Problem Description
The n schools participating in the sports meeting are numbered 1~n. The competition is divided into m men's events and w women's events, and the event numbers are 1~m and m+1~m+w respectively. Due to the large difference in the number of participants in each project, some projects only take the top five, and the scoring order is 7, 5, 3, 2, 1; some projects only take the top three, and the scoring order is 5, 3, 2. Write a statistical program to generate various transcripts and score reports.
basic requirements
Generate transcripts for each school, including the item number, ranking (score), name, and score of each achievement obtained by each school; generate a team total score report, including the school number, total score of the men's team, and total score of the women's team. and total team score.
Test Data
For n=4, m=3, w=2, the top five items with odd numbers are selected, and the top three items with even numbers are selected. Design a set of example data.
Implementation tips
It can be assumed that n<=20, m<=30, w<=20, and the name length does not exceed 20 characters. At the end of each event, enter its number and type (differentiate between the top five or the top three), and enter the athlete's name, school name (and results) in order of ranking.
Selected content
Allows users to specify other ranking methods for a certain project.
The sports meeting score statistics system menu is as follows:
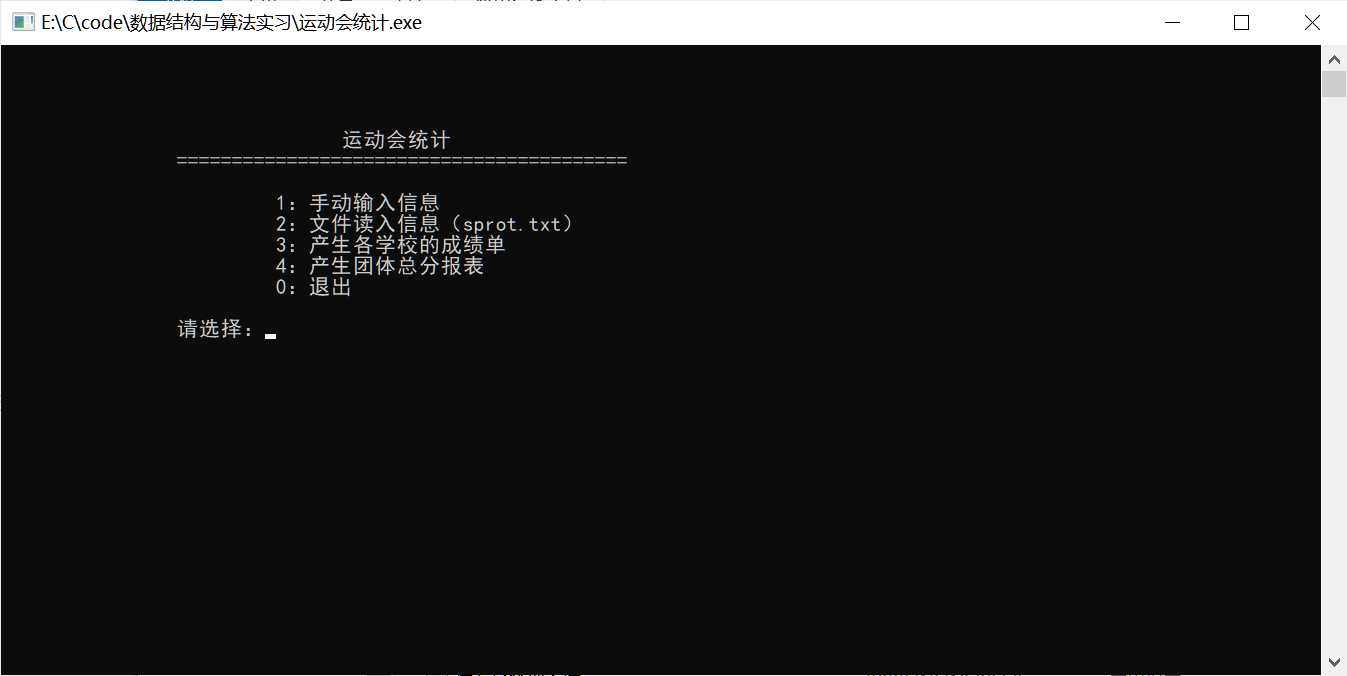
Tips:
Enter nmw as the number of schools, the number of men's/women's events, then enter the name of each school, and then enter the event name, athlete name, school name and results in order.
Can support using file input instead of manual to facilitate testing
It can record the scores of all students in each school within a certain range. Although the student's score is 0, his scores can be recorded and output.
Target file name: sport.txt (It is recommended to read from the file first to prevent the program from crashing and re-entering the data)
Some personal thoughts:
Personally, I think this question is quite simple. I just thought about how to input the data for a long time. I originally wanted to classify and collect statistics by school, but there are many problems in operation and data storage, so I still ranked according to the requirements of the question. Sequential input, final data storage problem, and then traversal output.
The complete code is as follows:
#include <iostream>
#include <iomanip>
#include <conio.h>
#include <stdio.h>
#include <process.h>
#include <string.h>
#include <math.h>
#include <stdlib.h>
using namespace std;
#define max 41
typedef struct
{
char name[10];//记录得奖人姓名
char school[max]; //记录得奖人学校号
int score; //记录得奖人的成绩
int mark; //得分
int ranking; //排名
}DataType;
typedef struct
{
char item[max]; //项目编号(名称)
int id,type; //编号、类型符
int sex; //加一个标识表示男,女
int length; //参加人数
DataType data[max]; //记录前几名的信息
char name[max]; //记录项目名称
}Project; //记录项目的信息
int n,m,w;//参数
char school[max][max];//各个学校名称
Project project[max];//记录各个项目数据
//产生各学校的成绩单并输出(输入参数为学校编号-无)
void makeGrade();
//产生团体总分报表(输入参数为学校个数-无)
void makeReport();
//公用的等待函数
void wait();
//数据输入
void input();
//根据排名返回得分
int Score(int type,int ranking);
//
void txtRead();
int main()
{
char choice;
while (1)
{
system("cls");
cout << "\n\n\n\n";
cout << "\t\t 运动会统计 \n";
cout << "\t\t=========================================";
cout << "\n\n";
cout << "\t\t 1:手动输入信息 \n";
cout << "\t\t 2:文件读入信息(sprot.txt) \n";
cout << "\t\t 3:产生各学校的成绩单 \n";
cout << "\t\t 4:产生团体总分报表 \n";
cout << "\t\t 0:退出 \n";
cout << "\n";
cout << "\t\t请选择:" << flush;
choice = getch();
system("cls");
switch(choice)
{
case '1':
input();
wait();
break;
case '2':
txtRead();
wait();
break;
case '3':
makeGrade();
wait();
break;
case '4':
makeReport();
wait();
break;
case '0':
exit(0);
}
}
return 0;
}
//公用的等待函数
void wait()
{
cout << "\n\n请按任意键继续" << flush;
getch();
}
//数据输入
void input()
{
int i,j,k,score;
cout<<"请输入参与学校数目n、男子项目数量m、女子项目数量w:";
scanf("%d %d %d",&n,&m,&w);
cout<<"请按顺序输入学校名称"<<endl;
for(i=1;i<=n;i++)
{
cout<<"请输入第"<<i<<"个学校名称:";
cin>>school[i];
}
cout<<"男:"<<endl;
for(j=1;j<=m;j++)
{
cout<<"第"<<j<<"个项目(默认编号奇数-前三 偶数-前五) 请输入名称/编号:";
cin>>project[j].name;
project->id=j;
cout<<"按名次顺序输入运动员姓名、学校名和成绩(结束输入:0 0 0)"<<endl;
if(j%2==0)
project[j].type=5;
else
project[j].type=3;
k=0;
do
{
k++;
cout<<"第"<<k<<"名:";
project[j].data[k].ranking=k;
cin>>project[j].data[k].name>>project[j].data[k].school>>project[j].data[k].score;
project[j].data[k].mark=Score(project[j].type,project[j].data[k].ranking);
}
while(strcmp(project[j].data[k].name,"0")!=0);
project[j].length=k-1;
}
cout<<"女:"<<endl;
for(j=m+1;j<=m+w;j++)
{
cout<<"第"<<j<<"个项目(默认编号奇数-前三 偶数-前五) 请输入名称/编号:";
cin>>project[j].name;
project->id=j;
cout<<"按名次顺序输入运动员姓名、学校名和成绩(结束输入:0 0 0)"<<endl;
if(j%2==0)
project[j].type=5;
else
project[j].type=3;
k=0;
do
{
k++;
cout<<"第"<<k<<"名:";
project[j].data[k].ranking=k;
cin>>project[j].data[k].name>>project[j].data[k].school>>project[j].data[k].score;
project[j].data[k].mark=Score(project[j].type,project[j].data[k].ranking);
}
while(strcmp(project[j].data[k].name,"0")!=0);
project[j].length=k-1;
}
}
//产生各学校的成绩单并输出(输入参数为学校编号-无)
void makeGrade()
{
int i,j,k,score,flag;
for(i=1;i<=n;i++)
{
printf("\n\t\t\t%-20s \n\t男子项目\n",school[i]);
for(j=1;j<=m;j++)
{
printf("项目\t \t姓名 成绩\t排名\t得分\n");
for(k=1;k<=project[j].length;k++)
{
if(strcmp(project[j].data[k].school,school[i])==0)
printf("%-16s%-15s%-9d%-8d%-12d\n",project[j].name,project[j].data[k].name,project[j].data[k].score,project[j].data[k].ranking,project[j].data[k].mark);
}
}
printf("\n\t女子项目\n");
for(j=m+1;j<=m+w;j++)
{
printf("项目\t \t姓名 成绩\t排名\t得分\n");
for(k=1;k<=project[j].length;k++)
{
if(strcmp(project[j].data[k].school,school[i])==0)
printf("%-16s%-15s%-9d%-8d%-12d\n",project[j].name,project[j].data[k].name,project[j].data[k].score,project[j].data[k].ranking,project[j].data[k].mark);
}
}
}
}
//产生团体总分报表(输入参数为学校个数-无)
void makeReport()
{
int score,total=0,score_m=0,score_w=0,i,j,k;
printf("\t\t\t团队得分\n学校\t\t男生得分\t女生得分\t总分\n");
for(i=1;i<=n;i++)
{
for(j=1;j<=m;j++)
{
for(k=1;k<=project[j].length;k++)
if(strcmp(project[j].data[k].school,school[i])==0)
score_m+=project[j].data[k].mark;
}
for(j=m+1;j<=m+w;j++)
{
for(k=1;k<=project[j].length;k++)
if(strcmp(project[j].data[k].school,school[i])==0)
score_w+=project[j].data[k].mark;
}
total=score_m+score_w;
printf("%-16s%-16d%-16d%-16d\n",school[i],score_m,score_w,total);
total=score_m=score_w=0;
}
}
void txtRead()
{
FILE *fp=NULL;
fp=fopen("sport.txt", "r");
int i,j,k,score;
fscanf(fp,"%d %d %d\n",&n,&m,&w);
printf("n:%d m:%d w:%d\n",n,m,w);
for(i=1;i<=n;i++)
fscanf(fp,"%s",school[i]);
fscanf(fp,"\n");
for(j=1;j<=m;j++)
{
fscanf(fp,"%s\n",project[j].name);
printf("%s(男)\n",project[j].name);
project->id=j;
if(j%2==0)
project[j].type=5;
else
project[j].type=3;
k=0;
do
{
k++;
project[j].data[k].ranking=k;
fscanf(fp,"%s %s %d\n",project[j].data[k].name,project[j].data[k].school,&project[j].data[k].score);
printf("第%d名:%s %s %d\n",k,project[j].data[k].name,project[j].data[k].school,project[j].data[k].score);
project[j].data[k].mark=Score(project[j].type,project[j].data[k].ranking);
}
while(strcmp(project[j].data[k].name,"0")!=0);
project[j].length=k-1;
}
for(j=m+1;j<=m+w;j++)
{
fscanf(fp,"%s\n",project[j].name);
printf("%s(女)\n",project[j].name);
project->id=j;
if(j%2==0)
project[j].type=5;
else
project[j].type=3;
k=0;
do
{
k++;
project[j].data[k].ranking=k;
fscanf(fp,"%s %s %d\n",project[j].data[k].name,project[j].data[k].school,&project[j].data[k].score);
printf("第%d名:%s %s %d\n",k,project[j].data[k].name,project[j].data[k].school,project[j].data[k].score);
project[j].data[k].mark=Score(project[j].type,project[j].data[k].ranking);
}
while(strcmp(project[j].data[k].name,"0")!=0);
project[j].length=k-1;
}
fclose(fp);
printf("\n读取成功!\n");
}
//根据排名返回得分
int Score(int type,int ranking)
{
if(type==3)
{
switch(ranking)
{
case 1:
return 5;
case 2:
return 3;
case 3:
return 2;
default:
return 0;
}
}
else
{
switch(ranking)
{
case 1:
return 7;
case 2:
return 5;
case 3:
return 3;
case 4:
return 2;
case 5:
return 1;
default:
return 0;
break;
}
}
return 0;
}