What is pygame?
Pygame is a Python module specially used to develop games. It is mainly designed for the development and design of 2D electronic games. It is free, open source, supports multiple operating systems, and has good cross-platform capabilities. It provides many operating modules, such as image module (image), sound module (mixer), input/output (mouse, keyboard, display) module, etc. To put it simply, if you use pygame, you can theoretically develop and design all 2D games on the market (only in theory).
1. Installation of pygame module
Install using pip interface
pip install pygame
For a detailed description of the pip interface, please see: https://blog.csdn.net/pengneng123/article/details/129556320
2. Use of basic functions of pygame library
pygame.init() initialization, this function must be called before calling any function of pygame.
pygame.init()
pygame.display.set_mode((400,300)) creates a window and passes in the width and height
screen = pygame.display.set_mode((400,300))
pygame.display.set_caption("xxxx") sets the title name at the top of the window
pygame.display.set_caption("猪了个猪")
Run the above code:
import pygame
pygame.init()
screen = pygame.display.set_mode((400,300))
pygame.display.set_caption("猪了个猪")
Run the above code and you will find that a window appears in the upper left corner and then flashes away. This is normal. Here we need to think about the running of python code. By executing the above code, you create a black window, but what will happen when the code is executed? Of course, python will automatically exit. So you have to use a certain method to prevent the code from ending. This requires adding an "infinite loop". This is easy to understand. while true will run the code inside it over and over again without exiting.
5.while True makes the game loop infinitely
while True: # 死循环确保窗口一直显示
for event in pygame.event.get(): # 遍历所有事件
if event.type == pygame.QUIT: # 如果单击关闭窗口,则退出
sys.exit() #按X 退出
6. Complete code example:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400,300))
pygame.display.set_caption("猪了个猪")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
Output:
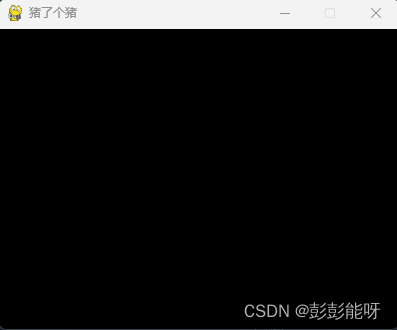
In this way, the most basic framework of a game comes out.
3. Operating pygame
Color and drawing, screen.fill() fills the color
#设置颜色
Black = (0,0,0)
White = (255,255,255)
Red = (255,0,0)
Green = (0,255,0)
Blue = (0,0,255)
screen.fill(White)
2. Draw graphics
###绘制多边形,pylygon(surface,color,pointlist,width)pointlist参数是一个元组或者点的
pygame.draw.polygon(screen,Green,((146,0),(291,106),(236,277),(56,277),(0,106)))
####画线,参数为 surface,color,起始位置,终止位置,粗细
pygame.draw.line(screen,Blue,(60,120),(120,120),4)
#画圆,参数为surface,color,圆心,半径,边粗细(0填充)
pygame.draw.circle(screen,Blue,(300,50),20,0)
#画椭圆,参数为surface,color,边界矩形(以矩形的位置来画椭圆),边粗细
pygame.draw.ellipse(screen,Red,(300,250,40,80),1)
#画矩形,参数为surface,color,矩形(左上角x坐标,左上角y坐标,宽,高),边粗细
pygame.draw.rect(screen,Red,(200,150,100,50))
3.pygame.display.flip() updates all displays
pygame.display.flip()
4.pygame.quit() #Exit pygame
pygame.quit()
Output the above code:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400,300))
color = (0, 0, 0) # 设置颜色
pygame.display.set_caption("猪了个猪")
Black = (0,0,0)
White = (255,255,255)
Red = (255,0,0)
Green = (0,255,0)
Blue = (0,0,255)
screen.fill(White)
###绘制多边形,pylygon(surface,color,pointlist,width)pointlist参数是一个元组或者点的
pygame.draw.polygon(screen,Green,((146,0),(291,106),(236,277),(56,277),(0,106)))
####画线,参数为 surface,color,起始位置,终止位置,粗细
pygame.draw.line(screen,Blue,(60,120),(120,120),4)
#画圆,参数为surface,color,圆心,半径,边粗细(0填充)
pygame.draw.circle(screen,Blue,(300,50),20,0)
#画椭圆,参数为surface,color,边界矩形(以矩形的位置来画椭圆),边粗细
pygame.draw.ellipse(screen,Red,(300,250,40,80),1)
#画矩形,参数为surface,color,矩形(左上角x坐标,左上角y坐标,宽,高),边粗细
pygame.draw.rect(screen,Red,(200,150,100,50))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
exit()
pygame.display.flip() # 更新全部显示
pygame.quit()
Output:
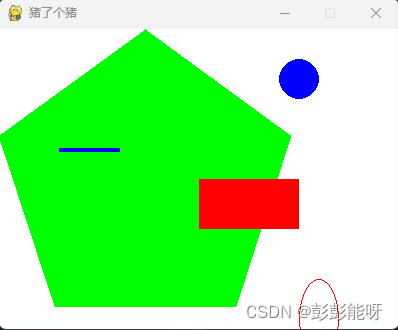
5. Insert the picture into pygame.image.load('xxx.png')
pygame.image.load('1158.png')
6.screen.blit(xx,(0,0))##Write to background
screen.blit(xx,(0,0))##写入到背景
Output:
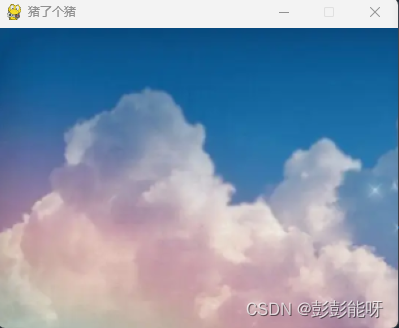
7.pygame.time.Clock() sets the clock
clock = pygame.time.Clock() # 设置时钟
8. Look at a complete code to better understand pygame
import pygame
import time
pygame.init() # 初始化pygame
size = width, height = 480, 700 # 设置窗口大小
screen = pygame.display.set_mode(size) # 显示窗口
pygame.display.set_caption("猪了个猪")
color = (0, 0, 0) # 设置颜色
pig = pygame.image.load('1158.png') # 加载图片
beijing121 = pygame.image.load("gr/147.png")##加载背景图片
beijing131 = pygame.image.load("gr/189.png")##加载背景图片
beijing141 = pygame.image.load("gr/199.png")##加载背景图片
beijing151 = pygame.image.load("gr/200.png")##加载背景图片
pigrect = pig.get_rect() # 获取矩形区域
pigrect1 = beijing121.get_rect() # 获取矩形区域
speed = [1, 1] # 设置移动的X轴、Y轴
speed1 = [80, 80]
speed2 = [160, 160]
speed3 = [200, 120]
speed4 = [120, 260] # 设置移动的X轴、Y轴
clock = pygame.time.Clock() # 设置时钟
def Map():
screen.fill((255,255,255))##填充颜色
screen.blit(background,(0,0))##写入到背景
while True: # 死循环确保窗口一直显示
clock.tick(10) # 每秒执行10次
for event in pygame.event.get(): # 遍历所有事件
if event.type == pygame.QUIT: # 如果单击关闭窗口,则退出
sys.exit()
background = pygame.image.load("beijing1.png")##加载背景图片
Map()
pigrect = pigrect.move(speed) # 移动小猪
pigrect1 = pigrect.move(speed1) # 移动小猪
pigrect2 = pigrect.move(speed2)
pigrect3 = pigrect.move(speed3)
pigrect4 = pigrect.move(speed4)
# 碰到左右边缘
if pigrect.left < 0 or pigrect.right > width:
speed[0] = -speed[0]
# 碰到上下边缘
if pigrectt.top < 0 or pigrect.bottom > height:
speed[1] = -speed[1]
if pigrect1.left < 0 or pigrect1.right > width:
speed[0] = -speed[0]
# 碰到上下边缘
if pigrect1.top < 0 or pigrect1.bottom > height:
speed[1] = -speed[1]
if pigrect2.left < 0 or pigrect2.right > width:
speed[0] = -speed[0]
# 碰到上下边缘
if pigrect2.top < 0 or pigrect2.bottom > height:
speed[1] = -speed[1]
if pigrect3.left < 0 or pigrect3.right > width:
speed[0] = -speed[0]
# 碰到上下边缘
if pigrect3.top < 0 or pigrect3.bottom > height:
speed[1] = -speed[1]
if pigrect4.left < 0 or pigrect4.right > width:
speed[0] = -speed[0]
# 碰到上下边缘
if pigrect4.top < 0 or pigrect4.bottom > height:
speed[1] = -speed[1]
screen.blit(ball, ballrect) # 将图片画到窗口上
screen.blit(beijing121, ballrect1) # 将图片画到窗口上
screen.blit(beijing131, ballrect2) # 将图片画到窗口上
screen.blit(beijing141, ballrect3) # 将图片画到窗口上
screen.blit(beijing151, ballrect4) # 将图片画到窗口上
pygame.display.flip() # 更新全部显示
pygame.quit() # 退出pygame
Final output:
Finally, you can slowly try to realize it based on what you want to do.
@Neng