Configuration log
Introduce the [log4net.config] file under [Photon-OnPremise-Server-SDK_v4-0-29-11263\src-server\Mmo\Photon.MmoDemo.Server] into the project
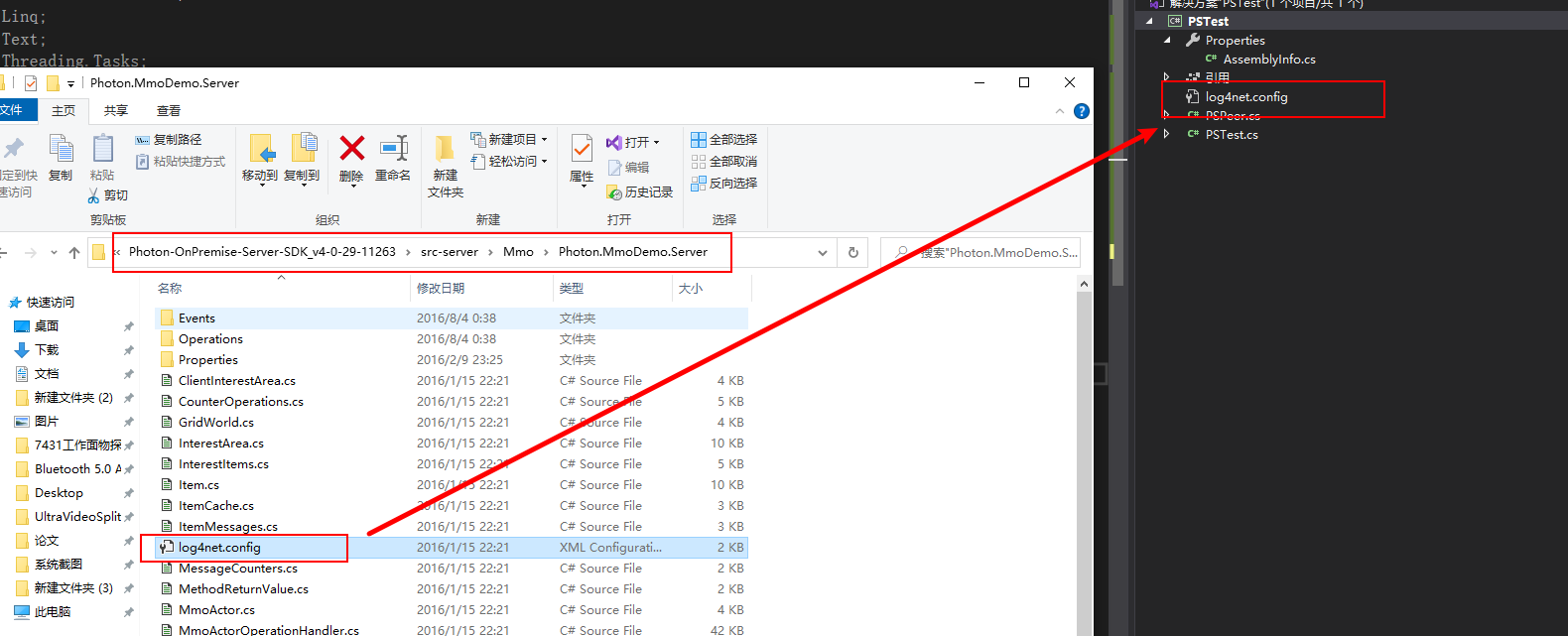
Change its attribute from [Do not copy] to [Always copy]
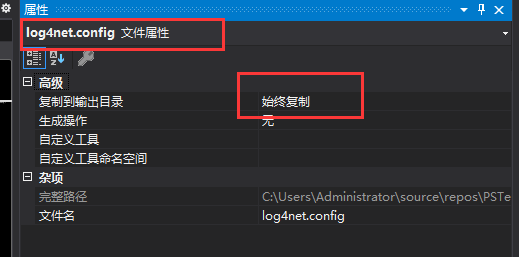
Open the file and modify the file name in the configuration properties to PSTest
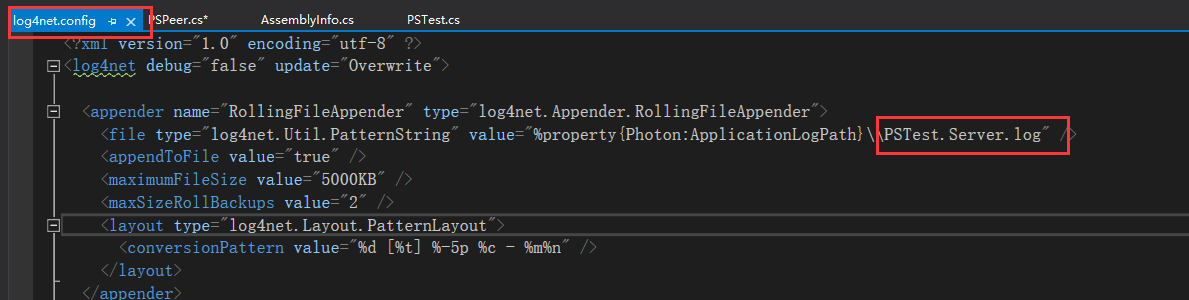
Usage log
Create a log object in the [PSTest] class in PSTest.cs, and configure the log initialization method in the Setup method
public static ILogger log = LogManager.GetCurrentClassLogger();
protected override void Setup()
{
//日志的初始化
log4net.GlobalContext.Properties["Photon:ApplicationLogPath"] = Path.Combine(ApplicationRootPath, "bin_Win64/log");
//对photonserver日志设置为log4net
LogManager.SetLoggerFactory(Log4NetLoggerFactory.Instance);
//让log4net插件读取配置文件
FileInfo file = new FileInfo(Path.Combine(BinaryPath, "log4net.config"));
XmlConfigurator.ConfigureAndWatch(file);
//记入日志
log.Info("初始化完成");
}
In PSPeer.cs, you can receive messages from the client and save them to the log.
//接收到客户端发来的请求
protected override void OnOperationRequest(OperationRequest operationRequest, SendParameters sendParameters)
{
if (operationRequest.OperationCode == 1)
{
Dictionary<byte, object> dic = operationRequest.Parameters;
PSTest.log.Info(dic[1]);
}
}
receive messages
Create an object in unity and mount the following script
Need to add reference
using ExitGames.Client.Photon;
Inherit the class IPhotonPeerListener and generate 4 overloaded methods
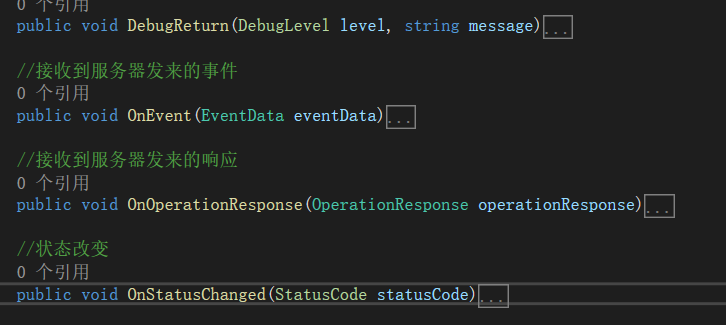
Create singleton and PhotonPeer objects of this class
public static PhotoManager Instance;
public PhotonPeer peer;
Instantiate and connect to the server in Awake. Check the port number in the PhotonServer.config file.
void Awake()
{
Instance = this;
DontDestroyOnLoad(gameObject);
//连接
peer = new PhotonPeer(this,ConnectionProtocol.Tcp);
peer.Connect("127.0.0.1:4530","PSTest");
}
Stay connected during Update and set up to send messages
void Update()
{
peer.Service();
//测试发消息
if (Input.GetKeyDown(KeyCode.Space))
{
//给服务器发消息
Dictionary < byte,object> dic = new Dictionary<byte,object>();
dic.Add(1,"你好,我是王小虎");
peer.OpCustom(1, dic, true);
}
}
Complete code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using ExitGames.Client.Photon;
public class PhotoManager : MonoBehaviour,IPhotonPeerListener
{
public static PhotoManager Instance;
public PhotonPeer peer;
void Awake()
{
Instance = this;
DontDestroyOnLoad(gameObject);
//连接
peer = new PhotonPeer(this,ConnectionProtocol.Tcp);
peer.Connect("127.0.0.1:4530","PSTest");
}
void Update()
{
peer.Service();
//测试发消息
if (Input.GetKeyDown(KeyCode.Space))
{
//给服务器发消息
Dictionary < byte,object> dic = new Dictionary<byte,object>();
dic.Add(1,"你好,我是王小虎");
peer.OpCustom(1, dic, true);
}
}
private void OnDestroy()
{
peer.Disconnect();
}
public void DebugReturn(DebugLevel level, string message)
{
}
//接收到服务器发来的事件
public void OnEvent(EventData eventData)
{
if (eventData.Code == 1)
{
Debug.Log(eventData
.Parameters[1]);
}
}
//接收到服务器发来的响应
public void OnOperationResponse(OperationResponse operationResponse)
{
if (operationResponse.OperationCode == 1)
{
Debug.Log(operationResponse.Parameters[1]);
}
}
//状态改变
public void OnStatusChanged(StatusCode statusCode)
{
if (statusCode == StatusCode.Connect)
{
Debug.Log("链接服务器成功!");
}
}
}
Receive messages in OnOperationRequest in PSPeer.cs
//接收到客户端发来的请求
protected override void OnOperationRequest(OperationRequest operationRequest, SendParameters sendParameters)
{
if (operationRequest.OperationCode == 1)
{
Dictionary<byte, object> dic = operationRequest.Parameters;
PSTest.log.Info(dic[1]);
}
}
Send a message
OnOperationRequest in PSPeer.cs resends the message after receiving it
protected override void OnOperationRequest(OperationRequest operationRequest, SendParameters sendParameters)
{
if (operationRequest.OperationCode == 1)
{
Dictionary<byte, object> dic = operationRequest.Parameters;
//PSTest.log.Info(dic[1]);
//创建一个响应
OperationResponse res = new OperationResponse(1, dic);
SendOperationResponse(res, sendParameters);
//创建一个事件
EventData data = new EventData(1, dic);
SendEvent(data, sendParameters);
}
}
Receive messages in script in unity
//接收到服务器发来的事件
public void OnEvent(EventData eventData)
{
if (eventData.Code == 1)
{
Debug.Log(eventData
.Parameters[1]);
}
}
//接收到服务器发来的响应
public void OnOperationResponse(OperationResponse operationResponse)
{
if (operationResponse.OperationCode == 1)
{
Debug.Log(operationResponse.Parameters[1]);
}
}
//状态改变
public void OnStatusChanged(StatusCode statusCode)
{
if (statusCode == StatusCode.Connect)
{
Debug.Log("链接服务器成功!");
}
}
Complete code
Script for PhotoManager in unity
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using ExitGames.Client.Photon;
public class PhotoManager : MonoBehaviour,IPhotonPeerListener
{
public static PhotoManager Instance;
public PhotonPeer peer;
void Awake()
{
Instance = this;
DontDestroyOnLoad(gameObject);
//连接
peer = new PhotonPeer(this,ConnectionProtocol.Tcp);
peer.Connect("127.0.0.1:4530","PSTest");
}
void Update()
{
peer.Service();
//测试发消息
if (Input.GetKeyDown(KeyCode.Space))
{
//给服务器发消息
Dictionary < byte,object> dic = new Dictionary<byte,object>();
dic.Add(1,"你好,我是王小虎");
peer.OpCustom(1, dic, true);
}
}
private void OnDestroy()
{
peer.Disconnect();
}
public void DebugReturn(DebugLevel level, string message)
{
}
//接收到服务器发来的事件
public void OnEvent(EventData eventData)
{
if (eventData.Code == 1)
{
Debug.Log(eventData
.Parameters[1]);
}
}
//接收到服务器发来的响应
public void OnOperationResponse(OperationResponse operationResponse)
{
if (operationResponse.OperationCode == 1)
{
Debug.Log(operationResponse.Parameters[1]);
}
}
//状态改变
public void OnStatusChanged(StatusCode statusCode)
{
if (statusCode == StatusCode.Connect)
{
Debug.Log("链接服务器成功!");
}
}
}
PSTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Photon.SocketServer;
using ExitGames.Logging;
using ExitGames.Logging.Log4Net;
using log4net.Config;
using System.IO;
namespace PSTest
{
public class PSTest : ApplicationBase
{
public static ILogger log = LogManager.GetCurrentClassLogger();
//有客户端链接会调用
protected override PeerBase CreatePeer(InitRequest initRequest)
{
PSPeer peer = new PSPeer(initRequest);
return peer;
}
protected override void Setup()
{
//日志的初始化
log4net.GlobalContext.Properties["Photon:ApplicationLogPath"] = Path.Combine(ApplicationRootPath, "bin_Win64/log");
//对photonserver日志设置为log4net
LogManager.SetLoggerFactory(Log4NetLoggerFactory.Instance);
//让log4net插件读取配置文件
FileInfo file = new FileInfo(Path.Combine(BinaryPath, "log4net.config"));
XmlConfigurator.ConfigureAndWatch(file);
log.Info("初始化完成");
}
protected override void TearDown()
{
}
}
}
PSPeer.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Photon.SocketServer;
using PhotonHostRuntimeInterfaces;
namespace PSTest
{
public class PSPeer : ClientPeer
{
public PSPeer(InitRequest initRequest) : base(initRequest)
{
}
//断开后调用
protected override void OnDisconnect(DisconnectReason reasonCode, string reasonDetail)
{
}
//接收到客户端发来的请求
protected override void OnOperationRequest(OperationRequest operationRequest, SendParameters sendParameters)
{
if (operationRequest.OperationCode == 1)
{
Dictionary<byte, object> dic = operationRequest.Parameters;
//PSTest.log.Info(dic[1]);
//创建一个响应
// OperationResponse res = new OperationResponse(1, dic);
// SendOperationResponse(res, sendParameters);
//创建一个事件
EventData data = new EventData(1, dic);
SendEvent(data, sendParameters);
}
}
}
}