A, CountDownLatch
1.1 Overview
So that some thread to block until after the completion of others to be awakened.
The class has two main methods, when one or more thread calls await method, the calling thread will be blocked. Other thread invokes the method countDown counter is decremented by one (thread does not block when calling countDown method), when the value of the counter becomes zero, because invoked await blocked thread will be woken continue.
1.2 simulation scenarios
There are six students after class after leaving the classroom, but not the same time they leave, the squad leader needs to last one to leave and locked the classroom door.
1.3 simulation program
Before 1.3.1 CountDownLatch
. 1 public void closeDoor () { 2 . 3 for ( int I =. 1; I <=. 6; I ++) { . 4 new new the Thread (() -> { . 5 . System.out.println (Thread.currentThread () getName () + " leave the room "); . 6 }, String.valueOf (I)) Start ();. . 7 } . 8 . 9 . System.out.println (Thread.currentThread () getName () + " monitor lock leave the room "); 10 }
1.3.2 After use CountDownLatch
1 public void closeDoor() throws InterruptedException { 2 CountDownLatch countDownLatch = new CountDownLatch(6); 3 4 for (int i = 1; i <= 6; i++) { 5 new Thread(() -> { 6 System.out.println(Thread.currentThread().getName() + " 离开教室"); 7 countDownLatch.countDown(); 8 }, String.valueOf(i)).start(); 9 } 10 11 countDownLatch.await(); 12 System.out.println (Thread.currentThread () getName () + ". Monitor lock leave the room "); 13 }
1.4 CountDownLatch with the use of enumeration class
1 private static void nationalUnification() throws InterruptedException { 2 CountDownLatch countDownLatch = new CountDownLatch(6); 3 4 for (int i = 1; i <= 6; i++) { 5 new Thread(() -> { 6 System.out.println(Thread.currentThread().getName() + "国被灭..."); 7 countDownLatch.countDown(); 8 }, CountryEnum.forEachCountryEnum(i).getCountry()).start(); 9 } 10 11 CountDownLatch.await (); 12 System.out.println (Thread.currentThread () getName () +. " Qin off six countries, unified China "); 13 } 14 15 // enum class, the name of the country store 16 public enum CountryEnum { . 17 18 is ONE (. 1, " Qi "), . 19 TWO (2, " Chu "), 20 is THREE (. 3, " swallow "), 21 is the FOUR (. 4, " Zhao "), 22 is FIVE (. 5, " Wei "), 23 is the SIX (. 6, " Korea "); 24 25 Private Integer code; 26 private String country; 27 28 CountryEnum(Integer code, String country) { 29 this.code = code; 30 this.country = country; 31 } 32 33 public Integer getCode() { 34 return code; 35 } 36 37 public String getCountry() { 38 return country; 39 } 40 41 public static CountryEnum forEachCountryEnum(int index) { 42 CountryEnum[] countryEnums = CountryEnum.values(); 43 for (CountryEnum element : countryEnums) { 44 if (element.getCode() == index) { 45 return element; 46 } 47 } 48 return null; 49 } 50 } 51
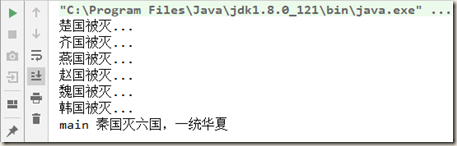
二、CyclicBarrier
2.1 Overview
Literally it means CyclicBarrier recyclable (Cyclic), barrier (Barrier) used. It needs to be done is to let a group of threads reach a barrier (also called synchronization point) is blocked when it until the last thread reaches the barrier, the barrier will open the door, all barriers blocked thread will continue to work, thread barriers to entry by await CyclicBarrier's () method.
2.2 Example
. 1 public void Meet () { 2 a CyclicBarrier CyclicBarrier = new new a CyclicBarrier (10, () -> { . 3 System.out.println ( " people together, to prepare a meeting "); . 4 }); . 5 . 6 for ( int I =. 1 ; I <= 10; I ++) { . 7 new new the Thread (() -> { . 8 System.out.println (. Thread.currentThread () getName () + " to room "); . 9 the try { 10 CyclicBarrier.await (); . 11 } the catch (InterruptedException | BrokenBarrierException e) { 12 e.printStackTrace(); 13 } 14 }, String.valueOf(i)).start(); 15 } 16 }
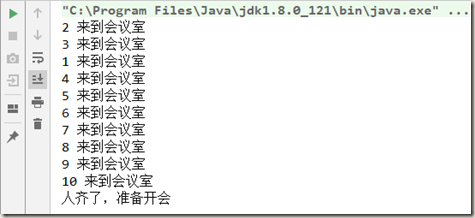
三、Semaphore
3.1 Overview
A counting semaphore. Conceptually, a semaphore maintains a set of permits. Each acquire() blocks if necessary until a permit is available, and then takes it. Each release() adds a permit, potentially releasing a blocking acquirer. However, no actual permit objects are used; the Semaphore just keeps a count of the number available and acts accordingly.
3.2 Example
1 public void semaphoreTest() { 2 Semaphore semaphore = new Semaphore(3); 3 4 for (int i = 1; i <= 6; i++) { 5 new Thread(() -> { 6 try { 7 semaphore.acquire(); 8 System.out.println(Thread.currentThread().getName() + " 抢到车位..."); 9 TimeUnit.SECONDS.sleep(3); 10 System.out.println(Thread.currentThread().getName() + "停车3秒,离开车位"); 11 } catch (InterruptedException e) { 12 e.printStackTrace(); 13 } finally { 14 semaphore.release(); 15 } 16 }, String.valueOf(i)).start(); 17 } 18 }
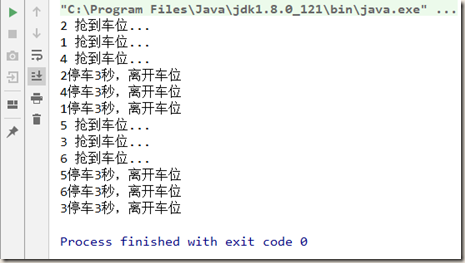