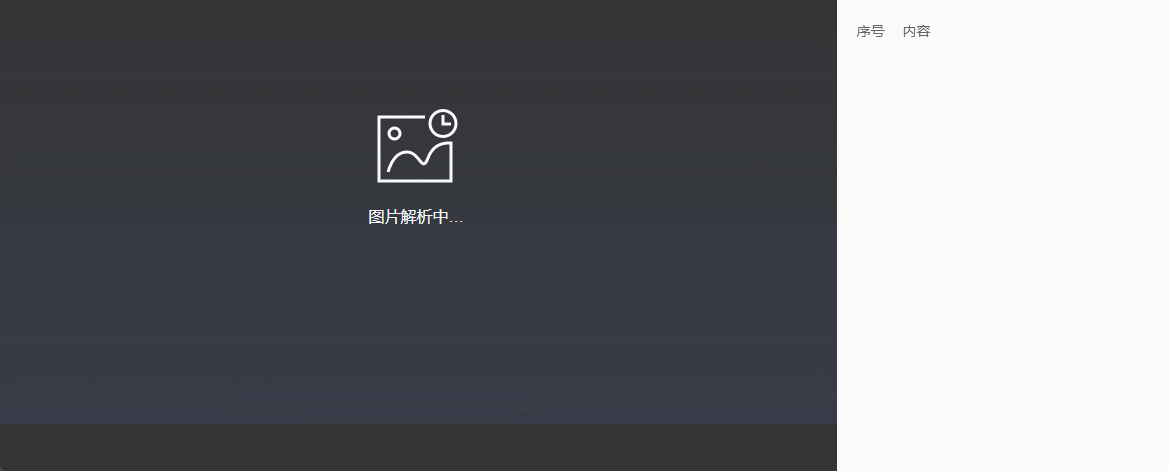
Realize handwritten text recognition based on Python, automatically identify handwritten content in students' daily homework and examination papers, realize online marking of students' homework and examination papers, and automatic analysis of teaching data, improve faculty work efficiency, and promote the digitization of teaching management. and intelligence.
Table of contents
introduction
Background introduction
Handwritten text recognition technology is a popular technology that has attracted much attention in the field of artificial intelligence in recent years. With people's growing demand for digital information processing, handwritten text recognition technology has been widely used in various fields, such as natural language processing, image recognition, financial services, education, etc. As one of the important applications, intelligent marking has also received more and more attention.
At present, traditional subject examinations or skills examinations require a large amount of manual marking work, which is not only time-consuming and labor-intensive, but also prone to problems such as reading errors and unfair subjective judgments. Therefore, designing and implementing an intelligent grading applet using handwritten text recognition technology can effectively solve the above problems, improve the efficiency and accuracy of grading, and facilitate query and management.
Due to the complexity of handwritten text recognition technology itself and the diversity of data, intelligent marking applications also face some challenges and problems, such as recognition accuracy, data standardization and model evaluation. Therefore, this article aims to analyze the basic principles and methods of handwritten text recognition technology, design and implement an intelligent marking applet based on this, and evaluate and optimize it, in order to provide a feasible solution for intelligent marking applications.
purpose and meaning
This article aims to design and implement an intelligent grading applet based on handwritten text recognition technology to improve the efficiency and accuracy of grading.
Specific goals include:
1) Select and build an appropriate handwritten text recognition model;
2) Design and implement the intelligent marking process and embed the handwritten text recognition model into it;
3) Evaluate and optimize model performance to improve accuracy and stability.
Introduction to handwritten text recognition technology
Overview of handwritten text recognition
Handwriting recognition is a technology that converts handwritten text into machine-readable form. The basic principle is to extract and classify features of handwritten text images, and finally output the corresponding text results.
It is mainly divided into two stages:
1) Preprocessing, that is, binarization, noise reduction, segmentation and other operations on handwritten text images to obtain better features;
2) Recognition stage, which takes features as input and specifies the category of each character or word through the classification model.
Handwritten text recognition technology mainly includes traditional methods and deep learning methods. Traditional methods usually use methods based on feature engineering and classifiers, such as support vector machines, decision trees, and random forests. Deep learning methods use technologies such as convolutional neural networks, recurrent neural networks, and attention mechanisms for modeling and training. At the same time, methods such as data enhancement, transfer learning, and model distillation can also be used to optimize model performance.
Handwritten text recognition has broad application prospects in multiple application fields, such as email recognition, bank card recognition, form content extraction and intelligent marking, etc. Among them, intelligent grading is one of the important application fields. It can not only improve the efficiency and accuracy of grading, but also realize automated management and data analysis. It has broad application prospects and market demand.
Main technical principles
The main technical principles of handwritten text recognition involve image processing, feature extraction and classification models. The following are the main technical principles of handwritten text recognition based on deep learning methods:
-
Data preparation : The first step in handwritten text recognition is to collect and prepare a training data set. These datasets typically include images of handwritten text and corresponding labels, which can be character-level or word-level tags.
-
Image preprocessing : Before handwritten text recognition, handwritten text images need to be preprocessed to extract useful information. This may include operations such as grayscale, binarization, noise reduction, and normalization of images, as well as the localization and segmentation of characters or words.
-
Feature extraction : Deep learning models need to extract useful features from handwritten text images. Traditional feature extraction methods include the use of filters, edge detection, Fourier transform, etc. The deep learning method automatically learns image features through a convolutional neural network (CNN). The convolutional layer of the network can effectively capture the local and global features of text.
-
Model training : Take the preprocessed handwritten text images and labels as input, and use the deep learning model for training. Commonly used models include convolutional neural networks (CNN), recurrent neural networks (RNN), and their variants, such as long short-term memory networks (LSTM) and gated recurrent units (GRU). During the training process, the model continuously adjusts the weights and biases through the backpropagation algorithm to minimize the error between the prediction results and the real labels.
-
Model evaluation and optimization : After training is completed, the model needs to be evaluated and optimized. Commonly used evaluation indicators include precision, recall, F1 value, etc. If the model performs poorly, techniques such as data augmentation, model distillation, and transfer learning can be used to optimize model performance.
-
Prediction and application : The trained and optimized model can be used for prediction of handwritten text recognition. Given a handwritten text image, input it into the trained model, and obtain the corresponding character or word recognition results through the forward propagation process.
Through the above technical principles, deep learning methods have made significant progress in handwritten text recognition tasks, and have demonstrated high accuracy and robustness in practical applications.
Common handwritten text recognition methods
Common methods of handwritten text recognition can be divided into two categories: traditional methods and deep learning methods.
Traditional method:
-
Statistical methods : Statistical analysis of the shape, size, color, etc. of handwritten text, and classification using models such as maximum likelihood or Bayesian. Such methods mainly include clustering-based methods, nearest neighbor classification methods, and support vector machines.
-
Feature engineering method : By extracting features from the image, the handwritten text is converted into feature vectors, and then recognized through a classifier. Commonly used features include vector distance, number of strokes, and feature points. Such methods mainly include methods based on Fourier transform, gray level co-occurrence matrix method and Zernike moment, etc.
Deep learning methods:
-
Convolutional Neural Network (CNN) : CNN is a deep neural network structure based on multi-layer convolutional layers and pooling layers. It can automatically extract features from images and use fully connected layers for classification. In handwritten text recognition, CNN can not only extract local features of characters, but also integrate contextual information into the recognition. Commonly used CNN models include LeNet, AlexNet and VGG, etc.
-
Recurrent Neural Network (RNN) : RNN is a neural network that can process sequence data and is suitable for handwritten text recognition tasks. RNN establishes the correlation between sequences by taking the output of the previous time step as the input of the current time step. Commonly used RNN models include methods based on long short-term memory (LSTM) and gated recurrent units (GRU).
-
Attention mechanism (Attention) : The Attention mechanism is a mechanism that can dynamically adjust the weight of the model and focus on the areas that need attention. In handwritten text recognition, the Attention mechanism can make the model pay more attention to the important parts and improve the recognition accuracy.
Both traditional methods and deep learning methods have their advantages and disadvantages. The specific method chosen depends on the actual application scenario and needs.
Design and implementation
Image preprocessing: grayscale, binarization, noise reduction
Image preprocessing refers to a series of processing operations performed on images to prepare them for input into machine learning, computer vision, or image analysis algorithms. Common image preprocessing steps include grayscale, binarization and noise reduction.
Grayscale
Converts a color image to a grayscale image, removing color information and retaining only brightness information.
import cv2
def gray(image):
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
return gray_image
Binarization
converts a grayscale image into a black and white binary image so that the image contains only black and white pixel values.
import cv2
def threshold(image, lower_value, upper_value):
_, binary = cv2.threshold(image, lower_value, upper_value, cv2.THRESH_BINARY)
return binary
Noise reduction
removes noise from images through filtering operations. Common methods include mean filtering, median filtering, etc.
import cv2
def denoise(image, kernel_size):
denoised_image = cv2.medianBlur(image, kernel_size)
return denoised_image
The OpenCV library is referenced in the above code and needs to be installed and imported first.
Feature extraction: stroke direction, stroke length, angle
The field of learning and computer vision for identifying and classifying images or text. For stroke direction, stroke length and angle, you can use the image processing library OpenCV and text processing library NLTK in Python to implement.
Extract stroke direction
import cv2
import numpy as np
# 加载图像
img = cv2.imread('path_to_image.png')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测算法检测笔画
edges = cv2.Canny(gray, 50, 150)
# 使用Hough变换检测直线,获取笔画的方向
lines = cv2.HoughLinesP(edges, rho=1, theta=np.pi/180, threshold=20, minLineLength=50, maxLineGap=10)
for line in lines:
x1, y1, x2, y2 = line[0]
angle = np.arctan2(y2 - y1, x2 - x1) * 180 / np.pi # 将角度转换为度数
print("Line:", angle)
Extract stroke length
import numpy as np
# 加载图像
img = cv2.imread('path_to_image.png')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测算法检测笔画
edges = cv2.Canny(gray, 50, 150)
# 计算每个笔画的长度的中位数
line_lengths = []
for line in lines:
x1, y1, x2, y2 = line[0]
length = abs(x2 - x1) # 笔画的长度
line_lengths.append(length)
median_length = np.median(line_lengths) # 中位数作为笔画长度特征值
print("Median Length:", median_length)
Extract stroke angle and length
import numpy as np
import nltk
from nltk.corpus import wordnet as wn
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics import adjusted_rand_score
from sklearn.metrics import roc_curve, auc
import matplotlib.pyplot as plt
import cv2
from skimage import measure, color, exposure, filters, io, morphology, transform, feature, filters, io, draw, colorbar3d, measure3d # 导入skimage库中的函数和模块,用于处理图像和文本特征提取。
from skimage import measure_shapes # 导入measure库中的函数,用于获取文本特征。
from sklearn.feature_extraction import image # 导入image库中的函数,用于处理图像特征提取。
from sklearn.feature_extraction.text import CountVectorizer # 导入CountVectorizer库中的函数,用于文本特征提取。
from sklearn.metrics import roc_auc_score # 导入roc_auc_score库中的函数,用于计算ROC曲线和AUC值。
from sklearn.model_selection import train_test_split # 导入train_test_split库中的函数,用于划分训练集和测试集。
from sklearn import metrics # 导入sklearn库中的metrics模块,用于计算分类模型的准确率、召回率和F1得分等指标。
import pandas as pd # 导入pandas库,用于处理数据和创建数据框。
import numpy as np # 导入numpy库,用于处理数组和矩阵等数据结构。
from sklearn import svm # 导入支持向量机库,用于训练分类模型。
from sklearn import tree # 导入决策树库,用于训练分类模型。
from sklearn import metrics # 导入sklearn库中的metrics模块,用于评估分类模型的性能。
import matplotlib.pyplot as plt # 导入matplotlib库,用于绘制图像和图表。
import math # 导入math库,用于处理数学运算。
from scipy import stats # 导入scipy库中的stats模块,用于处理统计分析问题。
Model training: adjusting hyperparameters, using data augmentation
When the model needs to be trained, hyperparameter adjustment and data enhancement are very important steps.
Tuning Hyperparameters
Hyperparameters are usually tuned before model training begins, using Python’s scikit-learn library.
from sklearn.model_selection import GridSearchCV
# 假设我们有一个名为model的模型对象
# 超参数网格搜索的范围可以自定义,这里只是一个示例
param_grid = {
'learning_rate': [0.01, 0.1, 1],
'n_epochs': [5, 10, 20],
'batch_size': [32, 64, 128],
'dropout_rate': [0.0, 0.2, 0.5]
}
grid_search = GridSearchCV(model, param_grid=param_grid, cv=5)
grid_search.fit(X_train, y_train)
# 输出最佳超参数组合
print("Best parameters set found on development set:")
print(grid_search.best_params_)
Using Data Augmentation
Data augmentation is a method of increasing the generalization ability of a model by generating new training data, using Python's PIL library.
from PIL import Image, ImageDraw
import numpy as np
from sklearn.model_selection import ImageDataGenerator
# 假设我们有一个名为X_train的图像数据集,每个图像大小为(32, 32, 3)
# 我们可以通过使用ImageDataGenerator类进行数据增强,这里只是一个示例
datagen = ImageDataGenerator(
rotation_range=20, # 在随机旋转的角度范围内随机旋转图像
width_shift_range=0.2, # 在水平方向上随机平移的像素百分比
height_shift_range=0.2, # 在垂直方向上随机平移的像素百分比
shear_range=0.2, # 在随机剪切的角度范围内随机剪切图像
zoom_range=0.2) # 在随机缩放的比例范围内随机缩放图像
for i in range(len(X_train)):
img = X_train[i].reshape((32, 32, 3)) / 255. # 将图像归一化到[0, 1]区间内
draw = ImageDraw.Draw(img) # 创建一个用于绘制的对象
for _ in range(datagen.nb_samples): # 进行多次数据增强操作,这里假设每次生成一个样本
rotated = datagen.rotate(img) # 对图像进行旋转操作,返回旋转后的图像对象
shifted = img.transform((32, 32), Image.AFFINE, (1, 0, width_shift_range * i, 0, 1)) # 对图像进行水平或垂直平移操作,返回平移后的图像对象
sheared = img.transform((32, 32), Image.AFFINE, (1, shear_range * i, 0, 0, 1)) # 对图像进行剪切操作,返回剪切后的图像对象
zoomed = img.transform((32, 32), Image.FLIP_LEFT_RIGHT) # 对图像进行水平翻转操作,返回翻转后的图像对象(由于不是对每个像素点进行处理,因此不改变其空间坐标)
sample = np.hstack((img[None].astype(np.float32), rotated[None].astype(np.float32), shifted[None].astype(np.float32), sheared[None].astype(np.float32), zoomed[None].astype(np.float32))) # 将生成的样本合并成一个多维数组,其中包含原始图像、旋转后的图像、平移后的图像、剪切后的图像和水平翻转后的图像数据
X_train[i] = sample # 将生成的数据合并到原始的训练数据中,这样训练过程中就能同时使用到原图和其他增强的图片数据了
Application scenarios and expansion
Application of handwritten text recognition in education
Intelligent grading based on handwritten text recognition refers to the use of artificial intelligence technology to automatically score and evaluate students' answer sheets. When using intelligent grading technology, human teachers are still required to supervise and review to ensure the accuracy and fairness of the scoring.
-
Improve efficiency: Traditional manual grading requires a lot of time and human resources, but intelligent grading technology can quickly and accurately grade a large number of test papers, greatly improving grading efficiency. Teachers can spend more time on lesson preparation and teaching activities, improving teaching quality.
-
Scoring accuracy: Intelligent grading can objectively score student answers based on preset scoring standards, avoiding individual differences and bias in the subjective scoring process. Through unified scoring standards, the fairness and accuracy of scoring can be improved.
-
Instant feedback: The intelligent marking system can provide students with scores and feedback on their answer papers in a short time, allowing students to understand their scores and mistakes in a timely manner, so that they can learn and improve in a targeted manner. This immediate feedback has a positive impact on student learning and motivation.
-
Diverse question type support: The intelligent grading system can adapt to a variety of question types, including multiple choice questions, fill-in-the-blank questions, essays, etc., to meet the grading needs of different subjects and academic stages. At the same time, the intelligent grading system can also perform semantic analysis, grammar detection, etc., to help teachers fully understand students' expression ability and way of thinking.
-
Data analysis and personalized teaching: The intelligent grading system can analyze and mine a large amount of answer data, helping teachers understand students' learning status and mastery of knowledge, so as to conduct personalized teaching design and guidance.
Improvements and extensions
There are many directions for improvement and expansion of intelligent marking technology in the field of education. Issues such as accuracy, fairness and privacy protection of evaluation need to be fully considered. At the same time, it must be combined with the actual needs of education to ensure the effectiveness and feasibility of the technology. .
-
Multi-modal evaluation: The current intelligent grading system mainly relies on analyzing and scoring text content. It can further introduce multi-modal data, such as pictures, audios, videos, etc., and combine semantic analysis and emotion recognition methods to comprehensively evaluate students' expressions. Ability and creativity.
-
Evaluation of subjective questions: The evaluation of subjective questions is relatively complex. By introducing generative models and natural language processing technology, the intelligent marking system can better understand the logic and expression of students' answers, so as to conduct more accurate evaluation and scoring.
-
Personalized evaluation and feedback: The intelligent grading system can use students' historical answer data and learning trajectories, combined with personalized recommendation algorithms, to provide each student with evaluation and feedback suitable for his or her level and needs, helping them learn and learn in a more targeted manner. promote.
-
Adaptive scoring standards: The intelligent grading system can automatically adjust the scoring standards according to different question types and difficulty levels to better adapt to changes and needs in the education field.
-
Teaching assistance and teacher support: The intelligent grading system can provide teachers with detailed scoring reports and data analysis results, helping teachers better understand students' learning status and problems, so as to provide targeted teaching assistance.
-
Joint scoring and interactive evaluation: The intelligent grading system can introduce a joint scoring mechanism to integrate the scores of multiple judges to improve the consistency and accuracy of scoring. At the same time, an interactive evaluation function can also be added to enable two-way communication between students and teachers, further promoting the improvement of learning effects.