名称。 1.该名称由字母、数字和下划线字符组成。 2."_top"、"_blank"、"_self"具有特殊意义的名称。 _blank:在新窗口显示目标网页 _self:在当前窗口显示目标网页 _top:框架网页中在上部窗口中显示目标网页 3.相同 name 的窗口只能创建一个,要想创建多个窗口则 name 不能相同。 4.name 不能包含有空格。 参数字符串:可选参数,设置窗口参数,各参数用逗号隔开。
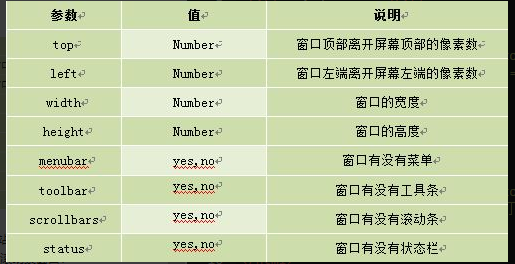
<script type="text/javascript">
window.open('http://www.imooc.com','_blank','width=300,height=200,menubar=no,toolbar=no, status=no,scrollbars=yes')
</script>
关闭窗口(window.close)
用法:
window.close(); //关闭本窗口
或
<窗口对象>.close(); //关闭指定的窗口
例子:
<script type="text/javascript"> var mywin=window.open('http://www.imooc.com'); //将新打的窗口对象,存储在变量mywin中 mywin.close(); </script>
注意:上面代码在打开新窗口的同时,关闭该窗口,看不到被打开的窗口。
HTML文档可以说由节点构成的集合,三种常见的DOM节点:
1. 元素节点:上图中<html>、<body>、<p>等都是元素节点,即标签。
2. 文本节点:向用户展示的内容,如<li>...</li>中的JavaScript、DOM、CSS等文本。
3. 属性节点:元素属性,如<a>标签的链接属性href="http://www.imooc.com"。
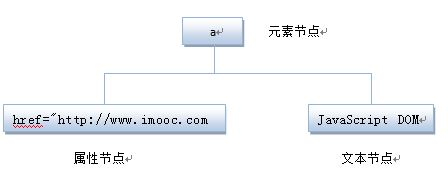
通过ID获取元素
语法:
document.getElementById("id")
结果:null或[object HTMLParagraphElement]
innerHTML 属性
innerHTML 属性用于获取或替换 HTML 元素的内容。
语法:
Object.innerHTML
注意:
1.Object是获取的元素对象,如通过document.getElementById("ID")获取的元素。
2.注意书写,innerHTML区分大小写。
例子:
<h2 id="con">javascript</H2>
<script type="text/javascript">
var mychar= document.getElementById("con") ;
document.write("原标题:"+mychar.innerHTML+"<br>"); //输出原h2标签内容
mychar.innerHTML = "Hello world";
document.write("修改后的标题:"+mychar.innerHTML); //输出修改后h2标签内容
</script>
改变 HTML 样式
语法:
Object.style.property=new style;
注意:Object是获取的元素对象,如通过document.getElementById("id")获取的元素。
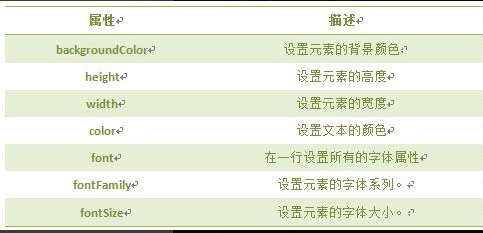
例子:
<p id="pcon">Hello World!</p> <script> var mychar = document.getElementById("pcon"); mychar.style.color="red"; mychar.style.fontSize="20"; mychar.style.backgroundColor ="blue"; </script>
显示和隐藏(display属性)
语法:
Object.style.display = value
注意:Object是获取的元素对象,如通过document.getElementById("id")获取的元素。
value取值:
例子:
<script type="text/javascript">
function hidetext()
{
var mychar = document.getElementById("con");
mychar.style.display = "none";
}
function showtext()
{
var mychar = document.getElementById("con");
mychar.style.display = "block";
}
</script>
<p id="con">实验内容</p>
<form>
<input type="button" onclick="hidetext()" value="隐藏内容" />
<input type="button" onclick="showtext()" value="显示内容" />
</form>
控制类名(className 属性)
语法:
object.className = classname
作用:
1.获取元素的class 属性
2. 为网页内的某个元素指定一个css样式来更改该元素的外观