I. Introduction
In C++, you can directly create UIs such as buttons and scroll wheels, and you can directly bind and handle response events. After creating C++ code, you still need to use blueprints to display it in the application. Generally speaking, it is not as convenient as using blueprints directly.
The Unreal Engine used in this article is 5.2.1.
2. Realization
2.1. Create a C++ class of type UUserWidgetl
Declare two buttons and create click event processing methods for the two buttons. The header file code is as follows. This only declares the two buttons but does not actually create the buttons. They need to be bound in the subsequent blueprint UI.
#pragma once
#include "CoreMinimal.h"
#include "Components/Button.h"
#include "Blueprint/UserWidget.h"
#include "MyUserWidget.generated.h"
/**
*
*/
UCLASS()
class CHAPTER2_API UMyUserWidget : public UUserWidget
{
GENERATED_BODY()
public:
//这里设定的“buttonStart"按钮名称后面创建蓝图类UI中按钮的名称和这个一样
UPROPERTY(meta = (BindWidget))
UButton* ButtonStart;
UPROPERTY(meta = (BindWidget))
UButton* ButtonQuit;
public:
virtual bool Initialize()override;
public:
UFUNCTION()
void BtnClick_Start();
UFUNCTION()
void BtnClick_Quit();
};
Note: When two buttons are declared in the code, they must be reflected in the subsequent blueprint class, including the names, otherwise a compilation error will occur, and the editor may even crash directly.
Bind the click of the button in the initialization function, and write the information of the button click event processing function. The code is as follows:
bool UMyUserWidget::Initialize()
{
if (!Super::Initialize())
{
return false;
}
ButtonStart->OnClicked.AddDynamic(this, &UMyUserWidget::BtnClick_Start);
ButtonQuit->OnClicked.AddDynamic(this, &UMyUserWidget::BtnClick_Quit);
return true;
}
void UMyUserWidget::BtnClick_Start()
{
GEngine->AddOnScreenDebugMessage(-1, 5.0f, FColor::Green, TEXT("Button Start"));
}
void UMyUserWidget::BtnClick_Quit()
{
GEngine->AddOnScreenDebugMessage(-1, 5.0f, FColor::Red, TEXT("Button Quit"));
}
2.2. Create UI blueprint
This step is different from the previous direct creation of a C++ blueprint class. As shown in Figure 2.2.1, if you click to create a blueprint class of this class, only a blueprint class will be created, which has no real UI elements.
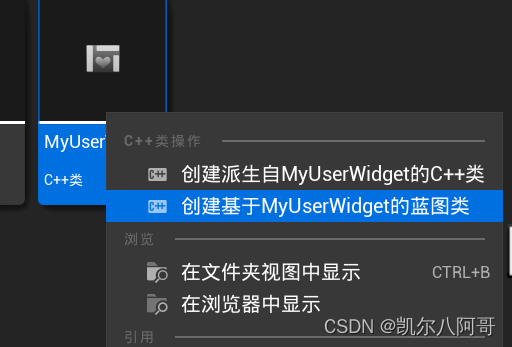
What we need is to create a separate control blueprint, and then select the class created in 2.1 when setting its parent class.
When selecting the parent class, you need to first create two classes in 2.1, and the names must be the same, as shown in Figure 2.2.2. You can create multiple buttons or other controls, but these two types must exist and the names must be the same. , otherwise it will directly lead to editing when binding the parent class
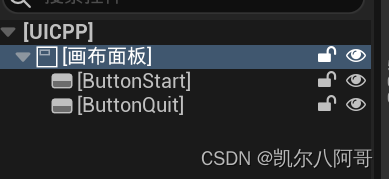
The editor crashes. As shown in Figure 2.2.3, setting the parent class without the "ButtonStart" button directly causes the editor to crash.
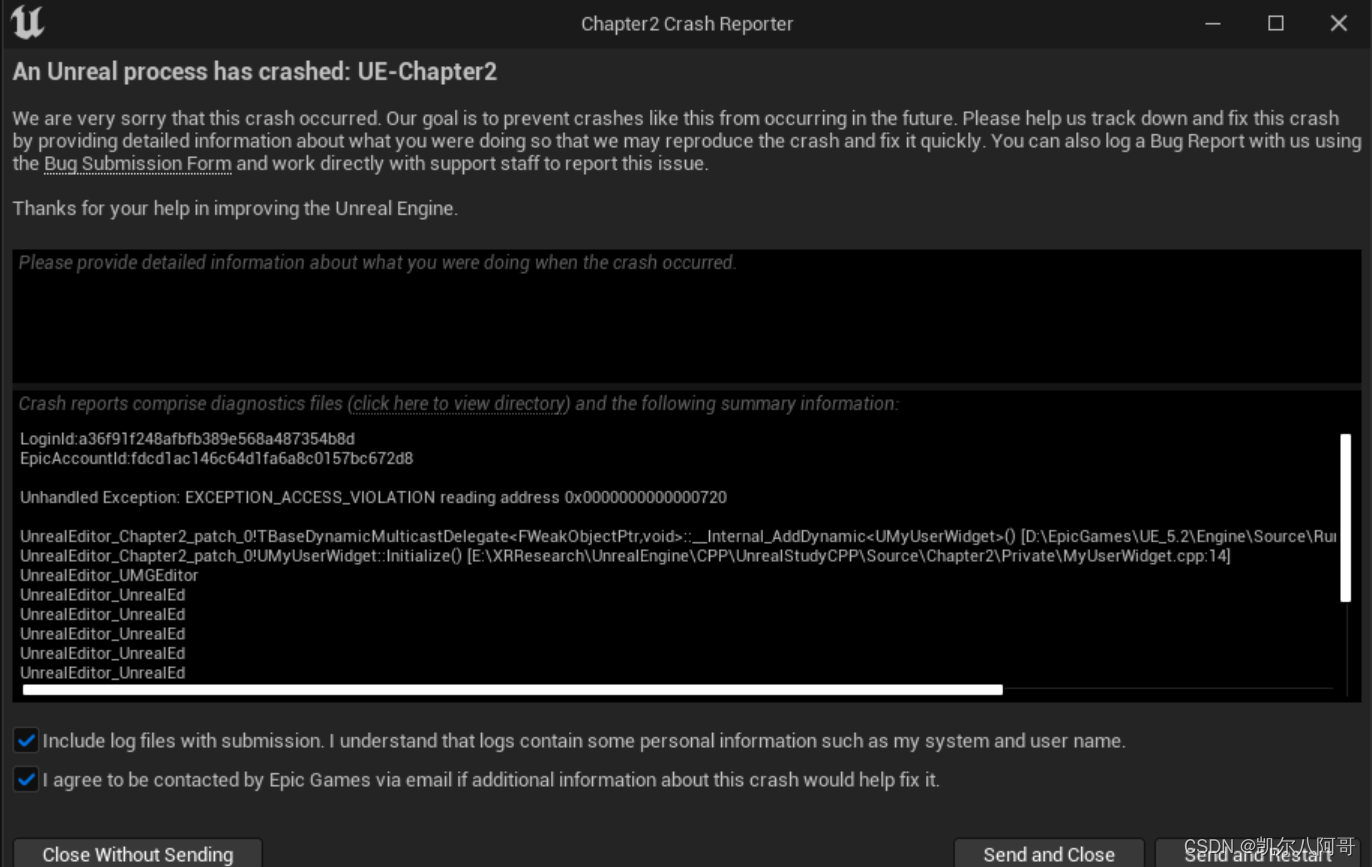
The crash message also indicates that there is no such button in the C++ code, and then it is bound. When you open the editor again and try to open the blueprint, you will be prompted that it cannot be opened, or the parent class of the blueprint will return to the default "User Control" after opening. And when selecting in the parent class, the class created before 2.1 can no longer be found, and this class is no longer in the C++ class file. The C++ code needs to be recompiled before it appears again.
2.3. Add to the scene
This step is the same as the logic of creating UI in the blueprint and adding it to the viewport. You need to create the blueprint class created in 2.2 in PlayController or other system management classes and add it to the viewport. The code is as follows, the created TEXT path That is the blueprint in 2.2
#include "Blueprint/UserWidget.h"//需要添加的头文件
void AMyPlayerController1::BeginPlay()
{
Super::BeginPlay();
//这里的参数NULL和表示只是加载到场景中,不会加载到这个类下面
UClass* WidgetCalss = LoadClass<UUserWidget>(NULL, TEXT("/Script/UMGEditor.WidgetBlueprint'/Game/BluePrint/UMG_Widget.UMG_Widget_C'"));
UUserWidget* MyWidgetClass = nullptr;
MyWidgetClass = CreateWidget<UUserWidget>(GetWorld(), WidgetCalss);
MyWidgetClass->AddToViewport();
}
The path after the "copy reference" of the class needs to be added with a "_C" suffix.
3. Summary
3.1. The button variable name created in C++ must be created in the subsequent blueprint control, and the type and name must be consistent. Otherwise, a compilation error will be reported, or the editor may even crash.