Recommended sql.js-- a pure js the sqlite tool.
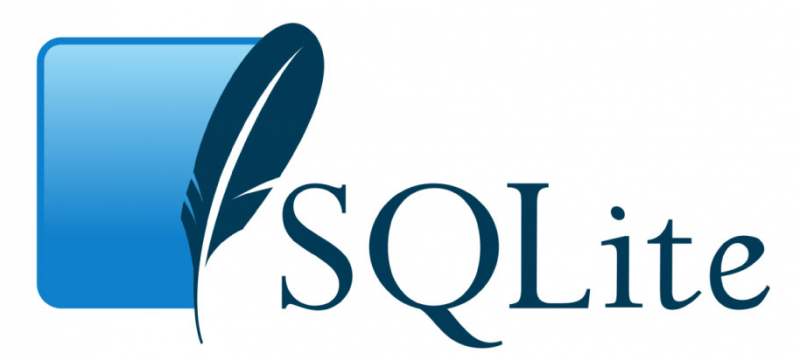
First, on sql.js
sql.js (https://github.com/kripken/sql.js) by using Emscripten SQLite compiling C code, SQLite transplanted to Webassembly. It uses virtual database files are stored in memory, it will not retain the changes made to the database. However, it allows you to import any existing sqlite file, and export the database created for the type of JavaScript arrays.
There is no binding or node-gyp C compiler, sql.js is a simple JavaScript file, like any traditional use as JavaScript libraries. In building native applications (for example, if you're using JavaScript Electron
), or work in node.js, then you may prefer to use a local SQLite bindings and the JavaScript (https://www.npmjs.com/package/ sqlite3).
SQLite is in the public domain, sql.js is MIT licensed.
Sql.js earlier than WebAssembly, so start from asm.js project. It still supports asm.js for backward compatibility.
Second, why should sql.js
When developing applications using electron Node native (https://electronjs.org/docs/tutorial/using-native modules electron official document of if you want to use sqlite3, except npm install step should rebuild, too much trouble, see -node-modules).
If you are looking for a sql database ready to use out of the box, then sql.js would be a good choice. sql.js is Webassembly version of sqlite, sqlite use and basically no difference. sql.js support CDN introduced directly into the browser, or download sql-wasm.js and sql-wasm.wasm into the local, supports npm introduced.
Third, the installation sql.js in Electron project
1, assume that we have built a good project a hello world Electron, such as https://xushanxiang.com/2019/11/installing-electron.html
2, installation sql.js
cnpm install sql.js --save
After installation, see the directory structure:
tree node_modules/sql.js -L 2
node_modules/sql.js
├── AUTHORS
├── GUI
│ └── index.html
├── LICENSE
├── Makefile
├── README.md
├── cache
│ ├── check.txt
│ ├── extension-functions.c
│ └── sqlite-amalgamation-3280000.zip
├── dist
│ ├── sql-asm-debug.js
│ ├── sql-asm-memory-growth.js
│ ├── sql-asm.js
│ ├── sql-wasm-debug.js
│ ├── sql-wasm-debug.wasm
│ ├── sql-wasm.js
│ ├── sql-wasm.wasm
│ ├── worker.sql-asm-debug.js
│ ├── worker.sql-asm.js
│ ├── worker.sql-wasm-debug.js
│ └── worker.sql-wasm.js
├── examples
│ ├── GUI
│ ├── README.md
│ ├── persistent.html
│ ├── repl.html
│ ├── requireJS.html
│ ├── simple.html
│ └── start_local_server.py
├── index.html
├── out
│ ├── api.js
│ ├── extension-functions.bc
│ ├── sqlite3.bc
│ └── worker.js
├── package.json
├── sqlite-src
│ └── sqlite-amalgamation-3280000
└── src
├── api-data.coffee
├── api.coffee
├── exported_functions.json
├── exported_runtime_methods.json
├── exports.coffee
├── output-post.js
├── output-pre.js
├── shell-post.js
├── shell-pre.js
└── worker.coffee
9 directories, 41 files
dist directory Description See https://github.com/kripken/sql.js#versions-of-sqljs-included-in-the-distributed-artifacts
Fourth, in the Electron project, how to use the SQLite database operations sql.js
1, create a SQLite database
We can create the command line, you can create an online SQL interpreter (http://kripken.github.io/sql.js/examples/GUI/) and a download.
When writing code, you can refer to the full documentation generated by the source code comments --http: //kripken.github.io/sql.js/documentation/#http: //kripken.github.io/sql.js/ documentation / class / Database.html
We use the latter to download a database sql.db into the project root directory. Look at our current project file structure:
d tree -L 1
.
├── index.html
├── main.js
├── node_modules
├── package.json
└── sql.db
1 directory, 4 files
Of course, we can also run the project, to create a dynamic, but create a static file directory, copy the relevant files to a static file directory.
→ mkdir -p static/js
→ cp node_modules/sql.js/dist/sql-wasm.* static/js/
→ ls static/js/
sql-wasm.js sql-wasm.wasm
And then change the contents of the index.html file:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>create the database</title>
</head>
<body>
<div id="result"></div>
<script src='./static/js/sql-wasm.js'></script>
<!--如果在主进程中,则用var initSqlJs=require('sql-wasm.js')-->
<script>
var fs = require("fs");
var config = {
// 指定加载sql-wasm.wasm文件的位置
locateFile: filename => `./static/js/${filename}`
};
initSqlJs(config).then(SQL => {
// 创建数据库
var db = new SQL.Database();
// 运行查询而不读取结果
db.run("CREATE TABLE test (col1, col2);");
// 插入两行:(1,111) and (2,222)
db.run("INSERT INTO test VALUES (?,?), (?,?)", [1,111,2,222]);
// 将数据库导出到包含SQLite数据库文件的Uint8Array
// export() 返回值: ( Uint8Array ) — SQLite3数据库文件的字节数组
var data = db.export();
// 由于安全性和可用性问题,不建议使用Buffer()和new Buffer()构造函数
// 改用new Buffer.alloc()、Buffer.allocUnsafe()或Buffer.from()构造方法
// var buffer = new Buffer(data);
var buffer = Buffer.from(data, 'binary');
// 被创建数据库名称
var filename = "d.sqlite";
fs.writeFileSync(filename, buffer);
document.querySelector('#result').innerHTML = filename + "Created successfully.";
});
</script>
</body>
</html>
If you do not SQLiteStudio like software installation, database files can be loaded dynamically created above http://kripken.github.io/sql.js/examples/GUI/, and then execute the following SQL statement to get the results table below.
SELECT * FROM test
col1 | col2 |
---|---|
1 | 111 |
2 | 222 |
2, SQLite database query operations
Replace the index.html file as follows:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Execute SELECT sql</title>
</head>
<body>
<div id="result"></div>
<script src='./static/js/sql-wasm.js'></script>
<script>
var fs = require("fs");
var filebuffer = fs.readFileSync('d.sqlite');
var config = {
// 指定加载sql-wasm.wasm文件的位置
locateFile: filename => `./static/js/${filename}`
};
initSqlJs(config).then(SQL => {
// 加载数据库到内存中
var db = new SQL.Database(filebuffer);
var res = db.exec("SELECT * FROM test");
console.log(JSON.stringify(res));
// [{"columns":["col1","col2"],"values":[[1,111],[2,222]]}]
});
</script>
</body>
</html>
3, SQLite database to add, change, delete operation
Replace the index.html file as follows:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Execute SELECT sql</title>
</head>
<body>
<div id="result"></div>
<script src='./static/js/sql-wasm.js'></script>
<script>
var fs = require("fs");
var filebuffer = fs.readFileSync('d.sqlite');
var config = {
// 指定加载sql-wasm.wasm文件的位置
locateFile: filename => `./static/js/${filename}`
};
initSqlJs(config).then(SQL => {
// 加载数据库到内存中
var db = new SQL.Database(filebuffer);
// 插入两行:(1,111) and (2,222)
db.run("INSERT INTO test VALUES (?,?), (?,?)", [3,333,4,444]);
var res = db.exec("SELECT * FROM test");
console.log(JSON.stringify(res));
// [{"columns":["col1","col2"],"values":[[1,111],[2,222],[3,333],[4,444]]}]
// 修改一行
db.run("UPDATE test SET col2=(?) WHERE col1=(?)", [3333,3]);
res = db.exec("SELECT * FROM test");
console.log(JSON.stringify(res));
// [{"columns":["col1","col2"],"values":[[1,111],[2,222],[3,3333],[4,444]]}]
// 删除一行
db.run("DELETE FROM test WHERE col1=(?)", [4]);
res = db.exec("SELECT * FROM test");
console.log(JSON.stringify(res));
// [{"columns":["col1","col2"],"values":[[1,111],[2,222],[3,3333]]}]
// 注意:上面的增、改、删都只是对载入内存中的数据进行变更,我们需要把内存中的数据再存入磁盘文件中,不然一切皆枉然。
var data = db.export();
var buffer = Buffer.from(data, 'binary');
fs.writeFileSync("d.sqlite", buffer);
document.querySelector('#result').innerHTML = "SQLite database changed.";
});
</script>
</body>
</html>
Original: https://xushanxiang.com/2019/11/electron-sql-js-sqlite.html