# Shortest path algorithm Dijkstra
# Input: A weighted directed graph G = (V, E), V = {1,2,3 ... n}
# Output: G 1 vertex to each vertex of the shortest distance
Dijkstra's algorithm weights each point changes:
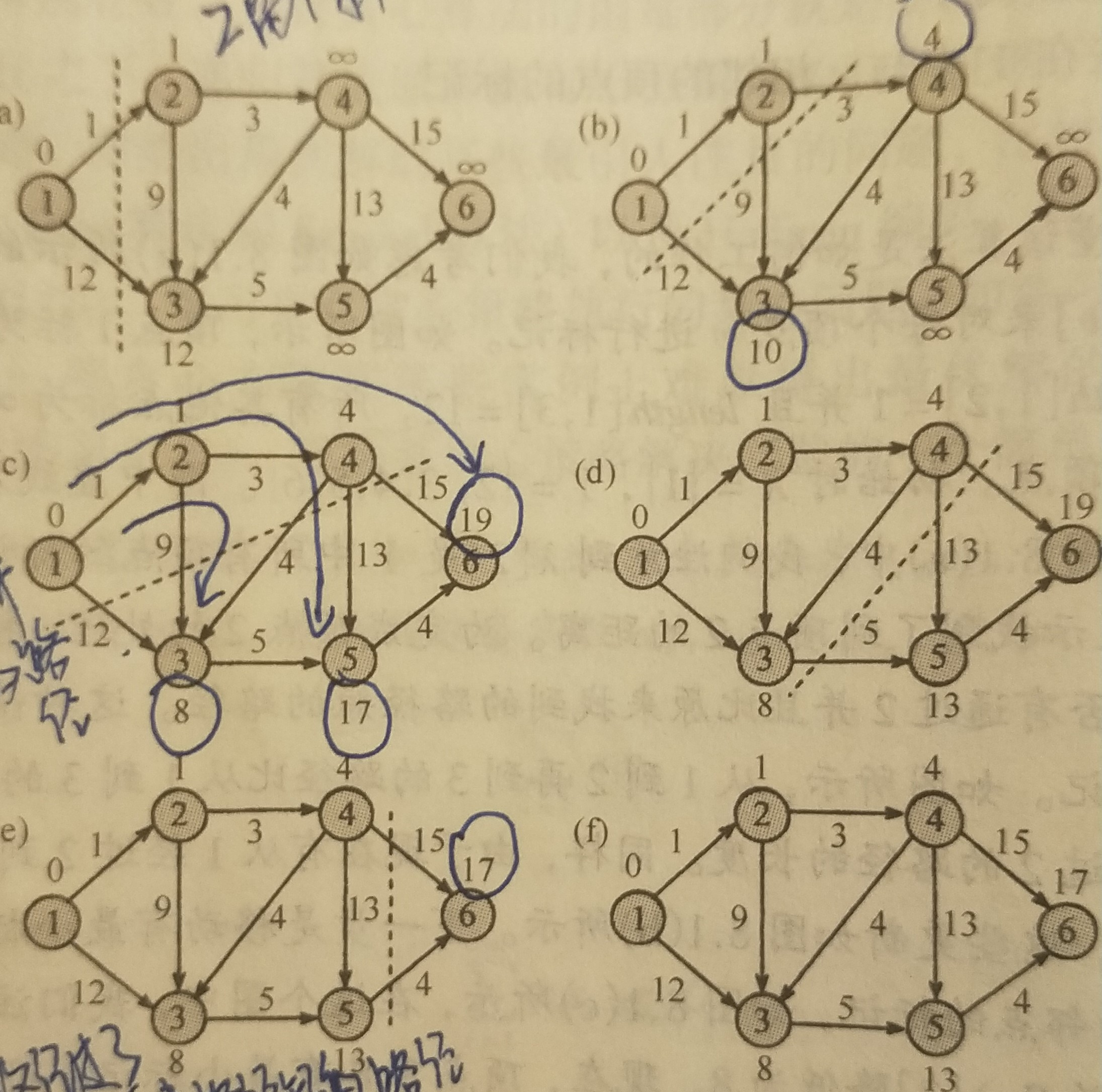
. 1 class Vertex: 2 # vertex class . 3 DEF the __init__ (Self, VID, OutList): . 4 self.vid VID = # an edge . 5 self.outList OutList = # a list of vertices directed edges id, the adjacency list can be understood as . 6 self.know = false # default is false . 7 self.dist = a float ( ' INF ' ) # S the distance from the point, the default is infinite . 8 self.prev 0 = # a vertex id, the default is 0 . 9 DEF __eq__ (Self, OTHER): 10 IF isinstance(other, self.__class__): 11 return self.vid == other.vid 12 else: 13 return False 14 def __hash__(self): 15 return hash(self.vid)
1 # create a vertex objects 2 V1 = Vertex (1, [2,3 ]) . 3 V2 = Vertex (2, [3,4- ]) . 4 V3 = Vertex (. 3, [. 5 ]) . 5 V4 = Vertex (. 4, [ 3,5,6 ]) . 6 V5 Vertex = (. 5, [. 6 ]) . 7 V6 = Vertex (. 6 , []) . 8 . 9 # weights stored edge 10 edges = dict () . 11 DEF add_edge member (Front, Back, value): 12 is Edges [(Front, Back)] = value 13 is add_edge member (1,2,1 ) 14 add_edge member (1,3,12 ) 15 add_edge member (2,3,9 ) 16 add_edge(2,4,3) 17 add_edge(3,5,5) 18 add_edge(4,3,4) 19 add_edge(4,5,13) 20 add_edge(4,6,15) 21 add_edge(5,6,4)
1 # Create an array of length 7 to store vertices 0 index element does not exist 2 Vlist = [False, V1, V2, V3, V4, V5, V6] . 3 # use set instead of the priority queue, select set primarily because there is a convenient method to remove set . 4 VSET set = ([V1, V2, V3, V4, V5, V6])
. 1 DEF get_unknown_min (): # This function is used instead of a priority queue dequeue 2 the_min = 0 . 3 the_index = 0 . 4 J = 0 . 5 for I in Range (. 1 , len (Vlist)): . 6 IF (Vlist [I] .know IS True): . 7 Continue . 8 the else : . 9 IF (J == 0): 10 the_min = Vlist [I] .dist . 11 the_index = I 12 is the else : 13 is IF(Vlist [I] .dist < the_min): 14 the_min = Vlist [I] .dist 15 the_index = I 16 J + =. 1 . 17 # time has been found that the smallest element of unknown who 18 is vset.remove (Vlist [ the_index]) # equivalent to the implementation team operating out of 19 return Vlist [the_index]
1 def main(): 2 #将v1设为顶点 3 v1.dist = 0 4 5 while(len(vset)!=0): 6 v = get_unknown_min() 7 print(v.vid,v.dist,v.outList) 8 v.know = True 9 for w in v.outList:#w为索引 10 if(vlist[w].know is True): 11 continue 12 if(vlist[w].dist == float('inf')): 13 Vlist [W] = v.dist .dist + Edges [(v.vid, W)] 14 Vlist [W] = .prev v.vid 15 the else : 16 IF ((+ v.dist Edges [(v.vid, W)]) < Vlist [W] .dist): . 17 Vlist [W] = v.dist .dist + Edges [(v.vid, W)] 18 is Vlist [W] = .prev v.vid . 19 the else : # the original path length is smaller, there is no need to update 20 Pass
Function call:
. 1 main () 2 Print ( ' v.dist is the shortest path length from the starting point to the point: ' ) . 3 Print ( ' v1.prev: ' , v1.prev, ' v1.dist ' , v1.dist) . 4 Print ( ' v2.prev: ' , v2.prev, ' v2.dist ' , v2.dist) . 5 Print ( ' v3.prev: ' , v3.prev, ' v3.dist ' , v3.dist) . 6 Print ( ' v4.prev: ' , v4.prev, 'v4.dist ' , v4.dist) 7 print ( ' v5.prev: ' , v5.prev, ' v5.dist ' , v5.dist) 8 print ( ' v6.prev: ' , v6.prev, ' v6. dist ' , v6.dist)
operation result:
0. 1 [2,. 3 ] 2. 1 [. 3,. 4 ] . 4. 4 [. 3,. 5,. 6 ] . 3. 8 [. 5 ] . 5 13 is [. 6 ] . 6. 17 [] v.dist is the shortest from the starting point to the point path length: v1.prev: 0 0 v1.dist v2.prev: . 1. 1 v2.dist v3.prev: . 4 v3.dist. 8 v4.prev: 2. 4 v4.dist v5.prev: . 3 v5.dist 13 is V6. PREV: . 5. 17 v6.dist