654. Maximum Binary Tree
Given an integer array with no duplicates. A maximum tree building on this array is defined as follow:
- The root is the maximum number in the array.
- The left subtree is the maximum tree constructed from left part subarray divided by the maximum number.
- The right subtree is the maximum tree constructed from right part subarray divided by the maximum number.
Construct the maximum tree by the given array and output the root node of this tree.
------------------------------------------------------------------------------------------------------------------
Given an array of integers is not repeated. Maximal tree defined on the array to establish the following:
- The root is the maximum number of array.
- The left subtree is divided by the maximum number of sub-array obtained from the largest tree on the left.
- Right subtree is the largest tree structure part of the right to sub-array divided by the maximum number.
Through a given array configuration tree and the maximum output of the root node of the tree.
------------------------------------------------------------------------------------------------------------------
Example 1:
Input: [3,2,1,6,0,5] Output: return the tree root node representing the following tree: 6 / \ 3 5 \ / 2 0 \ 1
Note:
- The size of the given array will be in the range [1,1000].
The subject is not difficult, it is easy to think Solutions:
- Create a root node, which is the maximum value of the array
- The maximum value of the left half of the array to create the root node of the left, the left node
- The maximum value of the right half of the array to create the right node of the root node, right node of
- Recursive above operations
# Definition for a binary tree node. # class TreeNode: # def __init__(self, x): # self.val = x # self.left = None # self.right = None class Solution: def constructMaximumBinaryTree(self, nums: List[int]) -> TreeNode: if nums ==[]: return None index = nums.index(max(nums)) root = TreeNode(max(nums)) root.left = self.constructMaximumBinaryTree(nums[:index]) root.right = self.constructMaximumBinaryTree(nums[index+1:]) return root
Given two binary search trees root1
and root2
.
Return a list containing all the integers from both trees sorted in ascending order.
Example 1:
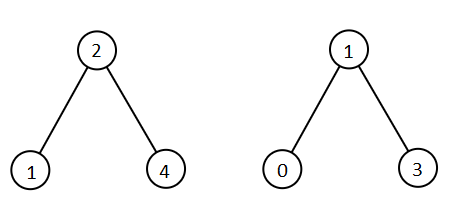
Input: root1 = [2,1,4], root2 = [1,0,3] Output: [0,1,1,2,3,4]
Example 2:
Input: root1 = [0,-10,10], root2 = [5,1,7,0,2] Output: [-10,0,0,1,2,5,7,10]
Example 3:
Input: root1 = [], root2 = [5,1,7,0,2] Output: [0,1,2,5,7]
Example 4:
Input: root1 = [0,-10,10], root2 = [] Output: [-10,0,10]
Example 5:
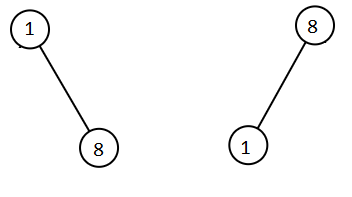
Input: root1 = [1,null,8], root2 = [8,1] Output: [1,1,8,8]
Constraints:
- Each tree has at most
5000
nodes. - Each node's value is between
[-10^5, 10^5]
.