A. Installation Prism
1. Use Package Manager Console #
Install-Package Prism.Unity -Version 7.2.0.1367
2. Use the package management solutions Nuget #
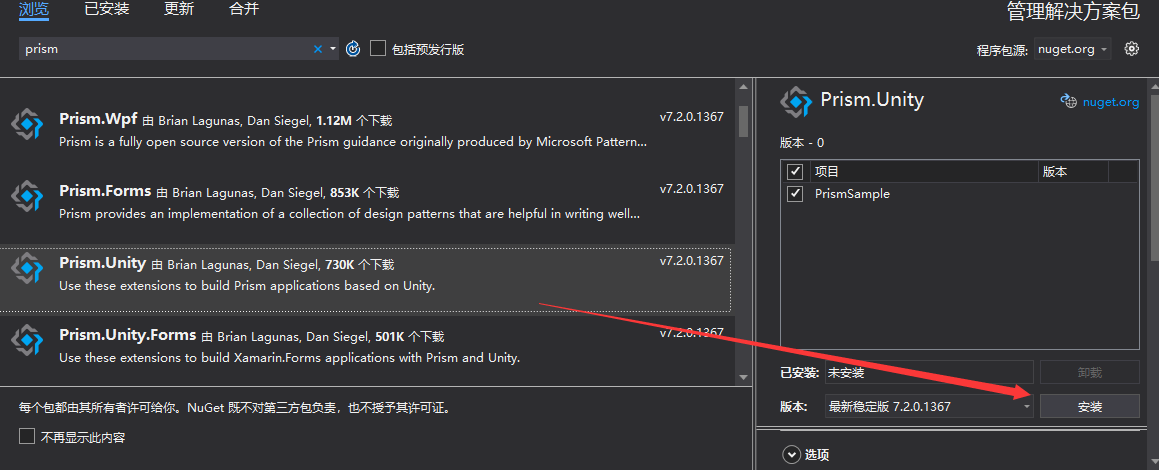
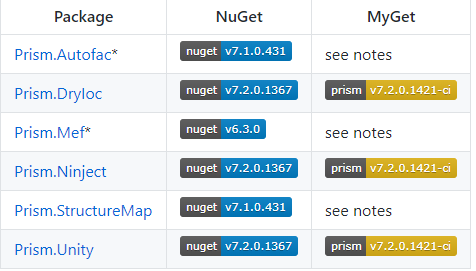
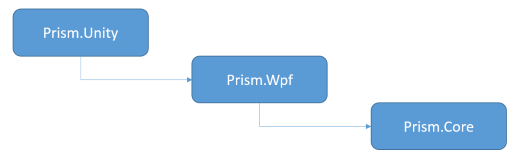
II. Data binding
We create folders and ViewModels Views folder, MainWindow placed Views folder, then create the following folder in ViewModels MainWindowViewModel categories, as follows:
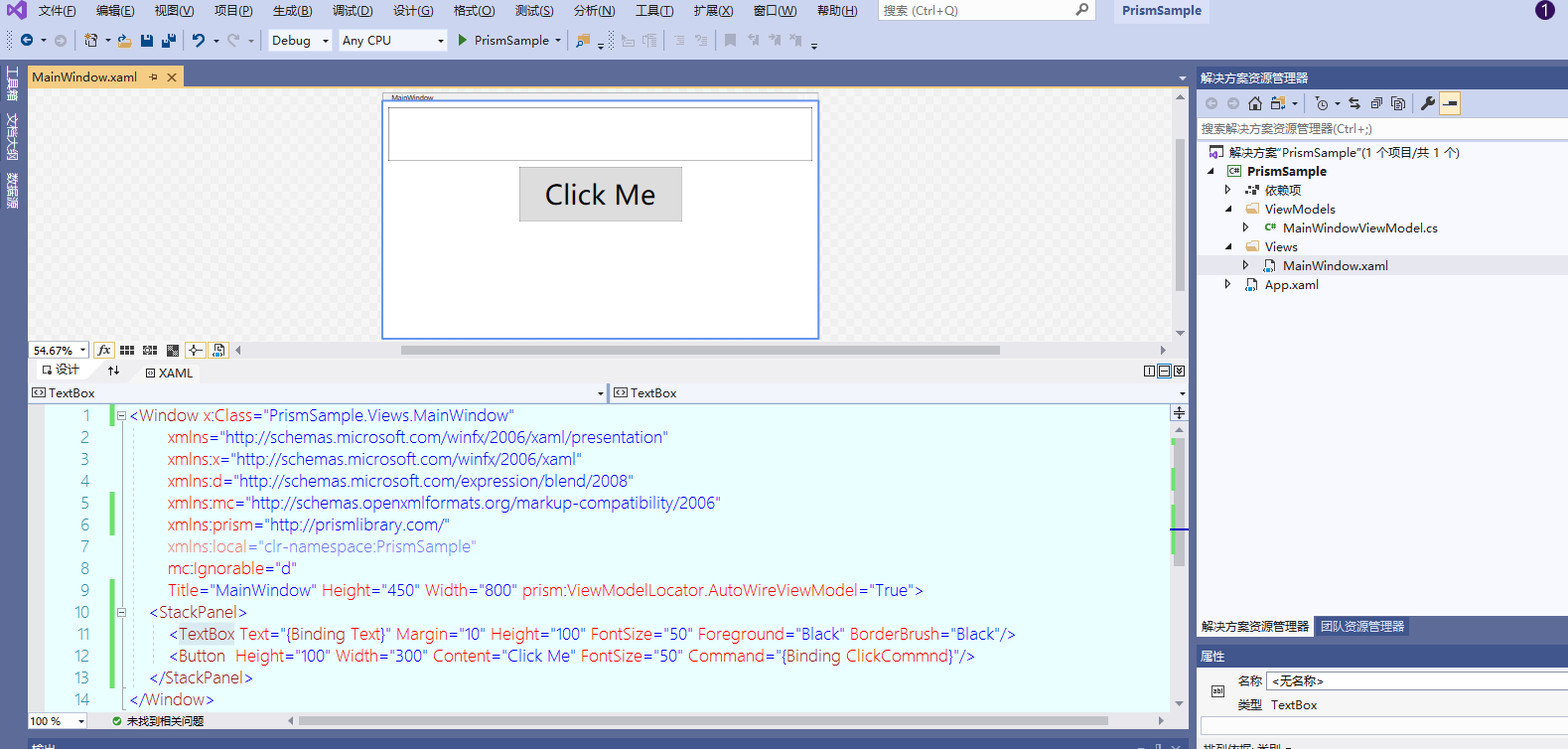
<Window x:Class="PrismSample.Views.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:prism="http://prismlibrary.com/" xmlns:local="clr-namespace:PrismSample" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800" prism:ViewModelLocator.AutoWireViewModel="True"> <StackPanel> <TextBox Text="{Binding Text}" Margin="10" Height="100" FontSize="50" Foreground="Black" BorderBrush="Black"/> <Button Height="100" Width="300" Content="Click Me" FontSize="50" Command="{Binding ClickCommnd}"/> </StackPanel> </Window>
using Prism.Commands; using Prism.Mvvm; namespace PrismSample.ViewModels { public class MainWindowViewModel:BindableBase { private string _text; public string Text { get { return _text; } set { SetProperty(ref _text, value); } } private DelegateCommand _clickCommnd; public DelegateCommand ClickCommnd => _clickCommnd ?? (_clickCommnd = new DelegateCommand(ExecuteClickCommnd)); void ExecuteClickCommnd() { this.Text = "Click Me!"; } public MainWindowViewModel() { this.Text = "Hello Prism!"; } } }
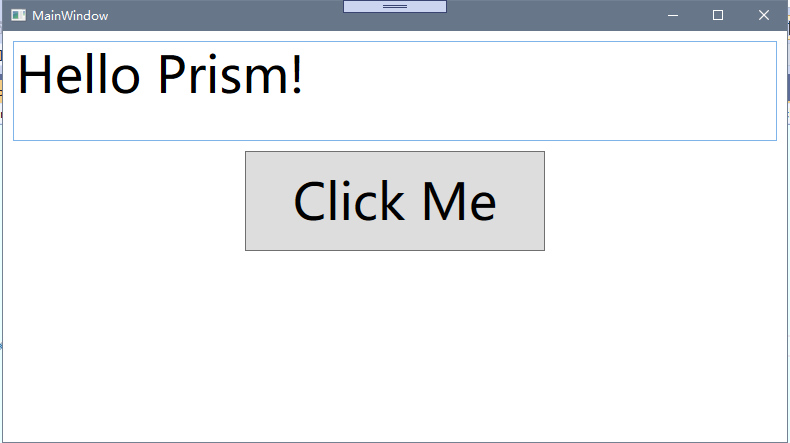
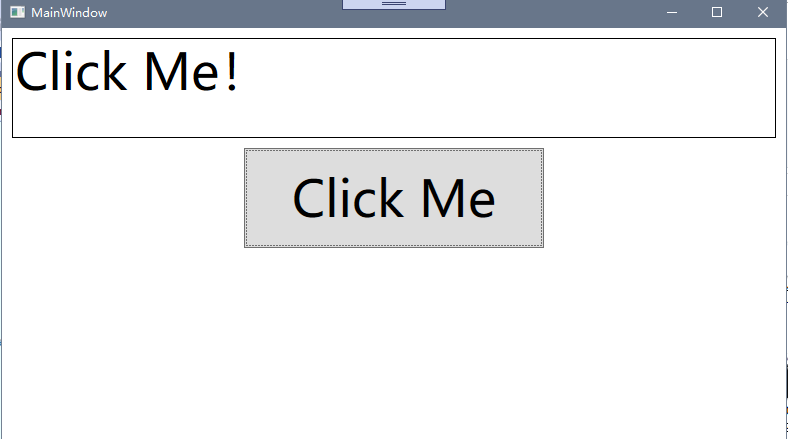
You can see, we have successfully used the prism data binding, and View and ViewModel perfect separation of the front and rear end
But now we also raises another question, when we do not want to follow the provisions of the prism will insist on the View and ViewModel and Views ViewModels inside, and perhaps your own project named rules vary from how to do, this time we should use In addition several ways:
1. Change the naming #
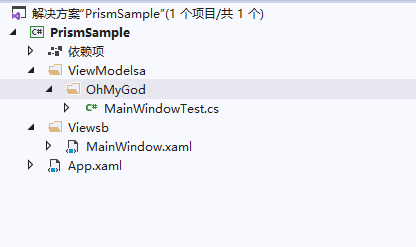
<prism:PrismApplication x:Class="PrismSample.App" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" xmlns:local="clr-namespace:PrismSample"> <Application.Resources> </Application.Resources> </prism:PrismApplication>
using Prism.Unity; using Prism.Ioc; using Prism.Mvvm; using System.Windows; using PrismSample.Viewsb; using System; using System.Reflection; namespace PrismSample { /// <summary> /// Interaction logic for App.xaml /// </summary> public partial class App : PrismApplication { //设置启动起始页 protected override Window CreateShell() { return Container.Resolve<MainWindow>(); } protected override void RegisterTypes(IContainerRegistry containerRegistry) { } //配置规则 protected override void ConfigureViewModelLocator() { base.ConfigureViewModelLocator(); ViewModelLocationProvider.SetDefaultViewTypeToViewModelTypeResolver((viewType) => { var viewName = viewType.FullName.Replace(".Viewsb.", ".ViewModelsa.OhMyGod."); var viewAssemblyName = viewType.GetTypeInfo().Assembly.FullName; var viewModelName = $"{viewName}Test, {viewAssemblyName}"; return Type.GetType(viewModelName); }); } } }
1
|
var
viewName = viewType.FullName.Replace(
".Viewsb."
,
".ViewModelsa.OhMyGod."
);
|
var viewModelName = $"{viewName}Test, {viewAssemblyName}";
2. Custom ViewModel registration #
using Prism.Commands; using Prism.Mvvm; namespace PrismSample { public class Foo:BindableBase { private string _text; public string Text { get { return _text; } set { SetProperty(ref _text, value); } } public Foo() { this.Text = "Foo"; } private DelegateCommand _clickCommnd; public DelegateCommand ClickCommnd => _clickCommnd ?? (_clickCommnd = new DelegateCommand(ExecuteClickCommnd)); void ExecuteClickCommnd() { this.Text = "Oh My God!"; } } }
protected override void ConfigureViewModelLocator() { base.ConfigureViewModelLocator(); ViewModelLocationProvider.Register<MainWindow, Foo>(); //ViewModelLocationProvider.SetDefaultViewTypeToViewModelTypeResolver((viewType) => //{ // var viewName = viewType.FullName.Replace(".Viewsb.", ".ViewModelsa.OhMyGod."); // var viewAssemblyName = viewType.GetTypeInfo().Assembly.FullName; // var viewModelName = $"{viewName}Test, {viewAssemblyName}"; // return Type.GetType(viewModelName); //}); }
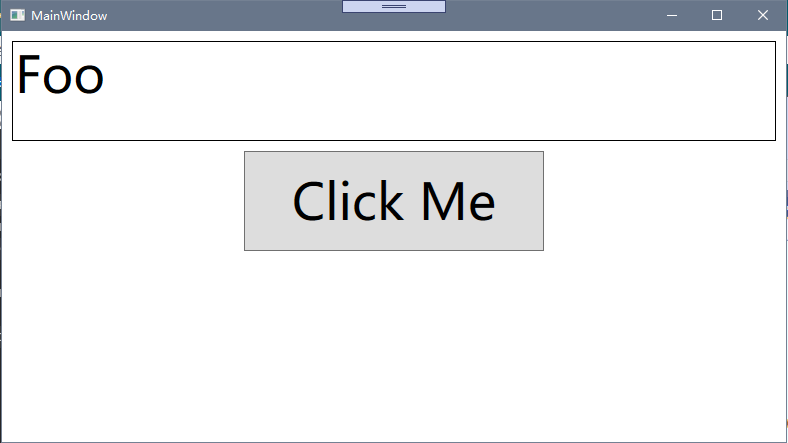
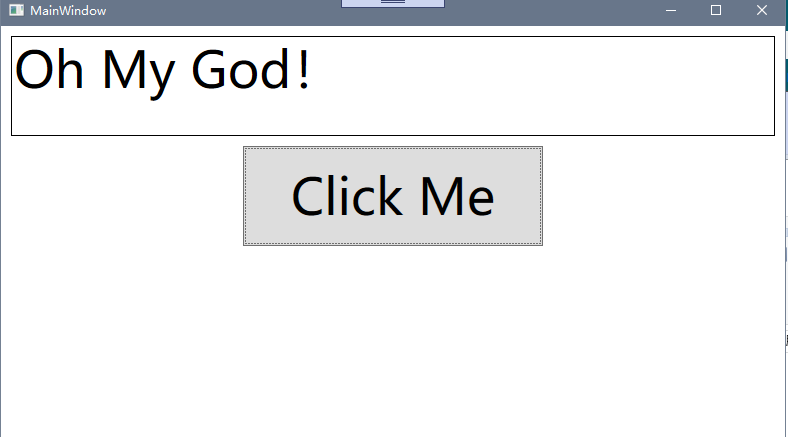
Even if the comment is not naming modify code, we find that the result is still the same run, so we can conclude,
This direct, not through reflection registered custom registration methods will be high priority in the official document also shows that in this way the efficiency will be high
And the official four ways, the other three are registered as follows:
ViewModelLocationProvider.Register(typeof(MainWindow).ToString(), typeof(MainWindowTest));
ViewModelLocationProvider.Register(typeof(MainWindow).ToString(), () => Container.Resolve<Foo>());
ViewModelLocationProvider.Register<MainWindow>(() => Container.Resolve<Foo>());
A. Installation Prism
1. Use Package Manager Console #
Install-Package Prism.Unity -Version 7.2.0.1367
2. Use the package management solutions Nuget #
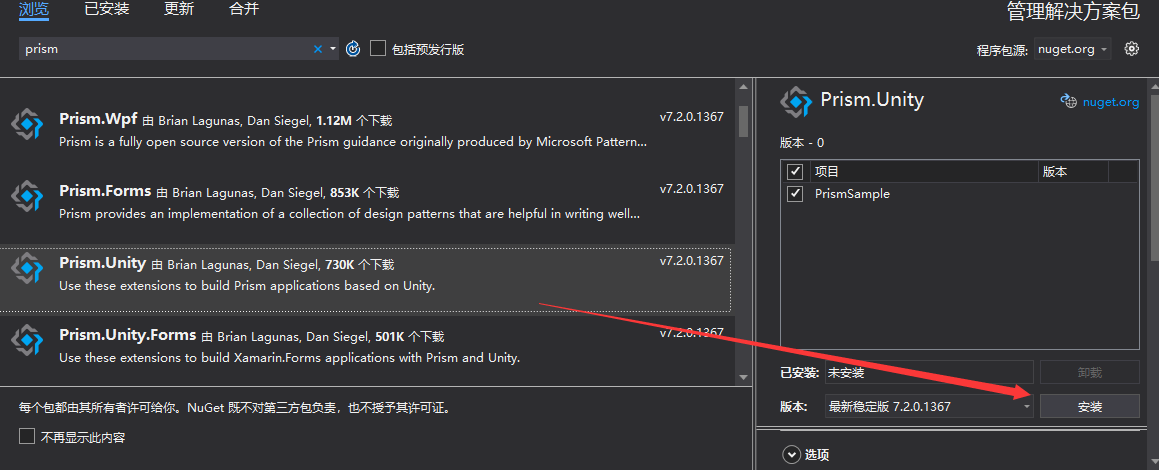
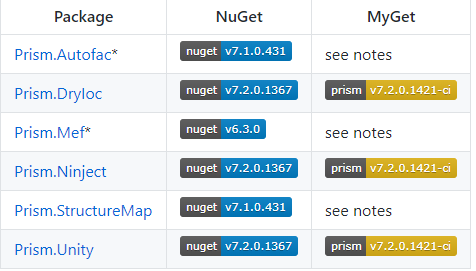
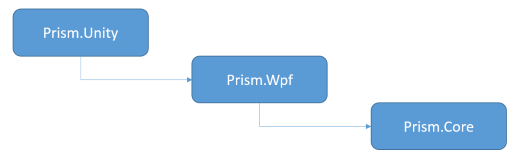
II. Data binding
We create folders and ViewModels Views folder, MainWindow placed Views folder, then create the following folder in ViewModels MainWindowViewModel categories, as follows:
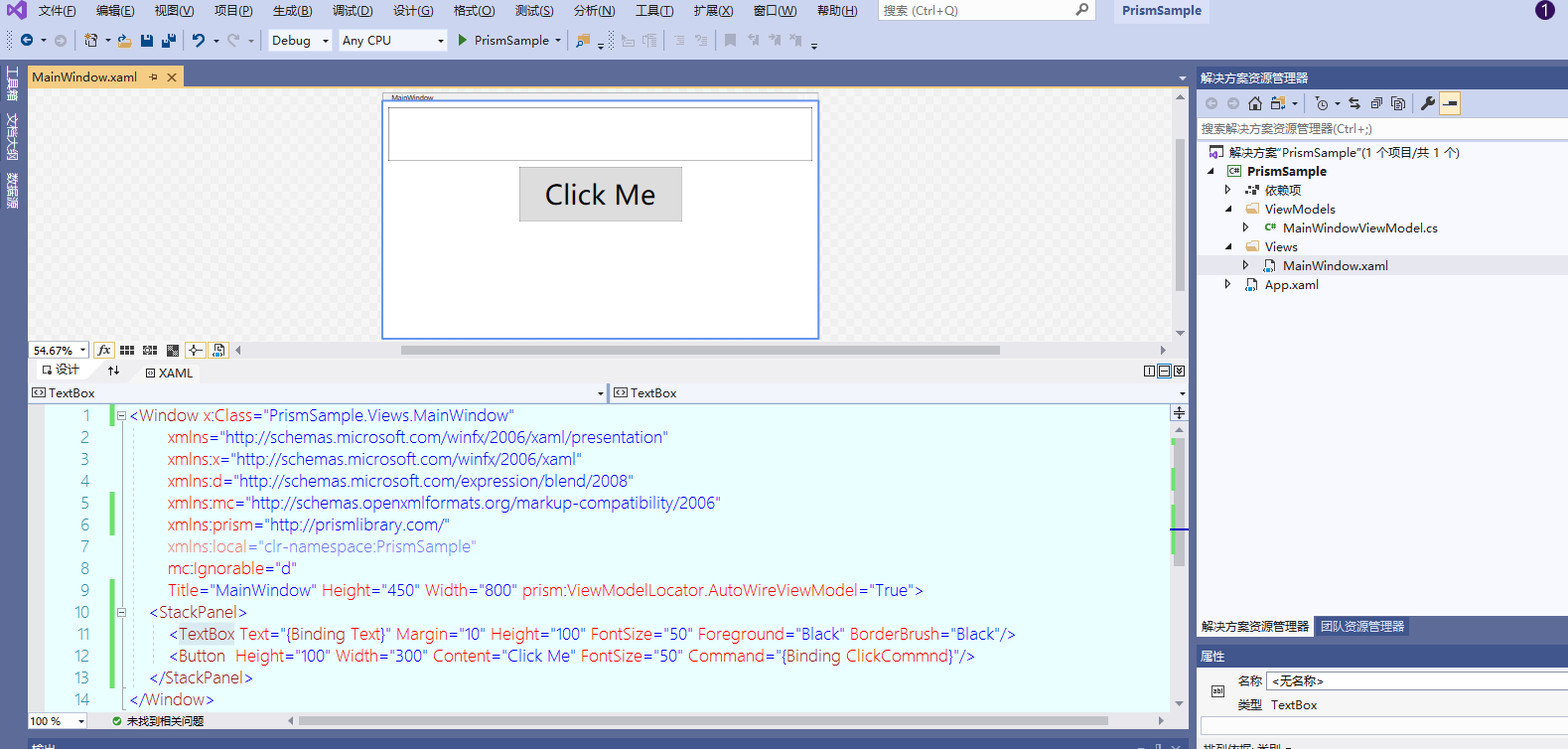
<Window x:Class="PrismSample.Views.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:prism="http://prismlibrary.com/" xmlns:local="clr-namespace:PrismSample" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800" prism:ViewModelLocator.AutoWireViewModel="True"> <StackPanel> <TextBox Text="{Binding Text}" Margin="10" Height="100" FontSize="50" Foreground="Black" BorderBrush="Black"/> <Button Height="100" Width="300" Content="Click Me" FontSize="50" Command="{Binding ClickCommnd}"/> </StackPanel> </Window>
using Prism.Commands; using Prism.Mvvm; namespace PrismSample.ViewModels { public class MainWindowViewModel:BindableBase { private string _text; public string Text { get { return _text; } set { SetProperty(ref _text, value); } } private DelegateCommand _clickCommnd; public DelegateCommand ClickCommnd => _clickCommnd ?? (_clickCommnd = new DelegateCommand(ExecuteClickCommnd)); void ExecuteClickCommnd() { this.Text = "Click Me!"; } public MainWindowViewModel() { this.Text = "Hello Prism!"; } } }
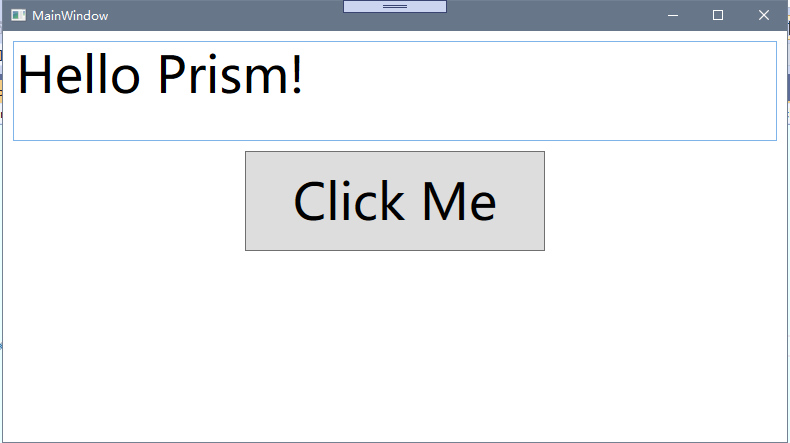
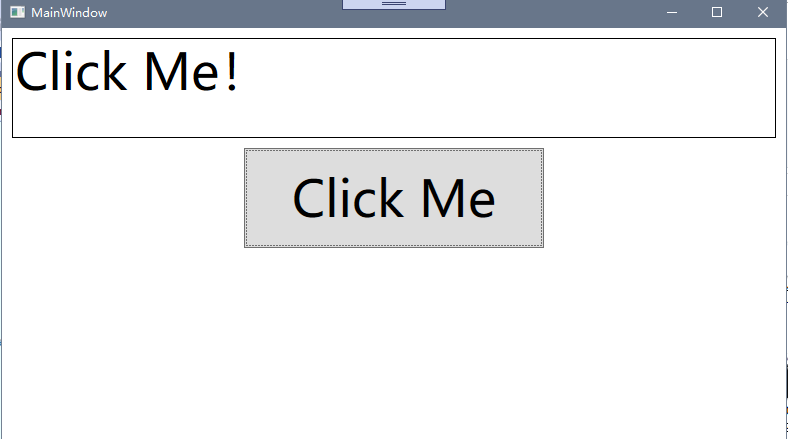
You can see, we have successfully used the prism data binding, and View and ViewModel perfect separation of the front and rear end
But now we also raises another question, when we do not want to follow the provisions of the prism will insist on the View and ViewModel and Views ViewModels inside, and perhaps your own project named rules vary from how to do, this time we should use In addition several ways:
1. Change the naming #
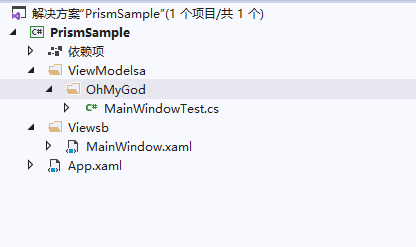
<prism:PrismApplication x:Class="PrismSample.App" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" xmlns:local="clr-namespace:PrismSample"> <Application.Resources> </Application.Resources> </prism:PrismApplication>
using Prism.Unity; using Prism.Ioc; using Prism.Mvvm; using System.Windows; using PrismSample.Viewsb; using System; using System.Reflection; namespace PrismSample { /// <summary> /// Interaction logic for App.xaml /// </summary> public partial class App : PrismApplication { //设置启动起始页 protected override Window CreateShell() { return Container.Resolve<MainWindow>(); } protected override void RegisterTypes(IContainerRegistry containerRegistry) { } //配置规则 protected override void ConfigureViewModelLocator() { base.ConfigureViewModelLocator(); ViewModelLocationProvider.SetDefaultViewTypeToViewModelTypeResolver((viewType) => { var viewName = viewType.FullName.Replace(".Viewsb.", ".ViewModelsa.OhMyGod."); var viewAssemblyName = viewType.GetTypeInfo().Assembly.FullName; var viewModelName = $"{viewName}Test, {viewAssemblyName}"; return Type.GetType(viewModelName); }); } } }
1
|
var
viewName = viewType.FullName.Replace(
".Viewsb."
,
".ViewModelsa.OhMyGod."
);
|
var viewModelName = $"{viewName}Test, {viewAssemblyName}";
2. Custom ViewModel registration #
using Prism.Commands; using Prism.Mvvm; namespace PrismSample { public class Foo:BindableBase { private string _text; public string Text { get { return _text; } set { SetProperty(ref _text, value); } } public Foo() { this.Text = "Foo"; } private DelegateCommand _clickCommnd; public DelegateCommand ClickCommnd => _clickCommnd ?? (_clickCommnd = new DelegateCommand(ExecuteClickCommnd)); void ExecuteClickCommnd() { this.Text = "Oh My God!"; } } }
protected override void ConfigureViewModelLocator() { base.ConfigureViewModelLocator(); ViewModelLocationProvider.Register<MainWindow, Foo>(); //ViewModelLocationProvider.SetDefaultViewTypeToViewModelTypeResolver((viewType) => //{ // var viewName = viewType.FullName.Replace(".Viewsb.", ".ViewModelsa.OhMyGod."); // var viewAssemblyName = viewType.GetTypeInfo().Assembly.FullName; // var viewModelName = $"{viewName}Test, {viewAssemblyName}"; // return Type.GetType(viewModelName); //}); }
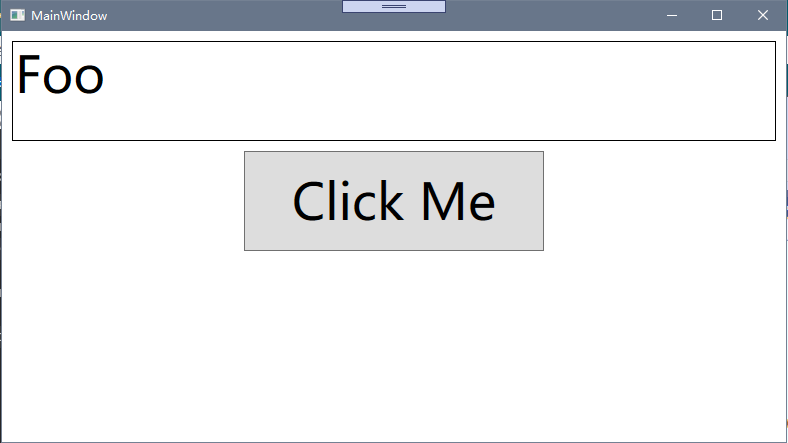
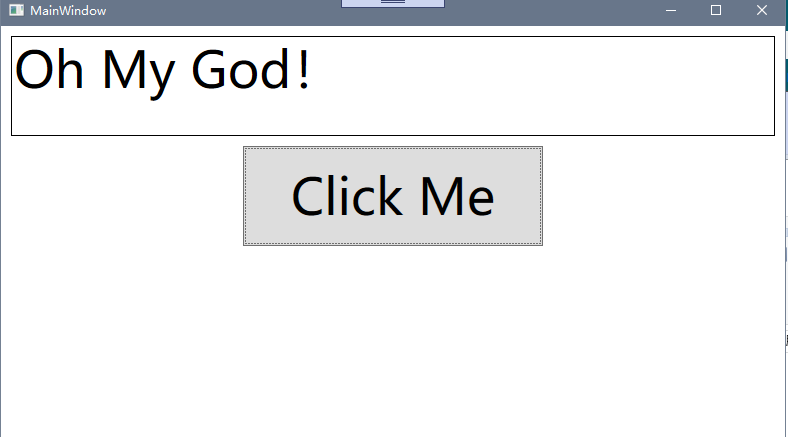
Even if the comment is not naming modify code, we find that the result is still the same run, so we can conclude,
This direct, not through reflection registered custom registration methods will be high priority in the official document also shows that in this way the efficiency will be high
And the official four ways, the other three are registered as follows:
ViewModelLocationProvider.Register(typeof(MainWindow).ToString(), typeof(MainWindowTest));
ViewModelLocationProvider.Register(typeof(MainWindow).ToString(), () => Container.Resolve<Foo>());
ViewModelLocationProvider.Register<MainWindow>(() => Container.Resolve<Foo>());