2. Experimental content Simulate online banking business. When logging in, the user needs to determine the bank card number and bank card password. When the entered card number and password are correct, the login is successful, the currently logged-in account name is prompted, and the user enters the next step to select the operation type. There are four types of operations (deposit: 1, withdrawal: 2, balance: 3, change personal password: 4, exit: 0). When you enter the numbers 1 and 2, the deposit and withdrawal operation will be performed. At this time, you need to enter the amount of the deposit and withdrawal, and perform Correct amount addition and subtraction calculation; when entering the number 3, the balance of the current account is displayed; when the number 4 is entered, the password of the current account can be modified; when the number 0 is entered, the entire system will be exited. Tip: You can use the HashMap collection to store simulated account information, where the key value is used to store the bank card number, and the value value is used to store the entire account object. |
3. Overall design (design principles, design plans and processes, etc.) This part has been deleted following the advice of friends. This code can only be used for reference. 4. Reference code Code for bank subclasses 1 BankAccount /*
original author: jacky Li
Email : [email protected]
Time of completion:2022.12.20
Last edited: 2022.12.20
*/
package bank;
/**
* 银行账户实体类
*
*/
public class BankAccount {
private String name;
private String bankId;
private String password;
private double money;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBankId() {
return bankId;
}
public void setBankId(String bankId) {
this.bankId = bankId;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public double getMoney() {
return money;
}
public void setMoney(double money) {
this.money = money;
}
@Override
public String toString() {
return "账户名:" + name + "\n银行卡号:" + bankId + "\n银行卡密码:" + password + "\n余额:" + money;
}
public BankAccount(String name, String bankId, String password, double money) {
super();
this.name = name;
this.bankId = bankId;
this.password = password;
this.money = money;
}
public BankAccount() {
super();
}
}
About the main body of the project implementation code 2 Main /*
original author: jacky Li
Email : [email protected]
Time of completion:2022.12.20
Last edited: 2022.12.20
*/
package bank;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class Main {
// 储存账户信息
private static Map<String, BankAccount> accounts;
private static Scanner scanner = new Scanner(System.in);
private static BankAccount bankAccount;
/**
* 初始化账户信息
*
* @return 初始化结果
*/
public static boolean init() {
BankAccount account1 = new BankAccount("张三", "11234", "123", 1000.99);
BankAccount account2 = new BankAccount("李四", "22134", "456", 2000);
BankAccount account3 = new BankAccount("王五", "33124", "789", 100.99);
accounts = new HashMap<String, BankAccount>();
accounts.put(account1.getBankId(), account1);
accounts.put(account2.getBankId(), account2);
accounts.put(account3.getBankId(), account3);
// 返回初始化结果
return accounts.isEmpty() ? false : true;
}
/**
* 系统入口
*
* @param args
*/
public static void main(String[] args) {
System.out.println("银行系统正在启动...");
if (init()) {
System.out.println("--系统启动成功--");
System.out.println("输入卡号:");
String id = scanner.next();
System.out.println("输入密码:");
String psw = scanner.next();
if (login(id, psw)) {
// 进入系统
System.out.println("--登录成功--");
menu(bankAccount);
} else {
System.out.println("--登录失败--");
}
} else {
System.out.println("--系统启动失败--");
}
}
/**
* 返回登录结果
*
* @param id
* @param psw
* @return
*/
private static boolean login(String id, String psw) {
bankAccount = accounts.get(id);
return null == bankAccount ? false : true;
}
/**
* 功能菜单
*
* @param account
*/
private static void menu(BankAccount account) {
System.out.println("--当前账户:" + account.getName() + "--");
main: while (true) {
System.out.println("1:存款 2:取款 3:余额 4:修改密码 0:退出");
int item = scanner.nextInt();
switch (item) {
case 0:
scanner.close();
System.out.println("--退出系统--");
break main;
case 1:
System.out.println("--存款系统--");
saveMoney(accounts, account);
break;
case 2:
System.out.println("--取款系统--");
ereduceMoney(accounts, account);
break;
case 3:
System.out.println("--余额系统--");
showMoney(account);
break;
case 4:
System.out.println("--修改密码--");
rePassword(accounts, account);
break;
default:
System.out.println("--错误的指令--");
break;
}
}
}
/**
* 修改密码
*
* @param accounts2
* @param account
*/
private static void rePassword(Map<String, BankAccount> accs, BankAccount account) {
System.out.println("输入旧的密码:");
String oldPsw = scanner.next();
if (oldPsw.equals(account.getPassword())) {
System.out.println("输入新的密码:");
String newPsw = scanner.next();
account.setPassword(newPsw);
accs.put(account.getBankId(), account);
System.out.println("--修改成功--");
} else {
System.out.println("--旧密码错误--");
}
}
private static void showMoney(BankAccount account) {
System.out.println(account);
}
/**
* 取款
*
* @param accounts2
* @param account
*/
private static void ereduceMoney(Map<String, BankAccount> accounts2, BankAccount account) {
System.out.println("输入取款金额:");
double money = scanner.nextDouble();
double now = account.getMoney();
if (money <= now) {
account.setMoney(now - money);
accounts2.put(account.getBankId(), account);
System.out.println("--取款成功--");
} else {
System.out.println("--余额不足--");
}
}
private static void saveMoney(Map<String, BankAccount> accounts2, BankAccount account) {
System.out.println("输入存入金额:");
double money = scanner.nextDouble();
account.setMoney(account.getMoney() + money);
accounts2.put(account.getBankId(), account);
System.out.println("--存款成功--");
}
}
5. Operation effect 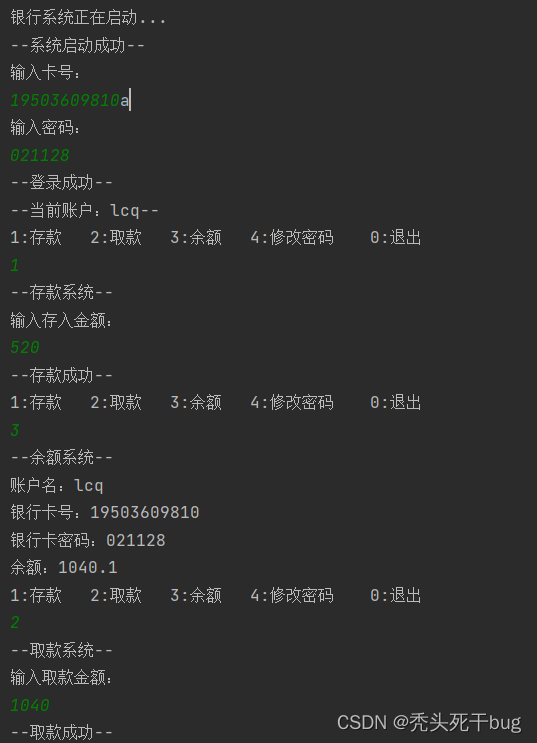 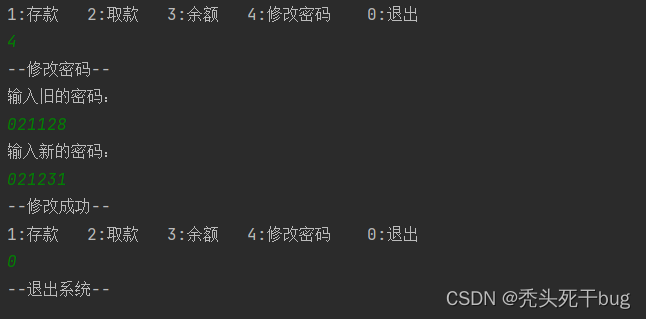 The author has something to say
If you feel that what the blogger said is useful to you, please click "Follow" to support it. We will continue to update such issues...
|