Dynamic proxy is a very useful design pattern in programming, which allows you to create a proxy object at runtime instead of the original object to perform additional logic before and after method calls. In Android development, dynamic proxies can be used for various use cases such asperformance monitoring, AOP (aspect-oriented programming), and event handling. This article will delve into the principles, uses, and practical examples of Android dynamic proxies.
What is a dynamic proxy?
Dynamic proxying is a technique that creates a proxy object in place of the original object to perform additional operations before and after method invocations. Proxy objects usually implement the same interface as the original object, but can add custom behavior. A dynamic proxy is generated at runtime, so it does not need to know the type of the original object at compile time.
The principle of dynamic proxy
The principle of dynamic proxy involves two key parts:
- InvocationHandler: This is an interface, usually implemented by developers. It contains a method
invoke
that is called when the method on the proxy object is called. Within theinvoke
method, you can define logic that is executed before and after the method is called. - Proxy (proxy class): This is a class provided by Java for creating proxy objects. You need to pass a
ClassLoader
, a set of interfaces, and aInvocationHandler
to theProxy.newProxyInstance
method, and it will generate the proxy object.
Here is a sample code that demonstrates how to create a simple dynamic proxy:
import java.lang.reflect.InvocationHandler
import java.lang.reflect.Method
import java.lang.reflect.Proxy
// 接口
interface MyInterface {
fun doSomething()
}
// 实现类
class MyImplementation : MyInterface {
override fun doSomething() {
println("Original method is called.")
}
}
// 调用处理器
class MyInvocationHandler(private val realObject: MyInterface) : InvocationHandler {
override fun invoke(proxy: Any, method: Method, args: Array<Any?>?): Any? {
println("Before method is called.")
val result = method.invoke(realObject, *(args ?: emptyArray()))
println("After method is called.")
return result
}
}
fun main() {
val realObject = MyImplementation()
val proxyObject = Proxy.newProxyInstance(
MyInterface::class.java.classLoader,
arrayOf(MyInterface::class.java),
MyInvocationHandler(realObject)
) as MyInterface
proxyObject.doSomething()
}
Running the above code will output:
Before method is called.
Original method is called.
After method is called.
Here, MyInvocationHandler
intercepts the call to the doSomething
method and adds additional logic before and after the method.
Dynamic proxy in Android
In Android, dynamic proxies are usually implemented using Java'sjava.lang.reflect.Proxy
classes. This class allows you to create a proxy object that implements a specified interface and can intercept calls to interface methods to perform additional logic. In Android development, common uses include performance monitoring, permission checking, logging, and event handling.
The purpose of dynamic proxy
Performance monitoring
You can use dynamic proxies to monitor method execution times to analyze application performance. For example, you can create a performance monitoring agent that records the current time before each method call and then calculates the execution time after the method call.
import android.util.Log
class PerformanceMonitorProxy(private val target: Any) : InvocationHandler {
override fun invoke(proxy: Any, method: Method, args: Array<Any?>?): Any? {
val startTime = System.currentTimeMillis()
val result = method.invoke(target, *(args ?: emptyArray()))
val endTime = System.currentTimeMillis()
val duration = endTime - startTime
Log.d("Performance", "${method.name} took $duration ms to execute.")
return result
}
}
AOP (Aspect Oriented Programming)
Dynamic proxy is also one of the core concepts of AOP. AOP allows you to separate cross-cutting concerns (such as logging, transaction management, and security checks) from business logic to better maintain and extend your code. You can apply these concerns to multiple classes and methods by creating appropriate proxies.
event handling
Android often needs to handle various events on the user interface, such as click events, sliding events, etc. You can use dynamic proxies to simplify event processing code and separate event processing logic from Activity or Fragment to make the code more modular and maintainable.
Practical example
Here is a simple example that demonstrates how to use dynamic proxies in Android to handle click events:
import android.util.Log
import java.lang.reflect.InvocationHandler
import java.lang.reflect.Method
import java.lang.reflect.Proxy
import android.view.View
class ClickHandlerProxy(private val target: View.OnClickListener) : InvocationHandler {
override fun invoke(proxy: Any, method: Method, args: Array<Any?>?): Any? {
if (method.name == "onClick") {
Log.d("ClickHandler", "Click event intercepted.")
// 在事件处理前可以执行自定义逻辑
}
return method.invoke(target, *args.orEmpty())
}
}
// 使用示例
val originalClickListener = View.OnClickListener {
// 原始的点击事件处理逻辑
}
val proxyClickListener = Proxy.newProxyInstance(
originalClickListener::class.java.classLoader,
originalClickListener::class.java.interfaces,
ClickHandlerProxy(originalClickListener)
) as View.OnClickListener
button.setOnClickListener(proxyClickListener)
This way, you can execute custom logic before and after the original click event handling logic without modifying the original OnClickListener implementation.
in conclusion
Dynamic proxies are one of the most powerful tools in Android development, allowing you to add additional behavior without modifying the original object. Dynamic agents are widely used in performance monitoring, AOP and event processing. With a deep understanding of the principles and uses of dynamic proxies, you can better design and maintain Android applications.
Finally, we share a complete set of Android learning materials to give some help to those who want to learn Android!
Applies to:
- Anyone who wants to learn Android development but doesn't know where to start
- Also for anyone who's already started Android development but wants to get better at it
1. Learning routes in all directions of Android
Here is a general roadmap for you to become a better Android developer. Its usefulness is that you can find corresponding learning resources according to the above knowledge points to ensure that you learn more comprehensively. If the following learning route can help everyone become a better Android developer, then my mission will be accomplished:
Including: Android application development, system development, audio and video development, Flutter development, small program development, UI interface, vehicle system development, etc.
2. Learning software
If a worker wants to do his job well, he must first sharpen his tools. Commonly used Android Studio video tutorials for learning Android and the latest Android Studio installation package are here, saving everyone a lot of time.
3. Advanced learning videos
When we are studying, the source code of books is often difficult to understand and difficult to read. At this time, video tutorials are very suitable. With vivid images and practical cases, it is scientific and interesting to learn more conveniently.
4. Practical cases
Optical theory is useless. You must learn to follow along and practice it in order to apply what you have learned to practice. At this time, you can learn from some practical cases.
5. Reading classic books
Reading classic Android books can help readers improve their technical level, broaden their horizons, master core technologies, and improve their ability to solve problems. At the same time, they can also learn from the experiences of others. For readers who want to learn Android development in depth, reading classic Android books is very necessary.
6. Interview materials
We must learn Android to find a high-paying job. The following interview questions are the latest interview materials from first-tier Internet companies such as Alibaba, Tencent, Byte, etc., and Alibaba bosses have given authoritative answers. After finishing this set I believe everyone can find a satisfactory job based on the interview information.
This complete version of the complete set of Android learning materials has been uploaded to CSDN. If you need it, friends can scan the CSDN official certification QR code below on WeChat to get it for free [保证100%免费
]
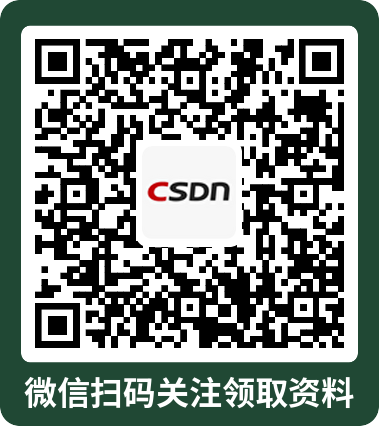