Table of contents
How to use JPA in Spring Boot projects, as follows
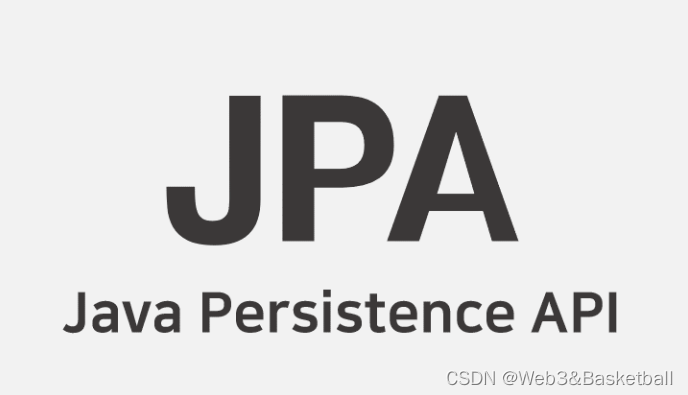
1. Steps to use JPA in Spring Boot project
- Add dependencies Add Spring Boot JPA and database driver dependencies to the
project files.pom.xml
Take MySQL as an example:
<dependencies>
<!-- Spring Boot JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL 驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
- Configure the database Configure the database connection information
in theapplication.properties
orapplication.yml
file. Takeapplication.properties
for example :
spring.datasource.url=jdbc:mysql://localhost:3306/mydb?useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
spring.jpa.hibernate.ddl-auto=update
- Create an entity class
Create an entity class, for exampleUser
:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private Integer age;
// Getters and setters
}
- Create Repository interface
Create anJpaRepository
interface inherited from , for exampleUserRepository
:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.example.demo.model.User;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
- Using the Repository interface
Inject the Repository interface into the Controller class and use it for query operations. For example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.example.demo.model.User;
import com.example.demo.repository.UserRepository;
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
At this point, you have successfully used JPA in your Spring Boot project. When the method UserController
of is called getAllUsers
, all users are queried from the database and returned.
2. Things to note when using JPA in Spring Boot projects
- Make sure you have added Spring Boot JPA and database driver dependencies.
- Make sure the database connection information is configured in the
application.properties
or file.application.yml
- Ensure that the namespace and package structure in the entity class, Repository interface, and Controller class are correct.
- Make sure that the database has been started and the table structure has been created before running the project. When using JPA in a Spring Boot project, you usually use the convenience methods provided by Spring Data JPA. The following are some commonly used JPA syntax:
3. Spring Boot projects use JPA common syntax
- Entity Class
First, you need to create an entity class, egUser
. Use@Entity
the annotation to mark the class as an entity class, and use@Table
the annotation to specify the table name in the database. Add appropriate JPA annotations such as@Id
,@GeneratedValue
and for each field@Column
.
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private Integer age;
// Getters and setters
}
- Repository Interface
Create anJpaRepository
interface that inherits from , for exampleUserRepository
. Spring Data JPA will automatically provide you with basic add, delete, modify and query operations.
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.example.demo.model.User;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
- Query example
In the Controller class, injectUserRepository
the interface and use it for query operations. For example:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.example.demo.model.User;
import com.example.demo.repository.UserRepository;
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
- Query methods
In addition to basic add, delete, modify and query operations, Spring Data JPA also provides some advanced query methods. Here are some common query methods:
findBy
: Find records based on the value of a certain field.findAll
: Query all records.findById
: Search records based on ID.findByExample
: Query records based on instances of entity classes.findAllByExample
: Query all records based on instances of entity classes.findAllByOrderBy
: Sort query records according to the specified field.findAllByPage
: Query records by page.
For example, you can usefindByName
the method to find users based on their username:
public User findByName(String name) {
return userRepository.findByName(name);
}
The above is the basic usage of JPA syntax in Spring Boot projects. In the actual development process, you may need to perform more complex query operations based on specific needs. In this case, it is recommended to consult the official documentation of Spring Data JPA for more information.