First, the definition of the function
The code is a function package. The implementation code is a function of the same, are encapsulated together. The next time you need to use, then you do not need to write code, you can directly call.
Built-in function is relatively large demand for some functions the same footprint, easy call.
Benefits: increase code reusability and increase code readability, reduce the amount of code written, and reduce maintenance costs.
Naming the function name follows the hump rule, it is best to borrow the name of justice.
Definition methods:
DEF function_name (params): #def keyword indicates that the definition of a function. function_name function names, variable names and the same naming requirements, begin with the letter and _, may contain letters, numbers and _. represents a parameter params, may be zero, one or more parameters, the parameters do not specify the type of function parameters. Block return expression The (expression) / value Examples: DEF DieDai (ITER): # function called DieDai, parameters ITER for ITER in I: # This is a removed inside iterable cycle value print (i)
output :
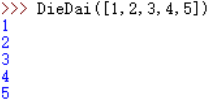
Second, the parameters of the form
1, do not pass parameters
def fun1 (): # function definition because when there is no input parameter, this function can not pass parameters, this function can be run in the absence of transmission parameters print ( 'not-parameters')
output:
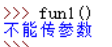
2, essential parameters
def fun2 (a): # function definition when the input parameters, the parameters must be passed when calling the print ( 'must pass parameters:', a)
output:
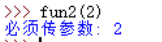
3, the default parameters
def fun3 (b = 1): # can pass parameters, may not pass parameters, output the default value does not pass the reference, the output transmission reference value parameter passing, can be directly input during mass participation 4, you may specify the input b = 4 print ( 'default parameters', b)
output:
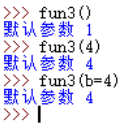
4, optional parameter (variable length parameter)
def fun4 (* arg): # 0 can be transmitted to a plurality of parameters; tuple space output parameter does not pass; * is required, arg customizable; packaging parameters into tuples; when the driving parameter passing asterisk can be unpacked (the inside of the casing removed), {*} wherein the output key, without the number * {element} as a print ( 'optional parameters to be passed more than 0:', arg)
output :
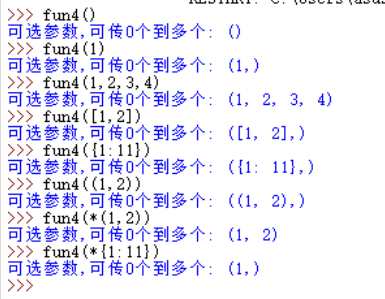
5, keyword arguments
def fun5 (a, b): # define when necessary with the same parameters, keyword parameters is actually a mandatory parameter, mixing time must pass the Senate to put the final Print (A, b)
DEF FUN6 (* * kwarg): # 0 can be transmitted to the plurality, the output does not pass an empty dictionary; follow when defined variable naming rules; ** is required, kwarg is conventional, can be customized; packaged into the dictionary parameter passing; ** {} which must be a string form of keyword print ( 'keyword parameter', kwarg) keyword parameters are two, one above no clear boundaries, when the parameter passing can be used in the form of number = to above per se are required parameters.
Output:
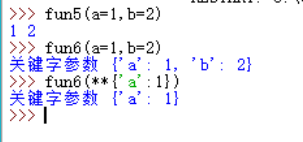
6, how to pass parameters when mixing parameters
参数混合的时候,关键字参数必须放最后面 默认参数加必备参数 def fun7(a,b=2): #默认参数必须在必备参数的后面 print(a,b)
调用的时候: fun7(1,b=2) fun7(a=1,b=2) fun7(1,2) #默认参数也可以不指定 fun7(a=1,2) #会报错,用为关键字参数必须放最后面 fun7(2,a=1) #会报错,在传参时会根据定义时的顺序来传参,确保必备参数能够拿到值,并且只能有一个
输出结果:
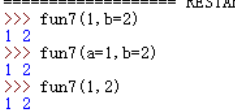
def fun8(b,m=1,*a):
print(b) print(m) print(a) 错误输入情况: fun8(1,m=1,1,2,3,4) #错误原因:因为关键字参数m=1必须放最后 fun8(1,1,2,3,4,m=1) #错误原因:因为根据定义顺序,先是第二位定义了一个m=1,最后面又定义了一个m=1,重复定义 正确输入: fun(1,2,3,4,5)
输出结果:
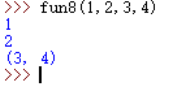
def fun9(*a,b,m):
print(a,b,m)
正确输入:
fun9(1,2,3,b=1,m=2)
输出结果:
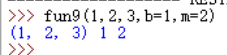
def fun11(a,b=1,*arg,**kwarg): print('这是必备参数:',a) print('这是默认参数:',b) print('这是可选参数(不定长参数)',arg) print('这是关键词参数',kwarg) return 'OK' 正确输入:fun11(1,2,1,2,3,x=1,y=2,z=3)
输出结果:
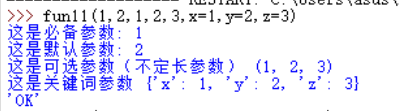
或者 def fun11(a,*arg,b=1,**kwarg): print('这是必备参数:',a) print('这是默认参数:',b) print('这是可选参数(不定长参数)',arg) print('这是关键词参数',kwarg) return 'OK' 正确输入:fun11(1,1,2,3,b=3,x=1,y=2,z=3) #b的位置可以在字典的前后
输出结果:
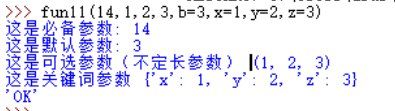
三、return
return的作用:
1、返回函数的运行结果
def fun1(a,b): if a>b: return a def fun2(a,b): if a>b: print(a)
fun1和fun2的区别是若将a=fun1(2,1),a=2;b=fun2(2,1),b=None
return返回了fun1的运行结果
运行结果:
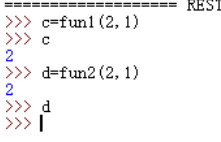
2、函数结束的标志
def fun10(a,b): return('我下不去了') #终止函数的标志,可以放在任何地方 if a>b: print(a)
输出结果:
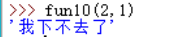
四、lambda 匿名函数
fun()是一个函数,fun就是一个函数体
lambda函数就是没有函数名的函数
g=lambda x:x+1 等同于: def g(x): return(x+1)
lambda简化了函数定义的书写形式。是代码更为简洁,但是使用函数的定义方式更为直观,易理解
应用场景:
1.有些函数如果只是临时一用,而且它的业务逻辑也很简单时,就没必要用def 来定义,这个时候就可以用lambda。
2.函数都支持函数作为参数,lambda 函数就可以应用
四、作业
1、找到1-100内的质数,用while和for来做,结束之后打印“搜索结束”。(质数:只能被1和自己整除,如2,只有除以1和2才能整除)
思路:先判断一个数字是不是质数,比如15,将15除以2到14,若存在能够整除无余,就说明这是一个合数,否则就是一个质数
j=15 #先确定一个数字j for i in range(2,15): #确定被除的数字也就是(2-14) if j%i==0: #遍历j除以i,若存在整除现象,说明是个合数 print(j,'是一个合数') break else: #否则,就是一个质数 print(j,'是一个质数')
输出结果:
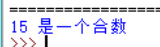
思路:然后再将1-100的数放入j即可
j=1 while j<101: #外面是一个将1-100的数字填入j的while循环 for i in range(2,j): #里面是一个检验每个数字是否是质数的for循环 if j%i==0: break else: print(j,end=' ') j+=1 else: print() print('搜索结束') 输出结果:

或者 num=[] #也可以建立个列表 for j in range(1,101): for i in range(2,j): if j%i==0: break else: num.append(j) #将每一个符合质数的数字填入列表num else: print(num) #最后输出列表 print('搜索结束')
输出结果:

3、定义一个函数,能够输入元组和字典。将字典的值(value)和元组的值交换,交换结束后返回字典和元组。
思路:输入元组和字典:要输入元组,说明要*arg;要输入字典,说明要**kwarg。def change(*arg,**kwarg)
思路:交换字典的值和元组的值:先定义一个元组tu=(1,2,3),一个字典di=('a':11,'b':22,'c':33),要将123和11,12,13进行交换,要交换必须要将元组改变为可变(列表):tu=list(tu)。在传参的时候应该输入change(*tu,**di),因为如果直接输入tu的话会把里面的元素直接定义为1个元素出现((1,2,3,),)的现象。若要交换可以直接用tu[0],di['a']=di['a'],tu[0]。
def change(*arg,**kwarg): arg=list(arg) #将元组arg转化列表 i=0 #为列表第一个元素定义索引 for key in kwarg.keys(): #遍历字典所有的键 arg[i],kwarg[key]=kwarg[key],arg[i] #交换字典kwarg和列表arg的值 i+=1 #让列表跟着字典的键做循环,字典键+1,列表索引也加1 else: print(kwarg) #输出更改后的字典 print(tuple(arg)) #输出更改后的元组,不过要将列表元组化
输出结果:
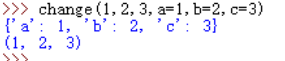
同时,由于列表arg是跟着字典kwarg的键做循环的,所以元组比字典多一个只没关系,反之则不行。