The employee table is as follows (will be used in the following demonstration operations):
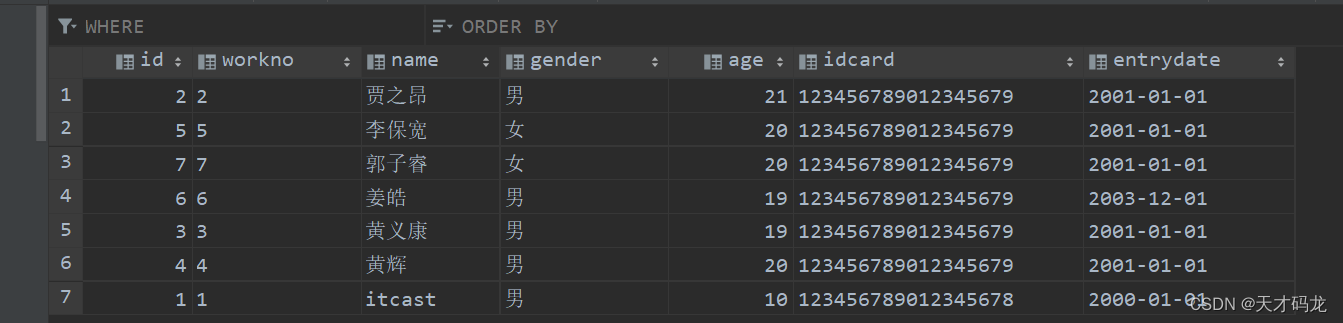
1 String functions
(1) Function implementation
function |
Function |
concat(s1,s2,…,sn) |
String concatenation, concatenate s1, s2...sn into a string |
lower(str) |
Convert all string str to lowercase |
Upper(str) |
Convert all string str to uppercase |
lpad(str,n,pad) |
Left padding, use string pad to pad the left side of str to reach n string lengths |
rpad(str,n,pad) |
Right padding, fill the right side of str with string pad to reach n string length |
trim(str) |
Remove leading and trailing spaces from string |
substring(str,start,len) |
Returns a string of len lengths from the start position of the string str. |
select concat('Hello', ' MySQL!');
select lower('Hello');
select upper('Hello');
select lpad('01',6,'X');
select rpad('01',6,'X');
select trim(' Hello MySQL! ');
select substring(' Hello MySQL! ',1,13);
(2) Realization of business needs
update employee set workno = lpad(workno,6,'0');
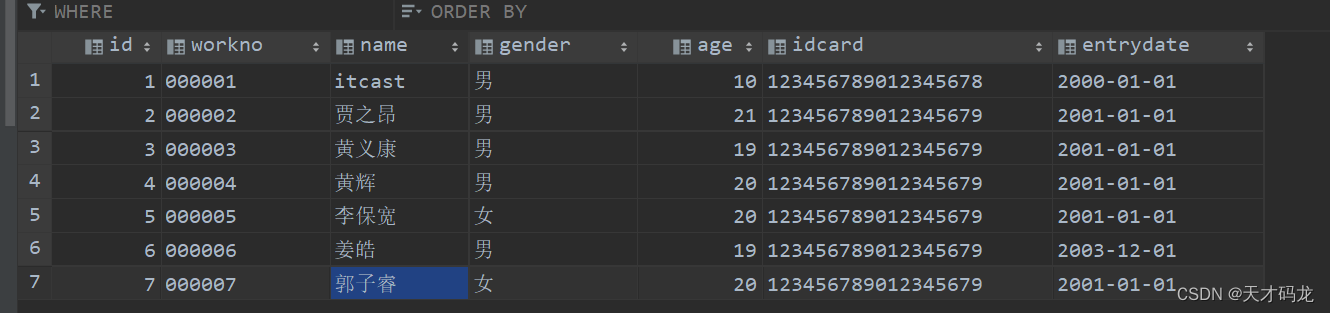
2 Numerical functions
Function implementation
function |
Function |
ceil(x) |
Rounded up |
floor(x) |
Round down |
mod(x,y) |
Returns the modulus of x/y |
rand() |
Returns a random number between 0 and 1 |
round(x,y) |
Find the rounded value of parameter x, retaining y decimal places |
select ceil(1.1);
select floor(1.1);
select mod(6,5);
select rand();
select round(6.6);
select round(6.3);
select round(6.365,2);
select lpad(round(rand()*1000000),6,'0');
3 date functions
(1) Function implementation
function |
Function |
curdate() |
Return current date |
curtime() |
Return current time |
now() |
Returns the current date and time |
year() |
Get the year of the specified date |
month() |
Get the month of the specified date |
day() |
Get the date of the specified date |
date_add(date,interval exprtype) |
Returns a date/time value plus a time value expr |
datediff(date1,date2) |
Returns the number of days between the start time date1 and the end time date2 |
select curdate();
select curtime();
select now();
select year(now());
select month(now());
select day(now());
select date_add(now(),interval 70 year);
select datediff('2022-12-01','2021-12-11');
select datediff('2023-3-26','2022-10-16');
(2) Realization of business needs
select name,datediff(curdate(),entrydate) as 'entrydays' from employee order by entrydays desc;
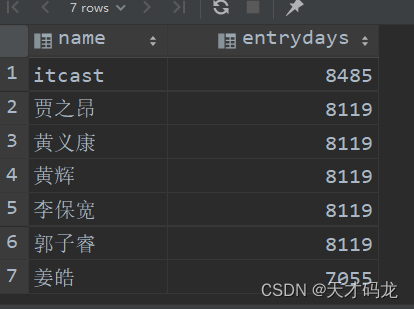
4 process function
(1) Function implementation
function |
Function |
if(value,t,f) |
If value is true, return t, otherwise return f |
ifnull(value1,value2) |
If value1 is not empty, return value1, otherwise return value2 |
case when [val1] then [res1]…else [fedault] end |
If val1 is true, return res1,...otherwise return default value |
case [expr] when [val1] then [res1] … else [default] end |
If the value of expr is equal to val1, return res1,...otherwise return the default value |
select if(false,'OK','ERROR');
select ifnull('OK','Default');
select ifnull('','Default');
select ifnull(null,'Default');
select
name,
(case when age<=19 then '少年' else '老年' end) as '类分'
from employee;
(2) Realization of business needs
create table score(
id int comment 'ID',
name varchar(20) comment '姓名',
math int comment '数学',
english int comment '英语',
chinese int comment '语文'
) comment '学员成绩表';
insert into score values(1,'Tom',67,88,95),(2,'Rose',23,66,90),(3,'Jack',56,98,76);
select
id,
name,
(case when math>=90 then '优秀' when math>=60 then '及格' else '不及格' end) '数学',
(case when english>=90 then '优秀' when english>=60 then '及格' else '不及格' end) '英语',
(case when chinese>=90 then '优秀' when chinese>=60 then '及格' else '不及格' end) '语文'
from score;
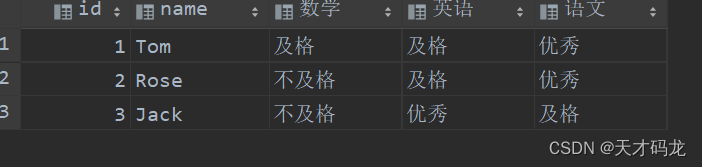