1: inline
Ordinary function is a direct function of the memory address to execute, but inline functions is to copy the main function inside the function, do not go to the specified location searched.
Function code execution time is very short, this time with inline call time is relatively long. But when the code is executed for a long time, will be transferred into memory cost function is greater than the time of the function call is not recommended at this time inline functions.
Inline function is generally higher efficiency than an ordinary function, the drawback is the large memory consumption.
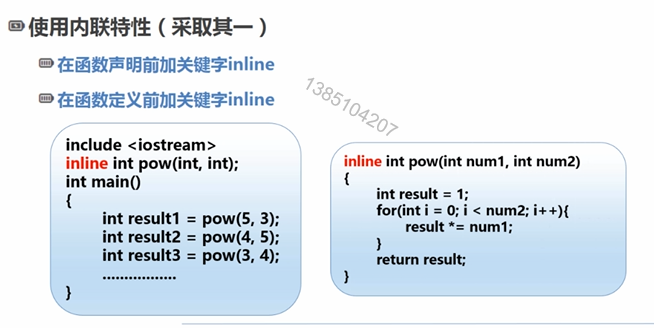
However, the general increase in the inline function definition
. 1 #include <bits / STDC ++ H.> 2 the using namespace STD; . 3 inline int S ( int NUM) . 4 { . 5 return NUM * NUM; . 6 } . 7 #define Sl (NUM) NUM * NUM // future use in all S (num) where * is automatically replaced NUM NUM . 8 int main () . 9 { 10 COUT << S ( . 5 ) << endl; // 25 . 11 COUT << S ( . 5 + 10 ) << endl; / / 225 12 << Sl COUT ( . 5 ) << endl; // 25 13 is COUT << Sl ( . 5 + 10 ) << endl; // 65 14 // Obviously, a final result of the error macro definition, his idea is calculated 5 * 10 + 5 + 10, therefore recommended c ++ inline, not recommended macros 15 return 0 ; 16 }
2: References
const int & refValue = 12 // constants can be followed
When using a reference habit plus const (unless you explicitly want to modify a reference variable), you can prevent arbitrary changes to the variable.
1 #include <bits/stdc++.h> 2 using namespace std; 3 void Swap1(int num1, int num2)//传参 4 { 5 int temp; 6 temp = num1; 7 num1 = num2; 8 num2 = temp; 9 } 10 void Swap2(int* num1, int* num2)//传指针 11 { 12 int temp; 13 temp = *num1; 14 * * = num1 num2; 15 * num2 = TEMP; 16 } . 17 void Swap3 ( int & num1, int & num2) // reference 18 is { . 19 int TEMP; 20 is TEMP = num1; 21 is num1 = num2; 22 is num2 = TEMP; 23 is } 24 int main () 25 { 26 is int num1, num2; 27 COUT << " two input parameters to be exchanged " <<endl; 28 cin >> >> num1 num2; 29 swap1 (num1, num2); // do not realize the exchange, because the pass into a copy of 30 cout << " mass participation as a result of the exchange: " << num1 << " \ T " << num2 << endl; 31 is Swap2 (& num1, & num2); // implemented exchange, because the address is inside the content is exchanged 32 COUT << " pass pointer exchange results: " << num1 << " \ T " << num2 << endl; 33 is Swap3 (num1, num2);// realized exchange reference variable is actually an alias for 34 cout << " reference exchange results:" << num1 << "\t" << num2 << endl; 35 return 0; 36 }
. 1 #include <bits / STDC ++ H.> 2 the using namespace STD; . 3 int & SUM () // return type is a reference type of the time, can not return a local variable . 4 { . 5 int NUM = 10 ; . 6 int & RNUM = NUM ; . 7 return RNUM; // can not return a local variable . 8 } . 9 void Test () 10 { . 11 int X = . 1 , Y = 2 , Z = . 3 ; 12 is } 13 is int main () 14 { 15 int & result = SUM (); // because rNum is a local variable, the function will be the end of the recovery system 16 Test (); // position result is referred to as recovered, it may apply to other variables, and modify the data occupancy . 17 COUT << " Result = " << endl << Result; // Result = 2, described spatial data is modified, and therefore can not return a local variable reference type 18 is return 0 ; . 19 }
The return type is a reference type can only return to the incoming reference
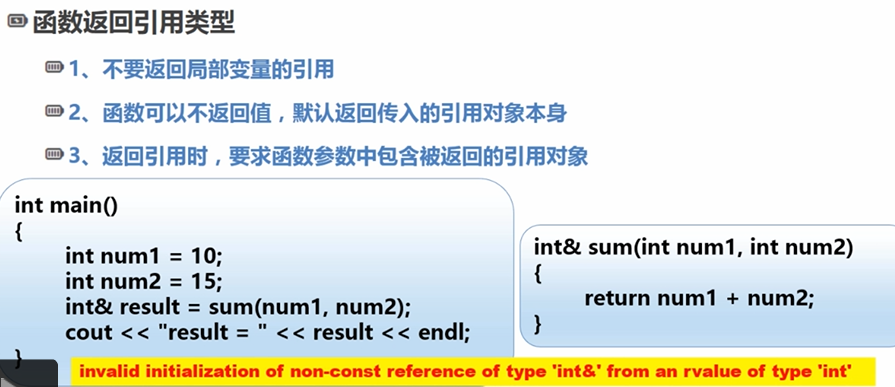
The code can only return a return value, the return value is a cut change parameter passed, or to that num1 num2.
And the above code because the result indicated num same space, the result is modified, num also modified, so as to prevent inadvertent modification reference is generally plus const.
The basic type of the array passed by reference discourages the use of ah, ah structure objects often used references
3: The default parameters
1 #include <bits/stdc++.h> 2 using namespace std; 3 void test(int x = 10)//默认值是10 4 { 5 cout << x << endl; 6 } 7 void test1(int x, int y = 10)//从右到左按顺序声明 8 { 9 10 } 11 void test2(int x = 10, int y){//必须先默认右边的 12 13 } 14 int main() 15 { 16 test();//不写参数默认传10 17 test(100);//指明了参数 18 19 return 0; 20 }
4: 模版
1 #include <bits/stdc++.h> 2 using namespace std; 3 template<typename T> 4 void Swap(T& a,T& b)//可以传入任何类型,系统根绝传入的数据判别类型,大大提高代码的通用性 5 { 6 T temp; 7 temp = a; 8 a = b; 9 b = temp; 10 } 11 int main() 12 { 13 cout << "请输入两个整数:" <<endl; 14 int a,b; 15 cin >> a >> b; 16 Swap(a, b); 17 cout << "交换的结果为: "; 18 cout << a << "\t"<< b << endl; 19 cout << "请输入两个字符串:" <<endl; 20 string a1, b1; 21 cin >> a1 >> b1; 22 Swap(a1, b1); 23 cout << "交换的结果为: "; 24 cout <<a1 << "\t" << b1; 25 return 0; 26 }
5:函数重载
1 #include <bits/stdc++.h> 2 using namespace std; 3 void test(int x)//默认值是10 4 { 5 cout << x << endl; 6 } 7 void test() 8 { 9 cout << "test()" << endl; 10 } 11 int main() 12 { 13 //名字相同,参数列表不同,所以认为重载 14 test();//不带参数的 15 test(100);//带参数的, 16 17 return 0; 18 }