Table of contents
- 1. Use Recurrent Neural Network (RNN) or Long Short-term Memory Network (LSTM) in deep learning to generate poems
- 2. Optimization: Use bidirectional LSTM or GRU unit to better capture contextual information
- 3. Optimization: Use Generative Adversarial Networks (GAN) or other techniques to improve the quality and diversity of generated results
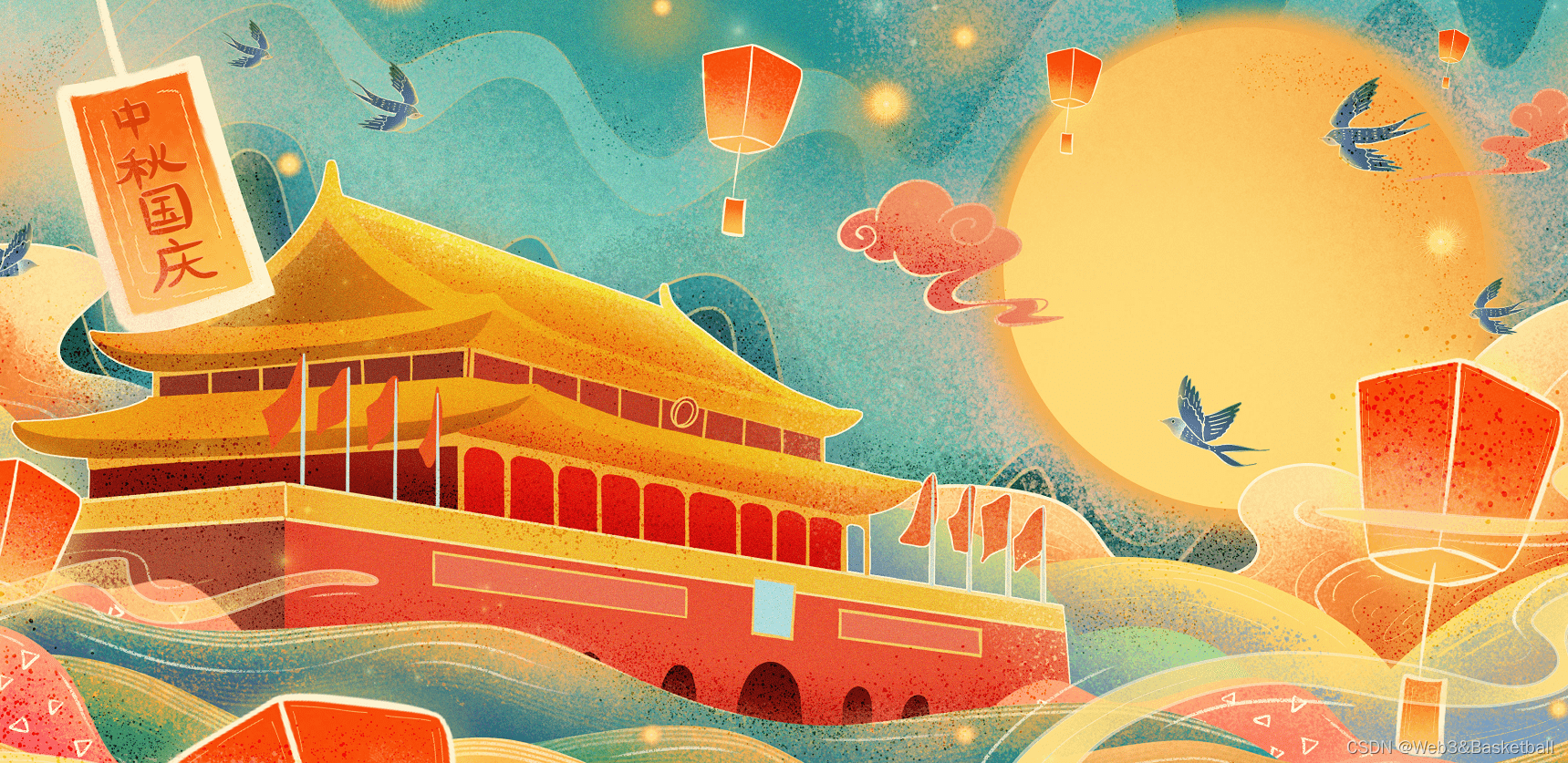
In order to use artificial intelligence technology to generate poetry, we can use recurrent neural network (RNN) or long short-term memory network (LSTM) in deep learning to learn the structure and semantics of poetry. Here is a simple example built using Python and Keras:
1. Use Recurrent Neural Network (RNN) or Long Short-term Memory Network (LSTM) in deep learning to generate poems
- First, we need to install the necessary libraries. In this example, we will use Keras and TensorFlow.
pip install keras tensorflow
- Prepare the data. To create a simple data set, we can use the following four lines of poetry:
明月几时有?把酒问青天。
不知天上宫阙,今夕是何年。
我欲乘风归去,又恐琼楼玉宇,高处不胜寒。
起舞弄清影,何似在人间?
We need to convert these poems into a format suitable for model input. We can treat each Chinese character as a time step and represent each Chinese character using a one-hot encoded integer sequence.
import numpy as np
# 创建一个字符到整数的映射字典
char_to_int = {
char: i for i, char in enumerate(sorted(set(诗词)))}
int_to_char = {
i: char for i, char in enumerate(sorted(set(诗词)))}
# 准备数据
data = [
[char_to_int[char] for char in line.split(',')] for line in 诗词
]
# one-hot 编码
data = np.array(data).astype('float32')
data = np.log(data + 1)
- Define the model. In this example, we will use a simple LSTM model. We divide the input data into batches and use an LSTM layer to process them.
from keras.models import Sequential
from keras.layers import LSTM, Dense
model = Sequential()
model.add(LSTM(128, input_shape=(len(word_index) + 1,)))
model.add(Dense(len(word_index), activation='softmax'))
- Compile the model. We need to specify the optimizer, loss function and evaluation metrics.
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
- Train the model. We will use the first 3 lines of poetry as training data and the 4th line of poetry as test data.
model.fit(data[:-1], data[-1], epochs=10, batch_size=64)
- Generate poetry. Use the trained model to generate the fifth verse.
predicted = np.argmax(model.predict(data[:-1], verbose=0), axis=-1)
predicted = [int_to_char[i] for i in predicted]
generated_poem = ','.join(predicted)
print(generated_poem)
This is a simplified example that you can build upon to improve the quality of your poetry. For example:
- Use bidirectional LSTM or GRU units to better capture contextual information.
- Increase the number of hidden layers and neurons to improve the expression ability of the model.
- Use more sophisticated data preprocessing methods such as word2vec or char2vec to obtain richer vocabulary representations.
- Use generative adversarial networks (GAN) or other techniques when generating poetry to improve the quality and diversity of generated results.
- Use a larger data set for training to improve the model's generalization ability.
Through the above methods, you can improve the quality of poetry generated using artificial intelligence technology. But please note that these methods are not isolated and you can use them in combination for better results. At the same time, more trials and adjustments may be required in actual applications.
2. Optimization: Use bidirectional LSTM or GRU unit to better capture contextual information
In order to use bidirectional LSTM or GRU units to better capture contextual information, we need to modify the previous model definition. Here is an example using a bidirectional LSTM:
- Define the bidirectional LSTM model.
from keras.models import Sequential
from keras.layers import LSTM, Bidirectional
from keras.layers import Dense
model = Sequential()
model.add(Bidirectional(LSTM(64)))
model.add(Dense(len(word_index), activation='softmax'))
In this example, we use a bidirectional LSTM layer, consisting of two independent LSTM layers (one forward and one backward) to better capture contextual information.
2. Compile the model.
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
- Train the model.
model.fit(data[:-1], data[-1], epochs=10, batch_size=64)
- Use the trained model to generate poems.
predicted = np.argmax(model.predict(data[:-1], verbose=0), axis=-1)
predicted = [int_to_char[i] for i in predicted]
generated_poem = ','.join(predicted)
print(generated_poem)
By using bidirectional LSTM units, the model will better understand contextual information, thereby improving the quality of generated poetry. Likewise, you can try other optimization methods, such as increasing the number of hidden layers and neurons, using more complex data preprocessing methods, introducing generative adversarial networks (GAN), etc., to further improve the quality and diversity of generated results.
3. Optimization: Use Generative Adversarial Networks (GAN) or other techniques to improve the quality and diversity of generated results
To use Generative Adversarial Networks (GAN) or other techniques when generating poetry to improve the quality and diversity of generated results, we can modify the original model. Here is an example using GAN:
- Define the generator and discriminator.
from keras.models import Sequential
from keras.layers import Dense, Bidirectional, LSTM, Input
def build_generator(latent_dim):
model = Sequential()
model.add(Input(latent_dim))
model.add(Bidirectional(LSTM(64)))
model.add(Dense(len(word_index), activation='softmax'))
return model
def build_discriminator():
model = Sequential()
model.add(Input(len(word_index)))
model.add(LSTM(64, return_sequences=True))
model.add(LSTM(32))
model.add(Dense(1, activation='sigmoid'))
return model
- Instantiate the generator and discriminator.
generator = build_generator(latent_dim=100)
discriminator = build_discriminator()
- Define GAN training function.
def train_gan(generator, discriminator, data, epochs=100, batch_size=64):
for epoch in range(epochs):
for real_data in data:
# 训练判别器
real_labels = tf.ones((batch_size, 1))
noise = tf.random_normal(0, 1, (batch_size, latent_dim))
fake_data = generator(noise)
fake_labels = tf.zeros((batch_size, 1))
all_data = tf.concat((real_data, fake_data), axis=0)
all_labels = tf.concat((real_labels, fake_labels), axis=0)
discriminator.train_on_batch(all_data, all_labels)
# 训练生成器
noise = tf.random_normal(0, 1, (batch_size, latent_dim))
generator.train_on_batch(noise, real_labels)
print(f'Epoch {
epoch + 1} finished.')
- Training GAN.
latent_dim = 100
data =... # 训练数据
epochs = 100
batch_size = 64
generator = build_generator(latent_dim)
discriminator = build_discriminator()
generator.compile(optimizer='adam', loss='categorical_crossentropy')
discriminator.compile(optimizer='adam', loss='binary_crossentropy')
train_gan(generator, discriminator, data, epochs, batch_size)
By using GAN technology, the model will be able to generate more diverse and high-quality poems during the training process. At the same time, you can also try other techniques, such as using more advanced loss functions, such as WGAN or CycleGAN, to further improve the quality of the generated results.