Outline
java.langObject class is the root class in the Java language, that is the parent of all classes. All methods described it (including arrays and custom class) can use subclass. . In the object instantiation when the final look of the parent class is Object. If a class is not specified parent class, then the default is inherited from the Object class. E.g:
public class MyClass /*extends Object*/ { // ... }
Common method
The JDK API documentation and source code Object class, which method comprises the Object class has 11
- public String toString (): Returns a string representation of the object.
toString method returns a string representation of the object, in fact, is the type of content that the string @ + + memory address value of the object. As a result toString method returns the memory address, and in development, often we need to give the corresponding string representation according to properties of the object, and therefore it needs to be rewritten.
Code Example
The definition of the Person class
Package demo01; public class the Person { // definition of member variables Private String name; Private int Age; // definition of the All parameters / constructor with no arguments public the Person () { } public the Person (String name, int Age) { the this .name = name; the this .age = Age; } // define the get / set public String getName () { return name; } public void the setName (String name) { the this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
Defines the test class
Package demo01; public class PersonTest { public static void main (String [] args) { the Person Person = new new the Person ( "Joe Smith", 18 is ); // the Person class inherits default Object class, Object class can be used in toString method System.out.println (person.toString ()); // name direct printing objects, in fact, call the object of the Person = person.toString toString (); // see if a class overrides the toString, direct print this object class can, if no override toString method is that printing the address value of the object System.out.println (Person); } }
The result of code execution
Person class toString method of rewriting
Package demo01; public class the Person { // definition of member variables Private String name; Private int Age; // override toString method @Override public String toString () { return "the Person {" + "name = '" + name +' \ '' + ", Age =" + Age + '}' ; } // definition of the All parameters / constructor with no arguments public the Person () { } public the Person (String name, int Age) { the this .name = name; this.age = age; } //定义get/set方法 public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
Perform a test class again the following results:
tips:
In IntelliJ IDEA, you can click on the Code menu Generate ..., you can also use the shortcut alt + insert, click toString () option. Select the member variables need to be included and OK. As shown below:
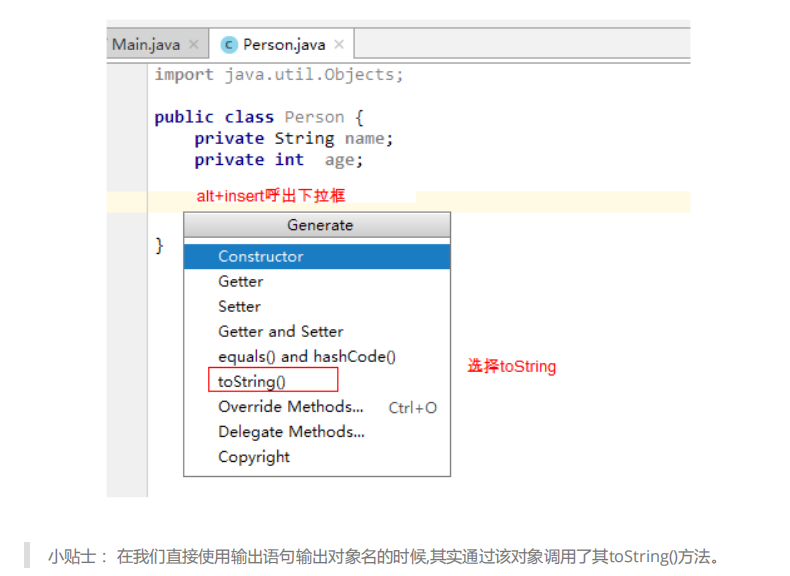
- public boolean equals (Object obj): indicate whether some other object with this object is "equal"
调用成员方法equals并指定参数为另一个对象,则可以判断这两个对象是否是相同的。这里的“相同”有默认和自定义两种方式。
- 默认地址比较:如果没有覆盖重写equals方法,那么Object类中默认进行==运算符的对象地址比较,只要不是同一个对象,结果必然为false。
public boolean equals(Object obj) { /* 详解: 方法参数:Object obj:可以接受传递任意的对象 this是谁?那个对象调用的方法,方法中的this就是那个对象;obj是谁?传递过来的参数是谁obj就是谁 == 比较运算符,返回一个boolean值 如果是基本数据类型:则==运算符比较的是值 如果是引用数据类型==运算符比较的是两个对象的地址值 */ return (this == obj); }
- 对象内容比较:如果希望进行对象的内容比较,即所有或指定的部分成员变量相同就判定两个对象相同,则可以覆盖重写equals方法。
package demo01; import java.util.Objects; public class Person { //定义成员变量 private String name; private int age; public boolean equals(Object o) { // 如果对象地址一样,则认为相同 if (this == o) return true; // 如果参数为空,或者类型信息不一样,则认为不同 if (o == null || getClass() != o.getClass()) return false; // 转换为当前类型 Person person = (Person) o; // 要求基本类型相等,并且将引用类型交给java.util.Objects类的equals静态方法取用结果 return age == person.age && Objects.equals(name, person.name); } }
这段代码充分考虑了对象为空、类型一致等问题,但方法内容并不唯一。大多数IDE都可以自动生成equals方法的代码内容。在IntelliJ IDEA中,可以使用 Code 菜单中的 Generate…选项,也可以使用快捷键 alt+insert ,并选择 equals() and hashCode() 进行自动代码生成。如下图所示:
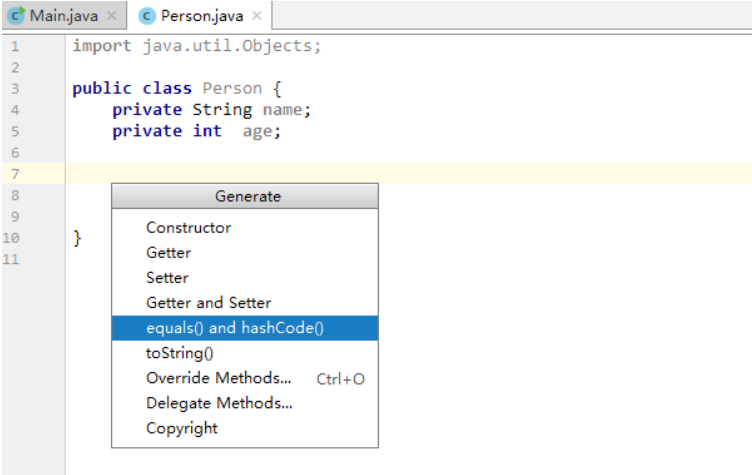
未完待续