Theoretically, each Vue instance manages its own state: after defining the initial data state, changing the initial state through event actions and driving changes in the view is simple state management, understood through a simple counting component:
<script>
exprot default {
//初始状态:定义一个值为0的数据count
data(){
return{
count:0;
}
},
//行为:通过行为改变了状态
methods:{
increment() {
this.count++
}
}
}
</script>
//视图状态
<template>{count}</template>
The above is the performance in a single page, simple state processing, when the count++ method is called, the value of count in the view will change from 0 to 1. But when multiple components share a state, this single data flow method is not very useful.
Under what circumstances does state management need to be used?
Multiple views rely on the same state
Need to change the same state from different views
For scenario 1, a feasible approach is to "promote" the shared state to a common ancestor component and then pass it down through props. However, doing this in a deep component tree structure can quickly become cumbersome and verbose. This will lead to another problem: Prop level-by-level transparent transmission problem .
For scenario 2, we often find ourselves getting parent/child instances directly via template references, or trying to mutate and synchronize multiple copies of state via triggered events. However, the robustness of these modes is not ideal, and it can easily make the code difficult to maintain.
A simpler and more direct solution is to extract the shared state between components and manage it in a global singleton. In this way, our component tree becomes a large "view", and components at any location can access its state or trigger actions.
In the optional API, reactive data is declared using the data() option. Internally, the return value object of data() will be converted to reactive through the reactive() public API function.
If you have a part of the state that needs to be shared between multiple component instances, you can use reactive() to create a responsive object and import it into multiple components: the following example is to make the value of count change. Components A and B need to change this state at the same time.
Use reactive() to create an initial count
// store.js
import { reactive } from 'vue'
export const store = reactive({
count: 0
})
Create components A and B and introduce the value of count. At this time, the value of count in the view is 0.
<!-- ComponentA.vue -->
<script>
import { store } from './store.js'
export default {
data() {
return {
store
}
}
}
</script>
<template>From A: {
{ store.count }}</template>
Create component B
<!-- ComponentB.vue -->
<script>
import { store } from './store.js'
export default {
data() {
return {
store
}
}
}
</script>
<template>From B: {
{ store.count }}</template>
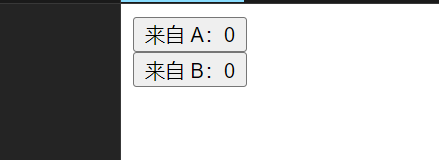
Both component A and component B have introduced the same state count:0 at the same time. Due to the existence of state management, whether you click the button in component A or component B, the value of count will be increased by 1. This is a simple state. In layman's terms, That is, two people receive the same item at the same time.
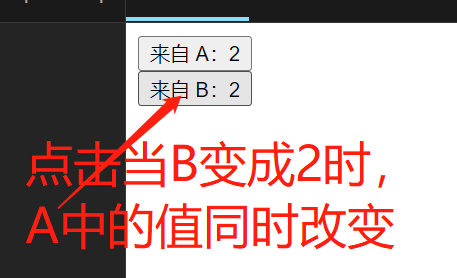