1. Dependence
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.12.0</version>
</dependency>
2. Prerequisite preparation
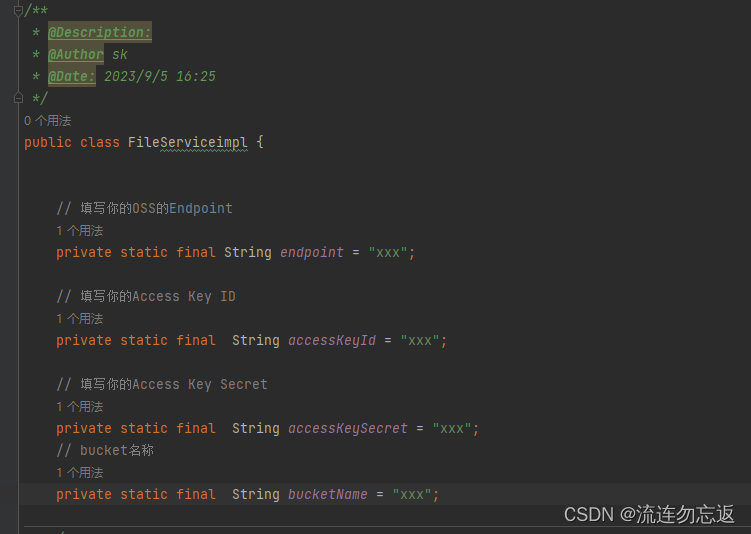
Among them, endpoint and bucketName parameters:
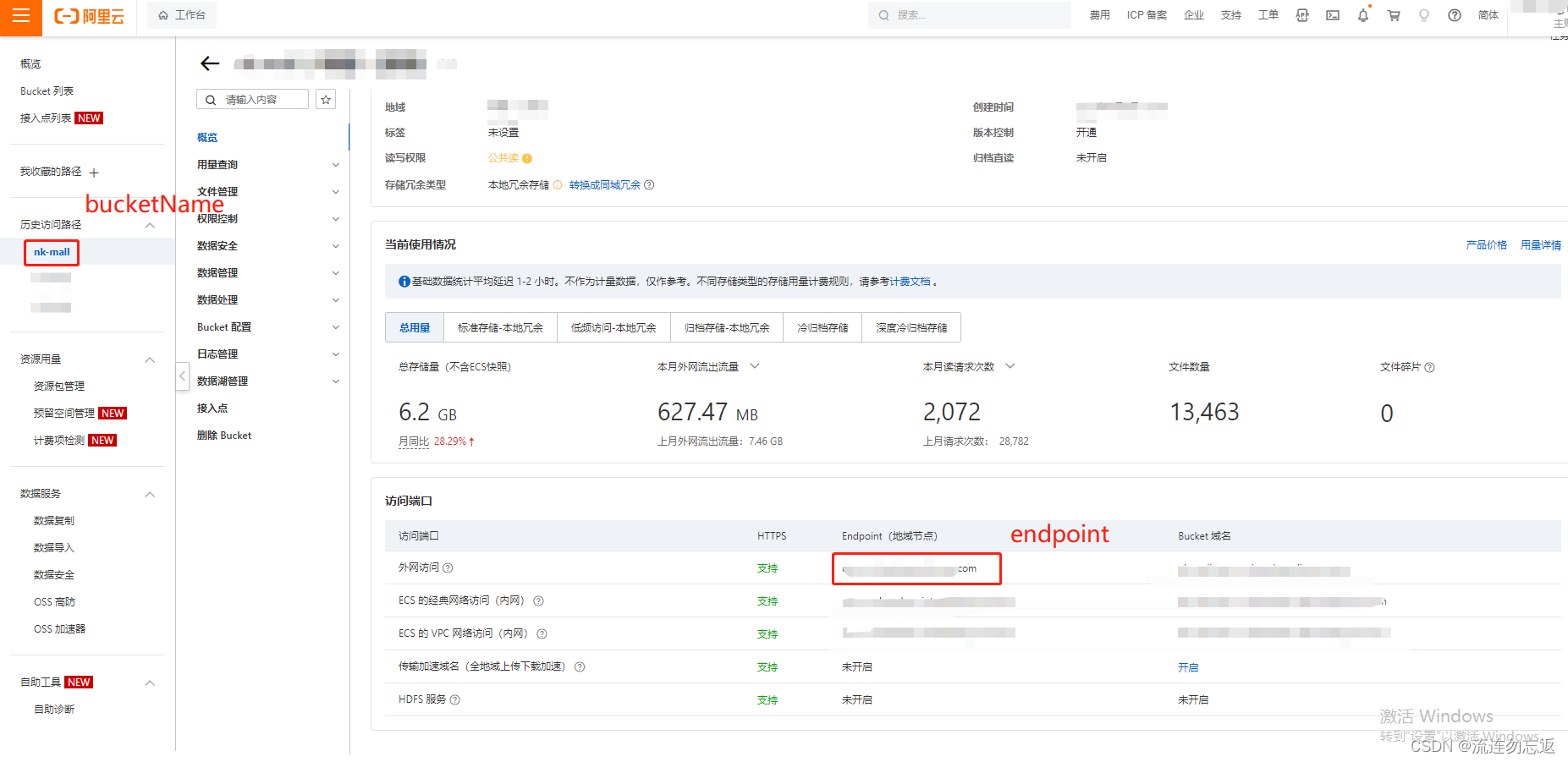
Among them, accessKeyId and accessKeySecret parameters:
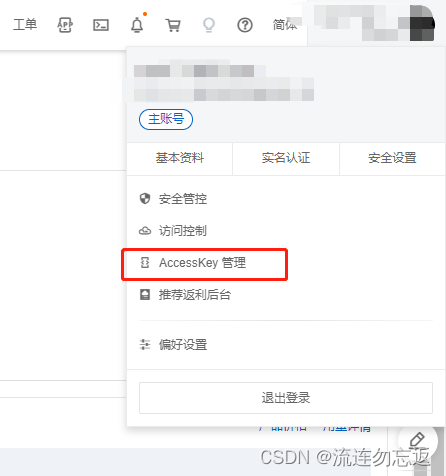
3. Common content types
以下是一些常见的内容类型(Content-Type):
1. 文本文件:
- `text/plain`:纯文本文件,没有特定格式或编码。
- `text/html`:HTML文档,用于网页显示。
- `text/css`:CSS文档,用于定义网页的样式。
- `text/xml`:XML文档,用于存储结构化数据。
2. 图片文件:
- `image/jpeg`:JPEG格式的图像文件。
- `image/png`:PNG格式的图像文件。
- `image/gif`:GIF格式的图像文件。
- `image/svg+xml`:SVG格式的矢量图像文件。
3. 视频文件:
- `video/mp4`:MP4视频文件。
- `video/mpeg`:MPEG格式的视频文件。
- `video/quicktime`:QuickTime视频文件。
- `video/x-ms-wmv`:Windows Media Video文件。
4. 音频文件:
- `audio/mpeg`:MP3音频文件。
- `audio/wav`:WAV音频文件。
- `audio/midi`:MIDI音频文件。
- `audio/ogg`:Ogg Vorbis音频文件。
5. 压缩文件:
- `application/zip`:ZIP压缩文件。
- `application/gzip`:GZIP压缩文件。
- `application/x-rar-compressed`:RAR压缩文件。
- `application/x-tar`:TAR归档文件。
6. 文档文件:
- `application/pdf`:PDF文档。
- `application/msword`:Microsoft Word文档(.doc)。
- `application/vnd.openxmlformats-officedocument.wordprocessingml.document`:Word文档(.docx)。
- `application/vnd.ms-excel`:Microsoft Excel电子表格文件(.xls)。
- `application/vnd.openxmlformats-officedocument.spreadsheetml.sheet`:Excel电子表格文件(.xlsx)。
- `application/vnd.ms-powerpoint`:Microsoft PowerPoint演示文稿(.ppt)。
- `application/vnd.openxmlformats-officedocument.presentationml.presentation`:PowerPoint演示文稿(.pptx)。
4. Directly upload the code
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.model.ObjectMetadata;
import com.aliyun.oss.model.PutObjectRequest;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* @Description:
* @Author sk
* @Date: 2023/9/5 16:25
*/
public class FileServiceimpl {
// 填写你的OSS的Endpoint
private static final String endpoint = "xxx";
// 填写你的Access Key ID
private static final String accessKeyId = "xxx";
// 填写你的Access Key Secret
private static final String accessKeySecret = "xxx";
// bucket名称
private static final String bucketName = "xxx";
/**
* 文件上传oss
* @param inputStream 文件的输入流
* @param contentLength 文件的长度
* @param contentType 文件的类型
* @param keyName 文件的名称
*/
public static String uploadFile(InputStream inputStream, long contentLength, String contentType, String keyName) {
try {
// 简单文件上传, 最大支持 5 GB, 适用于小文件上传, 建议 20M以下的文件使用该接口(超过5G用分片上传)
ObjectMetadata objectMetadata = new ObjectMetadata();
objectMetadata.setContentLength(contentLength);
objectMetadata.setContentType(contentType);
// 对象键(Key)是对象在存储桶中的唯一标识。
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, keyName, inputStream, objectMetadata);
getOSSClient().putObject(putObjectRequest);
// 返回地址
return "https://" + bucketName + "." + endpoint + "/" + keyName;
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
private static OSS getOSSClient() {
return new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
}
public static void main(String[] args) {
try {
FileInputStream fileInputStream = new FileInputStream("D:\\aaa.xlsx");
// String key = "codeFile/a.xlsx"; (这里可以指定把这个文件传到指定的oss文件夹里面,前提是 ‘codeFile’ 这个文件夹在oss中存在)
String key = "codeFile/a.xlsx";
String s = uploadFile(fileInputStream, fileInputStream.available(), "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", key);
System.out.println(s);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
/**
* 判断某个文件在oss是否存在
*/
public static boolean isExitoss(String path)
{
OSS ossClient = getOSSClient();
return ossClient.doesObjectExist(bucketName, path);
}
}