Table of contents
1. Introduction to steering gear
3 items: The steering gear rotates back and forth
4 Project 2: Light-controlled steering gear
4.1 Hardware circuit connection
5 Project 3: Serial port control servo and built-in LED light
6 Explanation of functions used
1. Introduction to steering gear
The servo is a position (angle) servo driver. Steering gear is just a popular name, and its essence is a servo motor. It is widely used in control systems where the angle needs to be constantly changing and maintained. Such as remote control robots, aircraft models, etc.
The rotation angle of the steering gear is 0~180°, and its internal structure includes three parts: motor, control circuit and mechanical structure. The motor has three wires leading out, which are connected to VCC, GNG and signal wires respectively. There are two main pinout formats:
- Brown, red, orange (brown connects to GND, red connects to VCC, and orange connects to signal);
- Red, black, yellow (red is connected to VCC, black is connected to GND, and yellow is connected to the signal).
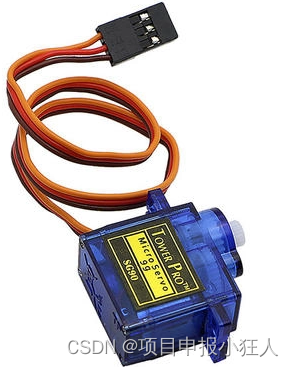
2 Hardware circuit connection
Arduino |
Function | steering gear | Function |
VCC | positive electrode | red | positive electrode |
GND | negative electrode | brown | negative electrode |
D9(PWM) | Digital pin (PWM) | Orange (signal transmission) | signal input |
3 items: The steering gear rotates back and forth
Implementation function: The steering gear rotates back and forth 0~180°.
3.1 Control code
#include <Servo.h> //加载文件库
int pos = 0;
Servo myservo;
void setup()
{
myservo.attach(9, 500, 2500); //修正脉冲宽度
}
void loop()
{
for (pos = 0; pos <= 180; pos += 1) { //pos+=1等价于pos=pos+1
myservo.write(pos);
delay(15);
}
for (pos = 180; pos >= 0; pos -= 1) {
myservo.write(pos);
delay(15);
}
}
3.2 Simulation results
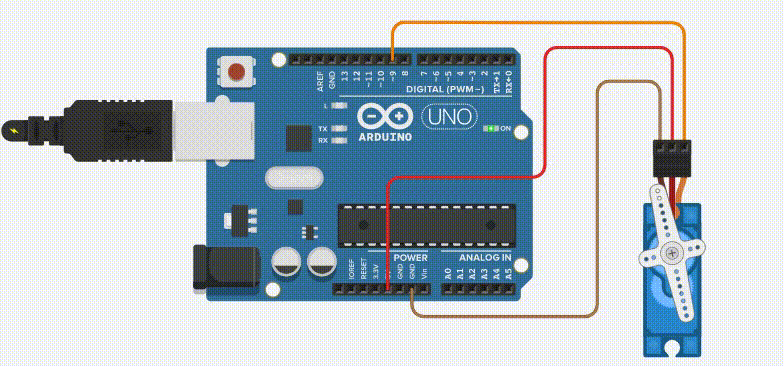
4 Project 2: Light-controlled steering gear
Implementation function: As the light intensity increases, the servo rotates accordingly. When the analog value generated by A0 is greater than 500, the built-in 13-pin programmable LED emits light.
4.1 Hardware circuit connection
Arduino |
Function | steering gear | Function |
VCC | positive electrode | red | positive electrode |
GND | negative electrode | brown | negative electrode |
D9(PWM) | Digital pin (PWM) | Orange (signal transmission) | signal input |
A0 | Analog interface (photoresistor) |
4.2 Control code
#include <Servo.h>
const int sensorPin = A0;
int led=13;
int pos = 0;
Servo myservo;//创建舵机对象
void setup(){
myservo.attach(9, 500, 2500);
pinMode(led,OUTPUT);
Serial.begin(9600);
}
void loop(){
int val=analogRead(sensorPin);
if(val>500){
digitalWrite(led,HIGH);
}
else{
digitalWrite(led,LOW);
}
int yp=map(val,0,1023,0,180);
//数值转换,将[0,1023]产生的模拟值转换成[0,180]中的值
Serial.println(yp);
myservo.write(yp);
delay(10);
}
4.3 Simulation results
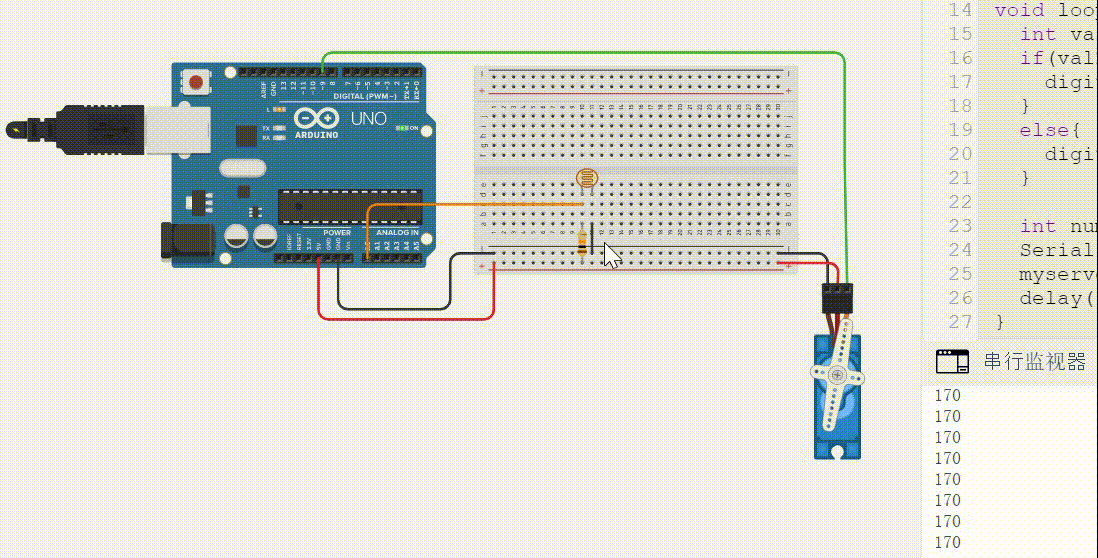
5 Project 3: Serial port control servo and built-in LED light
The implementation functions are as follows:
When the serial port inputs 2, the LED lights up, and the serial port prints "ON" at the same time, and the servo rotates to 90°.
When the serial port inputs 4, the LED goes out, and the serial port prints "OFF" at the same time, and the servo rotates to 180°.
#include <Servo.h>
int led4=4;
int led=13;
Servo myservo;//创建舵机对象
void setup(){
myservo.attach(9, 500, 2500);
pinMode(led,OUTPUT);
pinMode(led4,OUTPUT);
Serial.begin(9600);
}
void loop(){
if(Serial.available()>0){
char c=Serial.read();
if(c=='2'){
digitalWrite(led,HIGH);
digitalWrite(led4,HIGH);
myservo.write(90);
Serial.println("ON");
}
else if(c=='4'){
digitalWrite(led,LOW);
digitalWrite(led4,LOW);
myservo.write(180);
Serial.println("OFF");
}
}
}
5.1 Simulation results
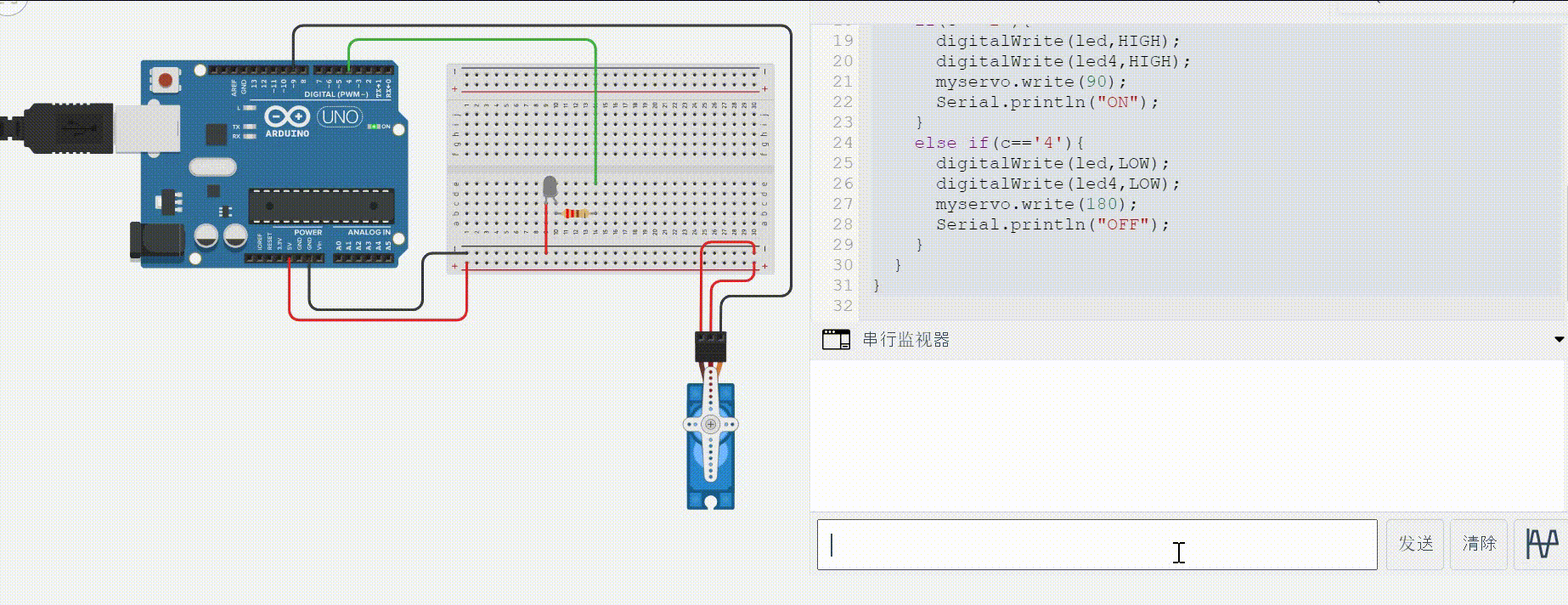
6 Explanation of functions used
- Serial.begin() is the serial port initialization function, which sets the transmission rate.
- The difference between Serial.println() and Serial.print() is that the former has a line break function, while the latter does not.
- Servo.h is the servo library file, which can be loaded directly in Aruidno IDE.
- Servo myservo is a created object, which is essentially a name. Myservo can be named by itself.
- myservo.attach(9) represents the pin that controls the servo.
- myservo.write(45) means that the servo rotates to a position of 45°, rather than rotating 45°.
- The map() function is highly used to convert values in a certain range into values in another range.
The syntax format is: val =map(value, fromLow, fromHigh, toLow, toHigh);
Val and value are variables of the same type, fromHigh and fromLow are the maximum and minimum values of variable a, toHigh and toLow are the maximum and minimum values of val variable. The map() function can scale the value of a certain interval to the value of another interval according to the range ratio, and assign the value to val.